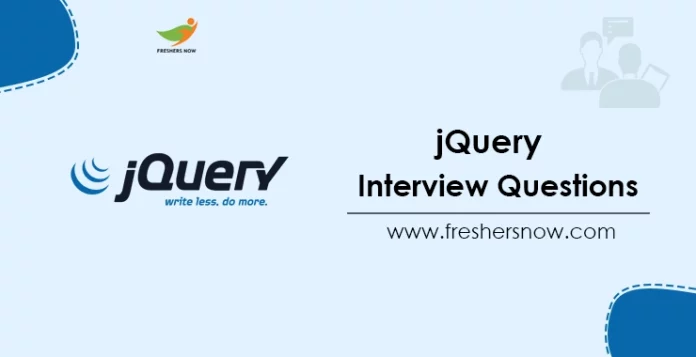
jQuery Interview Questions and Answers: If you are preparing for a jQuery technical interview, you might be looking for some guidance on the types of questions that you can expect. To help you out, we have compiled a list of the top 100 jQuery interview questions and answers that are frequently asked in interviews. Whether you are a fresher or an experienced professional, this list covers the latest jQuery interview questions and answers that can help you prepare for your next interview.
★★ Latest Technical Interview Questions ★★
jQuery Technical Interview Questions
By going through these jQuery interview questions for freshers and experienced professionals, you can not only test your knowledge but also improve your understanding of the jQuery framework. So, let’s dive into the world of jQuery interview questions and answers!
Top 100 jQuery Interview Questions and Answers
1. What is jQuery and what are its main features?
- jQuery is a popular JavaScript library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for web development.
- Main features of jQuery are:
- DOM manipulation
- Event handling
- Animation effects
- Ajax support
- Extensibility through plugins
- Cross-browser compatibility
2. What is the difference between jQuery and JavaScript?
- JavaScript is a programming language used for creating dynamic web pages and browser-based applications.
- jQuery is a library built with JavaScript that simplifies and enhances JavaScript coding by providing ready-to-use functions and syntax.
- jQuery is a tool to write JavaScript code more efficiently.
3. What is the syntax for selecting an element by ID in jQuery?
Syntax:
$(“#id”) |
Example: $(“#myDiv”) will select the element with the ID “myDiv”.
4. What is a selector in jQuery?
- A selector in jQuery is a function that is used to select HTML elements based on their name, class, ID, attributes, and more.
- jQuery uses CSS-like syntax to select elements, for example: $(“p”) selects all <p> elements, $(“.myClass”) selects all elements with class “myClass”, $(“#myId”) selects the element with ID “myId”.
5. What are the effects methods used in jQuery?
jQuery has several effects methods which are used to manipulate the display of elements on a webpage.
- The show() method is used to display or show the selected elements.
- The hide() method is used to hide the matched or selected elements.
- The toggle() method is used to show or hide the matched elements, toggling between the hide() and show() methods.
- The fadeIn() method is used to show the matched elements by fading it to opaque.
- The fadeOut() method is used to show the matched elements by fading it to transparent, effectively fading out the selected elements.
6. What is the use of $ symbol in jQuery?
- In jQuery, the $ symbol is used as a shorthand for the jQuery() function.
- It is used to access jQuery methods and functions, like $(“p”).hide() to hide all <p> elements.
7. What is the difference between the hide() and fadeOut() methods in jQuery?
Here’s a tabular format to summarize the differences between the hide() and fadeOut() methods in jQuery:
Hide() Method | FadeOut() Method |
---|---|
Hides selected elements instantly without any animation | Hides selected elements by gradually reducing their opacity over a specified duration |
Does not change the space occupied by the hidden elements | Changes the space occupied by the hidden elements as their opacity decreases |
Syntax: $(selector).hide(speed,callback) | Syntax: $(selector).fadeOut(speed,callback) |
Example: $(“p”).hide() | Example: $(“p”).fadeOut() |
8. How do you add a class to an element using jQuery?
- To add a class to an element using jQuery, use the addClass() method.
- Example: $(“p”).addClass(“myClass”) adds the class “myClass” to all <p> elements.
9. How do you remove a class from an element using jQuery?
- To remove a class from an element using jQuery, use the removeClass() method.
- Example: $(“p”).removeClass(“myClass”) removes the class “myClass” from all <p> elements.
10. What is the syntax for selecting an element by class in jQuery?
Syntax:
$(“.class”) |
Example: $(“.myClass”) will select all elements with the class “myClass”.
11. How do you select all elements with a certain class using jQuery?
- To select all elements with a certain class using jQuery, use the dot notation followed by the class name.
- Example: $(“.myClass”) selects all elements with class “myClass”.
12. How do you select all elements with a certain ID using jQuery?
- To select the element with a certain ID using jQuery, use the hash notation followed by the ID name.
- Example: $(“#myId”) selects the element with ID “myId”.
13. How do you select all elements of a certain type using jQuery?
- To select all elements of a certain type using jQuery, use the tag name without any prefix.
- Example: $(“p”) selects all <p> elements.
14. What is the syntax for selecting multiple elements in jQuery?
Syntax:
$(“selector1, selector2, selector3, …”) |
Example: $(“p, .myClass, #myDiv”) will select all <p> elements, elements with the class “myClass”, and the element with the ID “myDiv”.
15. What is the difference between the on() and click() methods in jQuery?
click() Method | on() Method |
---|---|
Attaches a click event handler to the selected elements | Attaches event handlers to the selected elements for a specified event |
Only attaches event handlers to the currently selected elements | Can attach event handlers to elements that are added to the DOM at a later time |
Only works for click events | Can be used for any event type, such as hover, focus, blur, etc. |
Syntax: $(selector).click(function) | Syntax: $(selector).on(event, function) |
Example: $(“p”).click(function() {…}) | Example: $(“p”).on(“click”, function() {…}) |
16. What is the purpose of the ready() method in jQuery?
- The ready() method in jQuery is used to execute a function when the DOM is fully loaded and ready to be manipulated.
- It ensures that the code inside the function is executed only when the DOM is ready.
- Example: $(document).ready(function(){ // code here });
17. How do you create a jQuery object?
To create a jQuery object, you can use the following syntax:
var jQueryObject = $(selector); |
where the selector parameter can be any valid CSS selector.
18. What is the difference between the find() and children() methods in jQuery?
The find() method searches for all descendant elements that match the specified selector, while the children() method only searches for immediate child elements that match the selector.
19. How do you add a new element to the DOM using jQuery?
To add a new element to the DOM using jQuery, you can use the following syntax:
$(parentSelector).append(newElement); |
where parentSelector is the selector for the parent element, and newElement is the HTML element that you want to add.
20. What is the difference between the append() and appendTo() methods in jQuery?
append() Method | appendTo() Method |
---|---|
Appends the specified content as the last child of the selected elements | Appends the selected elements as the last child of the specified content |
Syntax: $(selector).append(content) | Syntax: $(content).appendTo(selector) |
Example: $(“p”).append(“Hello World”) | Example: $(“<p>Hello World</p>”).appendTo(“body”) |
Can add multiple elements or text content to the end of each selected element | Can add a single element or multiple elements to the end of the specified container |
Can be used with a callback function to generate content dynamically | Can be chained with other methods to modify the appended elements after they are inserted |
Modifies the selected elements | Modifies the content that is passed in as an argument |
21. How do you remove an element from the DOM using jQuery?
To remove an element from the DOM using jQuery, you can use the following syntax:
$(selector).remove(); |
where selector is the selector for the element that you want to remove.
22. How do you change the content of an element using jQuery?
To change the content of an element using jQuery, you can use the following syntax:
$(selector).html(newContent); |
where selector is the selector for the element that you want to modify, and newContent is the new HTML content that you want to set.
23. How do you set an attribute of an element using jQuery?
To set an attribute of an element using jQuery, you can use the following syntax:
$(selector).attr(attributeName, attributeValue); |
where selector is the selector for the element that you want to modify, attributeName is the name of the attribute that you want to set, and attributeValue is the value that you want to set for the attribute.
24. How do you get the value of an input element using jQuery?
To get the value of an input element using jQuery, you can use the following syntax:
var inputValue = $(selector).val(); |
where selector is the selector for the input element.
25. How do you set the value of an input element using jQuery?
To set the value of an input element using jQuery, you can use the following syntax:
$(selector).val(newValue); |
where selector is the selector for the input element, and newValue is the new value that you want to set.
26. What is the purpose of the each() method in jQuery?
The each() method is used to iterate over a collection of elements and perform a function on each element. It can be used to perform a variety of tasks, such as modifying the elements or gathering data from them.
27. What is the difference between the toggleClass() and addClass() methods in jQuery?
addClass() Method | toggleClass() Method |
---|---|
Adds one or more classes to the selected elements | Toggles one or more classes on the selected elements |
Syntax: $(selector).addClass(classname) | Syntax: $(selector).toggleClass(classname) |
Example: $(“p”).addClass(“highlight”) | Example: $(“p”).toggleClass(“highlight”) |
Always adds the specified class(es) to the selected elements | Adds the specified class(es) if they are not already present, and removes them if they are |
Does not affect any other classes that may already be present on the selected elements | Can be used to toggle multiple classes at once by passing a space-separated list of class names as the argument |
Modifies the selected elements | Modifies the selected elements based on their current state |
28. What is the purpose of the map() method in jQuery?
The map() method is used to transform a collection of elements into a new array based on a function that is applied to each element. It can be used to create a new array of modified elements or to extract a specific property from each element in the collection.
29. How do you animate an element using jQuery?
- Use the animate() method and specify the CSS properties to be animated.
- Set the duration of the animation and optionally add a callback function to be executed after the animation.
30. What is the difference between the animate() and fade() methods in jQuery?
- The animate() method animates the CSS properties of an element over a specified duration.
- The fade() method changes the opacity of an element to create a fading effect.
31. How do you make an AJAX request using jQuery?
- Use the jQuery AJAX method such as $.ajax() or its shorthand equivalents $.get(), $.post() etc.
- Specify the URL to send the request to, the data to be sent, and the type of request (GET, POST, etc.).
- Optionally, add success and error callback functions to handle the response.
32. What is the syntax for chaining methods in jQuery?
Syntax:
$(selector).method1().method2().method3()… |
Example: $(“p”).addClass(“myClass”).hide().fadeIn() will add the class “myClass” to all <p> elements, then hide them, and then fade them in.
33. What is the difference between the get() and post() methods in jQuery AJAX?
- The get() method sends an AJAX request using the HTTP GET method.
- The post() method sends an AJAX request using the HTTP POST method.
- The get() method appends the data to the URL, while the post() method sends the data in the request body.
34. How do you handle AJAX errors in jQuery?
- Use the error callback function to handle AJAX errors.
- The error function is executed when the AJAX request encounters an error such as network issues, server errors, or invalid JSON responses.
35. What is the syntax for setting a CSS property using the css() method in jQuery?
Syntax:
$(selector).css(“property”, “value”) |
Example: $(“p”).css(“color”, “red”) will set the color of all <p> elements to red.
36. What is the difference between the slideDown() and slideToggle() methods in jQuery? in tabular format
slideDown() Method | slideToggle() Method |
---|---|
Displays the selected elements by sliding them down | Toggles the visibility of the selected elements by sliding them up or down |
Syntax: $(selector).slideDown(speed,callback) | Syntax: $(selector).slideToggle(speed,callback) |
Example: $(“p”).slideDown() | Example: $(“p”).slideToggle() |
Can only be used to show elements | Can be used to show or hide elements depending on their current visibility state |
Always slides the selected elements down from their current position | Slides the selected elements up or down depending on whether they are currently visible or not |
Modifies the selected elements | Modifies the visibility of the selected elements based on their current state |
37. How do you use the JSON data type in jQuery AJAX?
- Set the dataType option to ‘json’ in the AJAX request.
- The response data will automatically be parsed as a JSON object.
38. How do you use the XML data type in jQuery AJAX?
- Set the dataType option to ‘xml’ in the AJAX request.
- The response data will automatically be parsed as an XML document.
39. How do you use the JSONP data type in jQuery AJAX?
- Set the dataType option to ‘jsonp’ in the AJAX request.
- Use a JSONP-enabled server that wraps the response in a callback function specified in the URL.
40. What is the difference between synchronous and asynchronous AJAX requests in jQuery?
- Synchronous AJAX requests block the user interface until the response is received.
- Asynchronous AJAX requests do not block the user interface and allow other interactions while waiting for the response.
41. What is the syntax for adding an event handler using the on() method in jQuery?
Syntax:
$(selector).on(event, function) |
Example: $(“button”).on(“click”, function() { alert(“Button clicked!”); }) will add a click event handler to all <button> elements that displays an alert when clicked.
42. How do you chain multiple jQuery methods together?
- Use dot notation to chain jQuery methods together.
- Each method is executed on the returned object of the previous method, allowing for more concise and readable code.
43. What is the difference between the html() and text() methods in jQuery?
html() Method | text() Method |
---|---|
Gets or sets the HTML content of the selected elements, including any HTML tags | Gets or sets the text content of the selected elements, without any HTML tags |
Syntax: $(selector).html() or $(selector).html(content) | Syntax: $(selector).text() or $(selector).text(content) |
Example: $(“p”).html() or $(“p”).html(“<strong>Hello World</strong>”) | Example: $(“p”).text() or $(“p”).text(“Hello World”) |
Can be used to insert HTML content into the selected elements | Can be used to insert plain text content into the selected elements |
Can cause security vulnerabilities if user input is used to set the content | Does not allow for the insertion of HTML tags, preventing security vulnerabilities |
Modifies the selected elements | Modifies the selected elements |
44. How do you use the parent() method in jQuery?
- The parent() method in jQuery is used to get the direct parent element of the selected element.
- Syntax:
$(selector).parent() - Example: $(“span”).parent() will return the parent element of all <span> elements.
45. What is the syntax for removing an element from the DOM using the remove() method in jQuery?
Syntax: $(selector).remove()
Example: $(“#myDiv”).remove() will remove the element with the ID “myDiv” from the DOM.
46. How do you use the siblings() method in jQuery?
- The siblings() method in jQuery is used to get all the siblings of the selected element.
- Syntax:
$(selector).siblings() - Example: $(“span”).siblings() will return all the siblings of all <span> elements.
47. How do you use the next() and prev() methods in jQuery?
- The next() method in jQuery is used to get the next sibling element of the selected element.
- Syntax:
$(selector).next() - Example: $(“span”).next() will return the next sibling element of all <span> elements.
- The prev() method in jQuery is used to get the previous sibling element of the selected element.
- Syntax:
$(selector).prev() - Example: $(“span”).prev() will return the previous sibling element of all <span> elements.
48. What is the syntax for inserting content into an element using the html() method in jQuery?
Syntax:
$(selector).html(content) |
Example: $(“div”).html(“<p>Hello world!</p>”) will insert the HTML content “<p>Hello world!</p>” into all <div> elements.
49. What is the difference between the attr() and prop() methods in jQuery?
- The attr() method in jQuery is used to get or set the value of an attribute of an element.
- Syntax: $(selector).attr(attributeName) or $(selector).attr(attributeName, value)
- The prop() method in jQuery is used to get or set the value of a property of an element.
- Syntax: $(selector).prop(propertyName) or $(selector).prop(propertyName, value)
- The main difference between the two methods is that the attr() method works with the HTML attributes of an element, while the prop() method works with the DOM properties of an element.
50. How do you use the val() method in jQuery?
- The val() method in jQuery is used to get or set the value of form fields.
- Syntax:
$(selector).val() or $(selector).val(value) - Example: $(“input”).val() will return the value of the first <input> element, and $(“input”).val(“new value”) will set the value of all <input> elements to “new value”.
51. How do you use the css() method in jQuery?
- The css() method in jQuery is used to get or set the value of a CSS property of an element.
- Syntax:
$(selector).css(propertyName) or $(selector).css(propertyName, value) - Example: $(“div”).css(“background-color”) will return the background color of the first <div> element, and $(“div”).css(“background-color”, “red”) will set the background color of all <div> elements to red.
52. How do you use the height() and width() methods in jQuery?
- The height() method in jQuery is used to get or set the height of an element.
- Syntax: $(selector).height() or $(selector).height(value)
- Example: $(“div”).height() will return the height of the first <div> element, and $(“div”).height(“100px”) will set the height of all <div> elements to 100 pixels.
- The width() method in jQuery is used to get or set the width of an element.
- Syntax: $(selector).width() or $(selector).width(value)
- Example: $(“div”).width() will return the width of the first <div> element, and $(“div”).width(“200px”) will set the width of all <div> elements to 200 pixels.
53. What is the difference between the animate() and css() methods in jQuery?
animate() Method | css() Method |
---|---|
Animates the selected elements by changing CSS properties over time | Gets or sets the value of one or more CSS properties of the selected elements |
Syntax: $(selector).animate(properties,speed,easing,callback) | Syntax: $(selector).css(property,value) or $(selector).css({property1:value1, property2:value2, …}) |
Example: $(“div”).animate({left: ‘+=100px’}) | Example: $(“div”).css(“color”, “red”) or $(“div”).css({color: “red”, fontSize: “16px”}) |
Can be used to create custom animations with multiple CSS properties | Can only set or get the value of one or more CSS properties at a time |
Requires the use of properties that can be animated, such as numeric values or color codes | Can set or get any CSS property, including non-numeric values and non-visual properties such as “display” |
Uses animation options such as speed, easing, and callback functions to control the animation | Does not have animation options, but can be used to set or get CSS properties of the selected elements |
Modifies the selected elements by changing their CSS properties over time | Modifies the selected elements by setting or getting their CSS properties |
54. What is the purpose of the toggle() method in jQuery?
The toggle() method in jQuery is used to toggle between hiding and showing an element.
- Syntax: $(selector).toggle()
- Example: $(“div”).toggle() will hide or show all <div> elements depending on their current state.
55. How do you use the show() and hide() methods in jQuery?
- The show() method in jQuery is used to show an element.
- Syntax: $(selector).show()
- Example: $(“div”).show() will show all <div> elements.
- The hide() method in jQuery is used to hide an element.
- Syntax: $(selector).hide()
- Example: $(“div”).hide() will hide all <div> elements.
56. What is the syntax for creating a new element using the jQuery constructor?
Syntax: $(“<element>”)
Example: $(“<p>”) will create a new <p> element.
57. How do you use the fadeIn() and fadeOut() methods in jQuery?
- The fadeIn() method in jQuery is used to fade in an element.
- Syntax: $(selector).fadeIn()
- Example: $(“div”).fadeIn() will fade in all <div> elements.
- The fadeOut() method in jQuery is used to fade out an element.
- Syntax: $(selector).fadeOut()
- Example: $(“div”).fadeOut() will fade out all <div> elements.
58. How do you use the slideUp() and slideDown() methods in jQuery?
- slideUp() method hides the selected element with a sliding motion.
- slideDown() method shows the selected element with a sliding motion.
- Example: $(“p”).slideUp() hides all <p> elements with a sliding motion.
59. How do you use the delay() method in jQuery?
- delay() method is used to delay the execution of subsequent methods in the queue.
- Example: $(“p”).slideUp().delay(2000).slideDown() delays the execution of slideDown() method by 2 seconds after slideUp().
60. How do you use the queue() method in jQuery?
- queue() method allows adding custom functions to the default animation queue.
- Example: $(“p”).slideUp().queue(function() { $(this).css(“color”, “red”).dequeue(); }).slideDown() adds a custom function to the queue that changes the color of text to red after slideUp() method.
61. How do you use the stop() method in jQuery?
- stop() method is used to stop the currently running animation or effect.
- Example: $(“p”).stop() stops the currently running animation on all <p> elements.
62. How do you use the animate() method to create custom animations?
- animate() method is used to create custom animations on selected elements by changing CSS properties.
- Example: $(“p”).animate({left: ‘250px’, opacity: ‘0.5’}) moves all <p> elements 250px to the right and makes them semi-transparent.
63. What is the syntax for checking if an element has a specific class using the hasClass() method in jQuery?
Syntax:
$(selector).hasClass(“class”) |
Example: $(“p”).hasClass(“myClass”) will return true if any <p> element has the class “myClass”, and false otherwise.
64. What is the purpose of the unbind() method in jQuery?
- unbind() method is used to remove event handlers that were previously attached using the bind() method.
- Example: $(“p”).unbind(“click”) removes the click event handler from all <p> elements.
65. How do you use the on() method to attach event handlers in jQuery?
- on() method is used to attach event handlers to selected elements, even if they are added dynamically.
- Example: $(“p”).on(“click”, function(){ $(this).hide(); }) hides the clicked <p> element.
66. What is the difference between the val() and text() methods in jQuery?
val() Method | text() Method |
---|---|
Gets or sets the value of form elements, such as input, select, and textarea | Gets or sets the text content of the selected elements, without any HTML tags |
Syntax: $(selector).val() or $(selector).val(value) | Syntax: $(selector).text() or $(selector).text(content) |
Example: $(“input[type=’text’]”).val() or $(“input[type=’text’]”).val(“Hello World”) | Example: $(“p”).text() or $(“p”).text(“Hello World”) |
Can only be used on form elements with a “value” attribute, such as input, select, and textarea | Can be used on any element that contains text content |
Gets or sets the current value of the form element | Gets or sets the text content of the selected element, including any whitespace or line breaks |
Can be used to set a default value for form elements, such as setting the default value of a text input field | Can be used to set the text content of an element, such as changing the text of a paragraph |
Modifies the form element by changing its value | Modifies the selected element by changing its text content |
67. What is the difference between the click() and on() methods for attaching click event handlers in jQuery?
- click() method attaches a click event handler to selected elements, but it only works for elements that exist when the code is executed.
- on() method can attach click event handlers to both existing and dynamically added elements.
68. How do you use the hover() method in jQuery?
- hover() method is used to attach two event handlers to selected elements, one for when the mouse enters and one for when it leaves.
- Example: $(“p”).hover(function(){ $(this).addClass(“hover”) }, function(){ $(this).removeClass(“hover”) }) adds a “hover” class to a <p> element when the mouse enters and removes it when the mouse leaves.
69. What is the purpose of the delegate() method in jQuery?
- delegate() method is used to attach event handlers to elements that are added to the DOM dynamically, even if they don’t exist when the code is executed.
- It is a way to handle events on elements that are not yet created.
- Example: $( “table” ).delegate( “td”, “click”, function() { $( this ).toggleClass( “selected” ); }) toggles the “selected” class when a <td> element is clicked, even if it is added dynamically.
70. How do you use the ajaxStart() and ajaxStop() methods in jQuery?
The ajaxStart()
method is used to register a function to be called when an AJAX request is started, while the ajaxStop()
method is used to register a function to be called when all AJAX requests have completed. You can use these methods to show a loading animation or perform other tasks while an AJAX request is in progress.
71. How do you use the getJSON() method in jQuery?
The getJSON()
method is used to send an AJAX request to retrieve JSON data from a server. You can use this method to retrieve data from a server and then use it to update your web page.
72. How do you use the ajaxSetup() method in jQuery?
The ajaxSetup()
method is used to set default values for AJAX requests in jQuery. You can use this method to set default values for options such as type
, url
, data
, and dataType
.
73. What is the difference between the load() and get() methods in jQuery AJAX?
The load()
method is used to retrieve HTML content from a server and insert it into a specified element on the web page, while the get()
method is used to retrieve data from a server using AJAX. The get()
method allows you to specify the data type of the response, while the load()
method always retrieves HTML content.
74. What is the difference between the wrap() and wrapAll() methods in jQuery?
wrap() Method | wrapAll() Method |
---|---|
Wraps each selected element with HTML content | Wraps all selected elements as a group with HTML content |
Syntax: $(selector).wrap(wrapper) | Syntax: $(selector).wrapAll(wrapper) |
Example: $(“p”).wrap(“<div class=’wrapper’></div>”) | Example: $(“p”).wrapAll(“<div class=’wrapper’></div>”) |
Can be used to wrap each selected element with different HTML content | Wraps all selected elements with the same HTML content |
Can be used to wrap elements with dynamic HTML content, such as using a function to generate the wrapping element | Requires a single HTML element to wrap all selected elements |
Wraps the selected element(s) immediately inside the HTML content provided | Wraps the selected elements and any of their parent elements that are not already wrapped |
Adds the wrapping element as a sibling immediately before the selected element(s) | Adds the wrapping element as a parent element around the selected element(s) |
Modifies the selected element(s) by wrapping them with the provided HTML content | Modifies the selected element(s) by wrapping them with the provided HTML content as a group |
75. What is the purpose of the serialize() method in jQuery?
The serialize()
method is used to encode a set of form elements as a string for use in an AJAX request. You can use this method to easily send form data to a server using AJAX.
76. How do you use the each() method to loop through elements in jQuery?
The each()
method is used to loop through a collection of elements and perform a function on each element. You can use this method to modify the elements or gather data from them. To use the each()
method, you need to provide a function that will be called for each element in the collection.
77. What is the purpose of the data() method in jQuery?
The data()
method is used to attach data to a DOM element in jQuery. You can use this method to store arbitrary data for later use, such as state information or configuration options.
78. How do you use the extend() method in jQuery?
The extend()
method is used to merge two or more objects into a single object. You can use this method to combine configuration options or other data from multiple sources.
79. How do you use the clone() method in jQuery?
The clone()
method is used to create a copy of an element in the DOM. You can use this method to create a copy of an element that you can modify without affecting the original.
80. What is the purpose of the filter() method in jQuery?
The filter()
method is used to reduce the set of matched elements to those that match a specified selector or function. You can use this method to perform operations on a subset of the elements in a collection.
81. How do you use the hasClass() method in jQuery?
- Call the hasClass() method on the selected element and pass the class name as an argument.
- The method returns true if the element has the specified class, false otherwise.
82. What is the purpose of the is() method in jQuery?
- The is() method checks if an element matches a given selector.
- It returns true if the selected element matches the selector, false otherwise.
83. How do you use the toggleClass() method in jQuery?
- Call the toggleClass() method on the selected element and pass the class name as an argument.
- The method adds the class if it is not present and removes it if it is already present.
84. How do you use the closest() method in jQuery?
- Call the closest() method on a selected element and pass a selector as an argument.
- The method returns the closest ancestor element that matches the selector.
85. What is the purpose of the prevAll() method in jQuery?
- The prevAll() method selects all previous siblings of the selected element.
- It returns an array-like object of DOM elements.
86. How do you use the add() method in jQuery?
- Call the add() method on a selected element and pass a selector, element, or jQuery object as an argument.
- The method adds the specified elements to the selected set of elements.
87. What is the purpose of the remove() method in jQuery?
- The remove() method removes the selected element(s) from the DOM.
- It also removes any associated data and events.
88. How do you use the empty() method in jQuery?
Call the empty() method on a selected element to remove all of its child elements.
89. What is the purpose of the wrap() method in jQuery?
- The wrap() method wraps the selected element(s) with a specified HTML structure.
- It can be used to add additional markup or style to an element.
90. How do you use the after() method in jQuery?
- Call the after() method on a selected element and pass a content string, element, or jQuery object as an argument.
- The method inserts the specified content after the selected element.
91. What is the purpose of the not() method in jQuery?
- The not() method in jQuery is used to select all elements except the specified element(s) in the parameter.
- Syntax:
$(selector).not(element) - Example: $(“div”).not(“.special”) will select all <div> elements except the ones with class “special”.
92. How do you use the first() method in jQuery?
- The first() method in jQuery is used to select the first element in a set of matched elements.
- Syntax:
$(selector).first() - Example: $(“div”).first() will select the first <div> element.
93. What is the purpose of the last() method in jQuery?
- The last() method in jQuery is used to select the last element in a set of matched elements.
- Syntax:
$(selector).last() - Example: $(“div”).last() will select the last <div> element.
94. How do you use the eq() method in jQuery?
- The eq() method in jQuery is used to select a specific element based on its index in a set of matched elements.
- Syntax:
$(selector).eq(index) - Example: $(“div”).eq(2) will select the third <div> element.
95. What is the purpose of the slice() method in jQuery?
- The slice() method in jQuery is used to select a subset of elements in a set of matched elements.
- Syntax:
$(selector).slice(start, end) - Example: $(“div”).slice(1, 3) will select the second and third <div> elements.
96. How do you use the parentUntil() method in jQuery?
- The parentUntil() method in jQuery is used to select all ancestor elements of the selected element(s) until a specified element is found.
- Syntax:
$(selector).parentUntil(element) - Example: $(“span”).parentUntil(“div”) will select all ancestor elements of <span> elements until a <div> element is found.
97. What is the purpose of the childrenUntil() method in jQuery?
- The childrenUntil() method in jQuery is used to select all children elements of the selected element(s) until a specified element is found.
- Syntax:
$(selector).childrenUntil(element) - Example: $(“div”).childrenUntil(“p”) will select all child elements of <div> elements until a <p> element is found.
98. How do you use the prevUntil() and nextUntil() methods in jQuery?
- The prevUntil() method in jQuery is used to select all previous sibling elements of the selected element(s) until a specified element is found.
- Syntax: $(selector).prevUntil(element)
- Example: $(“span”).prevUntil(“div”) will select all previous sibling elements of <span> elements until a <div> element is found.
- The nextUntil() method in jQuery is used to select all next sibling elements of the selected element(s) until a specified element is found.
- Syntax: $(selector).nextUntil(element)
- Example: $(“span”).nextUntil(“div”) will select all next sibling elements of <span> elements until a <div> element is found.
99. What is the purpose of the one() method in jQuery?
- The one() method in jQuery is used to attach an event handler to an element that will only be executed once.
- Syntax:
$(selector).one(event, function) - Example: $(“button”).one(“click”, function() { alert(“Button clicked!”); }) will attach a click event handler to all <button> elements that will only be executed once.
100. How do you use the off() method to remove event handlers in jQuery?
- The off() method in jQuery is used to remove event handlers
- Syntax:
$(selector).off(event) - Example: $(“button”).off(“click”) will remove the click event handler from all <button> elements.
The Top 100 jQuery Interview Questions and Answers serve as a valuable resource for freshers and experienced professionals to prepare for technical interviews and enhance their jQuery knowledge. To expand your knowledge, follow us at freshersnow.com.