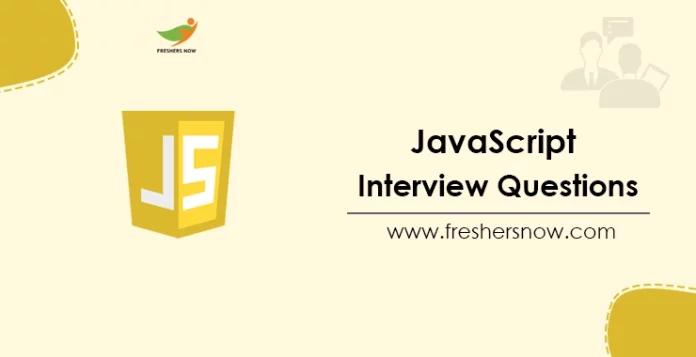
JavaScript Interview Questions and Answers: As JavaScript continues to dominate the world of web development, being well-versed in the language has become a must-have skill for developers. This is why employers often conduct technical interviews to assess the JavaScript proficiency of candidates, especially freshers. In this context, having a clear understanding of the latest JavaScript interview questions can be the key to landing your dream job. That’s why we have compiled a list of the top 100 JavaScript interview questions and answers, which cover a wide range of topics and scenarios.
★★ Latest Technical Interview Questions ★★
JavaScript Technical Interview Questions
So, whether you are a fresher looking to break into the field or an experienced developer, this comprehensive list of JavaScript Interview Questions for Freshers will help you prepare for any technical interview with confidence.
Top 100 JavaScript Interview Questions and Answers
1. What is JavaScript and what are its advantages?
- JavaScript is a high-level, interpreted programming language used to create interactive web pages and dynamic user interfaces.
- Some of its advantages are:
- Less server interaction − Validating user input before sending the page to the server reduces server traffic, resulting in a lower server load.
- Immediate feedback to the visitors − Visitors receive immediate feedback without waiting for a page reload
- Increased interactivity − Create interactive interfaces that react to user actions
- Richer interfaces − Use JavaScript to add drag-and-drop components and sliders for richer interfaces
2. What are the different data types in JavaScript?
- There are six primitive data types in JavaScript:
- number
- string
- boolean
- null
- undefined
- symbol
- There is also one non-primitive data type:
- object
3. What are object prototypes?
- All JavaScript objects inherit properties from a prototype, including Date, Math, and Array objects.
- The Date object inherits properties from the Date prototype, Math objects inherit properties from the Math prototype, and Array objects inherit properties from the Array prototype.
- At the top of the prototype chain is Object.prototype, which all prototypes inherit properties and methods from.
- The prototype serves as a blueprint for an object, enabling the use of properties and methods even if they don’t exist on the current object.
- The code above shows that the array “arr” has not defined a property or method called push, yet no error is thrown by the JavaScript engine.
- The reason is due to the use of prototypes. Array objects inherit properties from the Array prototype, as discussed previously.
- When the JavaScript engine doesn’t find the push method on the current array object, it searches for it within the Array prototype and finds it there.
- If a property or method isn’t found on the current object, the JavaScript engine will search through its prototype and continue to search through the prototype’s prototype until it finds the property or method or until there are no more prototypes to search.
4. How do you write a for loop in JavaScript?
The syntax for a for loop in JavaScript is:
for (initialization; condition; update) { statement } |
5. What is scope in JavaScript?
- Scope in JavaScript refers to the accessibility of variables.
- There are two types of scope: global scope and local scope.
- Global variables can be accessed from anywhere in the program, while local variables are only accessible within their own function or block.
6. Explain the difference between null and undefined in JavaScript.
null | undefined |
---|---|
Represents an intentional absence of any object value. | Represents a variable that has been declared but has not been assigned a value. |
Must be assigned explicitly. | Automatically assigned by JavaScript when a variable is declared but not assigned a value. |
typeof null returns “object”. | typeof undefined returns “undefined”. |
Example: let x = null; |
Example: let y; |
Example: console.log(x); // outputs null |
Example: console.log(y); // outputs undefined |
7. What is the use of the ‘use strict’ statement in JavaScript?
- The ‘use strict’ statement is used to enable strict mode in JavaScript.
- Strict mode enforces stricter parsing and error handling in the code, which can help prevent some common coding mistakes and improve performance.
8. What is the difference between let and var in JavaScript?
let | var |
---|---|
Introduced in ES6 (ECMAScript 2015). | Introduced in the early versions of JavaScript. |
Has a block scope. | Has a function scope or global scope. |
Variables declared with let are not hoisted. | Variables declared with var are hoisted. |
Cannot be re-declared in the same scope. | Can be re-declared in the same scope. |
Example: let x = 5; |
Example: var y = 10; |
Example: { let x = 5; } console.log(x); // ReferenceError: x is not defined |
Example: { var y = 10; } console.log(y); // Outputs 10 |
9. How do you define an array in JavaScript?
To define an array in JavaScript, use square brackets [] and separate the elements with commas: var myArray = [1, 2, 3];
10. What are the different types of operators in JavaScript?
There are several types of operators in JavaScript, including:
- Arithmetic operators (+, -, *, /, %)
- Assignment operators (=, +=, -=, *=, /=, %=)
- Comparison operators (==, ===, !=, !==, <, >, <=, >=)
- Logical operators (&&, ||, !)
- Bitwise operators (&, |, ^, ~, <<, >>, >>>)
11. Explain the difference between synchronous and asynchronous JavaScript.
- Synchronous JavaScript refers to code that is executed in a single thread, with each statement executed one after the other in the order that they appear in the code.
- Asynchronous JavaScript, on the other hand, refers to code that can be executed out of order, with some code running in the background while other code continues to execute.
12. What is the difference between client-side and server-side JavaScript?
- Client-side JavaScript consists of two main parts: a core language and predefined objects for executing JavaScript in a browser environment.
- JavaScript for the client is automatically incorporated into HTML pages and is interpreted by the browser at runtime.
- Server-side JavaScript is similar to client-side JavaScript, but it includes JavaScript code that runs on a server and is deployed only after processing.
13. What are the different ways to create an object in JavaScript?
- There are several ways to create objects in JavaScript, including:
- Object literals: defining an object with key-value pairs using curly braces ({})
- Constructor functions: creating an object using a function that acts as a constructor
- Object.create(): creating an object using an existing object as a prototype
- Class syntax (ES6): defining an object using class syntax with the constructor and methods.
14. What is the syntax for a switch statement in JavaScript?
The syntax for a switch statement in JavaScript is:
switch (expression) { case value1: statement1; break; case value2: statement2; break; default: defaultStatement; } |
15. What is prototypal inheritance in JavaScript?
- Prototypal inheritance is a mechanism in JavaScript that allows objects to inherit properties and methods from other objects.
- Every object in JavaScript has a prototype object that it can inherit from, and this inheritance can be used to create a hierarchy of objects.
- When a property or method is accessed on an object, JavaScript will first look for it on the object itself, and if it’s not found, it will look for it on the prototype object.
16. What are the features of JavaScript?
- JavaScript is a lightweight, interpreted programming language.
- It is intended for building network-centric applications.
- JavaScript is complementary to and integrated with Java.
- It is an open and cross-platform scripting language.
17. What is the event loop in JavaScript?
- The event loop is a mechanism in JavaScript that manages the order in which code is executed.
- JavaScript is a single-threaded language, which means that only one piece of code can be executed at a time.
- The event loop allows JavaScript to handle asynchronous operations by queuing up tasks and executing them in order when the main thread is free.
18. How do you declare a JavaScript object?
To declare an object in JavaScript, use curly braces {} and define properties and values separated by colons: var myObject = {property1: value1, property2: value2};
19. What is the difference between function declaration and arrow function in JavaScript?
Function Declaration | Arrow Function |
---|---|
Defined using the function keyword, followed by the function name, parameters, and the function body in curly braces. |
Defined using the arrow => symbol, followed by the parameters and the function body. |
Example: function square(x) { return x * x; } |
Example: const square = x => x * x; |
Can be used as constructors with the new keyword. |
Cannot be used as constructors. |
Has its own this keyword that refers to the current object. |
Inherit the this keyword from the enclosing scope. |
Example: function Person(name, age) { this.name = name; this.age = age; } |
Example: const Person = (name, age) => { this.name = name; this.age = age; }; |
Can be defined after they are called in the code. | Must be defined before they are called in the code. |
Example: console.log(square(5)); // Outputs 25 <br> function square(x) { return x * x; } |
Example: const square = x => x * x; <br> console.log(square(5)); // Outputs 25 |
20. What are promises in JavaScript?
- Promises are a way to handle asynchronous operations in JavaScript.
- A promise represents the eventual completion or failure of an asynchronous operation and can be in one of three states: pending, fulfilled, or rejected.
- Promises allow you to attach callbacks that will be executed when the promise is fulfilled or rejected.
21. Explain the concept of currying in JavaScript.
- Currying is a technique in functional programming where a function that takes multiple arguments is transformed into a series of functions that each take a single argument.
- Currying allows you to create more specialized functions by partially applying arguments.
- In JavaScript, currying can be achieved using closures and the Function.prototype.bind method.
22. Name some of the JavaScript Frameworks
- A JavaScript framework is an application framework that developers write in JavaScript. It differs from a JavaScript library in how it controls flow.
- There are several JavaScript frameworks available, but among the most frequently used ones are:
- Angular
- React
- Vue
23. What is the syntax for a try-catch block in JavaScript?
The syntax for a try-catch block in JavaScript is:
try { statement1; } catch (error) { statement2; } |
24. What is the spread operator in JavaScript?
- The spread operator (…) is a syntax that allows an iterable (like an array or string) to be expanded into individual elements.
- The spread operator can be used in function calls, array literals, and object literals to provide a more concise syntax for working with collections of values.
- The spread operator can also be used to concatenate arrays or objects and to create shallow copies of objects.
25. Explain the difference between let and const in JavaScript.
- Both let and const are block-scoped variables introduced in ES6.
- The difference between them
26. What is the difference between const and readonly in JavaScript?
const (JavaScript) | readonly (TypeScript) |
---|---|
Used to declare a variable that cannot be reassigned. | Used to declare a property of an object or a class that cannot be reassigned after initialization. |
Example: const PI = 3.14159; |
Example: class Person { readonly name: string; constructor(name: string) { this.name = name; } } |
Can be used with primitive types, objects, and arrays. | Can be used with properties of objects and class properties. |
Example: const x = 5; <br> const obj = { name: 'John', age: 30 }; <br> const arr = [1, 2, 3]; |
Example: class Person { readonly name: string; constructor(name: string) { this.name = name; } } <br> const obj = { name: 'John', age: 30 } as const; <br> type Person = { readonly name: string; age: number; }; |
Cannot be redeclared in the same scope. | Cannot be redeclared in the same scope. |
Example: const x = 5; <br> let x = 10; // SyntaxError: Identifier 'x' has already been declared |
Example: class Person { readonly name: string; constructor(name: string) { this.name = name; } } <br> class Person { readonly age: number; constructor(age: number) { this.age = age; } } // SyntaxError: Cannot assign to 'age' because it is a read-only property. |
27. What are arrow functions in JavaScript?
- Arrow functions are a shorthand syntax for writing function expressions in JavaScript.
- They are anonymous functions and do not have their own “this” value.
- They have a more concise syntax and are often used for callback functions.
28. What is the purpose of the ‘this’ keyword in JavaScript?
- The “this” keyword in JavaScript refers to the object that the function is a method of.
- It can also refer to the global object in non-strict mode, or be undefined in strict mode.
29. What is the difference between call and apply in JavaScript?
Aspect | call | apply |
---|---|---|
Syntax | function.call(thisArg, arg1, arg2, ...) |
function.apply(thisArg, [arg1, arg2, ...]) |
Arguments | Accepts comma-separated arguments | Accepts an array-like object of arguments |
Usage | Ideal for a small, known number of arguments | Ideal for a dynamic or unknown number of arguments |
Performance | Faster for a small number of arguments | Slower for a small number of arguments |
Compatibility | Works with arguments object and spread syntax | Works with array-like objects and apply methods |
Functionality | Calls the function with the specified this value and arguments |
Calls the function with the specified this value and an array of arguments |
Example | myFunction.call(this, arg1, arg2) |
myFunction.apply(this, [arg1, arg2]) |
30. Explain the difference between == and === in JavaScript.
== Operator | === Operator |
---|---|
Checks if the operands are equal without checking their types. | Checks if the operands are equal and checks their types. |
Performs type coercion. | Does not perform type coercion. |
If the operands have different types, JavaScript coerces one or both of them to a common type. | If the operands have different types, JavaScript does not coerce them and returns false. |
Example: "1" == 1 is true. |
Example: "1" === 1 is false. |
Example: null == undefined is true. |
Example: null === undefined is false. |
Example: true == 1 is true. |
Example: true === 1 is false. |
31. Explain the concept of higher-order functions in JavaScript.
- Higher-order functions are functions that take one or more functions as arguments, or return a function as their result.
- They allow for a more modular and reusable code, and enable functional programming paradigms in JavaScript.
32. What is the difference between map and filter in JavaScript?
- Both map() and filter() are array methods in JavaScript.
- map() creates a new array with the results of calling a provided function on every element in the array.
- filter() creates a new array with all elements that pass the test implemented by the provided function.
33. How do you declare a JavaScript function with a default parameter?
To declare a function with a default parameter in JavaScript, use the equals sign = after the parameter name: function myFunction(param1 = defaultValue) { statement; }
34. What are generators in JavaScript?
- Generators are functions in JavaScript that can be paused and resumed.
- They use the “yield” keyword to pause the function and return a value.
- They can be used for asynchronous programming, and are often used in combination with Promises.
35. What is the difference between a for loop and a forEach loop in JavaScript?
- A for loop is a traditional loop that iterates over an array or an object.
- A forEach loop is a method on arrays that calls a provided function once for each element in the array.
36. What are template literals in JavaScript?
- Template literals are a new way to create strings in JavaScript.
- They use backticks (`) instead of quotes, and allow for the interpolation of variables and expressions using ${ } syntax.
37. What are the different methods for string manipulation in JavaScript?
Some of the common string methods in JavaScript are:
- toUpperCase() and toLowerCase() for changing the case of a string.
- concat() for concatenating two or more strings.
- trim() for removing whitespace from the beginning and end of a string.
- slice() and substring() for extracting parts of a string.
- split() for splitting a string into an array of substrings based on a delimiter.
38. What is a closure and how does it work in JavaScript?
- A closure is a function that has access to variables in its outer (enclosing) function, even after the outer function has returned.
- This is achieved through the creation of a “closure” scope that retains a reference to the outer variables.
- Closures are often used for creating private variables and encapsulation in JavaScript.
39. What is the difference between class and function components in React?
Class Components | Function Components |
---|---|
Extends the React.Component class to create a component. |
A JavaScript function that returns a React element. |
Can contain state and lifecycle methods. | Can use hooks to contain state and lifecycle methods. |
Defined using ES6 classes. | Defined using JavaScript functions. |
Uses this.props to access props. |
Uses props as an argument to access props. |
Can use the constructor() method to set the initial state. |
Can use the useState() hook to set the initial state. |
Can use the componentDidMount() method to perform actions after the component is mounted. |
Can use the useEffect() hook to perform actions after the component is mounted. |
Can use the render() method to return JSX. |
Returns JSX directly from the function body. |
Example:<br>class MyComponent extends React.Component { <br>render() { <br>return <h1>Hello, {this.props.name}!</h1>; <br>} <br>} |
Example:<br>function MyComponent(props) { <br>return <h1>Hello, {props.name}!</h1>; <br>} <br>export default MyComponent; |
40. What are the different ways to handle errors in JavaScript?
- Using try…catch statement
- Using throw statement
- Using Error objects
- Using window.onerror event handler
41. What is the syntax for a rest parameter in JavaScript?
The syntax for a rest parameter in JavaScript is to use three dots … before the parameter name in the function declaration: function myFunction(…myParams) { statement; }
42. What are the built-in methods and the values returned by them?
Built-in Method | Values |
CharAt() | It returns the character at the specified index. |
Concat() | It joins two or more strings. |
length() | It returns the length of the string. |
pop() | It removes the last element from an array and returns that element. |
forEach() | It calls a function for each element in the array. |
indexOf() | It returns the index within the calling String object of the first occurrence of the specified value. |
push() | It adds one or more elements to the end of an array and returns the new length of the array. |
reverse() | It reverses the order of the elements of an array. |
43. What is a module in JavaScript?
A module in JavaScript is a way to organize code by encapsulating related functionality. It allows us to split a large codebase into smaller, more manageable pieces, which can be imported and used in other parts of our application.
44. What is the difference between export and export default in JavaScript?
- The export statement is used to export named bindings while the export default statement is used to export a default binding.
- With export, we can export multiple named bindings from a module, while with export default, we can only export one default binding.
45. What is the difference between async and defer in JavaScript?
- async and defer are attributes used in the script tag to control when a script is loaded and executed.
- async loads the script asynchronously and executes it as soon as it is available, while defer loads the script asynchronously and defers its execution until the document has been parsed.
46. What is the difference between innerHTML and outerHTML in JavaScript?
- innerHTML gets or sets the HTML content of an element’s descendants.
- outerHTML gets or sets the HTML content of an element and its descendants, including the element itself.
47. What is the difference between local and global variables in JavaScript?
- Local variables are declared inside a function and can only be accessed within that function, while global variables are declared outside of any function and can be accessed from anywhere in the code.
- Local variables are only accessible while the function is executing, while global variables remain accessible throughout the entire lifecycle of the application.
48. What is the difference between window and document in JavaScript?
- The window object represents the browser window and provides methods and properties for interacting with it.
- The document object represents the HTML document loaded into the browser and provides methods and properties for interacting with the document’s content.
49. What is the difference between innerText and textContent in JavaScript?
- innerText gets or sets the text content of an element and its descendants.
- textContent gets or sets the text content of an element and its descendants, including whitespace and newline characters.
50. How do you declare a JavaScript class?
To declare a class in JavaScript, use the class keyword followed by the class name and curly braces {} with the class properties and methods inside: class MyClass { property1; method1() { statement; } }
51. What is the difference between parentNode and parentElement in JavaScript?
- parentNode gets the parent node of an element, which can be any type of node.
- parentElement gets the parent element of an element, which can only be an element node.
52. What is the difference between children and childNodes in JavaScript?
- ‘children’ and ‘childNodes’ are both properties of a DOM element in JavaScript.
- ‘children’ only returns elements that are direct children of the element, while ‘childNodes’ returns all types of nodes, including text nodes and comments.
53. What is the difference between createElement and createTextNode in JavaScript?
- ‘createElement’ is a method that creates a new element node with the specified tag name.
- ‘createTextNode’ is a method that creates a new text node with the specified text content.
54. What is the difference between setAttribute and style in JavaScript?
- ‘setAttribute’ is a method that sets the value of a specified attribute on an element.
- ‘style’ is a property that allows you to set the inline styles of an element directly.
55. What is the difference between offsetWidth and clientWidth in JavaScript?
- ‘offsetWidth’ is a property that returns the width of an element, including the padding, border, and scrollbar.
- ‘clientWidth’ is a property that returns the width of an element, excluding the padding and scrollbar.
56. What is the difference between offsetHeight and clientHeight in JavaScript?
- ‘offsetHeight’ is a property that returns the height of an element, including the padding, border, and scrollbar.
- ‘clientHeight’ is a property that returns the height of an element, excluding the padding and scrollbar.
57. What is the difference between offsetLeft and clientLeft in JavaScript?
- ‘offsetLeft’ is a property that returns the distance between the left edge of an element and the left edge of its offset parent element.
- ‘clientLeft’ is a property that returns the width of the left border of an element.
58. What is the syntax for importing a module in JavaScript?
The syntax for importing a module in JavaScript is:
import { exportedName } from ‘module-name’; or import * as myModule from ‘module-name’; |
59. What is the difference between offsetTop and clientTop in JavaScript?
- ‘offsetTop’ is a property that returns the distance between the top edge of an element and the top edge of its offset parent element.
- ‘clientTop’ is a property that returns the width of the top border of an element.
60. What is the difference between scrollWidth and clientWidth in JavaScript?
- ‘scrollWidth’ is a property that returns the total width of an element, including any overflowed content.
- ‘clientWidth’ is a property that returns the visible width of an element, excluding the scrollbar.
61. What is the difference between scrollHeight and clientHeight in JavaScript?
- ‘scrollHeight’ is a property that returns the total height of an element, including any overflowed content.
- ‘clientHeight’ is a property that returns the visible height of an element, excluding the scrollbar.
62. What is the difference between scrollLeft and clientLeft in JavaScript?
- ‘scrollLeft’ is a property that sets or returns the number of pixels an element’s content is scrolled horizontally.
- ‘clientLeft’ is a property that returns the width of the left border of an element.
63. What is the difference between scrollTop and clientTop in JavaScript?
- scrollTop is a property that gets or sets the number of pixels that an element’s content is scrolled vertically.
- clientTop is a property that gets the height of an element’s top border.
64. What is the difference between getBoundingClientRect and offsetTop in JavaScript?
- getBoundingClientRect is a method that returns an object with the dimensions and position of an element relative to the viewport.
- offsetTop is a property that gets the distance between an element’s top edge and the top of its offset parent.
65. What is the difference between getComputedStyle and currentStyle in JavaScript?
- getComputedStyle is a method that returns an object containing the computed style of an element, including styles inherited from parent elements and styles applied by CSS rules.
- currentStyle is a property that gets the computed style of an element in Internet Explorer.
66. What is the difference between bind and call in JavaScript?
- Both bind and call are methods that allow you to set the value of this in a function.
- bind returns a new function with the value of this permanently set to the specified value, while call immediately invokes the function with the specified value of this.
67. What is the difference between indexOf and lastIndexOf in JavaScript?
- indexOf is a method that returns the index of the first occurrence of a specified value in an array or string, or -1 if the value is not found.
- lastIndexOf is a method that returns the index of the last occurrence of a specified value in an array or string, or -1 if the value is not found.
68. What is the difference between slice and splice in JavaScript?
- slice is a method that returns a portion of an array as a new array, without modifying the original array.
- splice is a method that adds or removes elements from an array, modifying the original array.
69. What is the difference between push and pop in JavaScript?
- push is a method that adds one or more elements to the end of an array and returns the new length of the array.
- pop is a method that removes the last element from an array and returns the removed element.
70. What is the difference between shift and unshift in JavaScript?
- shift is a method that removes the first element from an array and returns the removed element.
- unshift is a method that adds one or more elements to the beginning of an array and returns the new length of the array.
71. What is the difference between forEach and map in JavaScript?
Aspect | map() | forEach() |
---|---|---|
Return value | Returns a new array with the same length as the original array | Does not return a new array |
Mutability | Does not modify the original array | Does not modify the original array |
Usage | Used when a new array needs to be generated or transformed | Used when each element of the array needs to be operated on |
Callback | Invokes a callback function for each element of the array | Invokes a callback function for each element of the array |
Arguments | Passes the current element, index, and array to the callback | Passes the current element, index, and array to the callback |
Execution order | Executes the callback function first, then returns the new array | Executes the callback function for each element of the array in order |
Breaking | Can be broken early using some or every method |
Cannot be broken early using any built-in method |
72. What is the difference between reduce and reduceRight in JavaScript?
- reduce is a method that applies a function to each element in an array, accumulating a single value from left to right.
- reduceRight is a method that applies a function to each element in an array, accumulating a single value from right to left.
73. What is the difference between some and every in JavaScript?
- both some() and every() are array methods in JavaScript.
- some() returns true if at least one element in the array passes the test provided by a given function.
- every() returns true if all elements in the array pass the test provided by a given function.
74. What is the difference between find and findIndex in JavaScript?
- Both find() and findIndex() are array methods in JavaScript.
- find() returns the first element in the array that passes the test provided by a given function, or undefined if none do.
- findIndex() returns the index of the first element in the array that passes the test provided by a given function, or -1 if none do.
75. What is the difference between Object.keys() and Object.values() in JavaScript?
- Object.keys() is a method in JavaScript that returns an array of the keys (property names) of a given object.
- Object.values() is a method in JavaScript that returns an array of the values (property values) of a given object.
76. What is the difference between Object.freeze() and Object.seal() in JavaScript?
- Object.freeze() is a method in JavaScript that prevents any changes to the properties of a given object.
- Object.seal() is a method in JavaScript that prevents any new properties from being added to a given object, but allows for the modification of existing properties.
77. What is the difference between Object.assign() and spread operator in JavaScript?
- Object.assign() is a method in JavaScript that copies the properties of one or more source objects into a target object.
- The spread operator (…) is a new feature in JavaScript that allows for the copying of arrays and objects into new arrays or objects.
78. What is the difference between promises and callbacks in JavaScript?
- Both promises and callbacks are used for asynchronous programming in JavaScript.
- Promises are objects that represent the eventual completion (or failure) of an asynchronous operation, and allow for more structured error handling and chaining of multiple asynchronous operations.
- Callbacks are functions that are passed as arguments to other functions, and are executed when a certain event occurs (such as the completion of an asynchronous operation).
79. What is the difference between synchronous and asynchronous programming in JavaScript?
- Synchronous programming in JavaScript is when each statement in the code is executed one after the other, in a linear order.
- Asynchronous programming in JavaScript is when certain operations (such as network requests or file I/O) are performed in the background, without blocking the execution of the rest of the code.
80. How do you handle errors in promises in JavaScript?
- Errors in promises can be handled using the .catch() method, which allows for the handling of any errors that occur during the execution of the promise.
- This method can be chained onto the end of a promise chain, and can also be used to convert any rejected promises into resolved promises.
81. What is the difference between the Event object and the EventTarget object in JavaScript?
- The Event object in JavaScript represents an event that has occurred (such as a click or a keypress).
- The EventTarget object represents an object that can receive events and has methods for adding and removing event listeners.
82. What is the difference between event bubbling and event capturing in JavaScript?
Aspect | Event Bubbling | Event Capturing |
---|---|---|
Definition | When an event occurs on a nested element, it first triggers on the most specific element and then triggers on the ancestors until it reaches the top of the DOM tree | When an event occurs on a nested element, it first triggers on the top of the DOM tree and then triggers on the ancestors until it reaches the most specific element |
Direction | Bottom-up | Top-down |
Default behavior | Enabled | Disabled |
DOM Level | Level 2 | Level 2 |
Event order | The innermost element’s event handler is called first and then the outer elements’ event handlers are called in order up to the document’s event handler | The outermost element’s event handler is called first and then the inner elements’ event handlers are called in order down to the target element’s event handler |
Event phases | Bubbling phase, Target phase, Capturing phase | Capturing phase, Target phase, Bubbling phase |
Event listeners | Registered using the addEventListener() method with the third parameter set to false (default) | Registered using the addEventListener() method with the third parameter set to true |
83. What is the difference between bubbling and capturing in JavaScript event propagation?
- Event propagation in JavaScript can occur in two ways: bubbling and capturing.
- Bubbling is when an event is first captured by the innermost element, and then propagated up through its ancestors.
- Capturing is when an event is first captured by the outermost element, and then propagated down through its descendants.
84. How do you prevent default actions in JavaScript events?
- Using event.preventDefault() method
- Returning false from an event listener function
85. What are the different types of event listeners in JavaScript?
- DOM event listeners
- Window event listeners
- Form event listeners
- Keyboard event listeners
- Mouse event listeners
- Media event listeners
- Drag and drop event listeners
86. What is the difference between addEventListener and attachEvent in JavaScript?
- addEventListener is the standard method for attaching an event listener to an element in modern browsers.
- attachEvent is an older method used in Internet Explorer versions 6 to 8.
87. How do you create a custom event in JavaScript?
- Using the CustomEvent constructor
- Dispatching the custom event using the dispatchEvent method
88. What is the difference between class and prototype-based inheritance in JavaScript?
- Class-based inheritance is a way of creating objects that involves defining a class and creating instances of that class.
- Prototype-based inheritance is a way of creating objects by defining a prototype object and creating new objects that inherit from that prototype.
89. How do you inherit from multiple objects in JavaScript?
- Using object composition
- Using the Object.assign() method to merge multiple objects into a single object
90. What is the difference between the new keyword and Object.create() in JavaScript?
- The new keyword is used to create a new instance of a constructor function.
- Object.create() is used to create a new object that inherits from a specified prototype object.
91. What is the difference between the bind() and apply() methods in JavaScript?
- The bind() method returns a new function with a bound this value and optional arguments.
- The apply() method calls a function with a specified this value and arguments provided as an array.
92. How do you create a closure in JavaScript?
- By defining a function inside another function and returning it
- By using a function expression to assign a function to a variable and accessing that variable outside of the function
93. What is the difference between var, let, and const in JavaScript?
- var is function-scoped and can be redeclared and reassigned.
- let and const are block-scoped and cannot be redeclared in the same block, but let can be reassigned while const cannot be reassigned.
94. What is hoisting in JavaScript?
- Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their respective scopes.
- This means that variables and functions can be used before they are declared.
95. What are callback functions in JavaScript?
- A callback function is a function that is passed as an argument to another function and is executed after some operation is completed.
- Callback functions are commonly used in asynchronous programming, where code execution is not blocked and other operations can be performed while waiting for a response.
- In JavaScript, callbacks are often used with event listeners, timeouts, and AJAX requests.
96. Explain the concept of closures in JavaScript.
- A closure is a function that has access to variables from an outer function that has already returned.
- Closures allow functions to access variables from their parent scope even after the parent function has completed execution.
- Closures are often used to create private variables and functions that cannot be accessed from outside the closure.
97. What is the difference between a function declaration and a function expression in JavaScript?
- Function declarations are hoisted while function expressions are not.
- Function declarations can be called before they are declared while function expressions cannot.
- Function declarations create named functions while function expressions can be anonymous.
98. What is the difference between Local storage & Session storage?
- Local Storage is a client-side storage mechanism that reduces traffic between the client and server by storing data locally, and it persists until it is cleared manually through program settings.
- Session Storage is similar to Local Storage, but the data stored in Session Storage gets cleared automatically when the page session ends, while data stored in Local Storage has no expiration time.
- If you encounter any difficulties with these JavaScript interview questions, please feel free to comment on your challenges in the section below.
99. What is the difference between innerHTML & innerText?
- innerHTML – It will process an HTML tag if found in a string
- innerText – It will not process an HTML tag if found in a string
100. What is destructuring in JavaScript?
- Destructuring is a way to extract values from objects and arrays and assign them to variables.
- Destructuring can be used to extract values from complex data structures without having to manually access each value.
- In JavaScript, destructuring is done using syntax like { property } for objects and [ element ] for arrays.
The Top 100 JavaScript Interview Questions and Answers provide comprehensive coverage of the latest technical interview topics, ensuring candidates are well-prepared for any web development role. To acquire further knowledge, we encourage you to follow us at freshersnow.com.