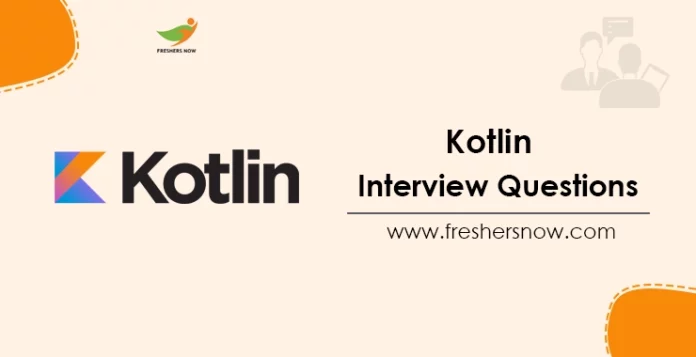
Kotlin Interview Questions and Answers: Kotlin, the modern programming language, has gained popularity among developers in recent years due to its concise syntax and interoperability with Java. As more and more companies adopt Kotlin, the demand for skilled Kotlin developers has increased. To help job seekers prepare for Kotlin technical interviews, we have compiled a list of the top 100 Kotlin Interview Questions and Answers. These latest Kotlin Interview Questions cover a range of topics, from basic syntax to advanced features, and are suitable for both experienced developers and freshers.
★★ Latest Technical Interview Questions ★★
Kotlin Technical Interview Questions
Whether you’re a seasoned Kotlin programmer or just starting with the language, our Kotlin Interview Questions for Freshers and experienced professionals can help you ace your next interview.
Top 100 Kotlin Interview Questions and Answers
1. What is Kotlin?
Answer: Kotlin is a statically typed, cross-platform programming language that runs on the Java Virtual Machine (JVM) and is designed to be a more concise and expressive alternative to Java.
2. What are the advantages of using Kotlin?
Answer: Some of the advantages of using Kotlin include improved code readability, reduced boilerplate code, better type inference, improved null-safety, support for functional programming, and seamless interoperability with Java code.
3. What is the difference between var and val in Kotlin?
- var is used to declare mutable variables, while val is used to declare immutable variables.
- Once assigned, the value of a val cannot be changed, while the value of a var can be changed.
4. What are the basic data types in Kotlin?
Answer: The basic data types in Kotlin are Byte, Short, Int, Long, Float, Double, Boolean, Char, and String.
5. What is the syntax for creating a range of values in Kotlin?
Example:
val range = 1..10 |
In this example, the range of values from 1 to 10 is created and stored in the variable range.
6. What is the difference between val and var in Kotlin?
Answer: “val” is used to declare a variable that is immutable (i.e., its value cannot be changed once it is assigned), while “var” is used to declare a variable that is mutable.
7. What is the difference between an interface and an abstract class in Kotlin?
- An interface in Kotlin defines a set of methods and properties that a class must implement.
- An abstract class in Kotlin is a class that cannot be instantiated and can have both abstract and non-abstract methods and properties.
8. What is the syntax for creating a nullable type in Kotlin?
Example:
var nullableString: String? = null |
In this example, the variable nullableString is declared as a nullable String type and initialized with a value of null.
9. What is a data class in Kotlin?
Answer: A data class in Kotlin is a class that is designed to hold data and has some built-in functionality, such as toString(), equals(), and hashCode().
10. What is a lambda expression in Kotlin?
Answer: A lambda expression in Kotlin is a function that can be passed around as a value, just like any other variable. It is defined using the syntax { argument-list -> body }.
11. What is the Elvis operator in Kotlin?
Answer: The Elvis operator (?:) in Kotlin is used to provide a default value when a nullable expression is null.
12. What is a higher-order function in Kotlin?
Answer: A higher-order function in Kotlin is a function that takes one or more functions as arguments or returns a function as its result.
13. What is the syntax for creating a lambda expression with a single parameter in Kotlin?
Example:
val square: (Int) -> Int = { num -> num * num } |
In this example, a lambda expression is defined with a single parameter num of type Int that returns the square of that number.
14. What are the differences between Kotlin and Java?
Feature | Kotlin | Java |
---|---|---|
Type Inference | Yes, supports | No, doesn’t support |
Null Safety | Yes, supports | No, null pointer exception is possible |
Interoperability | Yes, compatible with Java | Yes, fully compatible with itself |
Extension Functions | Yes, supports | No, doesn’t support |
Lambdas | Yes, supports | Yes, supports |
Checked Exceptions | No, doesn’t support | Yes, supports |
Properties | Yes, supports | Yes, supports |
Functional Programming | Yes, supports | Yes, supports |
Operator Overloading | Yes, supports | No, doesn’t support |
Primitive Data Types | Yes, supports | Yes, supports |
Syntax | Concise and expressive syntax | Verbose syntax |
Compiler | Faster compilation time | Slower compilation time |
Community Support | Rapidly growing community | Mature and extensive community support |
15. What is an extension function in Kotlin?
Answer: An extension function in Kotlin is a function that can be added to an existing class without modifying its source code.
16. What is the difference between a class and an object in Kotlin?
- A class in Kotlin is a blueprint for creating objects, while an object is a singleton instance of a class.
- A class can have multiple instances, while an object can only have one instance.
17. What is a sealed class in Kotlin?
Answer: A sealed class in Kotlin is a class that can have a fixed set of subclasses. It is often used to represent a restricted hierarchy of classes.
18. How does Kotlin work on Android?
Kotlin shares many similarities with the Java programming language. Like Java, Kotlin code is compiled into Java bytecode and executed by the Java Virtual Machine (JVM). When a Kotlin file, such as Main.kt, is compiled, it generates a class and bytecode file named MainKt.class, which is then executed by the JVM at runtime.
19. What is a companion object in Kotlin?
Answer: A companion object in Kotlin is an object that is associated with a class and can be accessed using the class name. It is often used to provide static methods and properties in Kotlin.
20. How many types of constructors are used in Kotlin?
Answer: There are two types of constructors available in Kotlin, they are:
- Primary constructor
- Secondary constructor
21. What is the syntax for creating a class with a primary constructor in Kotlin?
Example:
class Person(val name: String, var age: Int) |
In this example, a class named Person is defined with a primary constructor that takes a name parameter of type String (which is a read-only property) and an age parameter of type Int (which is a mutable property).
22. What is the use of the open keyword in Kotlin?
Answer: By default, Kotlin’s classes and functions are declared as final, which means that it is impossible to inherit from the class or override its functions. To enable this feature, the keyword “open” must be used before the class or function.
23. What is the safe call operator in Kotlin?
Answer: The safe call operator (?.) in Kotlin is used to avoid null pointer exceptions. It allows you to call a method or access a property on an object only if the object is not null.
24. What is the non-null assertion operator in Kotlin?
Answer: The non-null assertion operator (!!) in Kotlin is used to tell the compiler that a variable is not null, even if it is not explicitly declared as such. It should be used with caution, as it can lead to NullPointerExceptions if the variable is actually null.
25. What is the syntax for creating an instance of a class in Kotlin?
Example:
val person = Person(“John”, 30) |
In this example, an instance of the Person class is created with a name of “John” and an age of 30, and stored in the variable person.
26. What is the difference between a higher-order function and a regular function in Kotlin?
- A higher-order function in Kotlin is a function that takes one or more functions as parameters or returns a function as a result.
- A regular function in Kotlin does not take functions as parameters or return functions as a result.
27. Why is Kotlin interoperable with Java?
Answer: Kotlin offers interoperability with Java since it leverages JVM bytecode, which enables direct compilation to bytecode. As a result, compile-time is improved and there is no discernible difference between Java and Kotlin for JVM.
28. What are some features which are available in Kotlin but not in Java?
Answer: Below are some Kotlin features that are not available in Java:
- Null Safety
- Operator Overloading
- Coroutines
- Range expressions
- Smart casts
- Companion Objects
29. How can we convert a Kotlin source file to a Java source file?
Answer: To convert a Kotlin source file to a Java source file, follow these steps:
- Open your Kotlin project in IntelliJ IDEA / Android Studio.
- Navigate to Tools > Kotlin > Show Kotlin Bytecode.
- Click on the Decompile button to obtain the Java code from the bytecode.
30. What is the difference between a top-level function and a member function in Kotlin?
- A top-level function in Kotlin is a function that is defined outside of a class, while a member function is a function that is defined within a class.
- Top-level functions can be accessed from anywhere in the code, while member functions can only be accessed through an instance of the class.
31. What is the syntax for defining a function with a default parameter value in Kotlin?
Example:
funCopy code for (i in 1..count) { println(message) } }“` |
In this example, a function named `printMessage` is defined with a default value of 1 for the `count` parameter.
32. What is the difference between an abstract class and an interface in Kotlin?
Answer: An abstract class in Kotlin is a class that cannot be instantiated and may contain both abstract and non-abstract methods, while an interface is a collection of abstract methods and properties that can be implemented by a class.
33. What kinds of programming types does Kotlin support?
Kotlin supports the following programming types:
- Procedural Programming
- Object-Oriented Programming
34. What is the difference between an open and a final class in Kotlin?
Answer: An open class in Kotlin can be inherited from and its methods can be overridden by subclasses, while a final class cannot be inherited from or overridden.
35. What is the difference between a public and an internal visibility modifier in Kotlin?
Answer: A public visibility modifier in Kotlin means that the class or function can be accessed from anywhere in the code, while an internal visibility modifier means that the class or function can only be accessed within the same module.
36. What is the difference between a companion object and an object in Kotlin?
- A companion object in Kotlin is an object that is tied to a class and can access its private members.
- An object in Kotlin is a singleton instance of a class that is not tied to a specific class.
37. What is the difference between a lateinit property and an observable property in Kotlin?
- A lateinit property in Kotlin is a property that is not initialized at declaration time but is initialized later before being accessed.
- An observable property in Kotlin is a property that allows you to observe changes to its value.
38. What is the difference between a tail-recursive function and a non-tail-recursive function in Kotlin?
- A tail-recursive function in Kotlin is a function that calls itself at the end of the function body and is optimized by the compiler to avoid stack overflow.
- A non-tail-recursive function in Kotlin is a function that calls itself within the function body and can cause stack overflow.
39. What is the syntax for creating an enum class in Kotlin?
Example:
“`enum class Color { RED, GREEN, BLUE }“` |
In this example, an enum class named `Color` is defined with three possible values: `RED`, `GREEN`, and `BLUE`.
40. What are the names of some extension methods that Kotlin provides to java.io.File?
Answer: Kotlin provides several extension methods to java.io.File, including:
- bufferedReader(): Reads the contents of a file into a BufferedReader.
- readBytes(): Reads the contents of a file to a ByteArray.
- readText(): Reads the contents of a file to a single String.
- forEachLine(): Reads a file line by line in Kotlin.
- readLines(): Reads lines in the file to a List.
41. What is the difference between a generic class and a generic function in Kotlin?
- A generic class in Kotlin is a class that can be instantiated with different types of arguments.
- A generic function in Kotlin is a function that can be called with different types of arguments.
42. What is the difference between a lateinit and a nullable property in Kotlin?
Answer: A lateinit property in Kotlin is a property that is declared without an initial value and is initialized later, while a nullable property is a property that can have a null value from the start.
43. What is the syntax for declaring a variable as a constant in Kotlin?
Example:
“`val PI = 3.14159“` |
In this example, a variable named `PI` is declared as a constant using the `val` keyword.
44. What is the difference between a property and a field in Kotlin?
Answer: A property in Kotlin is a combination of a getter and/or a setter method, while a field is a variable that holds the actual value of the property.
45. What is the difference between an open class and a final class in Kotlin?
- An open class in Kotlin is a class that can be subclassed, while a final class is a class that cannot be subclassed.
- By default, classes in Kotlin are final and must be explicitly marked as open to allow subclassing.
46. What is the difference between a lambda expression and an anonymous function in Kotlin?
- A lambda expression in Kotlin is a function literal that can be passed as an argument or returned from a function.
- An anonymous function in Kotlin is a function literal that can be used as an expression or assigned to a variable.
47. What is the difference between a lazy property and a lateinit property in Kotlin?
- A lazy property in Kotlin is a property that is initialized only when it is first accessed.
- A lateinit property in Kotlin is a property that is not initialized at declaration time but is initialized later before being accessed.
48. What is the difference between a collection and an array in Kotlin?
Answer: A collection in Kotlin is a generic interface that represents a group of objects, while an array is a fixed-size collection of objects that are all of the same type.
49. What is the syntax for creating an extension function in Kotlin?
Example:
“`fun String.printWithExclamation() { println(“$this!”) }“` |
In this example, an extension function named `printWithExclamation` is defined for the `String` class.
50. What is a coroutine in Kotlin?
Answer: A coroutine in Kotlin is a lightweight thread that can suspend execution without blocking the calling thread. It is used to perform asynchronous operations.
51. What is the difference between suspend and async in Kotlin?
Answer: The suspend keyword in Kotlin is used to mark a function that can be suspended and resumed later, while the async keyword is used to launch a coroutine that returns a Deferred object.
52. What is the difference between a nullable type and a non-nullable type in Kotlin?
- A nullable type in Kotlin is a type that can hold a null value.
- A non-nullable type in Kotlin is a type that cannot hold a null value.
53. What is the difference between a public and a private visibility modifier in Kotlin?
- A public visibility modifier in Kotlin makes a member or class accessible from anywhere in the code.
- A private visibility modifier in Kotlin makes a member or class accessible only from within the same file or class.
54. What is the difference between a function and a method in Kotlin?
- A function in Kotlin is a standalone piece of code that performs a task and can be called from anywhere in the code.
- A method in Kotlin is a function that is associated with a class or object and can only be called through an instance of the class or object.
55. What is the difference between a smart cast and a safe cast in Kotlin?
- A smart cast in Kotlin is a type cast that is automatically performed by the compiler based on type checks.
- A safe cast in Kotlin is a type cast that returns null if the cast fails instead of throwing an exception.
56. What is a channel in Kotlin?
Answer: A channel in Kotlin is a way of communicating between coroutines. It is a FIFO queue that can be used to send and receive messages.
57. What is the syntax for declaring a variable as a lazy property in Kotlin?
Example:
“`val expensiveObject: ExpensiveObject by lazy { createExpensiveObject() }“` |
In this example, a lazy property named `expensiveObject` is defined that is initialized with the result of the `createExpensiveObject()` function the first time it is accessed.
58. What is the difference between launch and runBlocking in Kotlin?
Answer: The launch function in Kotlin is used to launch a coroutine in a non-blocking way, while the runBlocking function is used to block the calling thread until the coroutine completes.
59. What is the difference between a nullable type and a non-null type in Kotlin?
Answer: A nullable type in Kotlin is a type that can have a null value, while a non-null type is a type that cannot have a null value.
60. What is the Elvis operator used for in Kotlin?
Answer: The Elvis operator in Kotlin is used to provide a default value in case a variable is null. It is represented by the ?: symbol.
61. What is the difference between a suspend function and a regular function in Kotlin?
- A suspend function in Kotlin is a function that can be paused and resumed at a later time, making it useful for asynchronous programming.
- A regular function in Kotlin is a function that runs to completion and does not support pausing and resuming.
62. What is the difference between a nullable receiver and a non-nullable receiver in Kotlin?
- A nullable receiver in Kotlin is a receiver that can hold a null value, while a non-nullable receiver is a receiver that cannot hold a null value.
- The receiver of a function or method is the object on which the function or method is called.
63. What is the difference between a single expression function and a block body function in Kotlin?
- A single expression function in Kotlin is a function that consists of a single expression and does not require a return statement.
- A block body function in Kotlin is a function that consists of a block of statements and requires a return statement.
64. What is the Safe Call operator used for in Kotlin?
Answer: The Safe Call operator in Kotlin is used to safely call a method or access a property of an object that may be null. It is represented by the ?. symbol.
65. What is the difference between a lambda and an anonymous function in Kotlin?
Answer: A lambda in Kotlin is a function that can be passed around as a value, while an anonymous function is a function that is not named and is defined inline.
66. What is the let function used for in Kotlin?
Answer: The let function in Kotlin is used to perform a series of operations on a variable and return a value. It is often used in combination with the Safe Call operator to handle null values.
67. What is the apply function used for in Kotlin?
Answer: The apply function in Kotlin is used to initialize an object and return the object itself. It is often used to simplify object initialization code.
68. What is the with function used for in Kotlin?
Answer: The with function in Kotlin is used to perform a series of operations on an object without having to repeat the object name each time. It is often used to simplify code that operates on a single object.
69. What is the difference between a sealed class and an abstract class in Kotlin?
Answer: A sealed class in Kotlin is a class that can have a limited number of subclasses, while an abstract class is a class that cannot be instantiated and must be subclassed.
70. What is the difference between a private constructor and a public constructor in Kotlin?
- A private constructor in Kotlin is a constructor that can only be called from within the same class or object.
- A public constructor in Kotlin is a constructor that can be called from anywhere in the code.
71. What is the difference between a primary constructor and a secondary constructor in Kotlin?
- A primary constructor in Kotlin is the constructor that is defined in the class header and is used to initialize the properties of the class.
- A secondary constructor in Kotlin is a constructor that is defined within the body of the class and can have different parameters than the primary constructor.
72. What is the difference between the rangeTo operator (..) and the downTo operator in Kotlin?
- The rangeTo operator (..) in Kotlin is used to create a range of values from a starting value to an ending value.
- The downTo operator in Kotlin is used to create a range of values from a starting value to an ending value in descending order.
73. What is the difference between a sealed class and an enum class in Kotlin?
- A sealed class in Kotlin is a class that restricts the types of its subclasses and can be used for exhaustive when expressions.
- An enum class in Kotlin is a class that represents a set of values that are named constants.
74. What is the difference between a data class and a regular class in Kotlin?
Answer: A data class in Kotlin is a class that is designed to hold data, while a regular class can hold any type of functionality.
75. What is the difference between a property getter and a property setter in Kotlin?
Answer: A property getter in Kotlin is a method that is used to retrieve the value of a property, while a property setter is a method that is used to set the value of a property.
76. What is the difference between a companion object and a static class in Java?
Answer: A companion object in Kotlin is an object that is associated with a class and can access its private members, while a static class in Java is a class that cannot access private members of another class.
77. What is the difference between a companion object and a regular object in Kotlin?
- A companion object in Kotlin is an object that is associated with a class and can be used to store static members of the class.
- A regular object in Kotlin is an object that is not associated with a class and can be used to store standalone functionality.
78. What is the difference between an infix function and a regular function in Kotlin?
- An infix function in Kotlin is a function that is called using infix notation, which means the function name is placed between the two operands.
- A regular function in Kotlin is a function that is called using the usual function call syntax.
79. What is the difference between an extension function and a member function in Kotlin?
- An extension function in Kotlin is a function that is defined outside of a class but can be called as if it were a member function of the class.
- A member function in Kotlin is a function that is defined within a class and can only be called on an instance of the class.
80. What is the difference between an extension function and a regular function in Kotlin?
Answer: An extension function in Kotlin is a function that can be added to an existing class without modifying its source code, while a regular function must be defined within its own class.
81. What is the difference between a lambda and a closure in Kotlin?
Answer: A lambda in Kotlin is a function that can be passed around as a value and does not retain any state, while a closure is a function that retains state from the environment in which it was created.
82. What is the difference between a default argument and a named argument in Kotlin?
Answer: A default argument in Kotlin is a function argument that has a default value and can be omitted in function calls, while a named argument is a function argument that is specified by name instead of position.
83. What is the difference between a private and a protected visibility modifier in Kotlin?
Answer: A private visibility modifier in Kotlin means that the class or function can only be accessed within the same file, while a protected visibility modifier means that the class or function can be accessed within the same file or within subclasses.
84. What is the difference between a lateinit property and a lazy property in Kotlin?
Answer: A lateinit property in Kotlin is a property that is initialized later, while a lazy property is a property that is initialized lazily on the first access.
85. What is the difference between a synchronized block and a mutex in Kotlin?
Answer: A synchronized block in Kotlin is a block of code that is executed by only one thread at a time, while a mutex is a synchronization primitive that is used to ensure that only one thread can access a resource at a time.
86. What is the difference between an object declaration and a companion object in Kotlin?
Answer: An object declaration in Kotlin is a way to create a singleton object, while a companion object is an object that is associated with a class and can access its private members.
87. What is the difference between a reified type parameter and a regular type parameter in Kotlin?
Answer: A reified type parameter in Kotlin is a type parameter that can be used at runtime, while a regular type parameter is erased at runtime and cannot be used in reflection.
88. What is the difference between a tail-recursive function and a regular recursive function in Kotlin?
Answer: A tail-recursive function in Kotlin is a function that is optimized to avoid stack overflow, while a regular recursive function may cause stack overflow when called too many times.
89. What is the difference between a safe call operator and a non-null assertion operator in Kotlin?
Answer: A safe call operator in Kotlin (?.) returns null if the object is null, while a non-null assertion operator (!!) throws an exception if the object is null.
90. What is the difference between a lateinit property and a nullable property in Kotlin?
Answer: A lateinit property in Kotlin must be initialized before it is accessed, while a nullable property can be accessed without being initialized and may return null.
91. What is the difference between a local function and a nested function in Kotlin?
Answer: A local function in Kotlin is a function that is defined within another function, while a nested function is a function that is defined within a class or object.
92. What is the difference between a tailwind and a jet stream in Kotlin?
Answer: There is no difference between tailwind and jet stream in Kotlin. These terms are not related to the Kotlin programming language.
93. What is the difference between a when expression and a switch statement in Java?
Answer: A when expression in Kotlin is a more powerful version of a switch statement in Java, as it can handle complex conditions and return values.
94. What is the difference between a suspend function and a coroutine in Kotlin?
Answer: A suspend function in Kotlin is a function that can be suspended and resumed later, while a coroutine is a higher-level abstraction that can be used to write asynchronous code in a more structured and readable way.
95. What are the structural expressions in kotlin?
Answer: There are three important structural expressions in Kotlin, which are:
- Break: This expression allows the closest enclosing loop to be exited.
- Return: This expression enables returning from the closest function or default function.
- Continue: This expression allows for the next loop iteration to proceed.
96. What is init block?
Answer: The “init” block is a login block that is executed in the primary constructor to initialize the object. If needed, it can be overridden in the secondary constructor, but it will only be executed after the primary constructor in a chain form.
97. What are the class members in kotlin?
Answer: Below are the class members in Kotlin:
- Initializer blocks
- Properties
- Open declarations
- Nested classes
- Inner classes
98. What does ‘Null Safety’ mean in Kotlin?
Answer: The Null Safety feature in Kotlin helps to eliminate the risk of encountering a NullPointerException during runtime. Additionally, it enables differentiation between nullable references and non-nullable references, providing an added layer of safety.
99. Does Kotlin allow macros?
Answer: Kotlin does not support macros as the language’s developers have found it challenging to incorporate them into the language.
100. How can you handle null exceptions in Kotlin?
Answer: The Elvis Operator in Kotlin is utilized to handle null expectations.
The Top 100 Kotlin Interview Questions and Answers provide a comprehensive guide for candidates to prepare and excel in Kotlin programming interviews. Please follow us at freshersnow.com to enhance your knowledge and gain valuable insights.