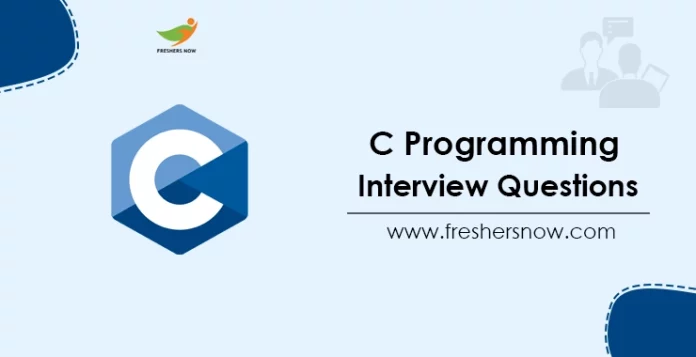
C Programming Interview Questions and Answers: C Programming is a widely used language in the world of software development. Whether you are a seasoned professional or a fresher, preparing for an interview can be a daunting task. To help you prepare, we have compiled a list of the top 100 C Programming interview questions and answers that are frequently asked in technical interviews. Our collection includes the latest C Programming interview questions, ranging from basic to advanced topics, that will help you test your knowledge and prepare yourself for technical interviews.
★★ Latest Technical Interview Questions ★★
C Programming Technical Interview Questions
Our C Programming Interview Questions for Freshers cover a wide range of topics and are suitable for both experienced professionals and freshers who are looking to start their career in software development.
Top 100 C Programming Interview Questions and Answers 2023
1. What is C programming and why is it important?
- C programming is a high-level programming language that was developed in the 1970s.
- It is important because it is widely used in developing operating systems, system software, embedded systems, and other applications.
- It is a powerful language that allows for efficient memory usage and low-level control over the hardware.
2. What is a variable in C programming?
- A variable is a named memory location in C programming.
- It is used to store values that can be used and modified throughout the program.
- Variables must be declared with a specific data type before they can be used.
3. What is the syntax for declaring a variable in C programming?
The syntax for declaring a variable in C programming is:
data_type variable_name; |
4. What is the difference between local and global variables in C programming?
- Local variables are declared inside a function and can only be accessed within that function.
- Global variables are declared outside of any function and can be accessed throughout the entire program.
- Local variables have a limited scope, while global variables have a wider scope.
5. What is a pointer in C programming?
- A pointer is a variable that stores the memory address of another variable.
- Pointers are used to pass values by reference, dynamically allocate memory, and access data structures.
6. What is the difference between pointer and array in C programming?
- An array is a collection of elements of the same data type, while a pointer is a variable that stores the memory address of another variable.
- An array can be accessed using an index, while a pointer must be dereferenced to access the value it points to.
- An array has a fixed size, while a pointer can be dynamically allocated and resized.
7. What is the use of malloc() function in C programming?
- The malloc() function is used to dynamically allocate memory in C programming.
- It returns a pointer to a block of memory of a specified size.
- The allocated memory must be explicitly freed using the free() function when it is no longer needed.
8. What is the difference between pass by value and pass by reference in C programming?
Feature | Pass by Value | Pass by Reference |
---|---|---|
Parameter Declaration | Formal parameters are declared as variables, e.g. void func(int x) |
Formal parameters are declared as pointers, e.g. void func(int *x) |
Parameter Passing | Copies the value of the actual parameter into the formal parameter | Passes a reference to the memory location of the actual parameter |
Modification of the actual parameter | Does not modify the actual parameter | Modifies the actual parameter |
Accessing the actual parameter | Accesses a copy of the actual parameter | Accesses the original actual parameter |
Memory | Uses less memory since copies are made | Uses more memory since pointers are used |
Examples | void swap(int a, int b) or int add(int x, int y) |
void swap(int *a, int *b) or void increment(int *x) |
9. What is the use of calloc() function in C programming?
- The calloc() function is used to dynamically allocate and initialize memory in C programming.
- It returns a pointer to a block of memory of a specified size, with all bytes initialized to 0.
- The allocated memory must be explicitly freed using the free() function when it is no longer needed.
10. What is the use of realloc() function in C programming?
- The realloc() function is used to dynamically reallocate memory in C programming.
- It is used to change the size of a previously allocated block of memory.
- The allocated memory must be explicitly freed using the free() function when it is no longer needed.
11. What is the difference between malloc() and calloc() in C programming?
- The malloc() function is used to dynamically allocate memory, while the calloc() function is used to dynamically allocate and initialize memory.
- The malloc() function does not initialize the allocated memory, while the calloc() function initializes all bytes to 0.
- The syntax for the two functions is slightly different, with the calloc() function taking two arguments instead of one.
12. What is a structure in C programming?
- A structure is a collection of related data items of different data types in C programming.
- It allows for the creation of complex data structures that can be easily manipulated as a single entity.
- Structures can be used to represent objects, records, and other complex data types.
13. What is the difference between structure and union in C programming?
- Structures allow for storage of different data types, while union stores only one value at a time.
- Structures allocate memory for each of its members, while union allocates memory for its largest member.
- Structure members can be accessed individually, while union members share the same memory location.
- Structures are used for storing multiple values, while union is used for optimization in memory usage.
14. What is the syntax for defining a function in C programming?
The syntax for defining a function in C programming is:
return_type function_name(parameter_list) { function_body } |
15. What is a token?
Programs are made up of individual elements called tokens in C programming. There are 6 types of tokens in C programming:
- Identifiers
- Keywords
- Constants
- Operators
- Special Characters
- Strings
16. What is the difference between fgets() and gets() functions in C programming?
- fgets() function reads a line from a file and stores it in a string, while gets() function reads a line from the input stream and stores it in a string.
- fgets() function takes two arguments- the buffer to store the string and the maximum number of characters to read, while gets() function takes only one argument, the buffer to store the string.
- fgets() function reads the newline character as part of the input, while gets() function does not.
17. What is the difference between a function declaration and a function definition in C programming?
Parameter | Function Declaration | Function Definition |
---|---|---|
Syntax | return_type function_name(argument_list); | return_type function_name(argument_list) { body_of_the_function } |
Meaning | Tells the compiler about the function’s name, return type, and parameter list. | Provides the actual implementation of the function, including its name, return type, and parameter list, as well as the code that will be executed when the function is called. |
Usage | Used when you want to define the function later in the program, or when you want to use a function that is defined in another file. | Used when you want to create a new function or define an existing one. |
Example | int add(int a, int b); | int add(int a, int b) { return a + b; } |
18. What is a Preprocessor?
The preprocessor is a software tool that processes the source file before it is compiled. It allows for various actions such as inclusion of header files, macro expansions, conditional compilation, and line control to be performed on the source file.
19. What is the use of fclose() function in C programming?
- fclose() function is used to close a file in C programming.
- It frees the memory that was allocated for the file pointer.
- It is good practice to close files after use to avoid data corruption or loss.
20. What are the features of the C language?
- C language follows a structured approach, making it a simple language to work with
- Programs written in C are highly portable, requiring little or no modifications to run on any machine
- C is a mid-level programming language, combining features of both low-level and high-level languages
- C is a structured language, where programs are divided into smaller parts
- The use of powerful data types and operators makes C a very fast language
- C provides inbuilt memory functions that improve program efficiency
- C is an extensible language, with the ability to adopt new features in the future
21. What is the syntax for accessing a member of a structure in C programming?
The syntax for accessing a member of a structure in C programming is:
structure_variable.member_name; |
22. What is the use of fprintf() function in C programming?
- fprintf() function is used to write formatted data to a file.
- It writes data to a file based on the format specifier provided.
- It can be used to write different data types to a file.
23. What is a typedef in C programming?
- typedef is a keyword used to create aliases for existing data types in C programming.
- It allows for the creation of custom data types with more meaningful names.
- typedef is used to make code more readable and easier to maintain.
24. How to check whether a linked list is circular?
Single Linked List
Circular Linked List: A circular linked list is a variant of a linked list in which the information part of the last node points to the information part of the first node, resulting in the last node not pointing to null.
25. What is the difference between a variable and a constant in C programming?
Feature | Variable | Constant |
---|---|---|
Declaration | Declared using a data type and an identifier, e.g. int x; |
Declared using the const keyword and an identifier, e.g. const int y; |
Value | Can be changed at runtime | Cannot be changed at runtime |
Storage | Stored in memory and may have an initial value | Stored in memory and must have an initial value |
Usage | Used to store data that may change during program execution | Used to store data that is known at compile time and does not change during program execution |
Scope | May have local or global scope | May have local or global scope |
Examples | int count = 0; or float pi = 3.14159; |
const int MAX_VALUE = 100; or const float GRAVITY = 9.81; |
26. What is the syntax for allocating memory dynamically using malloc() in C programming?
The syntax for allocating memory dynamically using malloc() in C programming is:
pointer_variable = (data_type*) malloc(number_of_bytes); |
27. What is the difference between const and #define in C programming?
- const is used to define variables that cannot be changed during the execution of a program, while #define is used to define constant values that can be used throughout the program.
- const variables have a data type and can be checked by the compiler for type errors, while #define does not have a data type and can lead to errors.
- const variables are stored in memory, while #define values are replaced by their values during preprocessing.
28. What is a function in C programming?
- A function is a block of code that performs a specific task.
- It can take input, perform some operations, and return output.
- Functions allow code reuse and make programs easier to understand and maintain.
29. What is the difference between call by value and call by reference in C programming?
- Call by value is a method of passing arguments to a function where the value of the variable is passed to the function.
- Call by reference is a method of passing arguments to a function where the address of the variable is passed to the function.
- In call by value, any changes made to the parameter inside the function do not affect the value of the original variable.
- In call by reference, changes made to the parameter inside the function affect the value of the original variable.
30. What is the syntax for using the conditional operator in C programming?
The syntax for using the conditional operator in C programming is:
condition ? expression1 : expression2; |
31. What is recursion in C programming?
- Recursion is a technique in which a function calls itself.
- It can be used to solve problems that can be broken down into smaller, simpler problems.
- Recursive functions have a base case that terminates the recursion and one or more recursive cases that call the function again.
32. What is the difference between recursive and iterative functions in C programming?
- Recursive functions call themselves to solve a problem, while iterative functions use loops.
- Recursive functions may require more memory and time than iterative functions, but they can sometimes be easier to understand and implement.
- Iterative functions can often be more efficient than recursive functions for large problems.
33. What are header files?
C header files are required to have a .h extension and contain function definitions, data type definitions, macros, and more. To import these definitions to the source code using the #include directive, headers are used. For instance, to take input from the user, perform some manipulation, and print the output on the terminal, the stdio.h file should be included as #include <stdio.h>. This allows for input to be taken using scanf(), manipulation to be performed, and printing to be done using printf().
34. What is the use of a header file in C programming?
- A header file contains function prototypes, constants, and variable declarations that can be used in multiple source files.
- It allows for code reuse and simplifies the process of adding new functionality to a program.
- Common header files in C include stdio.h, string.h, and math.h.
35. What is the difference between header file and source file in C programming?
- A header file contains function prototypes and declarations, while a source file contains the implementation of the functions.
- Header files are typically included at the top of a source file using the #include directive.
- Source files are compiled into object files and linked together to create an executable program.
36. What is a preprocessor directive in C programming?
- A preprocessor directive is a statement that begins with the # symbol and is processed by the preprocessor before compilation.
- Common preprocessor directives in C include #include, #define, and #ifdef.
- They are used to define constants, include header files, and conditionally compile code.
37. What is the difference between #include and #define in C programming?
- The #include directive is used to include header files in a program.
- The #define directive is used to define constants or macros.
- #include adds the contents of the specified header file to the program, while #define creates a new identifier with a specified value.
38. What is a macro in C programming?
- A macro is a preprocessor directive that defines a shorthand notation for a code sequence.
- Macros can be used to simplify code, improve readability, and reduce the chance of errors.
- Common examples of macros include #define PI 3.14159 and #define MAX(x,y) ((x)>(y)?(x):(y)).
39. What is the syntax for using the while loop in C programming?
The syntax for using the while loop in C programming is:
while(condition) { loop_body } |
40. What is the difference between a macro and a function in C programming?
- A macro is a preprocessor directive that is expanded by the preprocessor before compilation.
- A function is a block of code that is executed at runtime.
- Macros are generally faster than functions because they avoid the overhead of a function call, but they can be more error-prone and harder to debug.
- Functions can accept arguments and return values, while macros cannot.
41. What is the syntax for using the for loop in C programming?
The syntax for using the for loop in C programming is:
for(initialization; condition; increment/decrement) { loop_body } |
42. What is the use of the bitwise operator << in C programming?
- The bitwise operator << in C programming is used to shift the bits of a binary number to the left by a specified number of positions.
- The left operand specifies the number to be shifted, and the right operand specifies the number of positions to shift.
- This operator is often used in conjunction with the bitwise OR operator to set specific bits in a binary number.
43. What is the syntax for using the do-while loop in C programming?
The syntax for using the do-while loop in C programming is:
do { loop_body } while(condition); |
44. What is the use of the bitwise operator >> in C programming?
- The bitwise operator >> in C programming is used to shift the bits of a binary number to the right by a specified number of positions.
- The left operand specifies the number to be shifted, and the right operand specifies the number of positions to shift.
- This operator is often used in conjunction with the bitwise AND operator to clear specific bits in a binary number.
45. What is the syntax for using the switch statement in C programming?
The syntax for using the switch statement in C programming is:
switch(expression) { case constant1: statement1; break; case constant2: statement2; break; default: statementN; } |
46. What is a conditional operator in C programming?
- A conditional operator in C programming is also known as the ternary operator.
- It takes three operands, and the operator returns a value based on a condition.
- The syntax of the conditional operator is: condition ? value_if_true : value_if_false.
47. What is the use of the conditional operator ? : in C programming?
- The conditional operator ? : in C programming is used to make decisions based on a condition.
- It evaluates a condition and returns one of two values based on whether the condition is true or false.
- This operator is often used as a shorthand for if-else statements.
48. What is the difference between a while loop and a do-while loop in C programming?
Parameter | while loop | do-while loop |
---|---|---|
Syntax | while (condition) { body_of_the_loop } | do { body_of_the_loop } while (condition); |
Execution | First checks the condition, and if it’s true, executes the body of the loop. | Executes the body of the loop at least once, and then checks the condition. If the condition is true, the loop repeats; otherwise, the loop ends. |
Usage | Used when you want to execute the body of the loop only if the condition is true. | Used when you want to execute the body of the loop at least once, regardless of whether the condition is true or false. |
Example | int i = 0;<br>while (i < 5) { printf(“%d\n”, i); i++; } | int i = 0;<br>do { printf(“%d\n”, i); i++; } while (i < 5); |
49. What is a switch statement in C programming?
- A switch statement in C programming is a control statement that allows a program to compare a variable or expression with a list of possible values.
- It executes a corresponding block of code based on the matched value.
- The switch statement is often used as a replacement for long if-else chains.
50. What is the use of the break statement in C programming?
- The break statement in C programming is used to terminate the current loop or switch statement and transfer control to the statement following the loop or switch.
- It is often used in conjunction with a condition to exit a loop or switch statement early.
51. What is the use of the continue statement in C programming?
- The continue statement in C programming is used to skip the current iteration of a loop and continue with the next iteration.
- It is often used in conjunction with a condition to skip over certain iterations of a loop that meet a specific condition.
52. What is the use of the goto statement in C programming?
- The goto statement in C programming is used to transfer control to a labeled statement within the same function.
- It is often used for error handling or to jump to a specific section of code.
53. What is the syntax for using the pointer arithmetic in C programming?
The syntax for using the pointer arithmetic in C programming is:
pointer_variable + or – integer_value. |
54. What is a loop in C programming?
- A loop in C programming is a control statement that allows a program to execute a block of code repeatedly while a certain condition is true.
- There are three types of loops in C programming: the for loop, the while loop, and the do-while loop.
- Loops are often used for iterating over arrays, processing user input, and performing calculations.
55. What is the difference between a break statement and a continue statement in C programming?
Break Statement | Continue Statement |
---|---|
Terminates the execution of the innermost loop or switch statement | Skips the current iteration of the innermost loop |
Causes the program to exit the loop immediately and execute the statements following the loop | Causes the program to skip the remaining statements in the current iteration and move to the next iteration |
Can be used with any loop (for, while, do-while) or switch statement | Can only be used with loops (for, while, do-while) |
Does not affect the outer loops or switch statements | Does not affect the outer loops or switch statements |
Used when a certain condition is met and the loop needs to be terminated immediately | Used when a certain condition is met and the current iteration needs to be skipped, but the loop should continue executing |
Examples: <br> for(int i = 0; i < 10; i++) { if(i == 5) { break; } printf("%d", i); } <br> switch(x) { case 1: printf("Case 1"); break; case 2: printf("Case 2"); break; } |
Examples: <br> for(int i = 0; i < 10; i++) { if(i == 5) { continue; } printf("%d", i); } <br> while(x < 10) { if(x % 2 == 0) { continue; } printf("%d", x); x++; } |
56. What is the difference between a for loop and a while loop in C programming?
- The for loop is used when the number of iterations is known beforehand, while the while loop is used when the number of iterations is unknown.
- In the for loop, the initialization, condition check, and increment/decrement of the loop variable are done in a single line, while in the while loop, they are done in separate lines.
- The for loop is commonly used for iterating through arrays or lists, while the while loop is used for executing a block of code until a condition is met.
57. What is the use of the do-while loop in C programming?
- The do-while loop is used to execute a block of code at least once, regardless of whether the condition is true or false.
- The condition is checked at the end of each iteration, so the block of code is executed at least once before the condition is checked.
- The do-while loop is useful for situations where you need to execute a block of code at least once, such as reading input from the user.
58. What is the use of the continue statement in a loop in C programming?
- The continue statement is used to skip the current iteration of a loop and move on to the next iteration.
- It is useful for skipping certain iterations of a loop based on a condition, such as skipping iterations where a certain value is encountered.
- The continue statement is commonly used in loops that iterate through arrays or lists.
59. What is the use of the break statement in a loop in C programming?
- The break statement is used to exit a loop prematurely.
- It is useful for stopping the execution of a loop when a certain condition is met.
- The break statement is commonly used in loops that iterate through arrays or lists.
60. What is a multidimensional array in C programming?
- A multidimensional array is an array that contains multiple arrays.
- It is useful for storing data in a grid or table format, where each element has both a row and a column index.
- Multidimensional arrays can have any number of dimensions, such as 2D arrays or 3D arrays.
61. What is the difference between a two-dimensional array and a three-dimensional array in C programming?
- A two-dimensional array has two indices, representing rows and columns, while a three-dimensional array has three indices, representing rows, columns, and depth.
- A two-dimensional array can be thought of as a table, while a three-dimensional array can be thought of as a cube.
- Two-dimensional arrays are commonly used for storing data in a grid format, while three-dimensional arrays are used for storing data in a 3D space.
62. What is the use of the sizeof operator in C programming?
- The sizeof operator is used to determine the size, in bytes, of a variable or data type.
- It is useful for allocating memory or determining the amount of memory required to store a certain data type or variable.
- The sizeof operator is commonly used in dynamic memory allocation.
63. What is the difference between a float and a double in C programming?
- A float is a single-precision floating-point number, while a double is a double-precision floating-point number.
- A float occupies 4 bytes of memory, while a double occupies 8 bytes of memory.
- Doubles are more precise and can represent larger numbers than floats, but they also require more memory.
64. What is the difference between a character and a string in C programming?
- A character is a single letter or symbol, represented by a single character in C programming.
- A string is a sequence of characters, represented by an array of characters in C programming.
- Characters are used for single-letter variables or constants, while strings are used for storing text or sequences of characters.
65. What is the difference between a NULL pointer and a void pointer in C programming?
- A NULL pointer is a pointer that points to nothing, meaning it does not point to any valid memory location.
- A void pointer is a pointer that can point to any data type, but it does not have a specific data type of its own.
- NULL pointers are used to indicate that a pointer does not point to a valid memory location, while void pointers are used for generic pointers that can be cast to any data type.
66. What is the use of the const keyword with pointers in C programming?
- The const keyword is used to declare a variable as read-only.
- When used with pointers, it indicates that the memory location pointed to by the pointer cannot be modified.
- This can help prevent unintended changes to data and improve program stability.
67. What is a function pointer in C programming?
- A function pointer is a variable that stores the memory address of a function.
- It can be used to call a function indirectly or to pass a function as an argument to another function.
- Function pointers are often used in advanced programming techniques, such as callbacks and event-driven programming.
68. What is a callback function in C programming?
- A callback function is a function that is passed as an argument to another function and called by that function at a later time.
- Callback functions can be used to implement event-driven programming or to provide generic functionality that can be customized by the caller.
- Examples of callback functions in C include qsort() and signal().
69. What is the difference between a library function and a user-defined function in C programming?
- A library function is a pre-defined function that is included in a standard library or a third-party library.
- A user-defined function is a function that is written by the programmer.
- Library functions are typically optimized for performance and may be more efficient than user-defined functions, but user-defined functions can be customized to meet specific requirements.
70. What is the difference between a library function and a user-defined function in C programming?
- A library function is a pre-defined function that is included in a standard library or a third-party library.
- A user-defined function is a function that is written by the programmer.
- Library functions are typically optimized for performance and may be more efficient than user-defined functions, but user-defined functions can be customized to meet specific requirements.
71. What is a binary search in C programming?
- A binary search is an algorithm for finding the position of a value in a sorted array or list.
- It works by repeatedly dividing the search interval in half until the value is found or the interval is empty.
- Binary search has a time complexity of O(log n), which makes it efficient for large datasets.
72. What is the use of the qsort() function in C programming?
- The qsort() function is used to sort an array or list in ascending order.
- It takes as arguments the array, the number of elements in the array, the size of each element, and a comparison function.
- The comparison function is used to compare two elements and determine their order.
73. What is the use of the srand() and rand() functions in C programming?
- The srand() function is used to seed the random number generator in C.
- The rand() function is used to generate a pseudo-random number between 0 and RAND_MAX.
- By using srand() to set the seed value, the sequence of numbers generated by rand() can be made to appear random.
74. What is a linked list in C programming?
- A linked list is a data structure that consists of a sequence of nodes, where each node contains data and a pointer to the next node in the list.
- Linked lists can be used to implement dynamic data structures and can be modified efficiently at runtime.
- However, linked lists may have slower access times than arrays for random access of elements.
75. What is the difference between a singly linked list and a doubly linked list in C programming?
- A singly linked list has nodes that contain data and a pointer to the next node in the list, but not a pointer to the previous node.
- A doubly linked list has nodes that contain data and pointers to both the next and previous nodes in the list.
- Doubly linked lists allow for efficient traversal of the list in both directions, but require more memory to store the extra pointer. Singly linked lists are simpler and require less memory but may have slower access times for certain operations, such as reversing the list or deleting a specific node.
76. What is a stack in C programming?
- A stack is a linear data structure in C programming.
- It follows the Last-In-First-Out (LIFO) principle.
- The elements can be inserted and deleted only from one end of the stack, called the top.
- Stack operations include push (insertion) and pop (deletion) of elements.
77. What is a queue in C programming?
- A queue is a linear data structure in C programming.
- It follows the First-In-First-Out (FIFO) principle.
- Elements can be inserted at the rear end of the queue and deleted from the front end of the queue.
- Queue operations include enqueue (insertion) and dequeue (deletion) of elements.
78. What is the difference between a stack and a queue in C programming?
- In a stack, elements are inserted and deleted from the same end, called the top. In contrast, in a queue, elements are inserted at one end and deleted from the other end.
- Stack follows the LIFO principle while Queue follows the FIFO principle.
- Stack operations include push and pop while queue operations include enqueue and dequeue.
79. What is a tree in C programming?
- A tree is a non-linear data structure in C programming.
- It consists of nodes connected by edges.
- The topmost node is called the root node, and nodes with no children are called leaf nodes.
- Trees are used to represent hierarchical structures like file systems and organization charts.
80. What is the difference between a binary tree and a binary search tree in C programming?
- A binary tree is a tree where each node has at most two children.
- A binary search tree is a binary tree where the left child node has a smaller value than the parent node, and the right child node has a greater value than the parent node.
- Binary search trees are used to perform efficient search, insertion, and deletion operations.
81. What is a heap in C programming?
- A heap is a binary tree-based data structure in C programming.
- It is a complete binary tree, meaning all levels except possibly the last are filled.
- In a heap, the value of a parent node is either greater than or equal to (max heap) or less than or equal to (min heap) the values of its children nodes.
- Heaps are used to implement priority queues and sorting algorithms like heapsort.
82. What is the difference between a min heap and a max heap in C programming?
- In a min heap, the value of a parent node is less than or equal to the values of its children nodes.
- In a max heap, the value of a parent node is greater than or equal to the values of its children nodes.
- Min heaps are used to implement min-priority queues, while max heaps are used to implement max-priority queues.
83. What is a hash table in C programming?
- A hash table is a data structure in C programming that stores key-value pairs.
- It uses a hash function to compute an index into an array of buckets or slots.
- The value corresponding to the key is stored in the bucket at the computed index.
- Hash tables are used for efficient searching, insertion, and deletion operations.
84. What is the use of the hash function in a hash table in C programming?
- A hash function is used to compute an index into an array of buckets or slots in a hash table.
- The hash function takes a key as input and returns an integer that represents the index.
- A good hash function distributes the keys uniformly across the buckets, reducing collisions and improving performance.
85. What is a bubble sort in C programming?
- Bubble sort is a simple sorting algorithm in C programming.
- It works by repeatedly swapping adjacent
86. What is a selection sort in C programming?
Selection sort is a simple sorting algorithm that sorts an array by repeatedly finding the minimum element from the unsorted part and putting it at the beginning.
87. What is an insertion sort in C programming?
Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands.
88. What is a merge sort in C programming?
Merge sort is a divide and conquer algorithm that divides the input array into two halves, sorts each half, and then merges the sorted halves.
89. What is a quick sort in C programming?
Quick sort is a divide and conquer algorithm that selects a pivot element and partitions the array around the pivot, such that elements smaller than the pivot are placed before it and elements greater than the pivot are placed after it. The pivot is then placed in its final position.
90. What is the time complexity of each sorting algorithm in C programming?
- Bubble sort: O(n^2)
- Selection sort: O(n^2)
- Insertion sort: O(n^2)
- Merge sort: O(nlogn)
- Quick sort: O(nlogn) average case, O(n^2) worst case.
91. What is the difference between linear search and binary search in C programming?
Linear search checks each element of an array in sequence until the desired element is found or the end of the array is reached, while binary search is a more efficient search algorithm that works by dividing the sorted array in half repeatedly until the desired element is found.
92. What is a file in C programming?
A file is a collection of data or information that is stored in a storage device or on a secondary storage device like a hard disk.
93. What is the difference between a text file and a binary file in C programming?
A text file contains data in the form of ASCII or Unicode characters, while a binary file contains data in a binary format that can only be interpreted by a computer.
94. What is a command-line argument in C programming?
A command-line argument is an argument passed to a program when it is executed on the command line, usually used to provide input data or options to the program.
95. What is the difference between argc and argv in C programming?
argc is an integer that represents the number of arguments passed to the program, while argv is an array of strings that contains the actual arguments passed to the program.
96. What is a file pointer in C programming?
- A file pointer is a variable that is used to keep track of the current position within a file.
- It stores the address of the next character to be read or written from a file.
- File pointers are used to read, write or manipulate files in C programming.
97. What is the use of fopen() function in C programming?
- fopen() function is used to open a file in C programming.
- It returns a file pointer to the file that has been opened.
- fopen() function can be used to open files in different modes- read, write or append.
98. What is a constant in C programming?
- A constant is a value that cannot be changed during the execution of a program.
- In C programming, constants can be declared using the const keyword.
- Constants are used to define values that are fixed and do not change during the execution of a program.
99. What is a bitwise operator in C programming?
- A bitwise operator is an operator that operates on the individual bits of binary numbers in C programming.
- It is used to manipulate binary values, such as changing, checking, or shifting individual bits.
- Some examples of bitwise operators in C programming are AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
100. What is the use of fscanf() function in C programming?
- fscanf() function is used to read formatted data from a file.
- It reads data from a file based on the format specifier provided.
- It can be used to read different data types from a file.
These top 100 C programming interview questions and answers cover a wide range of topics, from basic syntax to advanced concepts, providing a comprehensive resource for anyone preparing for a C programming job interview. Follow freshersnow.com to expand your knowledge.