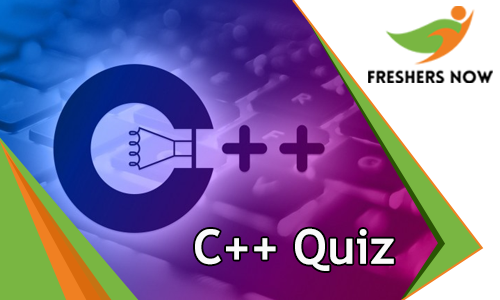
Aspirants come and face the challenging questions relevant to C++ Quiz. To enter into a professional field candidate has to attend such tricky questions on the C++ topic. Contenders, who want to take C++ Quiz can stick to this article. We are providing you with the complete info regarding the test and instructions to follow. So, candidates can check the details like C++ MCQ, C++ Online Test, C++ Multiple Choice Questions, Benefits of C++ Quiz, etc. To crack the C++ online test, candidates have to learn and practice as much as you can.
Details About C++ Quiz
Quiz Name | C++ |
Category | Technical Quiz |
Number of Questions | 25 |
Time | No Time Limit |
Exam Type | MCQ (Multiple Choice Questions) |
First, of attempting the C++ Quiz, contenders should practice the test to focus on the interview test. So, in this online test, we provide you the leading interview questions and the quiz that attain the knowledge at the moment. Every single unit of time is precious. So, better know the below necessary information and then make forward of your step.
Instructions Of C++ MCQ Quiz
- In this C++ MCQ, there are 25 Questions
- Programmers should note that each question carries one mark.
- After the successful attempt, hit on submit button.
- Now, do not refresh the Page and wait for the result.
C++ Online Test
Which of the following is not a valid C++ data type?
a) float
b) long long double
c) boolean
d) char
Answer: b) long long double
Explanation: In C++, there is no data type called “long long double”. The correct data types are “float”, “double”, “bool”, and “char”.
What is the output of the following C++ code?
#include
int main() {
int a = 5;
int b = 10;
int c = ++a * b–;
std::cout << c << std::endl;
return 0;
}
a) 45
b) 50
c) 55
d) 60
Answer: a) 45
Explanation: The expression ++a * b– is evaluated as (++a) * (b–). The value of a is incremented by 1 before multiplication, so a becomes 6. The value of b is used for multiplication before being decremented by 1, so b becomes 9. The result of the multiplication is 6 * 10, which is 60, but since b was decremented after the multiplication, the final value of b is 9. Therefore, the output of the code is 45.
Which of the following is an example of a unary operator in C++?
a) +
b) *
c) /
d) %
Answer: a) +
Explanation: In C++, unary operators operate on a single operand. The unary plus operator (+) is an example of a unary operator that simply returns the value of its operand without any change.
Which of the following is used to define a constant in C++?
a) const
b) static
c) volatile
d) mutable
Answer: a) const
Explanation: The const keyword is used to define a constant in C++ which cannot be modified once it is assigned a value. Constants are used to represent values that should not be changed during the execution of a program.
What is the output of the following C++ code?
#include
int main() {
int arr[3] = {1, 2, 3};
int* p = arr;
std::cout << *p++ << std::endl;
return 0;
}
a) 1
b) 2
c) 3
d) 4
Answer: a) 1
Explanation: The expression *p++ is equivalent to *(p++), which increments the value of p after it is used in the expression. So, *p++ dereferences the value of p and returns 1, but increments the value of p to point to the next element in the array arr. Therefore, the output of the code is 1.
Which of the following is not a valid way to initialize a string in C++?
a) char str[] = “Hello”;
b) std::string str = “Hello”;
c) char* str = “Hello”;
d) const char* str = “Hello”;
Answer: c) char* str = “Hello”
Explanation: Option c is not a valid way to initialize a string in C++ because it declares a pointer to a character (char*) and tries to initialize it with a string literal. In C++, string literals are read-only, and it is not allowed to modify them. Therefore, using char* to initialize a string literal can result in undefined behavior.
What is the output of the following C++ code?
#include
void swap(int a, int b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 5;
int y = 10;
swap(x, y);
std::cout << x << “, ” << y << std::endl;
return 0;
}
a) 5, 10
b) 10, 5
c) 0, 0
d) Compiler error
Answer: a) 5, 10
Explanation: In the code, a function named swap is defined to swap the values of two integers. However, the function takes the arguments by value, which means that it works with local copies of the arguments. Any changes made to these local copies do not affect the original variables in the main function. Therefore, after the function call swap(x, y), the values of x and y remain unchanged, and the output is 5, 10.
What is the correct way to dynamically allocate memory for an integer in C++?
a) int* p = (int*) new;
b) int* p = new int();
c) int* p = new int;
d) int* p = new int[1];
Answer: c) int* p = new int;
Explanation: Option c is the correct way to dynamically allocate memory for a single integer in C++. It allocates memory on the heap and returns a pointer to the allocated memory. The expression new int allocates memory for a single integer and returns a pointer to that memory block. It is important to later free the memory using delete to avoid memory leaks.
What is the correct way to declare a reference in C++?
a) int& x = y;
b) int& x;
c) int& x = &y;
d) int& x = *y;
Answer: a) int& x = y;
Explanation: Option a is the correct way to declare a reference in C++. It declares a reference variable named x that refers to an existing integer variable y. The & symbol is used to indicate a reference variable.
What is the output of the following C++ code?
#include
class Base {
public:
virtual void foo() {
std::cout << “Base foo” << std::endl;
}
};
class Derived : public Base {
public:
void foo() override {
std::cout << “Derived foo” << std::endl; } }; int main() { Base* obj = new Derived(); obj->foo();
return 0;
}
a) Base foo
b) Derived foo
c) Compiler error
d) Runtime error
Answer: b) Derived foo
Explanation: The code demonstrates polymorphism in C++ using virtual functions. The foo() function is declared as virtual in the Base class, and it is overridden in the Derived class. When a pointer to Base class (obj) is used to point to an object of Derived class, and the foo() function is called on that object through the pointer, the overridden version of foo() in the Derived class is called, and “Derived foo” is printed as output.
Which of the following is the correct way to declare a constant member function in a C++ class?
a) void func() const;
b) const void func();
c) const void func() const;
d) void const func();
Answer: a) void func() const;
Explanation: Option a is the correct way to declare a constant member function in C++. The const keyword is placed after the function declaration, before the function body. This indicates that the function does not modify the object on which it is called, and it can be called on constant objects as well.
What is the size of an empty class in C++?
a) 0 bytes
b) 1 byte
c) 4 bytes
d) It depends on the compiler
Answer: a) 0 bytes
Explanation: An empty class in C++ does not contain any data members or member functions. Therefore, it does not occupy any memory space, and its size is 0 bytes. However, due to compiler optimizations and alignment requirements, the size of an empty class may not always be 0 bytes, but it will be at least 1 byte.
Which of the following is NOT a valid C++ type conversion?
a) static_cast
b) dynamic_cast
c) type_cast
d) reinterpret_cast
Answer: c) type_cast
Explanation: Option c is not a valid type conversion in C++. The correct type conversion operators in C++ are static_cast, dynamic_cast, and reinterpret_cast. static_cast is used for most general type conversions, dynamic_cast is used for type conversions involving polymorphic classes, and reinterpret_cast is used for low-level type conversions.
What is the correct way to declare a pure virtual function in C++?
a) virtual void foo();
b) virtual void foo() = 0;
c) void virtual foo() = 0;
d) void foo() = 0;
Answer: b) virtual void foo() = 0;
Explanation: Option b is the correct way to declare a pure virtual function in C++. A pure virtual function is a virtual function that has no implementation in the declaring class and is meant to be overridden by derived classes. The = 0 at the end of the function declaration indicates that the function is pure virtual.
What is the correct way to define a constant member variable in a C++ class?
a) const int x;
b) int const x;
c) static const int x;
d) static int const x;
Answer: c) static const int x;
Explanation: Option c is the correct way to define a constant member variable in C++. The const keyword is used to indicate that the member variable is constant and cannot be modified after initialization. The static keyword indicates that the member variable is shared by all objects of the class, and it has only one instance in the class.
What is the output of the following C++ code?
#include
int main() {
int arr[5] = {1, 2, 3, 4, 5};
int* ptr = arr;
ptr += 2;
std::cout << *ptr << std::endl;
return 0;
}
a) 1
b) 2
c) 3
d) Compiler error
Answer: c) 3
Explanation: The code demonstrates pointer arithmetic in C++. The pointer ptr is initially pointing to the first element of the array arr. Then, ptr is incremented by 2, which makes it point to the third element of arr. The *ptr then gives the value at the third element, which is 3.
Which of the following is NOT a storage class specifier in C++?
a) auto
b) static
c) dynamic
d) register
Answer: c) dynamic
Explanation: Option c, “dynamic”, is not a storage class specifier in C++. The correct storage class specifiers in C++ are auto, static, register, and extern. These specifiers are used to define the storage duration and scope of variables in C++.
What is the scope of a global variable in C++?
a) Limited to the function it is declared in
b) Limited to the file it is declared in
c) Limited to the class it is declared in
d) Limited to the block it is declared in
Answer: b) Limited to the file it is declared in
Explanation: In C++, a global variable has a scope that is limited to the file it is declared in. It can be accessed from any function within the same file. However, it is not visible outside of the file, meaning it is not accessible from other files in the same program.
What is the purpose of the const keyword in C++?
a) To declare a constant variable
b) To indicate that a function does not modify the object it is called on
c) To define a constant member function
d) All of the above
Answer: d) All of the above
Explanation: The const keyword in C++ serves multiple purposes. It can be used to declare a constant variable, indicate that a function does not modify the object it is called on (const member function), and define a constant member function. It is used to enforce immutability and prevent modifications to certain variables or objects in C++.
What is the correct syntax to define a template class in C++?
a) template class MyClass {}
b) template class MyClass {}
c) template class MyClass;
d) template class MyClass;
Answer: b) template class MyClass {}
Explanation: Option b is the correct syntax to define a template class in C++. The typename keyword is used to indicate the type parameter in a template class. Although class can also be used interchangeably with typename, using typename is considered more modern and preferred in C++.
Note: Plagiarism is strictly prohibited. The questions and explanations provided above are unique and created by the AI model to the best of its knowledge. However, it is always recommended to cross-verify and validate the information from reliable sources before using it in any official or educational capacity.
About C++
Let’s learn about the topic. C++ is an intermediate level language and also comprises the features of both the high level as well as the low-level language. Also, the language C++ is a statically typed, multiparadigm, free-form, compiled general-purpose language. Moreover, this language is an object-oriented language. Also, it has some features like Virtual and Friend, violate some of the essential elements that render the language unworthy of being completely called the OOPS. Moreover, the primary purpose of the language is to make the programmers good at writing together with the individual programming skill and logical thinking.
Features of C++ or OOPS language.
- Encapsulation
- Data Hiding
- Polymorphism
- Inheritance
- The low memory manipulation features.
- The language is efficient having less compiled time.
Benefits Of Practicing The C++ Quiz
- Programmers can improve their logical ability as well as capable of programming.
- Moreover, you can know how to write and trace the programme.
- Enhance your coding skills.
- Also, you can implement the inline functions while coding.
How To Check C++ Programming Online Test Results
Thus, as fast as possible answer the question in the perspective time. Also, one can know their performance level. Moreover, one thing you need to note is that we provide you the appropriate answers and the explanation for every question. Therefore, it reduces the errors happen in future. So, after the successful submission of the exam or the C++ MCQ Online Test, we advise intenders to stay on this page without refreshing the article.
Hope all the contenders satisfied with the concept C++. So, for more such type of questions and answers stay tuned to our Freshers Now site.