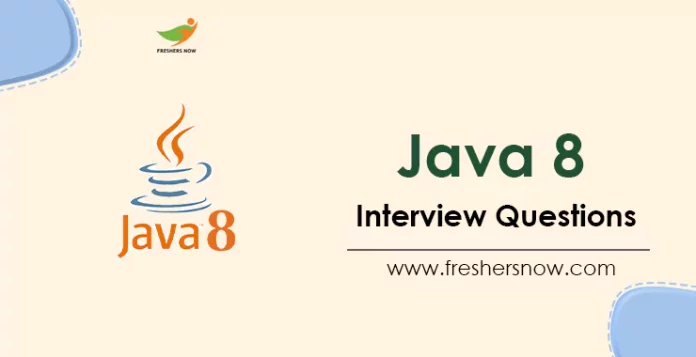
In this article, we will provide a Top 100 Java 8 Interview Questions and Answers suitable for both freshers and experienced individuals. We will also discuss the scope of the Latest Java 8 Interview Questions and career opportunities associated with Java 8 Technical Interview Questions.
★★ Latest Technical Interview Questions ★★
Java 8 Technical Interview Questions
To help you succeed in your Java 8 interview, we have put together a comprehensive list of the Top 100 Java 8 Interview Questions and Answers. Our list includes Java 8 Technical Interview Questions as well as Java 8 Interview Questions for Freshers. By going through these questions, you can ensure that you are well-prepared to ace your Java 8 interview.
Top 100 Java 8 Interview Questions and Answers
1. What is Java 8?
Java 8 is a major version of the Java programming language that was released in 2014. It introduced significant new features and enhancements, including lambda expressions, functional interfaces, and the stream API.
2. In Java SE 8, what do you mean by Streams?
- Java SE 8 introduced a new package known as java.util.stream, which contains various interfaces, classes, and enums for performing functional-style operations on elements. Java streams are not data structures but an abstraction that allows for functional-style processing of streams of elements.
- Unlike collections or sets, Java streams do not store data or elements. Instead, streams represent a collection of operations that can be applied to any data set. The main difference between a data structure and a stream is that a stream is a representation of a fixed set of operations to be performed on a data set, rather than a container for storing data or elements.
3. What is the syntax for creating a spliterator in Java 8?
To create a spliterator in Java 8, you can extend the “Spliterators.AbstractSpliterator” class and implement the “tryAdvance()” and “estimateSize()” methods. The syntax is:
class MySpliterator extends Spliterators.AbstractSpliterator<String> { public MySpliterator() { super(Long.MAX_VALUE, Spliterator.ORDERED); } public boolean tryAdvance(Consumer<? super String> action) { // implementation } public long estimateSize() { // implementation } }
4. What are some of the new features in Java 8?
Some of the new features in Java 8 include lambda expressions, functional interfaces, method references, default methods, and the stream API.
5. What are functional interfaces in Java 8?
Functional interfaces are interfaces that contain only one abstract method. They are used as the basis for lambda expressions and method references in Java 8.
6. What are the two types of common operations of Java Streams?
Intermediate operations in Java streams refer to the operations that transform a stream into another stream by applying various operations such as filter, map, distinct, sorted, etc. The result of an intermediate operation is another stream, which allows the chaining of multiple intermediate operations together.
On the other hand, terminal operations in Java streams refer to the operations that produce a result or side-effect as a non-stream value. These operations are typically used to consume the elements of a stream and perform some final action, such as forEach, reduce, collect, toArray, etc. Once a terminal operation is applied, the stream is consumed and cannot be reused again.
7. What is a lambda expression in Java 8?
A lambda expression is a concise way of representing an anonymous function. It is a key feature of Java 8 that allows you to write functional-style code.
8. How do you define a lambda expression in Java 8?
A lambda expression in Java 8 is defined using the following syntax: (parameters) -> expression or statement block.
9. What is the syntax for a lambda expression in Java 8?
The syntax for a lambda expression in Java 8 is as follows:
(parameters) -> expression or (parameters) -> { statement block }
10. What are the main components of a Stream?
- A data source
- Set of Intermediate Operations to process the data source
- Single Terminal Operation that produces the result
11. How does reduce() differ from collect() in Java 8 streams?
Feature | reduce() | collect() |
---|---|---|
Return value | Returns a single value that results from a reduction operation | Returns a collection or data structure that contains the reduced values |
Mutability | May use mutable reduction operations | Typically uses immutable reduction operations |
Ordering | May not preserve the order of the elements | May preserve the order of the elements |
12. What is a method reference in Java 8?
A method reference is a shorthand syntax for creating a lambda expression that calls a method.
13. What are Java 8 streams?
- A Stream in Java is a pipeline of operations that allows you to express data processing queries in a declarative way. It represents a sequence of data objects and operations that can be performed on that data. Unlike Java I/O Streams, a Java Stream does not hold any data permanently.
- The key interface for working with Streams is java.util.stream.Stream<T>, which is a generic interface that can accept functional interfaces, allowing you to pass lambdas as arguments. Streams also support a fluent interface or chaining, which allows you to chain multiple operations together.
- To better understand the sequence of operations in a Stream, you can visualize it using a timeline or marble diagram. The basic Stream timeline diagram shows how data is passed through the pipeline of intermediate and terminal operations, which ultimately produce a result.
14. How do you define a method reference in Java 8?
A method reference in Java 8 is defined using the following syntax: Class::method or object::method.
15. What are the default methods in Java 8?
Default methods are methods that have a default implementation in an interface. They allow you to add new methods to an interface without breaking existing implementations.
16. How do default methods help in backward compatibility?
Default methods help in backward compatibility by allowing you to add new methods to an interface without breaking existing code that implements the interface.
17. What are static methods in Java 8?
Static methods in Java 8 are methods that are defined on an interface and can be called without creating an instance of the interface.
18. How do you use the “toMap()” method in Java 8 streams?
The “toMap()” method in Java 8 streams is used to collect the elements of a stream into a map. You need to provide a key mapper and a value mapper. The syntax is
"stream.collect(Collectors.toMap(keyMapper, valueMapper))"
For example:
List<String> list = Arrays.asList("apple", "banana", "cherry"); Map<String, Integer> map = list.stream().collect(Collectors.toMap (Function.identity(), String::length));
19. What is a stream in Java 8?
A stream in Java 8 is a sequence of elements that can be processed in parallel or sequentially. It provides a powerful way to work with collections and other data sources.
20. How do you create a stream in Java 8?
To create a stream in Java 8, you can call the “stream()” method on a collection or create a stream from an array using the “Arrays.stream()” method.
21. What is the filter() method in Java 8 streams?
The filter() method in Java 8 streams is used to return a new stream consisting of elements that match a given predicate. It takes a Predicate as a parameter, which is a functional interface that returns a boolean.
22. What is the map() method in Java 8 streams?
The map() method in Java 8 streams is used to transform a stream by applying a given function to each element in the stream. It takes a Function as a parameter, which is a functional interface that returns a new value based on the input value.
23. What is the difference between limit() and skip() in Java 8 streams?
Feature | limit() | skip() |
---|---|---|
Return value | Returns a stream with a limited number of elements | Returns a stream with skipped elements |
Functionality | Truncates the stream to a specified maximum size | Skips a specified number of elements from the beginning of the stream |
Performance | Performance is dependent on the limit value | Performance is dependent on the skip value |
Use case | Limiting the number of elements processed in a stream | Skipping a certain number of elements at the beginning of the stream |
24. What is the flatMap() method in Java 8 streams?
The flatMap() method in Java 8 streams is used to transform a stream of collections into a stream of individual elements. It takes a Function as a parameter, which is a functional interface that returns a stream of elements.
25. What is the forEach() method in Java 8 streams?
The forEach() method in Java 8 streams is used to iterate over each element in the stream and perform a given action. It takes a Consumer as a parameter, which is a functional interface that performs an action on each element.
26. What is the reduce() method in Java 8 streams?
The reduce() method in Java 8 streams is used to combine all elements in a stream into a single value. It takes a BinaryOperator as a parameter, which is a functional interface that combines two values and returns a new value.
27. What is the collect() method in Java 8 streams?
The collect() method in Java 8 streams is used to accumulate the elements of a stream into a collection or other data structure. It takes a Collector as a parameter, which is a functional interface that defines how to collect the elements of the stream.
28. What is the groupingBy() method in Java 8 streams?
The groupingBy() method in Java 8 streams is used to group the elements of a stream based on a given classifier function. It takes a Function as a parameter, which is a functional interface that returns the grouping key.
29. What is the syntax for using the “IntPredicate” functional interface in Java 8?
The “IntPredicate” functional interface in Java 8 is used to test an integer value. To create an instance of “IntPredicate”, you can use a lambda expression. The syntax is:
IntPredicate isPositive = (int i) -> i > 0; boolean result = isPositive.test(5);
30. What is the partitioningBy() method in Java 8 streams?
The partitioningBy() method in Java 8 streams is used to partition the elements of a stream into two groups based on a given predicate. It returns a Map containing two Boolean keys, “true” and “false”, with the elements that match the predicate and those that do not.
31. What is the joining() method in Java 8 streams?
The joining() method in Java 8 streams is used to concatenate the elements of a stream into a single string. It takes an optional delimiter and optional prefix/suffix strings as parameters.
32. What is the toArray() method in Java 8 streams?
The toArray() method in Java 8 streams is used to convert a stream into an array. It takes an IntFunction as a parameter, which is a functional interface that returns a new array.
33. What are method references used for in Java 8 streams?
Method references in Java 8 streams are used to refer to a method by name instead of defining a lambda expression. They can be used with functional interfaces that match the method signature, making the code more concise and readable. There are four types of method references: static method references, instance method references, constructor references, and array references.
34. What is the difference between a stream and a collection in Java 8?
Stream | Collection |
---|---|
Represents a sequence of elements that can be processed in parallel or sequentially. | Represents a group of individual objects that can be manipulated as a single unit. |
Can be created from any data source, including collections, arrays, or I/O channels. | Represents a single data structure that holds a collection of elements. |
Enables functional-style operations, such as filter, map, and reduce, that can be chained together to form a pipeline. | Uses imperative-style operations, such as loops and iterators, to access and manipulate elements. |
Does not store elements, but instead creates a view of the elements from the source data. | Stores elements directly in memory or on disk. |
Supports both sequential and parallel processing, allowing for efficient processing of large or infinite data sources. | Supports only sequential processing, which can limit performance for large or complex data sources. |
35. How do you use the “orElseGet()” method in Java 8?
The “orElseGet()” method in Java 8 is used to return a default value if the optional is empty. The difference between “orElse()” and “orElseGet()” is that “orElseGet()” takes a supplier function to generate the default value. The syntax is “optional.orElseGet(() -> defaultValue)”. For example:
Optional<String> optional = Optional.empty(); String result = optional.orElseGet(() -> "default");
36. What is the difference between sequential and parallel streams in Java 8?
Sequential Streams | Parallel Streams |
---|---|
Processes stream elements in a single thread, one after another. | Breaks the stream into multiple parts and processes each part in a separate thread, allowing for concurrent processing. |
Suitable for small or simple streams, or for cases where the order of the elements is important. | Suitable for large or complex streams, or for cases where the order of the elements is not important. |
Can be converted to a parallel stream using the parallel() method. | Can be converted to a sequential stream using the sequential() method. |
Can cause performance issues if the stream operations are not optimized, especially for large or complex data sources. | Can improve performance for large or complex data sources, but may require additional memory or processing resources. |
37. What is the Optional class in Java 8?
The Optional class is a container object used to represent the presence or absence of a value. It was introduced in Java 8 to help prevent NullPointerExceptions by explicitly indicating when a value may be null.
38. How do you use the Optional class in Java 8?
To use the Optional class, you can create an Optional object using the of() or empty() methods. You can then call methods such as isPresent(), get(), or orElse() to check if a value is present, retrieve the value if it is, or provide a default value if it is not.
39. What is the syntax for using the “OptionalDouble” class in Java 8?
The “OptionalDouble” class in Java 8 is used to represent an optional double value. To create an instance of “OptionalDouble”, you can call the “OptionalDouble.of()” method.
For example:
OptionalDouble optional = OptionalDouble.of(3.14);
40. What is the Date and Time API in Java 8?
The Date and Time API in Java 8 is a new API for working with dates, times, and time zones. It includes classes such as LocalDate, LocalTime, LocalDateTime, ZonedDateTime, Instant, Duration, Period, DateTimeFormatter, ChronoUnit, and ChronoField.
41. What are the advantages of the Date and Time API in Java 8?
The Date and Time API in Java 8 provides a more consistent and intuitive way of working with dates and times compared to the old Date and Calendar classes. It also includes support for time zones and daylight saving time and provides improved thread safety and immutability.
42. What is the difference between the Date and Time API and the old Date and Calendar classes?
The Date and Time API in Java 8 provides a more modern and comprehensive set of classes for working with dates and times, while the old Date and Calendar classes are based on an older, mutable design. The new API also includes improved support for time zones and daylight saving time.
43. How does parallelStream() differ from stream() in Java 8?
Feature | stream() | parallelStream() |
---|---|---|
Processing mode | Sequential | Parallel |
Execution order | Ordered | Unordered |
Thread safety | Safe for single-threaded environments | Safe for multi-threaded environments |
Use case | Small or medium data processing, or for ordered operations | Large data processing, or for unordered operations where ordering is not important |
44. What is the Instant class in Java 8 Date and Time API?
The Instant class represents an instant in time, measured in seconds and nanoseconds since the Unix epoch (January 1, 1970, 00:00:00 UTC). It is similar to the old Date class but with improved precision and thread safety.
45. What is the Duration class in Java 8 Date and Time API?
The Duration class represents a duration of time between two instants, measured in seconds and nanoseconds. It can be used to perform arithmetic operations on instants and to represent time intervals.
46. What is the Period class in Java 8 Date and Time API?
The Period class represents a period of time between two dates, measured in years, months, and days. It can be used to perform arithmetic operations on dates and to represent time intervals in calendar units.
47. How do you use the “findFirst()” method in Java 8 streams?
The “findFirst()” method in Java 8 streams is used to return the first element in the stream. The syntax is “stream.findFirst()”
For example:
List<Integer> numbers = Arrays.asList(1, 2, 3); Optional<Integer> result = numbers.stream().findFirst();
48. What is the LocalDateTime class in Java 8 Date and Time API?
The LocalDateTime class represents a date and time without a time zone. It can be used to represent a specific date and time in a specific time zone.
49. What is the difference between peek() and map() in Java 8 streams?
Feature | peek() | map() |
---|---|---|
Return value | Returns the same stream as input | Returns a new stream with mapped values |
Functionality | Executes a specified operation on each element of the stream, without modifying the element | Transforms each element of the stream using a specified function |
Side effects | Allows for side effects on each element of the stream | Should not allow for side effects on the original elements |
Use case | Debugging or logging operations | Transforming or mapping each element of the stream |
50. What is the ZonedDateTime class in Java 8 Date and Time API?
The ZonedDateTime class represents a date and time with a time zone. It can be used to represent a specific date and time in a specific time zone and to perform arithmetic operations across different time zones.
51. What is the DateTimeFormatter class in Java 8 Date and Time API?
The DateTimeFormatter class is used to format and parse dates and times in a specific format, using a pattern string that specifies the desired format.
52. What is the ChronoUnit enum in Java 8 Date and Time API?
The ChronoUnit enum represents a unit of time, such as days, hours, minutes, or seconds. It can be used to perform arithmetic operations on instants, durations, and periods.
53. What is the ChronoField enum in Java 8 Date and Time API?
The ChronoField enum represents a field of a date or time, such as year, month,
day, hour, minute, or second. It can be used to get or set the value of a specific field in a date or time object. The ChronoField enum is used in conjunction with the TemporalAccessor and TemporalField interfaces to provide a flexible and extensible way of working with date and time fields.
54. What is the difference between findFirst() and findAny() in Java 8 streams?
Feature | findFirst() | findAny() |
---|---|---|
Return value | Returns the first element of the stream, if present | Returns any element of the stream, if present |
Parallel behavior | Returns the first element of the encounter order, if the stream is ordered | Returns the first element found, without any ordering guarantees |
Use case | Used in ordered streams, where the first element has a specific meaning | Used in unordered streams, where any element can satisfy the search criteria |
55. What is the Comparator interface in Java 8?
The Comparator interface in Java 8 is a functional interface used to define a comparison function for objects of a specific type. It is commonly used to sort collections of objects based on a specific order.
56. What is the syntax for creating an immutable list in Java 8?
To create an immutable list in Java 8, you can use the “Collections.unmodifiableList()” method. The syntax is:
List<String> myList = new ArrayList<>(); List<String> immutableList = Collections.unmodifiableList(myList);
57. How do you use the Comparator interface in Java 8?
To use the Comparator interface, you can implement the compare() method to define a custom comparison function for a specific type of object. You can then use the Comparator object to sort collections of objects based on the defined comparison function.
58. How does filter() differ from distinct() in Java 8 streams?
Feature | filter() | distinct() |
---|---|---|
Return value | Returns a stream with filtered elements | Returns a stream with distinct elements |
Functionality | Filters elements based on a specified predicate | Removes duplicates from the stream |
Performance | Performance is dependent on the predicate complexity | Performance is dependent on the number of unique elements |
Use case | Filtering elements based on a certain condition | Removing duplicates from the stream |
59. What is the Spliterator interface in Java 8?
The Spliterator interface in Java 8 is used to split a collection of elements into multiple chunks that can be processed in parallel. It is designed to work with the Stream API and is used to provide a way to iterate over a collection in parallel.
60. What is the ForkJoinPool in Java 8?
The ForkJoinPool in Java 8 is a thread pool implementation designed to support the parallel processing of large data sets. It is used by the Stream API to automatically parallelize operations on collections of data.
61. What is the CompletableFuture class in Java 8?
The CompletableFuture class in Java 8 is used to represent a future result of an asynchronous computation. It is designed to support the chaining of asynchronous operations and can be used to perform non-blocking I/O operations.
62. What is the difference between CompletableFuture and Future in Java 8?
The CompletableFuture class in Java 8 is an extension of the Future interface, with additional support for chaining asynchronous operations and other advanced features. CompletableFuture provides methods for registering callbacks to be executed when the future result is available and can be used to compose complex asynchronous workflows.
63. How do you create a CompletableFuture in Java 8?
You can create a CompletableFuture in Java 8 by using the static methods of the CompletableFuture class, such as supplyAsync(), which takes a supplier function and returns a CompletableFuture representing the result of that function.
64. How do you declare a functional interface in Java 8?
To declare a functional interface in Java 8, you can use the “@FunctionalInterface” annotation before the interface declaration. The interface should have only one abstract method. For example:
@FunctionalInterface interface MyFunction { void doSomething(); }
65. What is the thenApply() method in CompletableFuture in Java 8?
The thenApply() method in CompletableFuture in Java 8 is used to chain a function that operates on the result of a CompletableFuture and returns a new CompletableFuture representing the result of that function.
66. What is the thenCompose() method in CompletableFuture in Java 8?
The thenCompose() method in CompletableFuture in Java 8 is used to chain a function that returns a new CompletableFuture and flattens the result into a single CompletableFuture.
67. What is the thenCombine() method in CompletableFuture in Java 8?
The thenCombine() method in CompletableFuture in Java 8 is used to combine the result of two CompletableFuture objects using a specified function and return a new CompletableFuture representing the combined result.
68. How does Java 8 handle null values in the Optional class?
Java 8 handles null values in the Optional class by explicitly indicating when a value may be null. The of() method of the Optional class will throw a NullPointerException if a null value is passed, while the empty() method returns an Optional object with no value.
69. What is the purpose of the Stream API in Java 8?
The Stream API in Java 8 is used to process collections of data in a functional style using lambda expressions. It provides a way to perform operations such as filtering, mapping, and reducing on collections of data in a concise and expressive way.
70. What is a functional interface in Java 8?
A functional interface in Java 8 is an interface that contains only one abstract method and can be used as the basis for a lambda expression or method reference. Functional interfaces are used extensively in Java 8 for the Stream API, CompletableFuture, and other functional programming features.
71. How do you create a lambda expression in Java 8?
Lambda expressions are created using the following syntax:
(parameters) -> expression
72. What is a Predicate in Java 8?
In Java 8, a Predicate is a functional interface that represents a function that takes an argument and returns a boolean value. It can be used to test whether a certain condition is met. The Predicate interface has a single abstract method named test(), which takes an argument and returns a boolean value.
73. How does Java 8 handle multiple inheritances?
Java 8 allows default methods in interfaces, which helps to handle multiple inheritances. A default method is a method that has a body and is declared with the default keyword. When a class implements multiple interfaces that have default methods with the same signature, the compiler resolves the ambiguity by choosing the method that is most specific to the implementing class.
74. What is a static method reference in Java 8?
A static method reference is a shorthand notation for a lambda expression that calls a static method. It is created using the following syntax:
ClassName::staticMethodName
For example, to create a static method reference that refers to the parseInt() method of the Integer class, you can write:
Function<String, Integer> parseInt = Integer::parseInt;
75. What is the difference between forEach() and map() methods in Java 8?
The forEach() method is a terminal operation that applies an action to each element of a stream, whereas the map() method is an intermediate operation that applies a function to each element of a stream and returns a new stream consisting of the results. The forEach() method does not return a value, whereas the map() method returns a new stream.
76. How does Java 8 handle parallelism in streams?
Java 8 provides the parallel() method to enable parallel processing of streams. The parallel() method returns a parallel stream, which is a stream that is capable of processing elements concurrently on multiple threads. Parallel streams can be used to improve the performance of operations that can be parallelized, such as filtering, mapping, and reducing.
77. What is the purpose of the CompletableFuture class in Java 8?
The CompletableFuture class in Java 8 is used to represent a future result that can be completed asynchronously. It provides a way to perform asynchronous computations and compose them in a non-blocking way. CompletableFuture supports the chaining of multiple asynchronous computations, handling of exceptions, and combining the results of multiple computations.
78. What is the difference between a Consumer and a Supplier in Java 8?
In Java 8, a Consumer is a functional interface that represents a function that takes an argument and returns no result. It is used to perform side effects, such as printing a message or updating a variable. A Supplier, on the other hand, is a functional interface that represents a function that takes no arguments and returns a result. It is used to supply values, such as generating a random number or reading a value from a database.
79. What is the purpose of the Collectors class in Java 8?
The Collectors class in Java 8 provides a set of predefined collectors that can be used to accumulate elements of a stream into various data structures, such as lists, sets, and maps. The Collectors class also provides methods for grouping and partitioning elements of a stream based on certain criteria.
80. What is the difference between forEach() and map() in Java 8 streams?
forEach() | map() | |
---|---|---|
Operation | Performs a given action on each element of the stream. | Transforms each element of the stream using the given function. |
Return Type | void | Stream<T> |
Example | stream.forEach(System.out::println); | Stream<Integer> newStream = stream.map(x -> x * 2); |
Usage | Use when you want to perform an action on each element of the stream, such as printing or updating values. | Use when you want to transform each element of the stream into a new value, such as converting from one data type to another or performing some calculation. |
Side Effects | Can be used to perform side effects on each element of the stream, such as updating a variable or printing to the console. | Should not have any side effects, as the purpose is to create a new stream with transformed values. |
Lazy Evaluation | Not lazy – performs the action on each element immediately. | Lazy – does not actually perform the transformation until the resulting stream is used. |
81. What is the purpose of the flatMap() method in Java 8?
The flatMap() method is used to convert a Stream of elements to a Stream of other elements, by applying a function that returns a Stream for each element.
82. How does Java 8 handle type inference in lambda expressions?
Java 8 introduced a new type inference algorithm called “target typing,” which infers the type of a lambda expression based on the context in which it is used.
83. How does method reference differ from lambda expression in Java 8?
Lambda Expression | Method Reference |
---|---|
Provides a concise way to represent an anonymous function. | Provides a way to reference an existing method or constructor. |
Can be used to represent any functional interface. | Can only be used to represent functional interfaces that match the method signature. |
Uses the arrow operator -> to separate the parameters from the body. |
Uses the double colon operator :: to reference a method or constructor. |
Creates a new instance of a functional interface. | Uses an existing method or constructor. |
Can be more verbose for simple operations. | Can be more concise for simple operations. |
84. What is the difference between Optional and null in Java 8?
Optional is a container object that may or may not contain a non-null value, while null represents the absence of any value.
85. What is the purpose of the tryAdvance() method in Java 8 streams?
The tryAdvance() method is used to sequentially iterate over the elements of a stream, returning true if there are more elements to process and false otherwise.
86. What is a method reference in Java 8?
A method reference is a shorthand syntax for referring to a method without actually invoking it and can be used as an argument to a lambda expression or as the target of a method call.
87. How does Java 8 handle date and time operations?
Java 8 introduced a new Date and Time API that provides a more flexible and intuitive way of handling date and time operations, including support for time zones and daylight saving time.
88. What is the purpose of the Supplier interface in Java 8?
The Supplier interface is a functional interface that represents a supplier of results and can be used to generate or provide values on demand.
89. How does Java 8 handle checked exceptions in lambda expressions?
Java 8 introduced a new feature called “exception transparency,” which allows lambda expressions to throw checked exceptions without requiring the caller to handle them.
90. What is the purpose of the reduce() method in Java 8 streams?
The reduce() method is used to perform a reduction operation on the elements of a stream, by applying a binary operator to successive elements and accumulating the result.
91. What is a BiConsumer in Java 8?
A BiConsumer is a functional interface that represents an operation that takes two input arguments and returns no result and can be used as a target for method references or lambda expressions.
92. How does Java 8 handle default methods in multiple interfaces?
Java 8 allows interfaces to define default methods, which can be inherited by implementing classes, and provides a set of rules to resolve conflicts when multiple interfaces define the same default method.
93. What is the difference between a Consumer and a Supplier in Java 8?
Consumer | Supplier |
---|---|
Accepts a single input and performs an operation on it. | Returns a result without any input. |
Represents an operation that takes an input and returns no result. | Represents an operation that takes no input and returns a result. |
Has a single method named accept(T t) . |
Has a single method named get() . |
Can be used with the forEach() method in streams. |
Can be used with the generate() method in streams. |
Cannot be composed with other functional interfaces. | Can be composed with other functional interfaces. |
94. What is the purpose of the peek() method in Java 8 streams?
The peek() method is used to apply an action to each element of a stream, while still allowing the stream to continue flowing and be processed by subsequent operations.
95. What are the significant advantages of Java 8?
- Compact, readable, and reusable code.
- Less boilerplate code.
- Parallel operations and execution.
- Can be ported across operating systems.
- High stability.
- Stable environment.
- Adequate support
96. Explain, for example, LocalDate, LocalTime, and LocalDateTime APIs.
LocalDate
Date with no time component
Default format - yyyy-MM-dd (2020-02-20) LocalDate today = LocalDate.now(); // gives today's date LocalDate aDate = LocalDate.of(2011, 12, 30); //(year, month, date) LocalTime
Time with no date with nanosecond precision
Default format - hh:mm:ss:zzz (12:06:03.015) nanosecond is optional LocalTime now = LocalTime.now(); // gives time now LocalTime aTime2 = LocalTime.of(18, 20, 30); // (hours, min, sec) LocalDateTime
Holds both Date and Time
Default format - yyyy-MM-dd-HH-mm-ss.zzz (2020-02-20T12:06:03.015) LocalDateTime timestamp = LocalDateTime.now(); // gives timestamp now //(year, month, date, hours, min, sec) LocalDateTime dt1 = LocalDateTime.of(2011, 12, 30, 18, 20, 30);
97. What are Intermediate and Terminal operations?
Intermediate Operations:
- Process the stream elements.
- Typically transforms a stream into another stream.
- Are lazy, i.e., not executed till a terminal operation is invoked.
- Does internal iteration of all source elements.
- Any number of operations can be chained in the processing pipeline.
- Operations are applied as per the defined order.
- Intermediate operations are mostly lambda functions. Terminal Operations:
- Kick-starts the Stream pipeline.
- used to collect the processed Stream data.
98. What does the String::ValueOf expression mean?
It is a static method reference to the method Valueof() of class String. It will return the string representation of the argument passed.
99. In which programming paradigm Java 8 falls?
- Object-oriented programming language.
- Functional programming language.
- Procedural programming language.
- Logic programming language
100. Define Nashorn in Java 8
Nashorn is a JavaScript processing engine that is bundled with Java 8. It provides better compliance with ECMA (European Computer Manufacturers Association) normalized JavaScript specifications and better performance at run-time than older versions.
Acquiring expertise in the Top 100 Java 8 Interview Questions and Answers can provide job seekers with a competitive advantage in obtaining employment and advancing their careers in the Java 8 field. Join us at freshersnow.com to broaden your understanding.