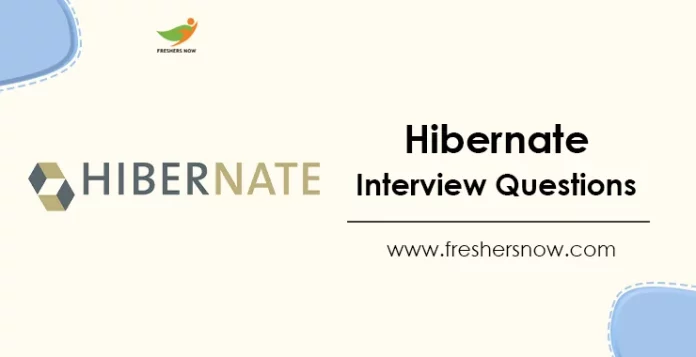
Hibernate Interview Questions and Answers: Hibernate is an open-source object-relational mapping (ORM) tool used to develop data persistence applications for Java. It simplifies the process of mapping an object-oriented domain model to a relational database by automating most of the tedious and error-prone code. With its increasing popularity, it is important to be well-prepared for the Latest Hibernate Interview Questions.
★★ Latest Technical Interview Questions ★★
Hibernate Technical Interview Questions
This article will provide the latest and top 100 Hibernate interview questions and answers, including Hibernate interview questions for freshers, to help you excel in your next Hibernate interview.
Top 100 Hibernate Interview Questions and Answers
1. What is Hibernate and what are its benefits?
- Hibernate is an open-source object-relational mapping (ORM) tool for Java.
- Its benefits include:
- Simplifies database programming
- Reduces development time
- Provides object-oriented query language
- Promotes modular programming
- Supports caching mechanisms
2. What is the difference between Hibernate and JDBC?
- JDBC is a Java API used to interact with relational databases directly.
- Hibernate is a Java-based ORM framework that provides an abstraction layer between the application code and the database.
3. What are the various ways to configure Hibernate?
- Hibernate can be configured in three ways:
- Using hibernate.cfg.xml file
- Using hibernate.properties file
- Programmatically using the Configuration class.
4. What is the difference between session.get() and session.load() methods in Hibernate?
Feature | session.get() |
session.load() |
---|---|---|
What it does | Retrieves an object from the database with the specified ID. | Retrieves a proxy object (a lightweight reference to an entity) for an object with the specified ID. |
Returns | The actual object from the database. | A proxy object that extends the specified class and lazily fetches data when needed. |
Database hit | Executes an immediate database query. | Does not hit the database until an attribute is accessed on the proxy object. |
Returns null | If the object with the specified ID does not exist in the database. | If the object with the specified ID does not exist in the database, an ObjectNotFoundException is thrown when an attribute is accessed on the proxy object. |
Eager loading associations | By default, it eagerly loads all associated objects. | By default, it lazily loads associated objects when they are accessed on the proxy object. |
Use case | Use it when you need to immediately fetch an object from the database. | Use it when you want to load an object’s data lazily and avoid unnecessary database hits. |
5. What is the syntax for creating a Hibernate SessionFactory object?
The syntax for creating a Hibernate SessionFactory object is:
Configuration configuration = new Configuration(); configuration.configure(); SessionFactory sessionFactory = configuration.buildSessionFactory(); |
6. How does Hibernate mapping work?
- Hibernate mapping maps Java objects to database tables and vice versa.
- It uses annotations or XML files to define the mapping.
7. What are the different types of mapping in Hibernate?
- The different types of mapping in Hibernate are:
- One-to-One
- One-to-Many
- Many-to-One
- Many-to-Many
- Component Mapping
- Inheritance Mapping.
8. What is ORM in Hibernate?
The acronym ORM in Hibernate stands for Object Relational Mapping. It is a pattern for converting relational database data into object-oriented programming constructs. In addition to facilitating data retrieval, creation, and manipulation, this tool greatly simplifies the mapping process.
9. What is the syntax for creating a Hibernate Session object?
The syntax for creating a Hibernate Session object is:
Session session = sessionFactory.openSession(); |
10. How does Hibernate differ from other ORM frameworks such as JPA or MyBatis?
Feature | Hibernate | JPA | MyBatis |
---|---|---|---|
Type of ORM | Full-featured | Standardized | Semi-automatic |
Architecture | Three-tier | Three-tier | Three-tier |
Database support | All | All | All |
Query language | HQL | JPQL | SQL |
Caching | Yes | Yes | No |
Ease of use | Complex | Easy | Easy |
Performance | Slow | Fast | Fast |
Code generation | Yes | Yes | No |
Mapping | XML, Annotation | Annotation | XML, Annotation |
Transactions | Yes | Yes | Yes |
11. What is a Session in Hibernate?
- A Session is a lightweight object that represents a single connection between Java application and database.
- It is used to perform CRUD (Create, Read, Update, Delete) operations on database tables.
12. How do you create a SessionFactory in Hibernate?
- SessionFactory is created only once per application.
- It is created using Configuration object as follows:
- Configuration cfg = new Configuration();
- SessionFactory factory = cfg.buildSessionFactory();
13. How do you create a Hibernate configuration file?
To create a Hibernate configuration file, follow these steps:
- Create a new XML file with the name “hibernate.cfg.xml”
- Add the required configuration properties in the file
- Save the file in the project classpath.
14. What is the use of the hbm2ddl.auto property in Hibernate?
- The hbm2ddl.auto property is used to automatically generate database tables based on the entity classes defined in the application.
- It can also be used to automatically update the schema if there are any changes made to the entity classes.
15. How does Hibernate differ from JDBC in terms of its approach to managing database connections?
Feature | JDBC | Hibernate |
---|---|---|
Connection management | JDBC requires manual management of database connections using DriverManager or DataSource. | Hibernate provides automatic connection management using its built-in connection pooling mechanism. |
Mapping between Java objects and database tables | JDBC requires manual mapping of Java objects to database tables using SQL statements. | Hibernate provides automatic object-relational mapping (ORM) between Java objects and database tables using annotations or XML mappings. |
Querying database | JDBC requires manual creation and execution of SQL queries. | Hibernate provides a powerful query language called Hibernate Query Language (HQL) that allows developers to query the database using object-oriented syntax. |
Transaction management | JDBC requires manual management of transactions using Connection.commit() and Connection.rollback() methods. | Hibernate provides automatic transaction management using its built-in transaction manager. Developers can use Hibernate APIs to begin, commit, and rollback transactions. |
Caching | JDBC doesn’t provide caching. | Hibernate provides first-level and second-level caching mechanisms to improve performance by reducing the number of database calls. |
Portability | JDBC code is specific to the database vendor and requires changes if you switch databases. | Hibernate code is database-agnostic, and you can switch databases by changing the configuration file. |
16. What is lazy loading in Hibernate?
- Lazy loading is a technique used in Hibernate to load data only when it is actually needed by the application.
- When an application requests an entity or collection, Hibernate loads only the minimum amount of data necessary, and additional data is fetched on demand.
- Lazy loading is useful in reducing the amount of data that is transferred between the application and the database, and can improve performance.
17. What is the difference between the save() and persist() methods in Hibernate?
Features | save() |
persist() |
---|---|---|
Return type | Serializable |
void |
Transaction | Requires a transaction to be open | Throws an exception if no transaction is open |
Identifier value | Generated before calling save() |
Generated after calling persist() |
Object state | Changes to the object after save() |
Changes to the object after persist() are not guaranteed to be persisted |
Detached objects | Can save both transient and detached objects | Can only save transient objects |
18. What is the difference between lazy loading and eager loading in Hibernate?
- In eager loading, all the associated entities or collections are loaded at the time when the parent entity is loaded, even if they are not needed by the application.
- In lazy loading, data is loaded on demand, i.e., only when it is actually needed by the application.
- Eager loading is generally used when the associated entities or collections are small and their data is frequently used, while lazy loading is used when the data is large or not always needed.
19. What is the syntax for creating a Hibernate Transaction object?
The syntax for creating a Hibernate Transaction object is:
Transaction transaction = session.beginTransaction(); |
20. Explain the concept behind Hibernate Inheritance Mapping
Java is an Object-Oriented Programming Language that utilizes Inheritance as a fundamental principle. Inheritance is commonly used to simplify relationships between models in Java. However, relational databases have a flat structure and do not support inheritance, posing a challenge.
Hibernate, as an ORM, addresses this issue through its Inheritance Mapping strategies. In the example of dividing InterviewBitEmployee into Contract and Permanent Employees represented by IBContractEmployee and IBPermanentEmployee classes, Hibernate’s task is to represent these two employee types while adhering to certain restrictions. Specifically, general employee details are defined in the parent InterviewBitEmployee class, while Contract and Permanent employee-specific details are stored in IBContractEmployee and IBPermanentEmployee classes, respectively. The class diagram for this system is presented below.
There are different inheritance mapping strategies available:
- Single Table Strategy
- Table Per Class Strategy
- Mapped Super Class Strategy
- Joined Table Strategy
21. What is the use of Session.clear() method in Hibernate?
- The Session.clear() method in Hibernate is used to clear the session level cache of the first level cache.
- It removes all the persistent objects from the first-level cache.
22. What is the difference between a Hibernate session and a Hibernate transaction?
Hibernate Session | Hibernate Transaction |
---|---|
Represents a single-threaded unit of work | Represents a unit of work that is transactional |
Manages a cache of persistent objects | Manages the state of the database |
Acts as a factory for creating and loading | Ensures atomicity, consistency, isolation, and |
persistent objects | durability (ACID) properties of the database |
Can be used to perform CRUD operations | Must be used to perform CRUD operations |
Can be long-lived or short-lived | Is always short-lived |
Can be used to read or write to the database | Must be used to write to the database |
Can be used to load, update or delete objects | Must be used to group operations into a single |
transaction | |
Can be used to open, close or reconnect to | Must be used to manage the state of the database |
the database | during a transaction |
23. What is the use of Session.evict() method in Hibernate?
- The Session.evict() method in Hibernate is used to remove a particular object from the first-level cache.
- It detaches the object from the persistence context and doesn’t synchronize the changes with the database.
24. What is the difference between get() and load() methods in Hibernate?
- The get() method in Hibernate returns the object immediately if it exists in the database, or returns null if it doesn’t exist.
- The load() method in Hibernate returns a proxy object immediately and loads the actual object only when it’s needed. It throws an exception if the object doesn’t exist.
25. Explain Hibernate architecture
- The Hibernate architecture comprises various objects, including a persistent object, session factory, session, query, transaction, and more.
- Applications developed with Hibernate fall into four main categories:
- Java Application
- Hibernate framework – Configuration and Mapping Files
- Internal API –
- JDBC (Java Database Connectivity)
- JTA (Java Transaction API)
- JNDI (Java Naming Directory Interface)
- Database – MySQL, PostGreSQL, Oracle, etc.
The main components of the Hibernate framework include:
- SessionFactory: This offers a factory method to acquire session objects and clients of ConnectionProvider, and it maintains an optional second-level cache of data.
- Session: This is a short-lived object that serves as an interface between Java application objects and database data. The session can be used to generate transaction, query, and criteria objects, and it has a required first-level cache of data.
- Transaction: This object specifies the atomic unit of work and contains useful methods for transaction management. It is optional.
- ConnectionProvider: This factory generates JDBC connection objects and provides an abstraction to the application from the DriverManager. It is optional.
- TransactionFactory: This factory generates Transaction objects. It is optional.
26. What is the use of @Table annotation in Hibernate?
- The @Table annotation in Hibernate is used to specify the name of the database table that is mapped to an entity class.
- It can also specify other details such as the schema, catalog, and indexes.
27. How does Hibernate differ from traditional SQL database operations?
Aspect | Hibernate | Traditional SQL |
---|---|---|
Transactions | Hibernate provides a built-in transaction management mechanism, which automatically handles transaction boundaries, committing and rolling back transactions as needed. | Traditional SQL requires manual transaction management using SQL commands such as BEGIN, COMMIT, and ROLLBACK. |
Object-Relational Mapping | Hibernate maps Java objects to relational database tables and vice versa, providing an abstraction layer that makes it easier to work with data in an object-oriented way. | Traditional SQL requires manual mapping between Java objects and relational database tables, which can be time-consuming and error-prone. |
Query Language | Hibernate provides its own query language called Hibernate Query Language (HQL), which is similar to SQL but uses object-oriented concepts like inheritance and polymorphism. | Traditional SQL uses a standard SQL query language, which does not support object-oriented concepts like inheritance and polymorphism. |
Performance | Hibernate can provide performance benefits by optimizing SQL queries and caching data in memory. | Traditional SQL can be faster in some cases because it allows more direct control over database operations. |
Portability | Hibernate can work with multiple databases, as it provides a layer of abstraction between the application and the database. | Traditional SQL is specific to the database being used, making it less portable between different databases. |
28. What is the syntax for saving an object using Hibernate?
The syntax for saving an object using Hibernate is:
session.save(object); |
29. In Hibernate, what is the difference between eager loading and lazy loading?
Property | Eager Loading | Lazy Loading |
---|---|---|
Definition | Loads all associated objects when the parent is loaded. | Loads associated objects only when they are explicitly requested. |
Performance | Can lead to performance issues due to loading too much data at once. | Improves performance as only necessary data is loaded. |
Execution Time | Longer execution time due to loading all associated objects upfront. | Shorter execution time as only necessary data is loaded on demand. |
Use Cases | Small datasets or when all associated objects are needed. | Large datasets or when only certain associated objects are needed. |
Memory Usage | Eager loading uses more memory as all associated objects are loaded upfront. | Lazy loading uses less memory as only necessary data is loaded. |
Configuration | Eager loading is configured using the fetch attribute in the mapping. | Lazy loading is configured using the lazy attribute in the mapping. |
Data Retrieval | Retrieves all associated data when the parent object is retrieved. | Retrieves associated data only when it is explicitly requested using getters or method calls. |
30. What is the use of @Column annotation in Hibernate?
- The @Column annotation in Hibernate is used to map a class property to a database column.
- It specifies the column name, data type, length, nullable, and other attributes.
31. What is the use of @GeneratedValue annotation in Hibernate?
- The @GeneratedValue annotation in Hibernate is used to specify the generation strategy for the primary key of an entity class.
- It generates a unique primary key value automatically based on the specified strategy, such as identity, sequence, or table.
32. What is hibernate caching?
Hibernate caching is a performance enhancement strategy that involves pooling objects in the cache to accelerate query execution. This approach is particularly valuable when retrieving identical data multiple times. Instead of accessing the database repeatedly, we can retrieve the data from the cache, resulting in a faster application throughput time.
Types of Hibernate Caching:
First Level Cache:
- This level is activated by default.
- The first level cache is stored in the Hibernate session object.
- Because it is bound to the session object, the scope of the data stored in the first level cache is not available to the entire application. An application may utilize multiple session objects.
Second Level Cache:
- The second level cache is located in the SessionFactory object, allowing the entire application to access the cached data.
- This cache is not enabled by default and must be enabled explicitly.
- EH (Easy Hibernate) Cache, Swarm Cache, OS Cache, and JBoss Cache are several examples of cache providers.
33. What is the use of @OneToMany annotation in Hibernate?
- The @OneToMany annotation in Hibernate is used to map a one-to-many relationship between two entities.
- It specifies the target entity class, the foreign key column name, and other details.
34. What is the use of @OneToOne annotation in Hibernate?
- The @OneToOne annotation in Hibernate is used to map a one-to-one relationship between two entities.
- It specifies the target entity class, the foreign key column name, and other details.
35. What is the use of @JoinColumn annotation in Hibernate?
- @JoinColumn annotation is used to specify the column used for joining an entity association or collection.
- It is used to declare the foreign key column when two tables are joined in a database.
- It is used to specify the name of the foreign key column and the table that contains it.
- It is used to control the mapping of the foreign key column by providing options like nullable, unique, and insertable.
36. What is the syntax for updating an object using Hibernate?
The syntax for updating an object using Hibernate is:
session.update(object); |
37. What is the difference between a Hibernate Criteria and a Hibernate Query?
Criteria | Query |
---|---|
Object-oriented API | SQL-like API |
Provides type-safety and compile-time error checking | No type-safety and error checking at compile-time |
Facilitates the creation of dynamic queries | Suitable for static queries |
Generates SQL query at runtime | Predefined SQL query |
Supports projection, grouping, and aggregate functions | Supports simple queries that return entities or collections of entities |
Provides support for association traversal | Does not provide direct support for association traversal |
Facilitates pagination and sorting of results | Can handle pagination and sorting, but not as easily as Criteria |
Can be more complex to use and understand | Simpler and more straightforward to use and understand |
38. What is the use of @JoinTable annotation in Hibernate?
- @JoinTable annotation is used to specify a join table for a many-to-many association.
- It is used to define the join table’s name and the names of its columns that reference the associated tables.
- It is used to specify the foreign key columns of the join table and their references to the associated tables.
- It is used to control the mapping of the join table by providing options like indexes, constraints, and cascade behavior.
39. What is the use of @Embedded annotation in Hibernate?
- @Embedded annotation is used to embed an object into another entity.
- It is used to indicate that a persistent field or property of an entity contains an instance of another embeddable class.
- It is used to map the embedded object’s properties to the columns of the parent entity’s table.
- It is used to control the persistence of the embedded object’s state, such as whether it should be saved or updated when the parent entity is saved or updated.
40. What is the use of @EmbeddedId annotation in Hibernate?
- @EmbeddedId annotation is used to map a composite primary key to an entity.
- It is used to indicate that a persistent field or property of an entity represents the composite primary key.
- It is used to embed the primary key object’s properties into the parent entity’s table.
- It is used to control the mapping of the primary key by providing options like access type, column names, and column types.
41. What are Many to Many associations?
A many-to-many association denotes multiple connections between two entity instances, such as multiple students participating in multiple courses and vice versa. To represent this relationship, we create a separate table to hold foreign keys for both the student and course entities, since each refers to the other through foreign keys.
In this scenario, the table that links students and courses is known as the Join Table, and it consists of a composite primary key comprising the student_id and course_id.
42. What is the use of @Version annotation in Hibernate?
- @Version annotation is used to enable optimistic locking of an entity.
- It is used to indicate that a persistent field or property of an entity represents the version number of the entity’s state.
- It is used to ensure that multiple transactions do not overwrite each other’s changes to the same entity by comparing the version numbers.
- It is used to control the concurrency of the entity’s state, such as by specifying the type of version number or the strategy for generating it.
43. What is the syntax for deleting an object using Hibernate?
The syntax for deleting an object using Hibernate is:
session.delete(object); |
44. How does Hibernate differ from other object-relational mapping (ORM) frameworks?
Framework | Features | Advantages | Disadvantages |
---|---|---|---|
Hibernate | – Full-featured ORM <br>- Provides caching mechanisms <br>- Supports JPA <br>- Flexible object model mapping | – Supports a wide range of database vendors <br>- Offers efficient performance through lazy loading <br>- Provides good documentation and community support | – Steep learning curve <br>- May be overkill for small projects <br>- Can result in complex queries |
Entity Framework | – Provides easy-to-use API <br>- Enables LINQ to Entities <br>- Supports automatic migrations <br>- Offers model-first and code-first approaches | – Excellent integration with Visual Studio <br>- Offers good performance and scalability <br>- Supports multiple databases | – Limited support for database vendors <br>- Requires deep understanding of underlying technologies <br>- Can be difficult to debug |
Django ORM | – Provides easy-to-use API <br>- Supports object-relational mapping <br>- Enables model migration <br>- Offers query caching | – Offers excellent integration with Django <br>- Supports multiple databases <br>- Easy to set up and use | – Limited support for non-Django projects <br>- Can result in complex queries <br>- May not be suitable for complex data models |
MyBatis | – Provides flexible XML-based configuration <br>- Supports dynamic SQL generation <br>- Supports custom SQL <br>- Offers caching | – Offers good performance and scalability <br>- Provides good documentation and community support <br>- Easy to learn and use | – Limited support for complex data models <br>- Requires manual mapping of objects to SQL statements <br>- Can result in complex queries |
45. What is the use of @NamedQuery annotation in Hibernate?
- @NamedQuery annotation is used to define a named query in an entity.
- It is used to specify a JPQL query string and its parameters as a named query that can be executed later by its name.
- It is used to improve query performance by precompiling and caching the named query.
- It is used to simplify complex queries by allowing them to be reused and parameterized in multiple places.
46. What is the use of @NamedNativeQuery annotation in Hibernate?
- @NamedNativeQuery annotation is used to define a named native SQL query in an entity.
- It is used to specify a native SQL query string and its parameters as a named query that can be executed later by its name.
- It is used to execute complex queries that cannot be expressed in JPQL, such as vendor-specific functions or native SQL queries.
- It is used to improve query performance by precompiling and caching the named native query.
- It is used to simplify complex queries by allowing them to be reused and parameterized in multiple places.
47. What is the use of @Formula annotation in Hibernate?
- @Formula annotation is used to define a calculated column in an entity.
- It is used to specify a SQL expression that calculates the value of a column based on other columns in the same table.
- It is used to map the calculated column to a transient field or property of the entity.
- It is used to improve query performance by calculating the value of the column on the database side instead of the application side.
48. What is the syntax for retrieving an object by its ID using Hibernate?
The syntax for retrieving an object by its ID using Hibernate is:
Object object = session.get(Object.class, id); |
49. What is the use of @AccessType annotation in Hibernate?
- @AccessType annotation is used to specify the access type for a persistent field or property of an entity.
- It is used to control how the field or property is accessed by the persistence provider, either by field or by property.
- It is used to improve performance by reducing the overhead of invoking getters and setters.
- It is used to enforce encapsulation by hiding the internal state of the entity from the persistence provider.
50. What is the use of @Cache annotation in Hibernate?
- The @Cache annotation is used to specify the caching behavior of an entity or collection in Hibernate.
- It can be used to configure the first-level cache, second-level cache, or both.
- The @Cache annotation is used to improve application performance by reducing the number of database queries.
51. What is the difference between first-level cache and second-level cache in Hibernate?
- The first-level cache is session-level cache and is enabled by default in Hibernate.
- It stores the data within the session scope and is cleared when the session is closed or cleared.
- The second-level cache is an application-level cache that stores data across multiple sessions.
- It is shared by all sessions that use the same cache provider and stores data in a distributed or local cache.
52. What is the syntax for retrieving all objects of a particular class using Hibernate?
The syntax for retrieving all objects of a particular class using Hibernate is:
List<Object> objects = session.createQuery(“from Object”).list(); |
53. What are the different types of second-level caches supported by Hibernate?
- Hibernate supports several types of second-level caches, including:
- EHCache: an open-source, high-performance, distributed cache.
- Infinispan: a scalable, distributed, and highly available data grid platform.
- Hazelcast: an open-source, distributed data processing and caching platform.
- Memcached: a high-performance, distributed memory object caching system.
54. What is the use of Query in Hibernate?
- Query in Hibernate is used to retrieve data from the database based on certain conditions.
- It is an object-oriented representation of a SQL query and can be used to execute both simple and complex queries.
- Query allows developers to specify parameters dynamically and supports pagination, sorting, and aggregation.
55. What is the use of Criteria in Hibernate?
- Criteria in Hibernate is used to build and execute object-oriented queries.
- It provides a type-safe and object-oriented way to build queries at runtime.
- Criteria can be used to specify conditions using a wide range of operators and supports pagination, sorting, and aggregation.
56. What is the difference between a Hibernate cache and a database cache?
Features | Hibernate Cache | Database Cache |
---|---|---|
Purpose | Improves performance by caching frequently accessed data | Improves performance by caching frequently accessed data |
Scope | Applies to a session or application level | Applies to a database level |
Types of Cache | First Level Cache (Session Cache), Second Level Cache | Buffer Cache, Result Cache, Shared Pool, Dictionary Cache |
Cache Level | Object-level caching | Query-level caching |
Data Stored | Stores objects or collections of objects in memory | Stores query results and frequently used data in memory or disk |
Management | Managed by Hibernate framework | Managed by the database system |
Configuration | Configured in Hibernate configuration files (e.g., XML, YAML) | Configured in database configuration files (e.g., SQL) |
57. What is the use of CriteriaBuilder in Hibernate?
- CriteriaBuilder is used to create criteria queries dynamically in Hibernate.
- It provides a type-safe and object-oriented way to build queries at runtime, similar to Criteria, but with more flexibility.
- CriteriaBuilder can be used to specify conditions using a wide range of operators and supports pagination, sorting, and aggregation.
58. What is the use of Projections in Hibernate?
- Projections in Hibernate is used to query a subset of data from an entity or collection.
- It allows developers to retrieve only the necessary data by specifying the fields to be retrieved.
- Projections can be used to perform aggregate operations, such as counting, summing, or averaging.
59. What is the use of Restrictions in Hibernate?
- Restrictions in Hibernate is used to add conditions to a query.
- It allows developers to filter the data based on certain conditions, such as equality, inequality, like, and between.
- Restrictions can be combined with logical operators, such as and, or, and not.
60. What is the use of DetachedCriteria in Hibernate?
- DetachedCriteria in Hibernate is used to create criteria queries that are not associated with a session.
- It allows developers to create a query once and execute it multiple times in different sessions.
- DetachedCriteria can be used to specify conditions using a wide range of operators and supports pagination, sorting, and aggregation.
61. What is the use of HibernateTemplate in Hibernate?
- HibernateTemplate is a utility class in Hibernate that simplifies the use of Hibernate API.
- It provides a set of convenience methods for common CRUD operations, such as save, update, delete, and get.
- HibernateTemplate eliminates the boilerplate code and improves the readability and maintainability of the code.
62. What is the syntax for executing a HQL query using Hibernate?
The syntax for executing a HQL query using Hibernate is:
Query query = session.createQuery(“from Object where condition”); List<Object> objects = query.list(); |
63. What is the use of HibernateCallback in Hibernate?
- HibernateCallback is an interface in Hibernate that provides a callback mechanism to execute Hibernate code.
- It can be used to execute Hibernate operations within the context of a Hibernate session.
64. What is the use of HibernateInterceptor in Hibernate?
- HibernateInterceptor is an interface in Hibernate that provides an interception mechanism to intercept Hibernate operations.
- It can be used to intercept and modify the behavior of Hibernate operations, such as adding custom logic before or after an operation.
65. What is the use of SessionFactoryUtils in Hibernate?
- SessionFactoryUtils is a utility class in Hibernate that provides various utility methods for working with a Hibernate session factory.
- It provides methods for opening a session, getting the current session, and closing a session.
66. What is the use of SessionFactoryBean in Hibernate?
- SessionFactoryBean is a Spring framework class that provides a convenient way to configure and initialize a Hibernate session factory.
- It simplifies the configuration of a Hibernate session factory and provides various configuration options.
67. What is the use of Transaction in Hibernate?
- Transaction is an interface in Hibernate that provides a way to perform database operations in a transactional manner.
- It ensures that a group of database operations are executed atomically and that they are either all committed or all rolled back in case of an error.
68. What is the use of TransactionTemplate in Hibernate?
- TransactionTemplate is a Spring framework class that provides a way to perform database operations in a transactional manner.
- It simplifies the configuration of transactions and provides various options, such as transaction propagation behavior and rollback rules.
69. What is the syntax for creating a Criteria object in Hibernate?
The syntax for creating a Criteria object in Hibernate is:
Criteria criteria = session.createCriteria(Object.class); |
70. What is the use of SessionFactory.getCurrentSession() method in Hibernate?
- SessionFactory.getCurrentSession() method in Hibernate is used to get the current Hibernate session.
- It provides a thread-safe way to obtain the current Hibernate session and ensures that it is bound to the current thread.
71. What is the use of @Cacheable annotation in Hibernate?
- @Cacheable annotation in Hibernate is used to enable caching for an entity or a collection.
- It specifies that the results of a query for the annotated entity or collection should be cached and reused for subsequent requests.
72. What is the use of @CachePut annotation in Hibernate?
- @CachePut annotation in Hibernate is used to update the cache for an entity or a collection.
- It specifies that the annotated entity or collection should be added or updated in the cache, ensuring that subsequent requests use the updated value.
73. What is the use of @CacheEvict annotation in Hibernate?
- @CacheEvict annotation in Hibernate is used to evict an entity or a collection from the cache.
- It specifies that the annotated entity or collection should be removed from the cache, ensuring that subsequent requests retrieve the latest data from the database.
74. What is the use of @NaturalId annotation in Hibernate?
- @NaturalId is an annotation in Hibernate that marks a property as a natural identifier.
- It is used to optimize query performance as it avoids full table scans by using the natural identifier as a unique key.
75. What is the use of @Immutable annotation in Hibernate?
- @Immutable is an annotation in Hibernate that marks an entity as immutable.
- It is used to optimize query performance as it avoids database updates, caching updates and optimizes second-level cache performance.
76. What is the use of @DynamicInsert annotation in Hibernate?
- @DynamicInsert is an annotation in Hibernate that generates the INSERT SQL statement dynamically at runtime.
- It is used to improve performance by reducing the size of the generated SQL statement as it only includes the non-null columns.
77. What is the use of @DynamicUpdate annotation in Hibernate?
- @DynamicUpdate is an annotation in Hibernate that generates the UPDATE SQL statement dynamically at runtime.
- It is used to improve performance by reducing the size of the generated SQL statement as it only includes the modified columns.
78. What is the use of @BatchSize annotation in Hibernate?
- @BatchSize is an annotation in Hibernate that groups multiple SQL statements into a single SQL statement.
- It is used to improve performance by reducing the number of SQL statements sent to the database.
79. What is the use of @LazyCollection annotation in Hibernate?
- @LazyCollection is an annotation in Hibernate that allows collections to be lazily initialized.
- It is used to improve performance by reducing the amount of data loaded from the database and also improves memory usage.
80. What is the use of @Type annotation in Hibernate?
- @Type is an annotation in Hibernate that specifies the type of data for a property.
- It is used to map custom data types to a database column.
81. What is the use of @TypeDef annotation in Hibernate?
- @TypeDef is an annotation in Hibernate that defines a custom data type for a property.
- It is used to map a custom data type to a database column.
82. What is the use of @Lob annotation in Hibernate?
- @Lob is an annotation in Hibernate that marks a property as a Large Object.
- It is used to store large data objects such as images or documents in the database.
83. What is the use of @Temporal annotation in Hibernate?
- @Temporal is an annotation in Hibernate that specifies the type of temporal data for a property such as DATE, TIME, or TIMESTAMP.
- It is used to map a temporal data type to a database column.
84. What is the use of @SequenceGenerator annotation in Hibernate?
- @SequenceGenerator annotation is used to specify the name of the database sequence object used to generate primary key values for an entity.
- It is used to customize the sequence object’s properties, such as its name, initial value, and allocation size.
- It is used in conjunction with @GeneratedValue annotation to specify the primary key generation strategy for an entity.
85. What is the use of @TableGenerator annotation in Hibernate?
- @TableGenerator annotation is used to specify the name of the database table used to generate primary key values for an entity.
- It is used to customize the table’s properties, such as its name, initial value, and allocation size.
- It is used in conjunction with @GeneratedValue annotation to specify the primary key generation strategy for an entity.
86. What is the use of @GeneratedValue(strategy=GenerationType.AUTO) annotation in Hibernate?
- @GeneratedValue(strategy=GenerationType.AUTO) annotation is used to let Hibernate choose the primary key generation strategy based on the underlying database and dialect.
- It is the default primary key generation strategy in Hibernate.
87. What is the use of @GeneratedValue(strategy=GenerationType.IDENTITY) annotation in Hibernate?
- @GeneratedValue(strategy=GenerationType.IDENTITY) annotation is used to specify that the primary key values are generated by the database’s identity column feature.
- It is used for databases that support auto-incrementing primary key columns, such as MySQL and PostgreSQL.
88. What is the use of @GeneratedValue(strategy=GenerationType.SEQUENCE) annotation in Hibernate?
- @GeneratedValue(strategy=GenerationType.SEQUENCE) annotation is used to specify that the primary key values are generated by a database sequence object.
- It is used for databases that support sequence objects, such as Oracle and PostgreSQL.
89. What is the use of @GeneratedValue(strategy=GenerationType.TABLE) annotation in Hibernate?
- @GeneratedValue(strategy=GenerationType.TABLE) annotation is used to specify that the primary key values are generated by a database table.
- It is a portable primary key generation strategy that works with any database, but it may be slower than other strategies.
90. What is the use of @AttributeOverrides and @AttributeOverride annotations in Hibernate?
- @AttributeOverrides and @AttributeOverride annotations are used to customize the mapping of embedded objects in an entity.
- @AttributeOverrides annotation is used to specify a list of @AttributeOverride annotations that override the default mapping of the embedded object’s attributes.
- @AttributeOverride annotation is used to specify the new mapping for a single attribute of the embedded object.
91. What is the use of @EmbeddedId and @IdClass annotations in Hibernate?
- @EmbeddedId and @IdClass annotations are used to specify composite primary keys in an entity.
- @EmbeddedId annotation is used to specify that the primary key consists of an embedded object, which is a value type that contains multiple attributes.
- @IdClass annotation is used to specify that the primary key consists of multiple simple or composite attributes, which are mapped as fields or properties of the entity.
92. What is the use of @DynamicInsert and @DynamicUpdate annotations in Hibernate?
- @DynamicInsert and @DynamicUpdate annotations are used to optimize the performance of insert and update operations on an entity.
- @DynamicInsert annotation is used to generate SQL statements that include only non-null columns when inserting a new row into the table, which can save time and disk space.
- @DynamicUpdate annotation is used to generate SQL statements that include only modified columns when updating an existing row in the table, which can reduce network traffic and improve concurrency.
93. What is the use of the SessionFactory.openSession() method in Hibernate?
- SessionFactory.openSession() method is used to create a new Hibernate Session object, which represents a single unit of work with the database.
- It is used to perform database operations, such as inserting, updating, and deleting entities, and querying data.
- The Session object is created and managed by the SessionFactory, which is typically a singleton object in a Hibernate application.
- The openSession() method returns a new Session object that is not bound to any transaction, so it is the developer’s responsibility to manage transactions using the Session.beginTransaction() and Session.getTransaction().commit() methods.
94. What is the difference between hibernate.cfg.xml and hibernate.properties?
- hibernate.cfg.xml is an XML file that contains configuration properties for Hibernate.
- hibernate.properties is a properties file that contains configuration properties for Hibernate.
95. What is a Criteria API in Hibernate?
- The Criteria API is an alternative way to write queries in Hibernate, which allows for more dynamic and type-safe queries.
- Instead of writing HQL queries as strings, the Criteria API allows developers to build queries programmatically using Java code.
- The Criteria API provides a set of classes and methods for creating complex queries that can be easily modified at runtime.
96. What is the use of @Entity annotation in Hibernate?
- The @Entity annotation in Hibernate is used to mark a class as an entity.
- It maps the entity to a database table and specifies the table name, primary key, and other details.
97. What is the use of @ManyToOne annotation in Hibernate?
- The @ManyToOne annotation in Hibernate is used to map a many-to-one relationship between two entities.
- It specifies the target entity class, foreign key column name, and other details.
98. What is the use of @Transient annotation in Hibernate?
- @Transient annotation is used to mark a field or property as not persistent.
- It is used to exclude a field or property from the entity’s persistence state, such as from being saved or updated in the database.
- It is used to prevent a field or property from being mapped to a database column.
- It is used to indicate that a field or property is only used for transient calculations or runtime operations and not for persistent storage.
99. Can we declare the Entity class final?
It’s not recommended to declare the entity class as final because Hibernate employs proxy classes and objects for the lazy loading of data, querying the database only when necessary, which is accomplished by extending the entity bean. If the entity class, or bean, is marked final, it can’t be extended, thus making it impossible to support lazy loading.
100. How do you create an immutable class in hibernate?
To create an immutable class in Hibernate, we can set mutable=false in the XML configuration. The default value is true, indicating that the class is mutable. Alternatively, we can use the @Immutable annotation when using annotations.
Being familiar with the top 100 Hibernate interview questions and answers can help you succeed in technical interviews and advance your career in Java development. To enhance your knowledge, do follow us at freshersnow.com without any hesitation.