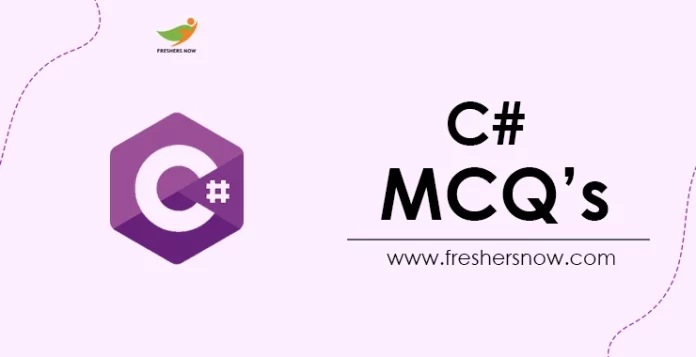
C# MCQs and Answers With Explanation – C# is a powerful and widely used object-oriented programming language developed by Microsoft. It is commonly used for building desktop applications, web applications, games, and mobile apps. C# is known for its simplicity, scalability, and versatility, making it a popular choice for developers across various domains. Furthermore, to increase your confidence for appearing in interviews or placement exams, you can utilize the Top 40 C# Multiple Choice Questions included in this article.
C# MCQ Questions
The C# MCQs with Answers offers a comprehensive overview of the key concepts and functionalities of the language, providing developers with an opportunity to improve their skills and knowledge. With the increasing demand for C# skills in the IT industry, mastering this language is essential for any aspiring developer looking to build high-quality and scalable applications. By scrolling down to the next sections you can find the C# Online Quiz in question and answer format. Answer these C# MCQ Questions and know where you need to do better so that you can gain complete knowledge on C#.
C# Multiple Choice Questions
Name | C# |
Exam Type | MCQ (Multiple Choice Questions) |
Category | Technical Quiz |
Mode of Quiz | Online |
Top 40 C# Quiz Questions | Practice Online Quiz
1. Which of the following is not a primitive data type in C#?
a. char
b. float
c. int
d. string
Answer: d. string
Explanation: string is not a primitive data type in C#. It is a reference type.
2. Which of the following operators is used to create a new instance of a class?
a. new
b. void
c. return
d. break
Answer: a. new
Explanation: The new operator is used to create a new instance of a class.
3. What is the output of the following code?
int x = 5;
Console.WriteLine(x++);
a. 4
b. 5
c. 6
d. 7
Answer: b. 5
Explanation: The postfix increment operator (++) increments the value of x after it has been printed.
4. Which of the following statements is true about inheritance in C#?
a. A derived class can inherit from multiple base classes.
b. A base class can inherit from multiple derived classes.
c. A derived class can inherit from only one base class.
d. A base class can inherit from only one derived class.
Answer: c. A derived class can inherit from only one base class.
Explanation: Multiple inheritance is not supported in C#.
5. Which of the following access modifiers allows a member to be accessed from within the same class and any derived classes?
a. public
b. protected
c. private
d. internal
Answer: b. protected
Explanation: The protected access modifier allows a member to be accessed from within the same class and any derived classes.
6. Which of the following statements is true about the using directive in C#?
a. It is used to declare a variable.
b. It is used to include a namespace in a program.
c. It is used to declare a method.
d. It is used to declare a class.
Answer: b. It is used to include a namespace in a program.
Explanation: The using directive is used to include a namespace in a program.
7. What is the output of the following code?
string[] names = {“John”, “Mary”, “Tom”};
Console.WriteLine(names[1]);
a. John
b. Mary
c. Tom
d. Compiler error
Answer: b. Mary
Explanation: The element at index 1 in the names array is “Mary”.
8. Which of the following statements is true about the static keyword in C#?
a. It is used to declare a class.
b. It is used to declare a method.
c. It is used to declare a variable or member that belongs to a class rather than to instances of the class.
d. It is used to declare a variable or member that belongs to instances of the class rather than to the class itself.
Answer: c. It is used to declare a variable or member that belongs to a class rather than to instances of the class.
Explanation: The static keyword is used to declare a variable or member that belongs to a class rather than to instances of the class.
9. What is the output of the following code?
for (int i = 0; i < 5; i++)
{
Console.WriteLine(i);
}
a. 0 1 2 3 4
b. 1 2 3 4 5
c. 5 4 3 2 1
d. Compiler error
Answer: a. 0 1 2 3 4
Explanation: The loop iterates 5 times and outputs the value of i on each iteration.
10. Which of the following statements is true about the finally block in a try-catch-finally statement?
a. It is executed if an exception is thrown.
b. It is executed before the try block.
c. It is executed before the catch block.
d. It is always executed, regardless of whether an exception is thrown or not.
Answer: d. It is always executed, regardless of whether an exception is thrown or not.
Explanation: The finally block is always executed, regardless of whether an exception is thrown or not.
11. Which of the following statements is true about the out keyword in C#?
a. It is used to pass a variable by reference.
b. It is used to pass a variable by value.
c. It is used to specify a default value for a parameter.
d. It is used to indicate that a parameter is optional.
Answer: a. It is used to pass a variable by reference.
Explanation: The out keyword is used to pass a variable by reference.
12. What is the output of the following code?
int x = 5;
int y = x + 3;
Console.WriteLine(y);
a. 2
b. 3
c. 5
d. 8
Answer: d. 8
Explanation: The value of y is the sum of x and 3, which is 8.
13. Which of the following statements is true about the ref keyword in C#?
a. It is used to pass a variable by reference.
b. It is used to pass a variable by value.
c. It is used to specify a default value for a parameter.
d. It is used to indicate that a parameter is optional.
Answer: a. It is used to pass a variable by reference.
Explanation: The ref keyword is used to pass a variable by reference.
14. What is the output of the following code?
int x = 5;
if (x > 3 && x < 7)
{
Console.WriteLine(“x is between 3 and 7”);
}
a. x is between 3 and 7
b. x is greater than 3
c. x is less than 7
d. Nothing is printed
Answer: a. x is between 3 and 7
Explanation: The condition x > 3 && x < 7 is true, so the message “x is between 3 and 7” is printed.
15. Which of the following statements is true about the sealed keyword in C#?
a. It is used to prevent a class from being inherited.
b. It is used to prevent a method from being overridden.
c. It is used to prevent a variable from being changed.
d. It is used to prevent a variable from being accessed outside the class.
Answer: b. It is used to prevent a method from being overridden.
Explanation: The sealed keyword is used to prevent a method from being overridden.
16. What is the output of the following code?
int[] numbers = {1, 2, 3, 4, 5};
foreach (int number in numbers)
{
Console.WriteLine(number);
}
a. 1 2 3 4 5
b. 5 4 3 2 1
c. Compiler error
d. Nothing is printed
Answer: a. 1 2 3 4 5
Explanation: The foreach loop iterates over each element in the numbers array and outputs its value.
17. Which of the following statements is true about the virtual keyword in C#?
a. It is used to prevent a class from being inherited.
b. It is used to prevent a method from being overridden.
c. It is used to allow a method to be overridden in a derived class.
d. It is used to specify a default value for a parameter.
Answer: c. It is used to allow a method to be overridden in a derived class.
Explanation: The virtual keyword is used to allow a method to be overridden in a derived class.
18. What is the output of the following code?
int x = 5;
if (x > 10)
{
Console.WriteLine(“x is greater than 10”);
}
else
{
Console.WriteLine(“x is less than or equal to 10”);
}
a. x is greater than 10
b. x is less than or equal to 10
c. Compiler error
d. Nothing is printed
Answer: b. x is less than or equal to 10
Explanation: The condition x > 10 is false, so the else block is executed and the message “x is less than or equal to 10” is printed.
19. Which of the following statements is true about the this keyword in C#?
a. It is used to refer to the current instance of a class.
b. It is used to refer to a static member of a class.
c. It is used to refer to a base class.
d. It is used to refer to a derived class.
Answer: a. It is used to refer to the current instance of a class.
Explanation: The this keyword is used to refer to the current instance of a class.
20. What is the output of the following code?
int x = 5;
int y = 10;
if (x > y)
{
Console.WriteLine(“x is greater than y”);
}
else if (y > x)
{
Console.WriteLine(“y is greater than x”);
}
else
{
Console.WriteLine(“x and y are equal”);
}
a. x is greater than y
b. y is greater than x
c. x and y are equal
d. Compiler error
Answer: b. y is greater than x
Explanation: The condition y > x is true, so the message “y is greater than x” is printed.
21. Which of the following statements is true about the abstract keyword in C#?
a. It is used to prevent a class from being inherited.
b. It is used to prevent a method from being overridden.
c. It is used to mark a class as incomplete and force derived classes to implement certain methods.
d. It is used to specify a default value for a parameter.
Answer: c. It is used to mark a class as incomplete and force derived classes to implement certain methods.
Explanation: The abstract keyword is used to mark a class as incomplete and force derived classes to implement certain methods.
22. What is the output of the following code?
int i = 0;
while (i < 5)
{
Console.WriteLine(i);
i++;
}
a. 0 1 2 3 4
b. 4 3 2 1 0
c. Compiler error
d. Nothing is printed
Answer: a. 0 1 2 3 4
Explanation: The while loop iterates while the condition i < 5 is true, outputting the value of i and incrementing it by 1 on each iteration.
23. Which of the following statements is true about the using keyword in C#?
a. It is used to define a new class.
b. It is used to create a new object.
c. It is used to import a namespace.
d. It is used to define a new variable.
Answer: c. It is used to import a namespace.
Explanation: The using keyword is used to import a namespace.
24. Which of the following statements is true about the try-catch-finally block in C#?
a. It is used to catch exceptions that occur during program execution.
b. It is used to define a new class.
c. It is used to create a new object.
d. It is used to define a new variable.
Answer: a. It is used to catch exceptions that occur during program execution.
Explanation: The try-catch-finally block is used to catch exceptions that occur during program execution.
25. What is the output of the following code?
int x = 5;
switch (x)
{
case 1:
Console.WriteLine(“x is 1”);
break;
case 2:
Console.WriteLine(“x is 2”);
break;
default:
Console.WriteLine(“x is not 1 or 2”);
break;
}
a. x is 1
b. x is 2
c. x is not 1 or 2
d. Compiler error
Answer: c. x is not 1 or 2
Explanation: Since x is not equal to 1 or 2, the default case is executed and the message “x is not 1 or 2” is printed.
26. Which of the following statements is true about the ternary operator in C#?
a. It is used to define a new class.
b. It is used to create a new object.
c. It is used to define a new variable.
d. It is used to evaluate a Boolean expression and return one of two values based on the result.
Answer: d. It is used to evaluate a Boolean expression and return one of two values based on the result.
Explanation: The ternary operator is a shorthand way to evaluate a Boolean expression and return one of two values based on the result.
25. What is the output of the following code?
int x = 5;
int y = 3;
int z = x + y;
Console.WriteLine(z);
a. 8
b. 15
c. 53
d. Compiler error
Answer: a. 8
Explanation: The value of z is computed as the sum of x and y, which is 8, and then printed to the console.
26. Which of the following statements is true about arrays in C#?
a. The size of an array cannot be changed after it is created.
b. Arrays can only store elements of the same data type.
c. The index of the first element in an array is always 0.
d. All of the above
Answer: d. All of the above
Explanation: The size of an array cannot be changed after it is created, arrays can only store elements of the same data type, and the index of the first element in an array is always 0.
27. What is the output of the following code?
int x = 5;
if (x == 5)
{
Console.WriteLine(“x is 5”);
}
else if (x == 4)
{
Console.WriteLine(“x is 4”);
}
else
{
Console.WriteLine(“x is not 4 or 5”);
}
a. x is 5
b. x is 4
c. x is not 4 or 5
d. Compiler error
Answer: a. x is 5
Explanation: The condition x == 5 is true, so the message “x is 5” is printed to the console.
28. Which of the following statements is true about abstract classes in C#?
a. An abstract class cannot be instantiated.
b. An abstract class must contain at least one abstract method.
c. An abstract class can be inherited by a non-abstract class.
d. All of the above
Answer: d. All of the above
Explanation: An abstract class cannot be instantiated, must contain at least one abstract method, and can be inherited by a non-abstract class.
29. What is the output of the following code?
int x = 5;
while (x > 0)
{
Console.WriteLine(x);
x–;
}
a. 5 4 3 2 1
b. 1 2 3 4 5
c. 0
d. Compiler error
Answer: a. 5 4 3 2 1
Explanation: The while loop executes as long as the condition x > 0 is true, so the values of x from 5 to 1 are printed to the console. When x becomes 0, the loop terminates.
30. Which of the following statements is true about exception handling in C#?
a. Exception handling is used to handle errors that occur during program execution.
b. Exception handling is performed using try-catch blocks.
c. The catch block must be placed before the try block.
d. All of the above
Answer: d. All of the above
Explanation: Exception handling is used to handle errors that occur during program execution, and is performed using try-catch blocks. The catch block must be placed after the try block in order to catch any exceptions that are thrown within the try block.
31. What is the output of the following code?
int[] arr = {1, 2, 3, 4, 5};
foreach (int i in arr)
{
Console.WriteLine(i);
}
a. 1 2 3 4 5
b. 5 4 3 2 1
c. Compiler error
d. None of the above
Answer: a. 1 2 3 4 5
Explanation: The foreach loop iterates over each element in the array and assigns it to the variable i, which is then printed to the console. The output is therefore the values of the array elements, in order: 1 2 3 4 5.
32. Which of the following statements is true about delegates in C#?
a. A delegate is a type that represents a method signature.
b. A delegate can be used to pass methods as arguments to other methods.
c. A delegate can be used to define event handlers.
d. All of the above
Answer: d. All of the above
Explanation: A delegate is a type that represents a method signature, and can be used to pass methods as arguments to other methods, and to define event handlers.
33. What is the output of the following code?
string s1 = “hello”;
string s2 = “world”;
Console.WriteLine(s1 + ” ” + s2);
a. hello world
b. helloworld
c. “s1 s2”
d. Compiler error
Answer: a. hello world
Explanation: The + operator is used to concatenate the strings s1 and s2, separated by a space, and the resulting string “hello world” is printed to the console.
34. Which of the following statements is true about interfaces in C#?
a. An interface defines a contract for a class to implement.
b. An interface can contain method signatures, properties, events, and indexers.
c. A class can implement multiple interfaces.
d. All of the above
Answer: d. All of the above
Explanation: An interface defines a contract for a class to implement, and can contain method signatures, properties, events, and indexers. A class can implement multiple interfaces.
35. Which of the following statements is true about lambda expressions in C#?
a. A lambda expression is an anonymous function.
b. A lambda expression can be used to create delegates or expression trees.
c. A lambda expression can capture variables from the enclosing scope.
d. All of the above
Answer: d. All of the above
Explanation: A lambda expression is an anonymous function that can be used to create delegates or expression trees, and can capture variables from the enclosing scope.
36. What is the output of the following code?
int i = 5;
if (i < 10)
{
Console.WriteLine(“i is less than 10”);
}
else
{
Console.WriteLine(“i is greater than or equal to 10”);
}
a. i is less than 10
b. i is greater than or equal to 10
c. Compiler error
d. None of the above
Answer: a. i is less than 10
Explanation: The if statement tests whether the condition i < 10 is true, which it is in this case. Therefore, the first branch of the if statement is executed, which prints “i is less than 10” to the console.
37. Which of the following statements is true about properties in C#?
a. A property is a member of a class that provides access to a private field.
b. A property can have a get accessor and a set accessor.
c. A property can be read-only or write-only.
d. All of the above
Answer: d. All of the above
Explanation: A property is a member of a class that provides access to a private field, and can have a get accessor and a set accessor. A property can also be read-only or write-only.
38. What is the output of the following code?
int i = 5;
switch (i)
{
case 1:
Console.WriteLine(“i is 1”);
break;
case 2:
Console.WriteLine(“i is 2”);
break;
default:
Console.WriteLine(“i is not 1 or 2”);
break;
}
a. i is 1
b. i is 2
c. i is not 1 or 2
d. Compiler error
Answer: c. i is not 1 or 2
Explanation: The switch statement tests the value of i against the cases 1 and 2, but i has a value of 5, so none of the cases match. Therefore, the default branch of the switch statement is executed, which prints “i is not 1 or 2” to the console.
39. What is the output of the following code?
int[] numbers = { 1, 2, 3, 4, 5 };
foreach (int number in numbers)
{
Console.Write(number + ” “);
}
a. 1 2 3 4 5
b. 5 4 3 2 1
c. Compiler error
d. None of the above
Answer: a. 1 2 3 4 5
Explanation: The foreach loop iterates over the array of integers and assigns each element in turn to the variable number, which is then printed to the console. Therefore, the output is the values of the array from 1 to 5.
40. Which of the following statements is true about the using statement in C#?
a. The using statement is used to declare variables that are only used within a specific block of code.
b. The using statement is used to ensure that an object is disposed of when it is no longer needed.
c. The using statement is used to define a new class.
d. None of the above
Answer: b. The using statement is used to ensure that an object is disposed of when it is no longer needed.
Explanation: The using statement is used to ensure that an object is disposed of when it is no longer needed, and is often used with classes that implement the IDisposable interface.
We hope that these C# MCQs have helped you gain knowledge about the C# concept. If you’re interested in more technical quizzes in the software field, please follow our website, Freshersnow.