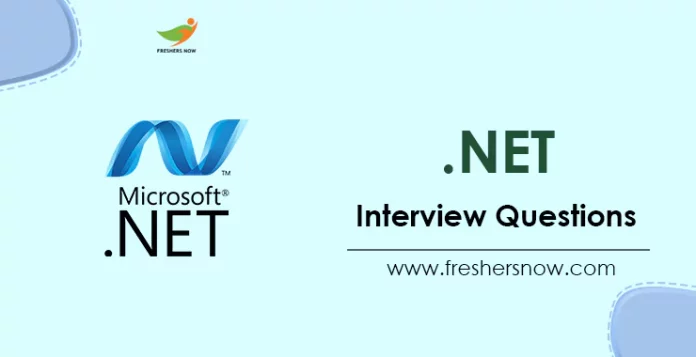
This article provides Top 100 .NET Interview Questions and Answers which will help you to excel in your interview and secure your desired job.
★★ Latest Technical Interview Questions ★★
.NET Technical Interview Questions
By familiarizing themselves with the key concepts, features, and applications of .NET, candidates can gain the confidence to tackle any .NET-related questions that may arise during their interviews. .NET Technical Interview Questions are designed to assess a candidate’s knowledge and skills related to the .NET framework. The Top .NET Technical Interview Questions are designed to determine a candidate’s level of expertise in .NET development and their ability to work with different technologies and tools within the .NET ecosystem.
Top 100 .NET Interview Questions and Answers
1. What is .NET Framework and what are its main components?
.NET Framework is a software development framework developed by Microsoft that allows developers to create and run applications for Windows operating systems. Its main components include the Common Language Runtime (CLR), the Framework Class Library (FCL), and various development tools and languages.
2. What is CLR and how does it work in .NET Framework?
CLR (Common Language Runtime) is the component of the .NET Framework that executes code written in any .NET compatible language. It provides various services such as memory management, security, and exception handling. CLR converts the .NET code into machine code that can be understood by the computer.
3. How does the .NET framework work?
4. What is the syntax for declaring a variable in C#?
The syntax for declaring a variable in C# is
datatype variableName = initialValue;
5. What are the advantages of using the .NET Framework for developing applications?
- Platform independence: .NET Framework applications can run on multiple platforms including Windows, Linux, and macOS.
- Language interoperability: The .NET Framework allows developers to use multiple programming languages such as C#, VB, and F# to develop applications.
- Rapid development: The .NET Framework provides a rich set of libraries and tools that simplify and accelerate application development.
- Security: The .NET Framework provides built-in security features that protect applications from malicious attacks.
6. Explain the different parts of an Assembly.
7. What is the difference between .NET Core and .NET Framework?
.NET Core | .NET Framework |
---|---|
Cross-platform, runs on Windows, Linux, and macOS | Windows-only |
Open-source | Proprietary |
Lightweight and modular | Monolithic and heavy |
Supports microservices and container-based development | Designed for traditional desktop and web applications |
A limited set of APIs and libraries compared to .NET Framework | A comprehensive set of APIs and libraries |
Best suited for cloud-based and web-based applications | Best suited for Windows desktop applications |
8. What is C# and why is it commonly used in .NET development?
C# is a programming language developed by Microsoft that is commonly used in .NET development. It is an object-oriented language that is similar to Java and C++. C# is easy to learn and use, and it is highly compatible with other .NET languages.
9. Explain about types of Common Type Systems (CTS).
10. What are the common data types in C#?
Numeric data types: These include integer types, floating-point types, and decimal types.
- Integer types: byte, byte, short, short, int, uint, long
- Floating-point types: float, double
- Decimal types: decimal
- Boolean data type: bool
- Character data types: char
- String data type: string
- Object data type: object
11. What is the syntax for writing a switch statement in C#?
The syntax for writing a switch statement in C# is:
switch (variable) { case value1: // code block break; case value2: // code block break; default: // code block break; }
12. What is the difference between value types and reference types in C#?
Value Types | Reference Types |
---|---|
Stores data directly in memory | Stores a reference to data in memory |
Simple data types such as integers, booleans, and floats | Complex data types such as objects, arrays, and strings |
Allocated on the stack | Allocated on the heap |
Faster access and performance | Slower access and performance |
Copied by value when passed to a method or assigned to a variable | Copied by reference when passed to a method or assigned to a variable |
Examples include int, bool, and float | Examples include object, array, and string |
13. Explain Explicit Compilation (Ahead Of Time compilation).
- Ahead-of-time(AOT) compilation is the process of compiling a high-level language into a low-level language during build-time, i.e., before program execution. AOT compilation reduces the workload during run time.
- AOT provides faster start-up time, in larger applications where most of the code executes on startup. But it needs more amount of disk space and memory or virtual address space to hold both IL(Intermediate Language) and precompiled images. In this case, the JIT(Just In Time) Compiler will do a lot of work like disk I/O actions, which are expensive.
14. What is the use of the ‘using’ keyword in C#?
The ‘using’ keyword in C# is used to define a scope for objects that need to be disposed of when they are no longer needed. This is useful for managing resources like database connections and file handles.
15. What is JWT and how is it used for web application authentication in .NET?
- JWT (JSON Web Tokens) is a standard for representing claims securely between two parties.
- It can be used for authentication and authorization in web applications.
- JWT is a compact, URL-safe means of representing claims to be transferred between two parties.
16. What is the syntax for using a lambda expression in C#?
The syntax for using a lambda expression in C# is:
(parameterList) => expression;
17. What is the syntax for creating a class in C#?
The syntax for creating a class in C# is:
accessModifier class ClassName { // class members }c
18. What is a delegate in C#?
A delegate in C# is a type that represents a method signature. It allows methods to be passed as parameters and enables event handling and callback mechanisms.
19. What is an interface in C# and how is it different from a class?
An interface in C# is a type that defines a set of methods and properties that a class can implement. It is different from a class in that it cannot be instantiated directly and can be implemented by multiple classes.
20. What is a constructor in C# and how is it used?
A constructor in C# is a special method that is called when an object of a class is created. It is used to initialize the state of the object and can have parameters to customize the initialization process.
21. What is an abstract class in C# and how is it used?
An abstract class in C# is a class that cannot be instantiated directly and is intended to be subclassed. It can contain abstract methods that must be implemented by the subclasses.
22. What is a sealed class in C# and how is it used?
A sealed class in C# is a class that cannot be subclassed. It is used to prevent further inheritance of a class and ensure that its behavior is consistent across all instances.
23. What is inheritance in C#?
Inheritance in C# is the concept of creating a new class by deriving properties and methods from an existing class. The new class, known as the derived class, can inherit all the public, protected and internal members of the base class. It is used to reuse code and simplify the code structure.
24. What is polymorphism in C# and how is it used?
Polymorphism is the ability of an object to take on multiple forms. In C#, it is achieved through method overloading and method overriding. Method overloading is the ability to have multiple methods with the same name but different parameters, while method overriding is the ability to change the implementation of a virtual or abstract method in a derived class. Polymorphism is used to create flexible and extensible code.
25. What is a namespace in C#?
A namespace in C# is a container for a group of related classes, interfaces, structures, enums, and delegates. It is used to organize code and avoid naming conflicts between classes. A namespace can be declared using the “namespace” keyword, and its members can be accessed using the dot notation.
26. What is the syntax for creating an interface in C#?
The syntax for creating an interface in C# is:
accessModifier interface InterfaceName { // interface members }
27. What are access modifiers in C#?
Access modifiers in C# are keywords that determine the level of access that a class member has from outside the class. The four access modifiers in C# are “public”, “private”, “protected” and “internal”. “Public” members can be accessed from anywhere, “private” members can only be accessed within the class, “protected” members can be accessed within the class and its derived classes, and “internal” members can be accessed within the same assembly. Access modifiers are used to control the visibility of class members and ensure data encapsulation.
28. What is the difference between ‘read-only’ and ‘const’ in C#?
‘read-only’ | ‘const’ |
---|---|
Can be initialized either at the time of declaration or later | Must be initialized at the time of declaration |
Can be initialized using a constructor | Cannot be initialized using a constructor |
Can be initialized with a non-constant expression | Can only be initialized with a constant expression |
Can be of any data type | Can only be of value types, enums, or string data type |
Value can be changed using the ‘readonly’ keyword | Value cannot be changed during runtime or at compile time |
Must be declared as an instance-level field | Can be declared as a field of a class or a local variable |
29. What is LINQ and how is it used in .NET development?
LINQ (Language Integrated Query) is a set of language extensions in C# that provides a powerful and expressive way to query data from various sources, including databases, XML documents, and collections. It allows developers to write queries using familiar SQL-like syntax, and provides type checking at compile-time. LINQ is used in .NET development to simplify and speed up data access and manipulation.
30. What is Dependency Injection in .NET and how is it used?
Dependency Injection is a design pattern used in .NET development to achieve loose coupling between components. It is a process of injecting dependencies into an object rather than creating them within the object itself. This allows for better testability, maintainability, and flexibility in the code. Dependency Injection can be implemented in various ways in .NET, such as Constructor Injection, Property Injection, and Method Injection.
31. What is a Unit Test in .NET and how is it written?
A Unit Test is a type of automated test used in .NET development to verify the behavior of a single unit of code, such as a method or function. It is written to test a specific scenario or use case of the code and ensures that it produces the expected output. Unit tests are typically written using a testing framework, such as NUnit or MSTest, and can be run automatically to detect and prevent bugs.
32. What is SignalR and how is it used for real-time web applications in .NET?
- SignalR is a framework used for building real-time web applications in .NET.
- It allows developers to add real-time web functionality to their applications by enabling server-side code to push content to connected clients as soon as it becomes available.
- SignalR supports multiple transport protocols, including WebSockets, Server-Sent Events (SSE), Long Polling, and others, to provide the best possible experience for different types of clients and browsers.
33. What is the syntax for creating a constructor in C#?
The syntax for creating a constructor in C# is:
accessModifier ClassName(parameterList) { // constructor code }
34. What is Moq and how is it used in .NET testing?
Moq is a popular mocking framework used in .NET testing to create mock objects for dependencies in Unit Tests. It allows for the creation of fake objects that simulate the behavior of real objects, which can be used to test code that relies on those objects. Moq is used to isolate the Unit Test from external dependencies, making the tests faster and more reliable.
35. What is NuGet and how is it used in .NET development?
NuGet is a package manager used in .NET development to manage and install third-party libraries and tools. It provides a centralized repository for developers to find and install packages, which can then be easily added as references to a project. NuGet makes it easier to manage dependencies and ensure that the latest version of a package is used in the project.
36. What is Entity Framework and how is it used for database access in .NET?
Entity Framework is an Object-Relational Mapping (ORM) framework used in .NET development for database access. It provides a way to interact with databases using .NET objects instead of writing raw SQL queries. Entity Framework supports different approaches for creating and configuring database models, such as Code-First and Database-First. It makes it easier to manage database connections and operations, and provides features such as change tracking and transactions.
37. What is the syntax for using a try-catch block in C#?
The syntax for using a try-catch block in C# is:
try { // code block } catch (ExceptionType e) { // code block }
38. What is the Code-First approach in Entity Framework?
Code-First is an approach in Entity Framework where the database schema is created based on the domain model classes defined in code. It allows for the creation of database models without the need for any pre-existing database. The code-First approach enables developers to focus on creating business logic and data access code without worrying about the database schema.
39. What is IIS and how is it used for web server deployment in .NET?
- IIS (Internet Information Services) is a web server developed by Microsoft for use on Windows operating systems.
- It provides a platform for hosting web applications and supports multiple protocols, including HTTP, HTTPS, FTP, SMTP, and others.
- IIS includes features such as load balancing, process isolation, and security, making it a popular choice for hosting web applications in .NET.
40. What is the Database-First approach in Entity Framework?
Database-First is an approach in Entity Framework where the database schema is generated from an existing database, and then the domain model classes are created based on the schema. It allows developers to start with an existing database schema and then create the necessary code to interact with the database. The database-First approach is useful when working with legacy databases or databases created by other teams.
41. What is the difference between Entity Framework and ADO.NET?
Entity Framework | ADO.NET |
---|---|
Object-relational mapper (ORM) that allows developers to work with data as objects | Data access technology that provides low-level access to data sources |
Uses LINQ to query data | Uses SQL to query data |
Provides automatic generation of SQL commands based on object model | Developers need to write SQL commands manually |
Offers support for domain-driven design and model-first development approaches | No specific support for domain-driven design or model-first development approaches |
Supports different database providers such as SQL Server, MySQL, Oracle, etc. | Requires different implementations for different database providers |
Requires a higher learning curve due to its complex architecture and features | Has a simpler architecture and is easier to learn |
42. What is WPF and how is it used for desktop application development in .NET?
WPF (Windows Presentation Foundation) is a graphical subsystem in .NET that is used to create desktop applications. It provides a unified framework for creating user interfaces that incorporate multimedia, animation, and graphics.
43. What is the syntax for creating a dictionary in C#?
The syntax for creating a dictionary in C# is: Dictionary<keyDataType, valueDataType> dictionaryName = new Dictionary<keyDataType>
44. What is Silverlight and how is it used for web application development in .NET?
Silverlight is a deprecated web application framework for .NET that enabled developers to create rich web applications with multimedia and animation capabilities. It has since been replaced by newer web frameworks like ASP.NET Core.
45. What is Xamarin and how is it used for mobile application development in .NET?
Xamarin is a cross-platform mobile application development framework that enables developers to create native mobile applications for iOS, Android, and Windows using .NET technologies. It allows developers to write code once and deploy it to multiple platforms, saving time and effort in the development process.
46. What is Azure and how is it used for cloud computing in .NET?
Azure is a cloud computing platform by Microsoft that offers a range of services such as virtual machines, web applications, databases, storage, and more. It enables developers to build, deploy, and manage applications in the cloud, making it an important part of the .NET development ecosystem.
47. What is OWIN and how is it used for web application authentication in .NET?
- OWIN (Open Web Interface for .NET) is a standard for building web applications in .NET.
- It decouples the web server from the web application, allowing developers to use different web servers (such as IIS or self-hosting) without changing the application code.
- OWIN includes a middleware pipeline that enables developers to add modular functionality to their web applications in a flexible and extensible way.
48. What is the syntax for using LINQ in C#?
The syntax for using LINQ in C# is:
var query = from element in collection where condition select element;
49. What is OAuth and how is it used for web application authorization in .NET?
- OAuth is a protocol used for authorization in web applications.
- It enables a user to grant a third-party application access to their resources on another site without sharing their credentials.
- OAuth provides a secure and standardized way for web applications to delegate authorization responsibilities to external services.
50. What is the difference between SQL Server and Oracle in .NET development?
SQL Server | Oracle |
---|---|
Developed by Microsoft | Developed by Oracle |
Supports Windows and Linux operating systems | Supports Windows, Linux, and Unix operating systems |
Uses T-SQL (Transact-SQL) | Uses PL/SQL |
Supports CLR integration for .NET code | Supports Java integration for Java code |
Has better integration with Visual Studio and .NET Framework | Has less integration with Visual Studio and .NET Framework |
Offers better performance for small and medium-sized databases | Offers better performance for large-scale databases |
Provides better support for business intelligence and data analysis | Provides better support for enterprise-level applications |
51. What is CORS and how is it used for cross-domain communication in .NET?
- CORS (Cross-Origin Resource Sharing) is a security feature used in web browsers to allow web pages to access resources from a different domain.
- It enables web pages to make AJAX requests to APIs hosted on different domains and is an essential security feature of modern web applications.
52. What is the syntax for using asynchronous programming in C#?
The syntax for using asynchronous programming in C# is:
async Task<returnType> methodName() { // code block await someTask; // code block }
53. What is the difference between synchronous and asynchronous programming in .NET?
Question | Synchronous Programming | Asynchronous Programming |
---|---|---|
What is it? | Executes code in a single thread of control, with each operation blocking the execution of subsequent code until it completes. | Executes code using multiple threads of control, allowing operations to be completed independently of one another. |
Execution | Blocks the execution of subsequent code until each operation completes. | Allows code to continue executing while operations are being completed. |
Performance | Generally less efficient, as threads may be blocked while waiting for operations to complete. | Generally more efficient, as threads can continue executing while operations are being completed. |
Examples | Traditional method calls, loops, and sequential code blocks. | Asynchronous method calls, event handlers, and parallel code blocks. |
54. What are the advantages of using Entity Framework over ADO.NET for database access in .NET?
Entity Framework provides a higher level of abstraction than ADO.NET, which makes it easier to work with databases. It also supports LINQ, which allows for more readable and maintainable code. Additionally, Entity Framework supports automatic code generation, which can save time and reduce errors.
55. What is the role of the Global.asax file in an ASP.NET application?
The Global.asax file is used to handle application-level events in an ASP.NET application, such as Application_Start, Session_Start, and Application_Error. It is also used to register application-level objects, such as HTTP modules and handlers.
56. What is the purpose of the “yield” keyword in C# and how is it used?
The “yield” keyword is used to create an iterator in C#. It allows a method to return a sequence of values one at a time, rather than returning a collection of values all at once. This can be useful for working with large data sets or for writing more efficient code.
57. What is the difference between an abstract class and an interface in C#?
Question | Abstract Class | Interface |
---|---|---|
What is it? | A class that cannot be instantiated and may contain abstract and/or concrete members. | A contract specifies a set of methods, properties, and events that a class must implement. |
Implementation | May implement any number of interfaces and may inherit from a single class. | Cannot implement classes or define concrete members. |
Members | May contain abstract or concrete members, including fields, properties, methods, and events. | Can only contain abstract members, including methods, properties, and events. |
Access Modifiers | Members can have public, protected, internal, or protected internal access modifiers. | Members are implicitly public and cannot have access modifiers. |
58. How are exceptions handled in .NET applications and what is the difference between checked and unchecked exceptions?
Exceptions in .NET applications are handled using try-catch blocks. When an exception is thrown, the runtime searches for a catch block that can handle the exception. Checked exceptions are exceptions that are checked at compile time, while unchecked exceptions are not.
59. What is the difference between a static class and a singleton pattern in C#?
A static class is a class that cannot be instantiated and can only contain static members, while a singleton is a class that is instantiated only once and provides a global point of access to that instance.
60. What is the difference between a constructor and a method in C#?
Question | Constructor | Method |
---|---|---|
What is it? | A special method called when an object is created is used to initialize object fields and properties. | A set of instructions grouped together to perform a specific task or return a value. |
Return Value | Does not return a value, but rather constructs a new object of the class type. | May return a value or void. |
Overloading | Can be overloaded to allow for multiple constructors with different parameters. | Can be overloaded to allow for multiple methods with different parameters or return types. |
Name | Shares the same name as the class it is defined in. | Can have any valid method name. |
61. How is the .NET Garbage Collector used to manage memory in .NET applications?
The .NET Garbage Collector automatically manages memory in .NET applications by periodically scanning the heap to identify objects that are no longer being used. It then frees up the memory used by these objects so that it can be used by other objects in the application.
62. What is the difference between a sealed class and a static class in C#?
Question | Sealed Class | Static Class |
---|---|---|
What is it? | A class that cannot be inherited, may contain abstract and/or concrete members. | A class that cannot be instantiated and may only contain static members. |
Inheritance | Cannot be inherited. | Cannot be inherited. |
Members | May contain abstract or concrete members, including fields, properties, methods, and events. | Can only contain static members, including fields, properties, methods, and events. |
Access Modifiers | Members can have public, protected, internal, or protected internal access modifiers. | Members can have public or internal access modifiers. |
63. What are security controls available on ASP.NET?
- <asp: Login> Provides a login capability that enables the users to enter their credentials with ID and password fields.
- <asp: LoginName> Used to display the user name who has logged in.
- <asp: LoginView> Provides a variety of views depending on the template that has been selected.
- <asp: LoginStatus> Used to check whether the user is authenticated or not.
- <asp: PasswordRecovery> Sends an email to a user while resetting the password.
64. What is the use of manifest in the .NET framework?
- Assembly version information.
- Scope checking of the assembly.
- Reference validation to classes.
- Security identification
65. What are the parameters that control the connection pooling behaviors?
- Connect Timeout
- Min Pool Size
- Max Pool Size
- Pooling
66. Whether ‘debug’ and ‘trace’ are the same?
No, they are not the same. Debug and Trace are two separate classes in .NET that are used for logging and tracing purposes respectively. Debug class is used for debugging purposes and is typically removed from the code in release mode. Trace class is used to log information and is typically kept in the code even in release mode.
67. What are Universal Windows Platform(UWP) Apps in .Net Core?
Universal Windows Platform (UWP) is a platform that allows developers to create apps that run on all Windows 10 devices, including desktop, mobile, Xbox, and IoT devices. UWP apps are built using .NET Core and can be deployed to the Microsoft Store.
68. What is CoreRT?
CoreRT is a .NET Core runtime optimized for native code execution. It is designed to produce small and fast native binaries for applications that are built using .NET Core.
70. What is .NET Core SDK?
.NET Core SDK is a set of tools used for developing .NET Core applications. It includes the .NET Core runtime, command-line tools, and libraries required for building and deploying .NET Core applications.
71. What is Docker?
Docker is a platform that allows developers to package, ship, and run applications as containers. Containers are lightweight, portable, and self-contained units that include everything needed to run an application, including code, runtime, system tools, and libraries.
72. What is a PE file?
PE (Portable Executable) file is a file format used by Microsoft Windows to store executable code and data for Windows programs. It contains information about the program, including the code, data, resources, and metadata required for the program to run.
73. What is the difference between DLL and EXE?
DLL (Dynamic Link Library) and EXE (Executable) are both file formats used by Windows to store program code and data. The main difference between the two is that an EXE file is a standalone program that can be executed directly, while a DLL file is a library of functions that can be called by other programs.
74. What is Serialization?
Serialization is the process of converting an object into a format that can be stored or transmitted. In .NET, serialization is used to convert objects into binary or XML format for storage or transmission over the network.
75. Describe the use of ErrorProvider Control in .NET?
ErrorProvider control is used to display validation errors for user input in Windows Forms applications. It displays an error icon next to the control that has failed validation, and displays a tooltip with the error message when the user hovers over the icon.
76. What is Multithreading?
Multithreading is the ability of an operating system or a program to manage multiple threads of execution concurrently. In .NET, multithreading is used to improve the performance of applications by allowing multiple threads to execute simultaneously.
77. What is an assembly in .NET?
An assembly in .NET is a unit of deployment that contains code, metadata, and resources required to run a .NET application. It can be in the form of a DLL or an EXE file.
78. What is the meaning of caching?
Caching is the process of storing frequently used data in memory or on disk to reduce the time and resources required to access it. In .NET, caching is often used to store frequently accessed data, such as database queries or web page content, to improve application performance.
79. What are the constructor types present in C# .NET?
The constructor types present in C# .NET are:
- Default constructor
- Parameterized constructor
- Static constructor
- Private constructor
80. What are memory-mapped files used for in .NET?
Memory-mapped files are used in .NET to efficiently share data between different processes or between a process and a file on disk. Memory-mapped files are mapped to a section of virtual memory that is shared between the processes, allowing them to read and write to the same memory location as if they were accessing their own private memory. This can be useful for scenarios such as interprocess communication, where large amounts of data need to be shared between processes.
81. What is MSIL code?
MSIL (Microsoft Intermediate Language) code is a low-level, platform-neutral language that is used by .NET applications. When a .NET application is compiled, it is compiled to MSIL code, which is then translated into machine code by the Just-In-Time (JIT) compiler when the application is run. This allows .NET applications to run on any platform that supports the .NET Framework or .NET Core, as long as a JIT compiler is available for that platform.
82. What is GAC?
GAC (Global Assembly Cache) is a central location in the .NET Framework where shared assemblies are stored. Assemblies in the GAC are globally accessible by all applications on the machine, regardless of their location or version. The GAC is used to store strongly named assemblies that need to be shared between multiple applications or components. Assemblies in the GAC must be signed with a strong name to ensure their uniqueness and integrity.
83. What is the difference between a stack and a heap in memory management?
In memory management, a stack is used for storing value types and reference types’ addresses, while a heap is used for storing reference type objects.
84. How does garbage collection work in .NET, and can you describe some best practices for optimizing memory management?
Garbage collection is an automatic process of freeing up memory in .NET. It works by periodically checking and identifying unused objects and releasing the associated memory. Some best practices for optimizing memory management include minimizing the use of global variables, disposing of unused resources, using value types instead of reference types, and limiting the use of large objects.
85. Can you walk me through how you would use LINQ to query a collection of data in C#?
To use LINQ to query a collection of data in C#, you would start by defining the data source, which can be an array, a list, a database table, or any other collection of data. Then, you would create a query expression using LINQ syntax, which typically includes keywords such as “from,” “where,” “select,” and “orderby.” Finally, you would execute the query using methods such as “ToList,” “FirstOrDefault,” or “Count.”
86. What is a unit of work in the context of the Entity Framework, and how does it relate to database transactions?
In the context of the Entity Framework, a unit of work is a pattern used to group together a set of related database operations into a single transaction. It allows for the entire set of operations to be treated as a single logical unit, ensuring that either all operations are completed successfully or none of them are. This helps to maintain consistency and integrity of the database.
87. How does ASP.NET handle state management in web applications?
ASP.NET provides several mechanisms for managing state in web applications, including ViewState, Session State, Application State, and Cache. ViewState is used to store state information about a specific web page, while Session State is used to store information across multiple pages for a single user. Application State and Cache are used to store information that is shared across all users of the application.
88. What is the purpose of the Web. config file in an ASP.NET application?
The Web.config file is used to store application settings, connection strings, and other configuration information for an ASP.NET application. It provides a centralized location for storing this information, allowing it to be easily modified and managed.
89. How does the .NET Framework handle threading, and can you explain some best practices for multithreaded programming?
The .NET Framework provides several classes and APIs for handling threading, including the Thread class, ThreadPool, and Task Parallel Library (TPL). Best practices for multithreaded programming in .NET include minimizing the use of locks and synchronization primitives, using immutable objects when possible, avoiding shared state between threads, and using the TPL for parallelizing CPU-bound work.
90. What are some of the differences between SOAP and REST web services, and which do you prefer to use in .NET applications?
SOAP is a protocol for exchanging structured data between applications, while REST is an architectural style for building web services. REST typically uses simpler, lightweight data formats such as JSON, while SOAP uses XML. REST is often preferred for its simplicity, scalability, and flexibility, while SOAP is preferred for its reliability and support for advanced features such as security and transactions. The choice between SOAP and REST depends on the specific requirements of the application.
91.What is MVC?
MVC stands for Model-View-Controller. It is an architectural model that is used to build applications in .NET. It serves as the foundation for the creation of applications.
Model: Used to store and pull back data from a database
View: Used to display the contents of an application
Controllers: Entities that handle user interaction
92. Can themes be applied to ASP.NET applications?
Yes, themes can be applied to ASP.NET applications easily by modifying the following code in the web.config file:
<configuration> <system.web> <pages theme="windows"/> </system.web> </configuration>
93. What is the use of MIME in .NET?
MIME is the short form of Multipurpose Internet Mail Extensions. It is an add-on to the existing email protocols that lets you exchange files over emails easily.
94. What is the order of the events that take place in a page life cycle?
Page_PreInit Page_Init Page_InitComplete Page_PreLoad Page_Load Page_LoadComplete Page_PreRender Render
95. What is the appSettings section in the web.config file?
<em> <configuration> <appSettings> <add key= "ConnectionString" value="server=local; pwd=password; database=default" /> </appSettings> </configuration> </em>
96. Explain CLS.
Common Language Specification (CLS) helps the application developers to use the components that are inter-language compatible with certain rules that come with CLS. It also helps in reusing the code among all of the .NET-compatible languages.
97. What is JIT?
JIT stands for Just In Time. It is a compiler that converts the intermediate code into the native language during the execution
98. Can a try block contain multiple catch blocks?
Yes, there can be multiple catch blocks for one try block.
99. What is a partial class?
A class can be spread across multiple source files using the keyword partial. All source files for the class definition are compiled as one file with all class members.
100. What is the purpose of webHostBuilder()?
WebHostBuilder function is used for HTTP pipeline creation through webHostBuilder.Use() chaining all at once with WebHostBuilder.Build() by using the builder pattern. This function is provided by Microsoft.AspNet.Hosting namespace. The Build() method’s purpose is building necessary services and a Microsoft.AspNetCore.Hosting.IWebHost for hosting a web application
Freshersnow.com is an excellent resource for job seekers and employers alike who want to evaluate technical knowledge and problem-solving skills. The Top 100 .NET Interview Questions and Answers are a comprehensive collection that can help you enhance your understanding of the subject.