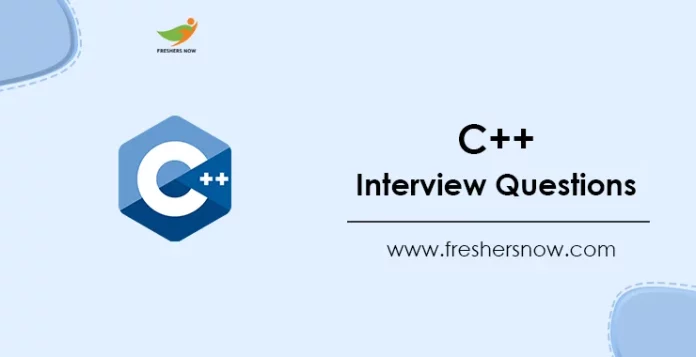
C++ Interview Questions and Answers: If you are preparing for a technical interview in the field of programming, then having a solid understanding of C++ is crucial. C++ is one of the most widely used programming languages in the world, and it is known for its high performance and flexibility. As a result, many companies require C++ skills in their technical interviews. To help you prepare for your next interview, we have compiled a comprehensive list of the top 100 C++ interview questions and answers.
★★ Latest Technical Interview Questions ★★
C++ Technical Interview Questions
This list includes the latest C++ interview questions as well as C++ technical interview questions and C++ interview questions for freshers. By going through these questions, you can improve your understanding of C++ concepts and increase your chances of acing your next interview.
Top 100 C++ Interview Questions and Answers 2023
1. What is C++?
Bjarne Stroustrup created C++, an object-oriented programming language that was released in 1985. C++ is an extension of C, with classes being the most significant addition to the C language. Stroustrup initially named the language “C with classes,” but it was later changed to C++. The name was inspired by the C increment operator ++, which represents the language’s evolution and progression.
2. What made you interested in C++ programming?
- I was interested in C++ programming because it is a powerful and versatile programming language that can be used to develop various types of applications, including system software, device drivers, and games.
- C++ also allows for efficient memory management and provides support for object-oriented programming, which makes it a popular choice for developers.
3. What is the difference between C and C++ programming?
- C++ is an extension of the C programming language and includes additional features such as classes and objects.
- C++ also supports function overloading, templates, and exception handling, which are not present in C.
- C++ also allows for object-oriented programming while C does not.
4. What is the syntax to declare a variable of type integer in C++?
To declare a variable of type integer in C++, you would use the following syntax:
int variableName; |
5. Can you explain the concept of OOP in C++?
- Object-oriented programming (OOP) is a programming paradigm that is based on the concept of objects.
- In C++, objects are created from classes, which are user-defined data types that encapsulate data and the functions that operate on that data.
- OOP allows for code reusability, encapsulation, and abstraction, which can result in more maintainable and modular code.
6. How are virtual functions different from non-virtual functions in C++?
Virtual Functions | Non-Virtual Functions |
---|---|
Virtual functions allow dynamic binding and late binding | Non-virtual functions are resolved at compile-time and static binding happens |
Virtual functions can be overridden in the derived class | Non-virtual functions cannot be overridden in the derived class |
A virtual function must have a definition in the base class | A non-virtual function can have only declaration in the base class |
A virtual function is declared with the keyword ‘virtual’ | A non-virtual function is declared without the keyword ‘virtual’ |
Virtual functions can be called through a base class pointer | Non-virtual functions cannot be called through a base class pointer |
Virtual functions can have a default implementation | Non-virtual functions must have implementation in the base class |
Virtual functions can be used to achieve polymorphism | Non-virtual functions do not support polymorphism |
7. What are the advantages of using C++ over other programming languages?
- C++ provides efficient memory management and performance due to its use of low-level features like pointers.
- C++ supports object-oriented programming, which allows for code reuse and abstraction.
- C++ has a large standard library that includes many useful functions and data structures.
- C++ is a popular language for developing system software, games, and other performance-critical applications.
8. What are the basic data types in C++?
- C++ includes several basic data types, including integers (int), floating-point numbers (float, double), characters (char), and boolean values (bool).
- There are also other data types such as unsigned integers, long integers, and short integers.
9. Can you explain the concept of pointers in C++?
- Pointers in C++ are variables that store memory addresses of other variables.
- They are used to manipulate and access data stored in memory, and allow for more efficient memory management.
- Pointers can be dereferenced to access the data at the memory address they are pointing to.
10. What is the difference between pass by reference and pass by value in C++?
- Pass by value means that a copy of the argument is passed to a function, while pass by reference means that a reference to the argument is passed.
- When passing by value, any changes made to the argument inside the function will not affect the original value.
- When passing by reference, any changes made to the argument inside the function will affect the original value.
11. How do you declare a constant in C++?
To declare a constant in C++, you would use the following syntax:
const dataType constantName = value; |
12. How is a pointer different from a reference in C++?
Pointers | References |
---|---|
A pointer is a variable that stores the memory address of another variable. | A reference is a variable that refers to another variable, and it is an alias to the original variable. |
Pointers are declared using the * symbol. | References are declared using the & symbol. |
Pointers can be reassigned to point to different variables or be set to null. | References cannot be reassigned to refer to another variable. Once a reference is initialized, it cannot be changed to refer to a different variable. |
Pointers can be assigned to point to the address of a variable or point to null. | References must be initialized when declared, and they cannot refer to null. |
Pointers can be used to perform pointer arithmetic. | References cannot be used for pointer arithmetic. |
Pointers can be used to create dynamic data structures like linked lists, trees, and graphs. | References are not suitable for creating dynamic data structures. |
Pointers have their own memory address and occupy their own memory space. | References do not have their memory address and do not occupy their memory space. |
Pointers are passed by value in function calls unless they are passed as pointers to pointers. | References are passed by reference in function calls. |
Pointers can be used to implement virtual functions and polymorphism. | References can also be used to implement virtual functions and polymorphism. |
13. What is the difference between a structure and a class in C++?
- A structure is a user-defined data type that groups related data together, while a class is a user-defined data type that encapsulates data and functions.
- Classes support object-oriented programming, while structures do not.
- By default, the members of a class are private, while the members of a structure are public.
14. What is inheritance in C++?
- Inheritance is a feature of object-oriented programming that allows a class to inherit the properties and functions of another class.
- The class that is being inherited from is called the base class or parent class, while the class that inherits from it is called the derived class or child class.
- Inheritance allows for code reuse and can result in more modular and maintainable code.
15. What is polymorphism in C++?
- Polymorphism is a feature of object-oriented programming that allows a single function to operate on different types of objects.
- This is achieved through function overloading and virtual functions.
- Polymorphism allows for more flexible and modular code, as functions can be designed to work with a variety of different objects without needing to know their specific types in advance.
16. What is the syntax for a while loop in C++?
The syntax for a while loop in C++ is as follows:
while (condition) { // code to execute } |
17. What is encapsulation in C++?
- Encapsulation is a mechanism in C++ that binds data and functions together and prevents direct access to data from outside the class.
- It is used to create a data abstraction layer to hide the implementation details of a class from its users.
- Encapsulation helps in improving the security and maintainability of code, as the internal data of a class can only be accessed through its member functions.
18. Can you explain the concept of virtual functions in C++?
- Virtual functions are functions that are declared with the keyword “virtual” in a base class and can be overridden by a derived class.
- They are used to achieve runtime polymorphism in C++, where the appropriate function is called at runtime based on the type of the object pointed to by the base class pointer.
- When a virtual function is called on a derived class object through a base class pointer or reference, the correct function is selected based on the actual object type.
19. Compare compile-time polymorphism and Runtime polymorphism
pile-Time Polymorphism | Runtime Polymorphism |
---|---|
It is also termed static binding and early binding. | It is also termed Dynamic binding and Late binding. |
It is achieved by function overloading and operator overloading. | It is achieved by virtual functions and function overriding. |
It is fast because execution is known early at compile time. | It is slow as compared to compile-time because execution is known at runtime. |
20. What is the syntax for a for loop in C++?
The syntax for a for loop in C++ is as follows:
for (initialization; condition; increment) { // code to execute } |
21. How is inheritance different from composition in C++?
Inheritance | Composition |
---|---|
Inheritance is a mechanism that allows a new class to be based on an existing class. | Composition is a mechanism that allows a class to have objects of other classes as its members. |
Inheritance creates an “is-a” relationship between the derived class and the base class. | Composition creates a “has-a” relationship between the containing class and the contained class. |
Inheritance allows the derived class to inherit the data members and member functions of the base class. | Composition allows the containing class to have objects of the contained class as its members. |
Inheritance provides the ability to override the member functions of the base class in the derived class. | Composition does not provide the ability to override the member functions of the contained class. |
Inheritance allows for polymorphism, which allows objects of the derived class to be treated as objects of the base class. | Composition does not allow for polymorphism between the containing class and the contained class. |
Inheritance can lead to tightly coupled code and may result in a complex class hierarchy. | Composition can lead to looser coupling and a more modular design. |
Inheritance can be used to implement interfaces and abstract classes. | Composition cannot be used to implement interfaces and abstract classes. |
Inheritance is used for “is-a” relationships such as a car is a vehicle. | Composition is used for “has-a” relationships such as a car has an engine. |
Inheritance can result in code duplication if multiple derived classes need to override the same member function. | Composition does not result in code duplication, but it may require more code to delegate member function calls to the contained objects. |
22. What is the difference between public, private, and protected access specifiers in C++?
- Public, private, and protected are access specifiers in C++ that are used to specify the level of access to the members of a class.
- Public members can be accessed from anywhere outside the class, private members can only be accessed from within the class, and protected members can be accessed from within the class and its derived classes.
- The access specifiers determine the visibility and accessibility of the members of a class, and help in achieving encapsulation in C++.
23. When should we use multiple inheritance?
Multiple inheritances allow a derived class to inherit two or more base or parent classes, which can be beneficial when the derived class requires a combination of various attributes or contracts and the implementation from these sources. For instance, consider the scenario of having two parents – Parent A (DAD) and Parent B (MOM), and the child C representing oneself.
24. Can you explain the concept of templates in C++?
- Templates are a feature in C++ that allow for generic programming by defining functions or classes that can work with multiple data types.
- They are used to write code that is independent of the data type, and can be reused for different data types.
- Templates are defined using the “template” keyword, and can be parameterized with one or more template parameters.
25. What is the difference between function overloading and function overriding in C++?
- Function overloading is a feature in C++ that allows defining multiple functions with the same name but different parameters.
- Function overriding is a feature in C++ that allows a derived class to provide its own implementation of a virtual function inherited from a base class.
- Function overloading is resolved at compile-time based on the number and types of arguments, while function overriding is resolved at runtime based on the type of the object pointed to by the base class pointer.
26. How do you declare a function in C++?
To declare a function in C++, you would use the following syntax:
returnType functionName(parameterType parameterName); |
27. What is a constructor in C++?
- A constructor is a special member function in C++ that gets executed automatically when an object of a class is created.
- It is used to initialize the data members of the class and allocate resources required by the object.
- A constructor has the same name as the class and no return type, not even void.
28. What is a destructor in C++?
- A destructor is also a special member function in C++ that is executed automatically when an object is destroyed.
- It is used to release the resources allocated by the object and clean up any mess created by the object.
- A destructor has the same name as the class, preceded by a tilde (~) symbol and no return type, not even void.
29. What is a copy constructor in C++?
- A copy constructor is a special constructor that is used to create a new object as a copy of an existing object.
- It takes an object of the same class as an argument and creates a new object with the same values of its data members.
- If a class doesn’t have a copy constructor, the compiler creates a default one which performs a shallow copy of the data members.
30. How is inheritance different from composition in C++?
Aspect | Inheritance | Composition |
---|---|---|
Relationship | “is-a” | “has-a” |
Syntax | Derived class inherits from base class using the : symbol |
Member object is declared within a class using the object’s type |
Code Reuse | Reuses code from the base class | Reuses code from the member object |
Access Control | Public, protected, or private inheritance determines access to base class members | Access to member object members is determined by the access control of the containing class |
Dependency | Tight coupling between base and derived class | Looser coupling between containing and contained class |
Dynamic Polymorphism | Derived class objects can be used in place of base class objects with virtual functions | Not applicable |
Object Lifetime | Derived object includes base object’s data members and can manipulate them | Contained object’s lifetime is managed by the containing object |
Object Creation | Derived object is created by calling base class constructor within derived class constructor | Contained object is created and destroyed independently of containing object |
Overhead | Can incur additional memory and performance overhead due to the inclusion of base class members in derived object | Typically has less overhead since contained object is separate from containing object |
31. What is a move constructor in C++?
- A move constructor is a special constructor that is used to create a new object by moving the resources of an existing object.
- It takes an object of the same class as an argument and creates a new object by transferring the ownership of the resources from the source object to the destination object.
- A move constructor is more efficient than a copy constructor when dealing with large objects, as it avoids the unnecessary copy of data.
32. What is overloading?
Overloading refers to the phenomenon where a single object can exhibit different behaviors. It involves providing various versions of the same function with the same name. C++ offers the ability to define multiple definitions for a function or operator in the same scope, known as function overloading and operator overloading, respectively. Overloading can be categorized into two types:
- Operator overloading: This is a type of compile-time polymorphism, where a standard operator can be overloaded to provide a user-defined definition. For instance, the ‘+’ operator can be overloaded to perform addition operations on different data types like int and float. Operator overloading can be implemented using member functions, non-member functions, and friend functions. The syntax for operator overloading is as follows:
Return_type classname :: Operator Operator_symbol(argument_list) { // body_statements; } |
- Function overloading: This is also a type of compile-time polymorphism that allows defining a family of functions with the same name. The function performs different operations based on the argument list in the function call, and the function invoked depends on the number and type of arguments in the argument list.
33. What is an operator overloading in C++?
- Operator overloading is a feature in C++ that allows operators to be used with user-defined data types.
- It allows operators such as +, -, *, /, and == to be redefined for a class, making it possible to perform operations on objects of that class.
- Operator overloading can be done using member functions or non-member functions, depending on the operator being overloaded.
34. What is the syntax for a switch statement in C++?
The syntax for a switch statement in C++ is as follows:
switch (expression) { case constantValue: // code to execute; break; default: // code to execute if none of the cases match; break; } |
35. What is a friend function in C++?
- A friend function in C++ is a non-member function that is granted access to the private and protected members of a class.
- It can be declared in the class definition with the keyword “friend”.
- It is not a member function of the class, but it can access private and protected members of the class if declared as a friend.
36. What is a friend class in C++?
- A friend class in C++ is a class that is granted access to the private and protected members of another class.
- It can be declared in the class definition with the keyword “friend”.
- All member functions and nested classes of a friend class can access the private and protected members of the class that granted friendship.
37. What is a class?
A class is a customized data type that is defined by the user using the keyword “class”. It comprises data members and member functions, whose accessibility is determined by three modifiers, namely private, public, and protected. The class specifies the type definition of a group of things and defines a data type by specifying the data structure, rather than the actual data itself.
Multiple objects can be created from a class, and there is no limit to the number of objects that can be created.
38. What is a static data member in C++?
- A static data member in C++ is a variable that is associated with a class rather than an object of the class.
- It can be accessed using the class name and the scope resolution operator.
- It is initialized only once, regardless of the number of objects created.
39. How do you declare a multidimensional array in C++?
To declare a multidimensional array in C++, you would use the following syntax:
dataType arrayName[dimension1][dimension2]; |
40. What is a virtual destructor in C++?
- A virtual destructor in C++ is a destructor that is declared as virtual in the base class.
- It ensures that the destructor of the derived class is called when an object of the derived class is deleted through a pointer to the base class.
- It is necessary when dealing with polymorphic classes to prevent memory leaks.
41. What is a pure virtual function in C++?
- A pure virtual function in C++ is a virtual function that is declared in the base class but has no implementation.
- It is declared with “= 0” at the end of the function declaration.
- It makes the base class abstract and cannot be instantiated on its own.
42. What is a pure virtual destructor in C++?
- A pure virtual destructor in C++ is a virtual destructor that is declared in the base class but has no implementation.
- It is declared with “= 0” at the end of the destructor declaration.
- It makes the base class abstract and cannot be instantiated on its own.
43. What is a reference in C++?
- A reference in C++ is an alias or nickname for an existing variable.
- It is declared with an ampersand (&) before the variable name.
- It is a type of pointer that provides a convenient way of accessing the value of a variable without actually copying it.
44. What is the difference between a pointer and a reference in C++?
- A pointer in C++ stores the memory address of a variable, while a reference is an alias or nickname for an existing variable.
- A pointer can be null, while a reference cannot.
- A pointer can be reassigned to point to a different object, while a reference cannot.
45. What is a const keyword in C++?
- The const keyword in C++ is used to specify that a variable or a function cannot be modified.
- For variables, it can be used to declare constants or to prevent modification of an object through a pointer or a reference.
- For functions, it can be used to indicate that the function does not modify the object on which it is called.
46. What is a volatile keyword in C++?
The volatile keyword is used to indicate that a variable’s value can be changed unexpectedly by external factors such as hardware or other processes, and that the compiler should not optimize the code that accesses that variable.
47. What is the difference between a constructor and a destructor in C++?
Constructor | Destructor |
---|---|
A constructor is a special member function that is called automatically when an object of a class is created. | A destructor is a special member function that is called automatically when an object of a class is destroyed. |
The purpose of a constructor is to initialize the data members of an object. | The purpose of a destructor is to release any resources held by the object. |
Constructors do not have a return type. | Destructors do not have a return type. |
Constructors have the same name as the class and are identified by the absence of a return type. | Destructors have the same name as the class, preceded by a tilde (~) symbol. |
Constructors can be overloaded, allowing multiple constructors to be defined with different sets of parameters. | Destructors cannot be overloaded. |
Constructors can be called explicitly to create an object. | Destructors cannot be called explicitly; they are called automatically when an object is destroyed. |
If a constructor is not defined for a class, the compiler will provide a default constructor. | If a destructor is not defined for a class, the compiler will provide a default destructor. |
Constructors are used to initialize the object’s state and allocate resources. | Destructors are used to deallocate resources and perform cleanup tasks. |
Constructors are called in the order in which they are declared in the class definition. | Destructors are called in the opposite order in which they are declared in the class definition. |
48. What is the syntax for a do-while loop in C++?
The syntax for a do-while loop in C++ is as follows:
do { // code to execute } while (condition); |
49. What is a static keyword in C++?
The static keyword can be used in different contexts to mean different things. When used with a global variable or a function inside a file, it limits its scope to that file only. When used with a class member variable or a function, it makes it shared among all instances of the class.
50. What is a virtual keyword in C++?
The virtual keyword is used to define a virtual function in C++. A virtual function is a function that can be overridden by a subclass. When a virtual function is called on a pointer to an object, the actual function called is determined at runtime based on the type of the object.
51. What is a template specialization in C++?
Template specialization is a technique used in C++ to provide a different implementation of a template for a specific data type or set of data types. It allows a programmer to provide a specific implementation for a certain type or set of types, instead of using the generic implementation provided by the template.
52. What is a lambda function in C++?
A lambda function, also known as an anonymous function, is a way to define a small function inline without having to give it a name. It can capture variables from its enclosing scope, and can be used as an argument to another function or stored in a variable.
53. What is an exception in C++?
An exception is an error condition that arises during program execution and interrupts the normal flow of the program. In C++, exceptions are objects that are thrown using the throw keyword and caught using the try-catch block.
54. What is the difference between try-catch and throw in C++?
The throw keyword is used to raise an exception, while the try-catch block is used to catch and handle an exception. When an exception is thrown, the program searches for the nearest catch block that can handle the exception. If no matching catch block is found, the program terminates.
55. How do you declare a structure in C++?
To declare a structure in C++, you would use the following syntax:
truct structName { dataType member1; dataType member2; // … }; |
56. What is a namespace in C++?
A namespace is a way to group related classes, functions, and variables under a single name. It helps to avoid naming conflicts between different parts of a program, especially when different libraries or frameworks are used.
57. What is a header file in C++?
A header file in C++ is a file that contains declarations of functions, variables, classes, and other objects that can be used in a program. It is typically included at the beginning of a source code file using the #include directive.
58. What is a preprocessor directive in C++?
A preprocessor directive is a command that is executed by the C++ preprocessor before the code is compiled. It can be used to include header files, define macros, and perform other tasks that are necessary before the code can be compiled. Preprocessor directives are indicated by the # symbol.
59. What is a macro in C++?
- A macro is a preprocessor directive in C++ that allows the programmer to define a symbolic name or constant value that can be used throughout the code.
- Macros are often used to define constants or to create short, reusable code snippets.
60. What is a typedef in C++?
- Typedef is a keyword in C++ that allows the programmer to create a new name for an existing data type.
- Typedef can be used to create more descriptive and meaningful names for data types, or to create aliases for complex data types.
61. What is a struct in C++?
- A struct is a user-defined data type in C++ that groups related data together.
- Structs are similar to classes, but they have all members public by default.
- Structs are often used to represent simple data structures.
62. What is a union in C++?
- A union is a user-defined data type in C++ that allows the programmer to store different data types in the same memory location.
- Unlike structs, only one member of a union can be accessed at a time.
- Unions are often used in situations where memory efficiency is important.
63. What is a bit-field in C++?
- A bit-field is a user-defined data type in C++ that allows the programmer to specify the number of bits used to represent a member of a struct or class.
- Bit-fields are often used to save memory when representing boolean values or other small data types.
64. What is the difference between struct and class?
Struct | Class | |
---|---|---|
Access Control | Members are public by default | Members are private by default |
Inheritance | Cannot be inherited | Can be inherited |
Polymorphism | Does not support virtual functions | Supports virtual functions |
Default Member Visibility | Public | Private |
Default Inheritance | Public | Private |
Constructor/Destructor | Can have constructor and destructor | Can have constructor and destructor |
Memory Allocation | Memory is allocated on stack | Memory is allocated on heap |
Usage | Suitable for small, simple data structures | Suitable for larger, more complex data structures and objects |
Default Value | Members are not initialized by default | Members are initialized by default |
65. What is a standard template library (STL) in C++?
- The standard template library (STL) is a collection of classes and functions that are part of the C++ standard library.
- The STL provides many useful data structures and algorithms, including containers, iterators, and algorithms for sorting and searching.
66. What is an iterator in C++?
- An iterator is a C++ object that allows the programmer to traverse the elements of a container (such as a vector or list) in a specific order.
- Iterators can be used to access and modify elements of the container, and they can be advanced or moved back to different positions within the container.
67. What is an algorithm in C++?
- An algorithm is a function or procedure in C++ that can be used to solve a specific problem or perform a specific task.
- The STL provides many useful algorithms, including sorting, searching, and manipulating elements of containers.
68. What is a container in C++?
- A container is a data structure in C++ that can hold a collection of other objects or data types.
- Containers can be used to store and manage large amounts of data, and they can provide useful functionality such as sorting, searching, and iteration.
69. What is a sequence container in C++?
- A sequence container is a type of container in C++ that stores elements in a specific order.
- Examples of sequence containers include arrays, vectors, lists, and deques.
- Sequence containers can be useful for representing ordered data or for performing operations on elements in a specific order.
70. What is an associative container in C++?
- An associative container in C++ is a type of container that stores elements in a way that allows direct access to them based on their values.
- It is implemented using data structures such as binary trees, hash tables, or skip lists.
- Examples of associative containers in C++ include sets, maps, and multimaps.
71. What is a stack in C++?
- A stack in C++ is a type of container that stores elements in a last-in, first-out (LIFO) order.
- It provides two main operations: push, which adds an element to the top of the stack, and pop, which removes the top element from the stack.
- It is implemented using a deque or a singly linked list.
72. What is a queue in C++?
- A queue in C++ is a type of container that stores elements in a first-in, first-out (FIFO) order.
- It provides two main operations: enqueue, which adds an element to the back of the queue, and dequeue, which removes the front element from the queue.
- It is implemented using a deque or a singly linked list.
73. What is a priority queue in C++?
- A priority queue in C++ is a type of container that stores elements in a way that allows quick access to the element with the highest priority.
- It is implemented using a heap data structure.
- It provides two main operations: push, which adds an element to the queue, and pop, which removes the element with the highest priority from the queue.
74. What is a set in C++?
- A set in C++ is an ordered collection of unique elements.
- It is implemented using a binary tree or a hash table.
- It provides operations for inserting, removing, and searching elements.
75. What is a map in C++?
- A map in C++ is an ordered collection of key-value pairs.
- It is implemented using a binary tree or a hash table.
- It provides operations for inserting, removing, and searching elements based on their keys.
76. What is a vector in C++?
- A vector in C++ is a dynamic array that stores elements in contiguous memory locations.
- It provides constant time access to elements, and linear time access to elements at the middle and end of the vector.
- It provides operations for inserting, removing, and accessing elements.
77. What is a deque in C++?
- A deque in C++ is a double-ended queue that allows efficient insertion and removal of elements at the beginning and end of the queue.
- It provides constant time access to elements at the beginning and end of the deque, and linear time access to elements in the middle.
- It is implemented using a dynamic array or a doubly linked list.
78. What is a list in C++?
- A list in C++ is a doubly linked list that allows efficient insertion and removal of elements at any position.
- It provides constant time access to the beginning and end of the list, and linear time access to elements in the middle.
- It provides operations for inserting, removing, and accessing elements.
79. What is a forward list in C++?
- A forward list in C++ is a singly linked list that allows efficient insertion and removal of elements at any position.
- It provides constant time access to the beginning of the list, and linear time access to elements in the middle.
- It provides operations for inserting, removing, and accessing elements.
80. What is a hash table in C++?
- A hash table is a data structure in C++ that stores a collection of key-value pairs. It uses a hash function to compute the index of the key-value pair in an array, allowing for fast retrieval of values based on their keys.
81. What is a multiset in C++?
- A multiset is a container in C++ that stores a collection of elements in which each element can occur multiple times. It is implemented as a balanced binary search tree, which allows for fast insertion, deletion, and retrieval of elements.
82. What is a multimap in C++?
- A multimap is a container in C++ that stores a collection of key-value pairs in which each key can be associated with multiple values. It is implemented as a balanced binary search tree, which allows for fast insertion, deletion, and retrieval of key-value pairs.
83. What is a unique pointer in C++?
- A unique pointer is a smart pointer in C++ that manages the lifetime of an object and ensures that it is deleted when it is no longer needed. It does not allow for multiple pointers to the same object, and cannot be copied or assigned.
84. What is a shared pointer in C++?
- A shared pointer is a smart pointer in C++ that manages the lifetime of an object and allows for multiple pointers to the same object. It uses a reference counting mechanism to keep track of the number of pointers that reference the object, and deletes the object when the count reaches zero.
85. What is a weak pointer in C++?
- A weak pointer is a smart pointer in C++ that provides a non-owning reference to an object that is managed by a shared pointer. It does not affect the reference count of the shared pointer, and can be used to avoid circular references between shared pointers.
86. What is the difference between unique pointer and shared pointer in C++?
- The main difference between unique pointer and shared pointer is that unique pointer does not allow for multiple pointers to the same object, while shared pointer does. Unique pointer uses move semantics to transfer ownership of an object, while shared pointer uses reference counting to manage the lifetime of an object.
87. What is RAII in C++?
- RAII stands for Resource Acquisition Is Initialization. It is a programming technique in C++ that involves acquiring resources such as memory or file handles during object construction, and releasing them during object destruction. RAII is used to ensure that resources are always properly managed and cleaned up, even in the presence of exceptions or other errors.
88. What is a smart pointer in C++?
- A smart pointer is a C++ class that manages the lifetime of an object and ensures that it is properly deleted when it is no longer needed. Smart pointers can be used to avoid memory leaks, null pointer dereferences, and other common problems associated with manual memory management.
89. What is the difference between an abstract class and an interface in C++?
- An abstract class is a class in C++ that contains at least one pure virtual function, which makes it impossible to create objects of that class. An interface is a class in C++ that contains only pure virtual functions, and serves as a contract that other classes can implement. The main difference between abstract classes and interfaces is that abstract classes can contain non-virtual functions and member variables, while interfaces cannot.
90. What is the difference between a vector and a list in C++?
- A vector is a container that stores elements in a contiguous memory location, whereas a list is a container that stores elements in a non-contiguous memory location.
- Vectors are more efficient for random access and can be resized, while lists are more efficient for insertion and deletion of elements at any position.
- Vectors provide better cache locality, whereas lists provide better memory utilization.
91. What is the difference between an array and a pointer in C++?
- An array is a collection of elements of the same data type that are stored in a contiguous memory location, while a pointer is a variable that stores the memory address of another variable.
- The size of an array is fixed and determined at compile time, while a pointer can be reassigned to point to a different memory location at runtime.
- An array name can decay into a pointer to its first element, which can be used to access the elements of the array.
92. What is the difference between const and constexpr in C++?
- Const is a keyword in C++ that is used to indicate that a variable cannot be modified, while constexpr is a keyword that is used to indicate that a variable or function can be evaluated at compile time.
- Const variables can be initialized at runtime, while constexpr variables must be initialized with a constant expression.
- Const functions can only read data members and cannot modify them, while constexpr functions must be pure functions and their arguments and return values must be of literal types.
93. What is the difference between a shallow copy and a deep copy in C++?
- Shallow copy is a type of copy that copies the memory address of an object’s data members to a new object, while deep copy is a type of copy that creates a new object and copies the data from the source object to the new object.
- In shallow copy, if the source object is modified, the copied object will also be modified, while in deep copy, the source and copied objects are independent of each other.
- Shallow copy is performed by default by the copy constructor and the assignment operator, while deep copy must be explicitly implemented.
94. What is the difference between new and malloc in C++?
- New is a keyword in C++ that is used to dynamically allocate memory for an object and initialize it, while malloc is a function in C that is used to dynamically allocate memory without initializing it.
- New is an operator and automatically calls the constructor, while malloc returns a void pointer and requires a cast to use with non-void pointer types.
- New throws an exception if it fails to allocate memory, while malloc returns a null pointer.
95. What is the difference between an iterator and a pointer in C++?
- An iterator is an object that is used to traverse the elements of a container, while a pointer is a variable that stores the memory address of another variable.
- Iterators can be used with a range of containers, while pointers can only be used with arrays or pointers to objects.
- Iterators can be overloaded with operators such as ++ and –, while pointers can be used with these operators by default.
96. What is a const_iterator in C++?
- A const_iterator is a type of iterator that is used to traverse the elements of a container without modifying them.
- It is used to enforce the const correctness of a container and can be used with const containers and non-const containers.
- A const_iterator cannot be used to modify the elements of a container.
97. What is the difference between a const_iterator and an iterator in C++?
- An iterator is used to traverse the elements of a container and modify them, while a const_iterator is used to traverse the elements of a container without modifying them.
- An iterator can be used to modify the elements of a container, while a const_iterator cannot modify the elements.
- A const_iterator can be used with const containers and non-const containers, while an iterator can only be used with non-const containers.
98. What is a reverse_iterator in C++?
- A reverse_iterator is a type of iterator that is used to traverse the elements of a container in reverse order.
- It is used to iterate over the elements of a container from the end to the beginning.
- It is used with the rbegin and rend functions of a container to iterate over the elements in reverse order.
99. What is the difference between a reverse_iterator and an iterator in C++?
- An iterator is used to traverse the elements of a container in forward order, while a reverse_iterator is used to traverse the elements of a container in reverse order.
- An iterator is used with the begin and end functions of a container to iterate over the elements in forward order, while a reverse_iterator is used with the rbegin and rend functions of a container to iterate over the elements in reverse order.
- An iterator can be used to modify the elements of a container, while a reverse_iterator can also be used to modify the elements in reverse order.
100. What is a static member function in C++?
- A static member function in C++ is a function that is associated with a class rather than an object of the class.
- It can be called using the class name and the scope resolution operator.
- It can only access static data members and other static member functions of the class.
The Top 100 C++ Interview Questions and Answers provide a comprehensive resource to help job seekers prepare for technical interviews in C++ programming, covering the latest questions and concepts. To access further knowledge, please follow us at freshersnow.com, where we offer a variety of resources to help you expand your understanding.