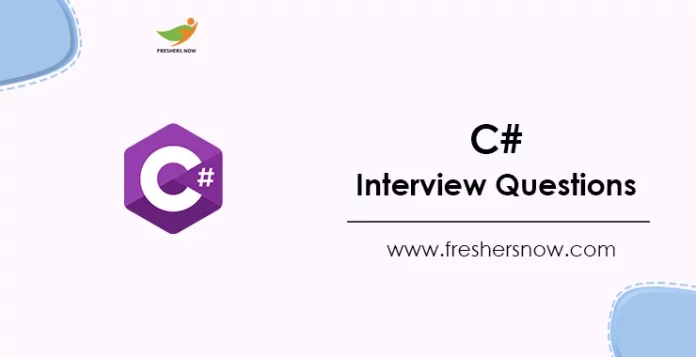
C# Interview Questions and Answers: C# is a popular object-oriented programming language that has gained immense popularity over the years, owing to its versatility and flexibility. Aspiring developers looking to start their career in C# development must have a strong foundation of the language and its concepts. To help them prepare for C# technical interviews, we have compiled a list of the top 100 C# interview questions and answers.
★★ Latest Technical Interview Questions ★★
C# Technical Interview Questions
This comprehensive list includes the latest C# interview questions that cover all aspects of the language, from the basic syntax to advanced topics like multithreading, LINQ, and more. Whether you’re a fresher or an experienced professional, these C# interview questions for freshers and technical interviews will help you brush up your knowledge and ace your next C# interview.
Top 100 C# Interview Questions and Answers
1. What is C#?
- C# (pronounced “C sharp”) is an object-oriented programming language designed and developed by Microsoft for building various types of applications that run on the .NET Framework.
- It is widely used to create Windows desktop applications, games, web applications, mobile apps, and more.
2. What is the difference between C# and other programming languages?
- C# is a strongly-typed language, which means that every variable or object must be explicitly declared with a data type.
- It is a statically-typed language, which means that the data types of variables are checked at compile-time.
- C# is an object-oriented language, which means that it uses classes and objects to organize code.
- C# has a garbage collector that automatically frees up memory for unused objects.
3. What are the data types in C#?
- C# has two main categories of data types: value types and reference types.
- Value types include primitive types like int, float, double, and bool, as well as user-defined structs.
- Reference types include objects, strings, and user-defined classes.
4. What is the syntax for declaring a variable in C#?
To declare a variable in C#, you use the syntax:
type variableName = value; |
5. What is Common Language Runtime (CLR)?
he execution of programs in various languages, including C#, is managed by the Common Language Runtime (CLR) architecture. The CLR is responsible for handling memory management, garbage collection, security management, and other related tasks.
6. What are the access modifiers in C#?
- C# has five access modifiers: public, private, protected, internal, and protected internal.
- Public members can be accessed from any code.
- Private members can only be accessed within the same class.
- Protected members can be accessed within the same class or any subclass.
- Internal members can be accessed within the same assembly (i.e., the same .dll or .exe file).
- Protected internal members can be accessed within the same assembly or any subclass outside the assembly.
7. What is the difference between value types and reference types in C#?
Aspect | Value Types | Reference Types |
---|---|---|
Definition | Data types that hold a value directly | Data types that hold a reference to an object |
Memory allocation | Stack | Heap |
Assignment | Copy the value to the new variable | Copy the reference to the new variable |
Default value | Depends on the data type | null |
Equality | Compare the values | Compare the references |
Performance | Faster, as they don’t require heap allocation | Slower, as they require heap allocation |
Examples | int, float, double, char, bool, struct | string, object, class, interface, delegate |
8. What are the different types of loops in C#?
- C# supports three types of loops: for, while, and do-while.
- The for loop is used to iterate over a sequence of values.
- The while loop is used to execute a block of code repeatedly as long as a certain condition is true.
- The do-while loop is similar to the while loop, but it guarantees that the loop body will be executed at least once.
9. How does exception handling work in C#?
- Exception handling in C# is done using try-catch-finally blocks.
- The try block contains the code that might throw an exception.
- The catch block is used to catch and handle the exception.
- The finally block is used to execute code that should be run regardless of whether an exception was thrown.
10. What is the syntax for defining a method in C#?
To define a method in C#, you use the syntax:
accessModifier returnType methodName(parameters) { // method body } |
11. What is object-oriented programming in C#?
- Object-oriented programming (OOP) is a programming paradigm that uses objects and classes to organize code.
- C# is an object-oriented language, which means that it provides support for OOP concepts like encapsulation, inheritance, and polymorphism.
12. What is inheritance in C#?
- Inheritance is a key feature of object-oriented programming that allows a subclass to inherit the properties and methods of its superclass.
- In C#, inheritance is achieved using the “extends” keyword.
- The subclass can override methods of the superclass and add new methods and properties.
13. What is polymorphism in C#?
- Polymorphism is another important concept in OOP that allows objects of different classes to be treated as if they are of the same type.
- C# supports polymorphism through inheritance and interfaces.
- Polymorphism allows for code reuse and simplifies the maintenance of large codebases.
14. What is the difference between a delegate and an event in C#?
Aspect | Delegate | Event |
---|---|---|
Definition | A type that represents a method signature | A mechanism for sending notifications |
Syntax | delegate void MyDelegate(string message); | public event EventHandler MyEvent; |
Usage | Used to call a method indirectly | Used to trigger an action in response to an event |
Invocation | Invoked using the () operator | Triggered by raising an event |
Handling | Can be handled by multiple subscribers | Can be handled by multiple event handlers |
Multicast | Supports multicast delegates | Supports multicast events |
Null Handling | Can be assigned null | Cannot be assigned null |
Examples | Action, Func, Predicate, custom delegates | Click, MouseMove, KeyPress, custom events |
15. What is encapsulation in C#?
- Encapsulation is the practice of hiding the implementation details of an object from the outside world.
- In C#, encapsulation is achieved using access modifiers like public, private, and protected.
- Encapsulation helps to ensure that the internal state of an object is not accidentally modified from outside the class.
16. What is abstraction in C#?
- Abstraction is the process of hiding implementation details while only showing the necessary information to the user.
- In C#, abstraction can be achieved through abstract classes, interfaces, and access modifiers.
17. What is a delegate in C#?
- A delegate is a type that represents a reference to a method with a specific signature.
- It allows methods to be passed as parameters, making it useful for implementing callback methods.
18. What is the syntax for creating a class in C#?
To create a class in C#, you use the syntax:
accessModifier class ClassName { // class members } |
19. What is a lambda expression in C#?
- A lambda expression is a concise way to represent an anonymous method in C#.
- It allows the creation of functions without defining a separate method and is commonly used in LINQ queries.
20. What are the main reasons to use C# language?
Here are the rewritten sentences in bullet points:
- C# is an easy language to learn
- C# is a general-purpose, object-oriented programming language
- C# is component-oriented
- C# is a structured language
- C# can be compiled on a variety of computer platforms
- C# produces efficient programs
- C# is part of the .NET Framework
21. What is a thread in C#?
- A thread is a separate execution path in a program that runs concurrently with the main program.
- It allows for multitasking and parallel processing, which can improve program performance.
22. What is the syntax for creating a constructor in C#?
To create a constructor in C#, you use the syntax:
accessModifier ClassName(parameters) { // constructor body } |
23. What is the difference between “throw” and “throw ex” in C#?
throw | throw ex | |
---|---|---|
Syntax | throw <expression>; | throw ex <expression>; |
Behavior | throws the exception and preserves the original stack trace | throws the exception and replaces the original stack trace with the current one |
Use case | Used to propagate an exception that has been caught | Used to propagate an exception that has been caught, but with a modified stack trace |
24. What is a lock in C#?
- A lock is a synchronization mechanism that prevents multiple threads from accessing a shared resource simultaneously.
- It is used to avoid race conditions and maintain data consistency in multithreaded applications.
25. What is the difference between a struct and a class in C#?
- A struct is a value type, while a class is a reference type in C#.
- Structs are stored on the stack, while classes are stored on the heap.
- Structs are typically used for lightweight objects, while classes are used for more complex objects.
26. What is a namespace in C#?
- A namespace is a way to organize code in C#.
- It provides a hierarchical structure for grouping related types and prevents naming conflicts between different code elements.
27. What is the syntax for creating a for loop in C#?
To create a for loop in C#, you use the syntax:
for (initialization; condition; increment) { // loop body } |
28. What is an interface in C#?
- An interface is a contract that specifies a set of methods, properties, and events that a class must implement.
- It allows for abstraction and loose coupling, making code more modular and flexible.
29. What is a constructor in C#?
- A constructor is a special method that is used to initialize objects in C#.
- It is called automatically when an object is created and can take parameters to set initial values for object properties.
30. What is a static constructor in C#?
- A static constructor in C# is a special type of constructor that is used to initialize static data members of a class.
- It is called only once, automatically before the first instance of the class is created or any static members are referenced.
- It can’t take any parameters, and its access modifier must be private.
- The purpose of a static constructor is to ensure that static members are initialized before they are accessed.
31. What is the syntax for creating a switch statement in C#?
To create a switch statement in C#, you use the syntax:
switch (expression) { case value1: // statement(s); break; case value2: // statement(s); break; default: // statement(s); break; } |
32. What are the differences between “override” and “new” keywords in C#?
override | new | |
---|---|---|
Syntax | override <member-declaration> | new <member-declaration> |
Inheritance | Can only be used to override a member inherited from a base class | Can be used to hide a member inherited from a base class or to declare a member with the same name as a member in the base class, but with different implementation |
Polymorphism | Supports polymorphism, which means that the runtime type of the object is used to determine which implementation of the member to call | Does not support polymorphism, which means that the implementation of the member in the declaring class is always called |
Required inheritance | Requires the member being overridden to be declared as virtual, abstract, or override in the base class | Does not require any specific declaration in the base class |
Method resolution | Uses runtime method resolution to determine which implementation of the member to call based on the runtime type of the object | Uses compile-time method resolution to determine which implementation of the member to call based on the declared type of the variable |
Access modifier | Can be used to change the access modifier of the overridden member, but cannot reduce its visibility | Can be used to change the access modifier of the member being hidden or declared, including reducing its visibility |
33. What is an abstract class in C#?
- An abstract class in C# is a class that cannot be instantiated.
- It is designed to be a base class for other classes to inherit from and provide a common implementation.
- It may contain abstract and non-abstract members, and derived classes must implement the abstract members.
- It is marked with the ‘abstract’ keyword, and cannot be sealed or static.
34. What is a sealed class in C#?
- A sealed class in C# is a class that cannot be inherited from.
- It is marked with the ‘sealed’ keyword, and it prevents further derivation of the class hierarchy.
- It is often used to protect the implementation details of a class from being modified or extended.
35. What is a partial class in C#?
- A partial class in C# is a class that is split into multiple files.
- Each file contains a part of the class definition, and they are combined by the compiler to create a single class.
- It is often used to separate auto-generated code from user-written code or to break up a large class into smaller, more manageable pieces.
36. What is a generic class in C#?
- A generic class in C# is a class that can operate on multiple data types without having to be rewritten for each type.
- It is defined using type parameters, which are specified when the class is instantiated.
- It can have generic methods and properties, and it is often used to create reusable data structures and algorithms.
37. What is the syntax for creating a try-catch block in C#?
To create a try-catch block in C#, you use the syntax:
try { // statement(s); } catch (ExceptionType e) { // statement(s); } |
38. What is a generic method in C#?
- A generic method in C# is a method that can operate on multiple data types without having to be rewritten for each type.
- It is defined using type parameters, which are specified when the method is called.
- It can be used to create reusable algorithms and functions.
39. What is the difference between “==” and “Equals()” methods in C#?
“==” Operator | “Equals()” Method | |
---|---|---|
Definition | A binary operator used to compare the values of two objects or variables. | A method used to compare the values of two objects or variables. |
Syntax | operand1 == operand2 | operand1.Equals(operand2) |
Usage | Used to compare value types and reference types. | Used to compare reference types. |
Behavior | Compares the values of the operands. | Compares the values of the operands. |
Overriding | The operator can be overloaded to provide custom comparison logic. | The method can be overridden to provide custom comparison logic. |
Performance | Performs faster than the Equals() method when comparing value types. | Performs slower than the == operator when comparing value types. |
Exception | Throws a compile-time error when used with incompatible types. | Does not throw a compile-time error, but can throw a runtime error when used with null values. |
Example | int x = 5; int y = 5; Console.WriteLine(x == y); // true | string s1 = “Hello”; string s2 = “Hello”; Console.WriteLine(s1.Equals(s2)); // true |
40. What is a property in C#?
- A property in C# is a member of a class that provides a way to read or write a private field in a controlled manner.
- It is defined using the ‘get’ and ‘set’ accessors, which allow the value of the property to be retrieved or set.
- It can have additional access modifiers to control its visibility and can be used to encapsulate the implementation details of a class.
41. What is a get accessor in C#?
- A get accessor in C# is used to retrieve the value of a property.
- It is defined using the ‘get’ keyword, and it can contain code to calculate the property’s value if necessary.
- It is often used to provide controlled access to private data members.
42. What is the syntax for creating a while loop in C#?
To create a while loop in C#, you use the syntax:
while (condition) { // loop body } |
43. What is a set accessor in C#?
- A set accessor in C# is used to set the value of a property.
- It is defined using the ‘set’ keyword, and it can contain code to validate the value being set or perform other operations.
- It is often used to provide controlled access to private data members.
44. What is an event in C#?
- An event in C# is a way for one object to notify other objects that something has happened.
- It is defined using the ‘event’ keyword and is often associated with a delegate type.
- It can be subscribed to by other objects, and they will receive notifications when the event is raised.
- It is commonly used in GUI programming to respond to user actions, such as button clicks or mouse movements.
45. What is a collection in C#?
- A collection in C# is a group of related objects.
- It is a class that is used to store and manipulate a group of related objects.
- Collections allow programmers to work with groups of objects in a more efficient and organized manner.
46. What is an array in C#?
- An array in C# is a data structure that stores a fixed-size sequential collection of elements of the same type.
- It is a collection of elements, where each element is identified by an index or a key.
- Arrays can be multidimensional, which means they can have more than one index.
47. What are the differences between a “string” and “StringBuilder” in C#?
“string” | “StringBuilder” | |
---|---|---|
Definition | A sequence of characters that represents text. | A mutable string that can be modified without creating a new object. |
Memory | Immutable – once created, cannot be changed. | Mutable – can be modified multiple times without creating a new object. |
Performance | Creating a new string every time a modification is made can be slow and memory-intensive. | Performs better when multiple modifications need to be made to the string. |
Usage | Used when the string is not going to change frequently. | Used when the string needs to be modified frequently. |
Concatenation | String concatenation with “+” operator creates a new string object every time. | Appending strings to StringBuilder using Append() method does not create a new object. |
Memory | Consumes more memory because a new string object is created every time. | Consumes less memory because the same StringBuilder object is modified. |
Example | string greeting = “Hello, World!”; Console.WriteLine(greeting); | StringBuilder sb = new StringBuilder(); sb.Append(“Hello”); sb.Append(“, World!”); Console.WriteLine(sb.ToString()); |
48. What is a dictionary in C#?
- A dictionary in C# is a collection that stores key-value pairs.
- It is similar to an array, but instead of using integers to index elements, dictionaries use keys.
- The keys in a dictionary must be unique, while the values can be duplicated.
49. What is a list in C#?
- A list in C# is a collection that stores an ordered sequence of elements.
- It is similar to an array, but it can grow or shrink dynamically as elements are added or removed.
- Lists can store elements of any data type.
50. What is the syntax for creating a do-while loop in C#?
To create a do-while loop in C#, you use the syntax:
do { // loop body } while (condition); |
51. What is a tuple in C#?
- A tuple in C# is a data structure that contains a fixed number of elements, each of which can be of a different type.
- It is a lightweight way to combine multiple values into a single object.
- Tuples can be used as a return type for methods that need to return multiple values.
52. What is a nullable type in C#?
- A nullable type in C# is a value type that can also be assigned a null value.
- Normally, value types cannot be assigned a null value.
- Nullable types are useful when working with databases or other data sources that can have missing values.
53. What is a value type in C#?
- A value type in C# is a type that stores its value directly in memory.
- Examples of value types include int, float, bool, and struct.
- Value types are copied when they are passed as parameters or assigned to variables.
54. What is a stack in C#?
A stack is a data structure in C# that follows the Last-In-First-Out (LIFO) principle. It means that the last element added to the stack is the first element to be removed from it.
Some common operations performed on a stack in C# include:
- Push: Adding an element to the top of the stack
- Pop: Removing the top element from the stack
- Peek: Returning the top element of the stack without removing it
- Count: Returning the number of elements in the stack
- Clear: Removing all elements from the stack
Stacks are used in various programming scenarios, such as parsing expressions, implementing recursive algorithms, and undo-redo functionality.
55. What is the difference between “lock” and “Monitor” in C#?
Feature | lock | Monitor |
---|---|---|
Implementation | Implemented as a keyword in C# | Implemented as a static class in C# |
Purpose | Ensures mutual exclusion for a shared resource | Provides a way to synchronize threads in C# |
Syntax | lock (object) { … } |
Monitor.Enter(object); ... Monitor.Exit(object); |
Exception Handling | Automatically releases lock on exception | Need to manually release lock using try-finally block |
Multiple Locks | Cannot lock multiple objects at once | Can lock multiple objects using overload of Enter method |
Wait and Pulse | Cannot be used for wait and pulse operations | Provides Wait, Pulse, and PulseAll methods for signaling between threads |
Performance | Generally faster due to optimized implementation | May be slower due to more complex implementation |
56. What is a queue in C#?
A queue is a data structure in C# that follows the First-In-First-Out (FIFO) principle. It means that the first element added to the queue is the first element to be removed from it.
Some common operations performed on a queue in C# include:
- Enqueue: Adding an element to the back of the queue
- Dequeue: Removing the front element from the queue
- Peek: Returning the front element of the queue without removing it
- Count: Returning the number of elements in the queue
- Clear: Removing all elements from the queue
Queues are used in various programming scenarios, such as scheduling tasks, implementing a message queue, and breadth-first search algorithms.
57. What is the syntax for creating an if-else statement in C#?
To create an if-else statement in C#, you use the syntax:
if (condition) { // statement(s); } else { // statement(s); } |
58. What is a HashSet in C#?
- A HashSet is a collection in C# that stores unique elements.
- It uses hashing to achieve constant-time performance for operations like adding, removing, and checking for the presence of elements.
- HashSet is part of the System.Collections.Generic namespace in C#.
59. What is an IDictionary in C#?
- IDictionary is an interface in C# that represents a collection of key-value pairs.
- It allows for efficient lookup of values based on their keys.
- IDictionary is part of the System.Collections.Generic namespace in C#.
60. What is the difference between “foreach” and “for” loops in C#?
Feature | foreach | for |
---|---|---|
Syntax | foreach (var <variable> in <collection>) { <statements> } | for (<initializer>; <condition>; <increment>) { <statements> } |
Collection | Used to iterate over a collection of objects | Can be used to iterate over any sequence of values |
Type | Works with any type that implements the IEnumerable interface | Works with any type that can be iterated using an index |
Modifying elements | Cannot be used to modify elements in the collection | Can be used to modify elements in the sequence |
Index access | Does not support direct index access to the elements in the collection | Supports direct index access to the elements in the sequence |
Performance | Can be slower for large collections | Generally faster for large sequences |
Syntax sugar | Provides a simpler syntax and reduces code complexity | Provides more control and flexibility over the iteration process |
61. What is an IEnumerator in C#?
- IEnumerator is an interface in C# that provides a way to iterate over a collection of elements.
- It defines two methods: MoveNext(), which advances the enumerator to the next element in the collection, and Reset(), which resets the enumerator to its initial position.
- IEnumerator is part of the System.Collections namespace in C#.
62. What is an IEnumerable in C#?
- IEnumerable is an interface in C# that represents a collection of elements that can be enumerated.
- It defines a single method: GetEnumerator(), which returns an IEnumerator object that can be used to iterate over the elements of the collection.
- IEnumerable is part of the System.Collections namespace in C#.
63. What is a delegate chain in C#?
- A delegate chain is a series of delegates that are called in order to perform a specific action.
- In C#, multiple delegates can be combined into a single delegate using the “+” operator.
- When a delegate chain is called, each delegate in the chain is invoked in turn.
64. What is an anonymous method in C#?
- An anonymous method is a method that has no name and is defined inline with the rest of the code.
- Anonymous methods are typically used as event handlers or as parameters to methods that expect delegates.
- Anonymous methods were introduced in C# 2.0.
65. What is a multicast delegate in C#?
- A multicast delegate is a delegate that can have multiple methods assigned to it.
- When a multicast delegate is called, all the methods in the delegate chain are called in the order in which they were added.
- Multicast delegates are useful for implementing event handlers in C#.
66. What is a covariance in C#?
- Covariance is a feature in C# that allows for implicit conversion of a derived class to its base class.
- Covariance is supported for arrays and generic interfaces.
- Covariance is useful for writing more flexible and reusable code.
67. What is a contravariance in C#?
- Contravariance is a feature in C# that allows for implicit conversion of a base class to its derived class.
- Contravariance is supported for delegate types.
- Contravariance is useful for writing more flexible and reusable code.
68. What is a preprocessor directive in C#?
- A preprocessor directive is a statement in C# that is processed before the code is compiled.
- Preprocessor directives are used to include or exclude code based on certain conditions, define constants, and perform other operations.
- Preprocessor directives start with the “#” symbol and are evaluated by the compiler.
69. What is the #define directive in C#?
- The #define directive is used to define a symbol or constant that can be used throughout the program.
- It can be used to enable conditional compilation and to improve code readability.
70. What is the #if directive in C#?
- The #if directive is used to conditionally compile code based on the value of a symbol or constant.
- It allows for the creation of different versions of the same code, depending on the configuration of the program.
71. What is the #endif directive in C#?
- The #endif directive is used to mark the end of a conditional compilation block.
- It is used in conjunction with the #if directive to enable or disable sections of code based on preprocessor symbols.
72. What is the #else directive in C#?
- The #else directive is used in conjunction with the #if directive to create alternative code blocks.
- It is executed when the condition of the #if directive is false.
73. What is the #elif directive in C#?
- The #elif directive is used to test additional conditions after an initial #if directive.
- It is executed if the previous conditions of the #if or #elif directives were false.
74. What is the #warning directive in C#?
- The #warning directive is used to issue a warning message during compilation.
- It can be used to alert developers of potential issues or deprecated code.
75. What is the #error directive in C#?
- The #error directive is used to generate a compiler error during compilation.
- It can be used to prevent compilation of code that is known to cause issues.
76. What is a type converter in C#?
- A type converter is a class that is used to convert values between different data types in C#.
- It allows for the conversion of values from one type to another without the need for explicit casting.
77. What is the ToString() method in C#?
- The ToString() method is used to convert an object to its string representation in C#.
- It is used to display objects in a human-readable format.
78. What is the GetHashCode() method in C#?
- The GetHashCode() method is used to generate a hash code for an object in C#.
- It is used in conjunction with hashing algorithms and data structures like hash tables.
79. What is the Equals() method in C#?
- The Equals() method in C# is used to compare two objects for equality.
- It returns a boolean value indicating whether the two objects are equal or not.
- It is inherited from the Object class and can be overridden by derived classes to provide custom equality comparison.
80. What is the GetType() method in C#?
- The GetType() method in C# is used to get the runtime type of an object.
- It returns a Type object that describes the type of the object.
- It is inherited from the Object class and can be used to perform runtime type checking and reflection.
81. What is the typeof() operator in C#?
- The typeof() operator in C# is used to get the Type object that represents a given type.
- It is used to obtain metadata about the type at compile time and can be used to perform reflection and create instances of the type.
82. What is the is operator in C#?
- The is operator in C# is used to check if an object is of a given type.
- It returns a boolean value indicating whether the object is an instance of the specified type or a derived type.
- It can be used to perform runtime type checking and casting.
83. What is the as operator in C#?
- The as operator in C# is used to perform a safe cast of an object to a given type.
- It returns null if the object is not an instance of the specified type or a derived type.
- It can be used to avoid exceptions when casting and to simplify code.
84. What is the default operator in C#?
- The default operator in C# is used to get the default value of a given type.
- It returns null for reference types, zero for numeric types, and the default value for other value types.
- It can be used to initialize variables and parameters with default values.
85. What is the ref keyword in C#?
- The ref keyword in C# is used to pass a variable by reference to a method.
- It allows the method to modify the value of the variable in the calling code.
- It is often used to pass large objects and arrays to methods without creating a copy of the data.
86. What is the out keyword in C#?
- The out keyword in C# is used to pass a variable by reference to a method and also to initialize the variable.
- It allows the method to modify the value of the variable and also to return a value through the variable.
- It is often used to return multiple values from a method.
87. What is the params keyword in C#?
- The params keyword in C# is used to specify a variable number of arguments to a method.
- It allows the method to accept any number of arguments of the specified type, separated by commas.
- It is often used to create methods that can accept a variable number of arguments, such as formatting functions.
88. What is the yield keyword in C#?
- The yield keyword in C# is used to create an iterator method.
- It allows the method to return a sequence of values one at a time, without having to generate the entire sequence up front.
- It is often used to create custom collection classes and to simplify code that generates sequences of values.
89. What is a dynamic keyword in C#?
- The dynamic keyword is a type in C# that allows the runtime to determine the type of an object at runtime rather than compile time. It provides dynamic binding and late binding, allowing for more flexibility in coding.
- Some common operations performed using the dynamic keyword in C# include invoking methods and properties on objects without specifying their types, working with COM objects, and using dynamic languages.
90. What is a lock statement in C#?
- The lock statement in C# is used to ensure that a block of code is executed by only one thread at a time. It is used to synchronize access to shared resources in multithreaded applications.
- The lock statement works by obtaining a lock on a specified object, executing the block of code, and releasing the lock when the block is completed. If another thread attempts to obtain the lock while it is held by another thread, it will block until the lock is released.
91. What is an extension method in C#?
- An extension method in C# is a static method that is defined in a static class and is used to add new functionality to an existing type without modifying the type’s source code.
- Extension methods allow for the creation of custom methods that can be used on types that were not originally designed to have those methods. They are commonly used to add functionality to third-party libraries or to improve code readability.
92. What is a partial method in C#?
- A partial method in C# is a method that is defined in a partial class but does not have a method body. It is used to declare a method signature that can be implemented by another part of the class.
- Partial methods are useful for allowing code generators to add methods to a class without overwriting the manual code written by the developer. They are also used to improve performance by avoiding the need to check if a method has been implemented before calling it.
93. What is a composition in C#?
- Composition in C# is a technique of creating complex objects by combining smaller objects. It is used to build objects that have complex behavior by combining simpler objects that each have a specific role.
- Composition is used to achieve greater flexibility and modularity in software design. It allows for the creation of objects that can be easily modified and extended, and it promotes code reuse.
94. What is the difference between an abstract class and an interface in C#?
In C#, an abstract class is a class that cannot be instantiated and is used as a base class for other classes. It can contain abstract methods, concrete methods, and fields.
An interface in C# is a contract that defines a set of methods and properties that a class must implement. It cannot contain method bodies, fields, or constructors.
The main differences between abstract classes and interfaces are:
- An abstract class can provide a default implementation of a method, while an interface cannot.
- A class can implement multiple interfaces, but it can only inherit from one abstract class.
- An abstract class can have fields and constructors, while an interface cannot.
95. What is the difference between var and dynamic in C#?
In C#, var is used to declare a variable whose type is inferred by the compiler at compile time, while dynamic is used to declare a variable whose type is determined at runtime.
The main differences between var and dynamic are:
- The type of a var variable is fixed at compile time and cannot be changed, while the type of a dynamic variable can change at runtime.
- Var can only be used with local variables, while dynamic can be used with any type of variable.
- Var is used for static type checking, while dynamic is used for dynamic type checking.
- Var is a compile-time construct, while dynamic is a runtime construct.
96. What is a resource file in C#?
- A resource file in C# is a file that contains data that can be accessed and used by an application. It can contain strings, images, audio files, and other types of data.
- Resource files are used to separate application data from code, making it easier to localize, update, and maintain an application. They are commonly used for storing user interface elements, error messages, and other types of data that need to be easily modified.
97. What is a try-catch-finally block in C#?
- A try-catch-finally block in C# is used to handle exceptions that may occur during the execution of a block of code. It consists of a try block, one or more catch blocks, and an optional finally block.
- The try block contains the code that may throw an exception, and the catch block contains the code that handles the exception. The finally block is used to ensure that a piece of code is executed regardless of whether an exception is thrown or not.
- The try-catch-finally block is used to ensure that an application does not crash when an unexpected error occurs. It allows for the graceful handling of errors and the preservation of data integrity.
98. What is garbage collection in C#?
Garbage collection is the process of releasing memory captured by unnecessary objects. When a class object is created, memory space is automatically allocated to the object in the heap memory. Once all actions on the object are performed, the memory space occupied by the object becomes useless and needs to be freed up. Garbage collection is triggered in three scenarios: when the occupied memory by objects exceeds the pre-set threshold value, when the garbage collection method is invoked, or when the physical memory of the system is low.
99. What is LINQ in C#?
- LINQ (Language Integrated Query) is a set of language extensions in C# that allow queries to be written against various data sources.
- It allows the use of SQL-like syntax to query objects, arrays, databases, and other data sources.
100. What is ArrayList?
An ArrayList is a type of dynamic array that allows elements to be added or removed at runtime, but does not automatically sort those elements.
The Top 100 C# Interview Questions and Answers provide a comprehensive guide for aspiring developers to master C# concepts and prepare for technical interviews. To enhance your knowledge, do follow us at freshersnow.com, where we offer a plethora of resources to help you expand your understanding and excel in your career.