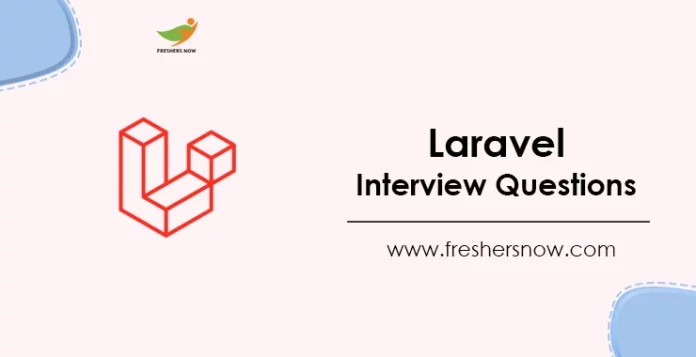
Laravel Interview Questions and Answers: Laravel is one of the most popular PHP web frameworks available today, and its popularity continues to grow rapidly. It offers a variety of features, including expressive syntax, a powerful ORM, built-in user authentication, and easy database migrations. If you are preparing for a Laravel technical interview, you may be wondering what kind of questions you can expect. This article provides the top 100 latest Laravel interview questions and answers that cover all aspects of the framework, including Laravel interview questions for freshers.
★★ Latest Technical Interview Questions ★★
Laravel Interview Questions for Freshers
These top Laravel interview questions and answers will help you prepare for your next Laravel technical interview and increase your chances of landing your dream job in web development.
Top 100 Laravel Interview Questions and Answers
Let’s delve into the top Laravel Interview Questions and Answers for Freshers, including Laravel technical interview questions and their corresponding answers. These Top Laravel Interview Questions 2023 covers a wide range of topics.
1. What is Laravel and why would you choose it over other PHP frameworks?
- Laravel is a free, open-source PHP web framework used for developing web applications.
- Laravel offers a variety of features, including an expressive syntax, a powerful ORM, built-in user authentication, and easy database migrations, making it a popular choice for developers.
- Laravel is also known for its robust documentation, active community, and ease of use.
2. How do you install Laravel?
- The easiest way to install Laravel is through Composer.
- First, you need to install Composer on your system.
- Then, run the following command in your terminal:
composer create-project --prefer-dist laravel/laravel projectname
. - This will create a new Laravel project in a directory named
projectname
.
3. What is the structure of a Laravel application?
- A Laravel application follows the Model-View-Controller (MVC) architectural pattern.
- The application’s core files are stored in the
app
directory, including controllers, models, and views. - The
routes
directory contains the application’s route definitions. - Configuration files can be found in the
config
directory, and database-related files are stored in thedatabase
directory. - Public files, such as images and stylesheets, are stored in the
public
directory.
4. What is Composer and how do you use it with Laravel?
- Composer is a package manager for PHP that allows you to manage dependencies for your project.
- Laravel relies on Composer to manage its dependencies.
- To use Composer with Laravel, you need to have Composer installed on your system.
- Then, you can add new packages to your Laravel project by running the
composer require
command.
5. How do you create a new Laravel project?
- To create a new Laravel project, you can use Composer.
- Open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel projectname
. - This will create a new Laravel project in a directory named
projectname
.
6. What is the syntax to create a new Laravel project from the command line?
The syntax to create a new Laravel project is
laravel new [project-name] |
7. What is the difference between Route::get() and Route::post()?
Route::get()
is used to define a route that responds to HTTP GET requests.Route::post()
is used to define a route that responds to HTTP POST requests.- The main difference is that GET requests are used to retrieve data from the server, while POST requests are used to submit data to the server.
8. How do you create a new route in Laravel?
- To create a new route in Laravel, you need to define it in the
routes/web.php
file. - You can use the
Route
facade to define a new route. - For example, to define a route that responds to GET requests to
/hello
, you can use the following code:Route::get('/hello', function () { return 'Hello, world!'; });
.
9. What is the difference between a Model and a Controller in Laravel?
Model | Controller |
---|---|
Represents a specific data entity in the application, usually maps to a database table or collection. | Handles user requests, interacts with models and views to process and respond to user input. |
Defines the structure of the data, provides methods to interact with the data, and includes business logic for the data. | Acts as an intermediary between the model and the view, and contains the application logic that handles the flow of data between the two. |
Typically contains methods for creating, reading, updating, and deleting records in the database. | Contains methods that are triggered by user actions, such as submitting a form or clicking a link. |
Can define relationships with other models in the application. | Does not define relationships, but may interact with multiple models to fulfill a single request. |
Can include validation rules and other business logic to ensure the integrity and accuracy of the data. | Can include middleware and other logic to handle authentication, authorization, and other cross-cutting concerns. |
Can be used to create and execute complex database queries. | Does not interact directly with the database, but may use Eloquent ORM or the Query Builder to retrieve data from the model. |
10. How do you create a new controller in Laravel?
- To create a new controller in Laravel, you can use the
make:controller
Artisan command. - Open your terminal and run the following command:
php artisan make:controller MyController
. - This will create a new controller named
MyController
in theapp/Http/Controllers
directory.
11. How do you define a route that accepts parameters in Laravel?
You can define a route that accepts parameters in Laravel by using curly braces {} to indicate the parameter name, like so: Route::get(‘/users/{id}’, function ($id) { return “User ID: ” . $id; });
12. What is a middleware in Laravel and how do you create one?
- A middleware is a piece of code that is executed before or after a request is handled by the application.
- Middleware can be used to perform tasks such as authentication, logging, and input validation.
- To create a new middleware in Laravel, you can use the
make:middleware
Artisan command. - Open your terminal and run the following command:
php artisan make:middleware MyMiddleware
. - This will create a new middleware named
MyMiddleware
in theapp/Http/Middleware
directory.
13. How do you create a new view in Laravel?
- To create a new view in Laravel, you need to create a new Blade template file in the
resources/views
directory. - For example, to create a new view named
welcome.blade.php
, you can create a new file in theresources/views
directory with the following code:<!DOCTYPE html>
<html>
<head>
<title>Welcome</title>
</head>
<body>
<h1>Welcome to my website!</h1>
</body>
</html> - You can then use the
view()
function in your controller to load the view. For example, to load thewelcome.blade.php
view, you can use the following code in your controller:return view('welcome');
.
14. What is Blade and how do you use it in Laravel?
- Blade is Laravel’s templating engine that enables developers to create views more efficiently and securely.
- Blade files use the
.blade.php
extension and include a variety of directives for rendering dynamic content. - Blade directives are enclosed in
@
symbols, such as@if
,@foreach
, and@extends
. - Blade also supports creating reusable template parts with
@include
and@yield
.
15. What is the syntax for defining a relationship between two Eloquent models in Laravel?
The syntax for defining a relationship between two Eloquent models in Laravel is to use the methods “belongsTo” and “hasMany”, like so:
class User extends Model { public function posts() { return $this->hasMany(Post::class); } }class Post extends Model { public function user() { return $this->belongsTo(User::class); } } |
16. What are Eloquent models in Laravel and how do you create one?
- Eloquent is Laravel’s ORM (Object Relational Mapping) system that provides a simple and elegant way to work with databases.
- Eloquent models are PHP classes that represent database tables and define their relationships.
- To create a new Eloquent model in Laravel, you can use the
make:model
Artisan command or manually create a new PHP file in theapp/Models
directory that extends theIlluminate\Database\Eloquent\Model
class.
17. How does Laravel’s “Migrations” feature differ from traditional database schema management methods?
Laravel Migrations | Traditional Database Schema Management |
---|---|
Uses a declarative syntax to define the structure of the database schema in code. | Requires manually creating and updating the schema using SQL scripts or other database management tools. |
Allows for version control of the database schema, so that changes can be tracked and rolled back if necessary. | May not have version control, making it difficult to track changes or revert to previous versions. |
Provides a consistent and repeatable way to manage database schema changes across different environments, such as development, staging, and production. | May require manual intervention to ensure that schema changes are applied correctly in each environment. |
Supports the creation of new database tables, columns, indexes, and other schema elements, as well as the modification and deletion of existing ones. | Typically requires manual SQL scripting to make changes to the schema, which can be error-prone and time-consuming. |
Provides a way to seed the database with sample data using PHP code, making it easy to populate a new database with initial data. | Requires manual insertion of data using SQL scripts or other database management tools, which can be time-consuming and error-prone. |
Integrates seamlessly with Laravel’s Eloquent ORM, allowing for easy management of relationships between tables and objects in the application code. | May require manual management of relationships between tables in the database, which can be difficult to maintain over time. |
18. What is a migration in Laravel and how do you create one?
- A migration in Laravel is a way to version control changes to the database schema.
- Migrations are stored as PHP files in the
database/migrations
directory and contain aup
method for making changes to the database and adown
method for rolling back those changes. - To create a new migration in Laravel, you can use the
make:migration
Artisan command followed by a descriptive name for the migration.
19. How do you seed a database in Laravel?
- Database seeding in Laravel is a way to quickly populate a database with sample data.
- Seeders are stored in the
database/seeders
directory and typically use Eloquent models to create new records. - To create a new seeder in Laravel, you can use the
make:seeder
Artisan command followed by a descriptive name for the seeder. - To run a seeder in Laravel, you can use the
db:seed
Artisan command.
20. What is a factory in Laravel and how do you create one?
- A factory in Laravel is a way to generate fake data for testing or seeding purposes.
- Factories are stored in the
database/factories
directory and define the data structure for generating fake records. - To create a new factory in Laravel, you can use the
make:factory
Artisan command followed by the name of the model you want to generate fake data for.
21. What is the syntax for querying the database using Laravel’s Query Builder?
The syntax for querying the database using Laravel’s Query Builder is as follows:
DB::table(‘table_name’) ->select(‘column_name’) ->where(‘column_name’, ‘=’, ‘value’) ->get(); |
22. What is the difference between soft deletes and hard deletes in Laravel?
- Soft deletes in Laravel allow developers to mark records as deleted without actually removing them from the database.
- Soft deletes use a
deleted_at
column on the database table to mark a record as deleted. - Hard deletes, on the other hand, completely remove records from the database.
- To enable soft deletes for an Eloquent model in Laravel, you can add the
Illuminate\Database\Eloquent\SoftDeletes
trait to the model class and add adeleted_at
column to the database table.
23. How do you create a validation rule in Laravel?
- Laravel provides a variety of built-in validation rules, such as
required
,email
, andmax
. - To create a custom validation rule in Laravel, you can use the
make:rule
Artisan command to create a new PHP class that defines the validation logic. - The validation rule class should implement the
Illuminate\Contracts\Validation\Rule
interface and define apasses
method that returnstrue
orfalse
depending on whether the validation passes.
24. What are contracts?
- The Laravel Contracts comprise a collection of interfaces containing implementation methods that handle the essential functions of Laravel.
- For instance, the Queue and Mailer are some examples of the Laravel Contracts. The Queue contract incorporates an implementation for queuing jobs, while the Mailer contract has an implementation for sending emails.
25. What is CSRF protection and how does Laravel handle it?
- CSRF (Cross-Site Request Forgery) is an attack that tricks a user into performing an action on a website without their knowledge or consent.
- Laravel provides built-in CSRF protection by generating a CSRF token for each session and verifying it on each POST, PUT, or DELETE request.
- To use the CSRF protection, you can include the csrf_token() function in your forms or AJAX requests.
- Laravel automatically includes the CSRF token in forms created using the form helper and in AJAX requests made using the axios library.
26. How does Laravel’s “Event” system differ from traditional observer patterns?
Laravel Events | Traditional Observer Patterns |
---|---|
Allows for decoupling of application components, as events can be broadcast without knowing which listeners will handle them. | May require tight coupling between observable and observer components, as the observable needs to know about its observers. |
Provides a unified way of handling and triggering events across different parts of the application. | May require separate implementation of the observer pattern in different parts of the application, leading to code duplication and inconsistency. |
Supports multiple listeners for a single event, making it easy to add or remove functionality without modifying the code that triggers the event. | Typically requires modifying the observable component to add or remove observers, which can be time-consuming and error-prone. |
Supports prioritization of listeners, so that certain listeners can handle an event before or after others. | Typically does not provide built-in support for prioritization of observers. |
Can be used to handle both synchronous and asynchronous events, making it easy to perform long-running tasks without blocking the application. | May not have built-in support for asynchronous processing, requiring additional coding and configuration. |
Integrates seamlessly with Laravel’s Service Container, allowing for easy management of dependencies between event listeners and other application components. | May require manual management of dependencies between observable and observer components, which can be difficult to maintain over time. |
27. How do you define a new migration in Laravel?
You can define a new migration in Laravel by using the “make:migration” Artisan command, like so: “php artisan make:migration create_users_table”.
28. How do you use the query builder in Laravel?
- The query builder is a powerful feature of Laravel that allows you to build SQL queries programmatically.
- To use the query builder, you can use the DB facade and chain methods to build your query. For example, DB::table(‘users’)->where(‘name’, ‘John’)->get() will retrieve all users where the name is “John”.
- The query builder supports many methods for constructing queries, such as select(), where(), join(), orderBy(), groupBy(), and having().
- You can also use raw SQL expressions and subqueries in your queries using the DB::raw() method.
- Once you have built your query, you can execute it using methods such as get(), first(), or value().
29. What is a collection in Laravel and how do you use it?
- A collection in Laravel is an object-oriented wrapper around arrays, providing a set of methods for manipulating and filtering the data.
- To use a collection, you can create it using the
collect()
function or by calling thecollect
method on an existing array, and then chain methods likemap()
,filter()
, andreduce()
to transform and manipulate the data.
30. What is the syntax for creating a new controller in Laravel?
The syntax for creating a new controller in Laravel is
php artisan make:controller [ControllerName] |
31. How do you create a custom artisan command in Laravel?
- To create a custom artisan command in Laravel, you can use the
make:command
Artisan command to generate a new command class. - Once you have the command class, you can define its behavior by implementing the
handle()
method, and register it with the Artisan command scheduler by adding it to the$commands
array in theapp/Console/Kernel.php
file.
32. What is a service container in Laravel and how do you use it?
- A service container in Laravel is a container that is responsible for managing and resolving dependencies within your application.
- To use the service container, you can bind dependencies to the container using the
bind()
method, and then use themake()
method to resolve them when needed.
33. What is the difference between a Route and a Middleware in Laravel?
Feature | Route | Middleware |
---|---|---|
Definition | A route is a URL that specifies an action to be taken. | Middleware is a way to filter HTTP requests entering your Laravel application. |
Execution | Executes the specified action for the matching URL. | Executes the specified operation on an HTTP request before it reaches the controller. |
Parameters | Receives a URL and maps it to a controller action. | Receives an HTTP request and can modify it before it is sent to a controller action. |
Structure | Routes are defined in the routes/web.php file. | Middleware is defined in the app/Http/Kernel.php file. |
Chaining | Multiple routes can be chained together to create complex actions. | Multiple middleware can be chained together to create complex operations on an HTTP request. |
Examples | Route::get(‘/users’, ‘UserController@index’); | Route::middleware([‘auth’])->group(function () { //… }); |
Route::post(‘/users’, ‘UserController@store’); | public function handle($request, $next) { //… } |
34. How do you use the dependency injection container in Laravel?
- The dependency injection container in Laravel is used to automatically inject dependencies into a class’s constructor or methods.
- To use dependency injection in Laravel, you can type-hint the dependencies in your class’s constructor or methods, and Laravel will automatically resolve and inject them for you.
35. What is a facade in Laravel and how do you use it?
- A facade in Laravel is a class that provides a static interface to an underlying class or service in your application.
- To use a facade, you can call its static methods directly, without having to instantiate the underlying class or service.
36. How do you define a named route in Laravel?
You can define a named route in Laravel by using the “name” method on the route, like so:
Route::get(‘/user/{id}’, function ($id) { // })->name(‘profile’); |
37. What are facades?
- Facades provide a method for registering a class and its methods in the Laravel Container, making them accessible throughout the application after being resolved by Reflection.
- Using facades eliminates the need to recall lengthy class names or import them into other classes for use, which improves the application’s testability.
- The following image illustrates why facades are employed:
38. What is the difference between a repository and a service in Laravel?
- In Laravel, a repository is a class that provides a way to abstract and encapsulate data access logic, while a service is a class that provides a specific set of functionality to your application.
- The main difference between the two is that a repository is typically used to access and manipulate data, while a service is used to provide more complex business logic.
39. What is the difference between a “ServiceProvider” and a “Facade” in Laravel?
ServiceProvider | Facade |
---|---|
Responsible for binding classes and interfaces into the service container. | A convenient way to use classes available from the application’s service container. |
It defines a set of instructions for bootstrapping the application services. | Acts as a static proxy to the underlying service in the service container. |
It provides various services such as registering, booting, publishing configuration files, etc. | Facades make it easy to call a class method without requiring an instance of the class. |
Service Providers help developers organize application services and keep the code structured. | Facades provide a simple interface to the more complex code under the hood. |
Laravel’s core functionality can be extended using Service Providers. | Facades can be used to make Laravel’s built-in services more convenient to use. |
40. How do you use the event system in Laravel?
- The event system in Laravel allows you to decouple different parts of your application by allowing them to communicate with each other using events and listeners.
- To use the event system, you can define events using the
Event
facade, and then register listeners to handle those events using thelisten()
method.
41. What is the syntax for including a Blade template in a Laravel view?
The syntax for including a Blade template in a Laravel view is
@include(‘path.to.template’) |
42. What is a job in Laravel and how do you create one?
- A job in Laravel is a class that represents a task that can be executed asynchronously in the background.
- To create a job, you can use the
make:job
Artisan command to generate a new job class, and then define its behavior by implementing thehandle()
method.
43. How do you use queues in Laravel?
- Queues in Laravel allow you to defer the processing of time-consuming tasks to a later time, improving the responsiveness and scalability of your application.
- To use queues, you can define jobs using the
make:job
Artisan command, and then dispatch those jobs to a queue using the `dispatch
44. What is a task scheduler in Laravel and how do you use it?
- A task scheduler in Laravel allows you to schedule repetitive tasks to run automatically.
- You can use the task scheduler by defining the tasks you want to run in the
App\Console\Kernel
class, which can be found in theapp/Console
directory. - You can define a task by using the
schedule
method, which takes a Closure or an instance of a command. - You can then use the
cron
method to define the frequency at which the task should run.
45. How do you define a middleware in Laravel?
You can define a middleware in Laravel by using the “make:middleware” Artisan command, like so: “php artisan make:middleware [MiddlewareName]”.
46. What is a service provider in Laravel and how do you create one?
- A service provider in Laravel is a class that binds a service to the Laravel container, which makes the service available throughout your application.
- You can create a service provider by using the
make:provider
Artisan command, which generates a new class file in theapp/Providers
directory. - The service provider class must implement the
Illuminate\Support\ServiceProvider
interface, and you can use theregister
method to bind the service to the container.
47. What is the difference between a service provider and a facade in Laravel?
- A service provider is responsible for registering a service with the Laravel container, while a facade provides a static interface to the service.
- Service providers are used to bind classes or interfaces to the container, while facades provide a more convenient way to access those services.
- Service providers are registered in the
config/app.php
file, while facades are registered in thealiases
array of the same file.
48. What is the difference between Laravel’s “Validation” system and other validation libraries?h(Post::class, User::
Criteria | Laravel’s Validation | Other Validation Libraries |
---|---|---|
Framework support | Native support | Requires integration with framework |
Ease of use | Easy to use and understand, with built-in rules and custom validation options | May require more configuration and code |
Error messages | Customizable error messages and localization support | Customizable, but may require more work to set up |
Security | Built-in protection against common attacks, such as SQL injection | May require additional measures to protect against attacks |
Dependency on models | Can directly reference models and their properties | May require additional configuration to integrate with models and databases |
Maintenance | Part of Laravel framework, so updates and maintenance are handled by Laravel team | Maintenance may be required by third-party library developers or the development team |
49. How do you create a new middleware group in Laravel?
- You can create a new middleware group by adding it to the
$middlewareGroups
property in theApp\Http\Kernel
class, which can be found in theapp/Http
directory. - You can define the middleware group by providing an array of middleware classes or middleware keys, which are defined in the
$routeMiddleware
property.
50. How do you create a custom validation message in Laravel?
- You can create a custom validation message by adding a
messages
array to the validation rule in your controller or form request class. - The
messages
array should contain a key for each rule you want to customize, with the value being the custom message you want to display.
51. What is a view composer in Laravel and how do you use it?
- A view composer in Laravel is a callback function that is registered to run when a view is being rendered.
- You can use a view composer by defining a closure or a class method in a service provider’s
boot
method, using theView::composer
method. - The closure or method should take a view object as a parameter, which can be used to modify the view before it is rendered.
52. What is the syntax for defining a new Eloquent model in Laravel?
The syntax for defining a new Eloquent model in Laravel is as follows:
use Illuminate\Database\Eloquent\Model; class User extends Model |
53. How do you create a new helper function in Laravel?
- You can create a new helper function by defining it in the
bootstrap/helpers.php
file, which is loaded automatically by Laravel. - The function should be defined as a global function and should follow the
snake_case
naming convention. - After defining the function, you should run the
composer dump-autoload
command to regenerate the autoloader.
54. What is the difference between the “hasOne” and “belongsTo” relationship in Laravel’s Eloquent ORM?
hasOne Relationship | belongsTo Relationship | |
---|---|---|
1 | Defines a one-to-one relationship, where the foreign key exists on the child model. | Defines a one-to-one or one-to-many relationship, where the foreign key exists on the parent model. |
2 | The child model contains the foreign key that references the parent model’s primary key. | The parent model contains the foreign key that references the child model’s primary key. |
3 | The “hasOne” method is used on the child model to define the relationship. | The “belongsTo” method is used on the child model to define the relationship. |
4 | Example: A user has one profile. The “users” table has a primary key “id”, and the “profiles” table has a foreign key “user_id”. | Example: A profile belongs to a user. The “profiles” table has a foreign key “user_id”, and the “users” table has a primary key “id”. |
5 | Syntax: $this->hasOne(RelatedModel::class, 'foreign_key'); | Syntax: $this->belongsTo(RelatedModel::class, 'foreign_key'); |
55. How do you use the debugbar package in Laravel?
- You can use the debugbar package in Laravel by installing it via Composer and adding it to your
config/app.php
file in theproviders
array. - Once the package is installed and registered, you can use the
Debugbar
facade to access the debugbar object and start logging debug information. - The debugbar will automatically collect information about the request and response, including database queries, execution time, and more.
56. What is a pivot table in Laravel and how do you use it?
- A pivot table in Laravel is a table that is used to represent a many-to-many relationship between two other tables.
- You can use a pivot table by defining the relationship between the two tables in the corresponding model classes, and then defining a new method for the relationship in each model.
- The pivot table should have two foreign keys, each referencing the primary key of the related tables.
57. How do you create a new migration with a foreign key in Laravel?
- You can create a new migration with a foreign key in Laravel by using the
make:migration
Artisan command, followed by a name for the migration and the--table
option to specify the name of the table you want to modify. - You can then use the
table
method on theSchema
facade to define the columns for the new table, including the foreign key column. - The foreign key column should be defined using the
foreign
method, which takes the name of the related table and the name of the related column as arguments.
58. How do you use the many-to-many relationship in Laravel?
- Many-to-many relationships in Laravel can be defined by creating a new migration to create a pivot table that holds the foreign keys of the two related models.
- Then, in the model files of the related models, you can define the many-to-many relationship by using the
belongsToMany()
method. - For example, if you have a
User
model and aRole
model, you can define the many-to-many relationship between them by adding the following code to both models:// User model
public function roles()
{
return $this->belongsToMany(Role::class);
}// Role model
public function users()
{
return $this->belongsToMany(User::class);
}
59. How do you use the one-to-many relationship in Laravel?
- One-to-many relationships in Laravel can be defined by adding a foreign key to the related model’s table that references the primary key of the other model’s table.
- Then, in the model file of the related model, you can define the one-to-many relationship by using the
hasMany()
method. - For example, if you have a
Post
model and aComment
model, where each post can have many comments, you can define the one-to-many relationship between them by adding the following code to thePost
model:public function comments()
{
return $this->hasMany(Comment::class);
}
60. How do you use the one-to-one relationship in Laravel?
- One-to-one relationships in Laravel can be defined by adding a foreign key to one of the related model’s tables that references the primary key of the other model’s table.
- Then, in the model file of the related model, you can define the one-to-one relationship by using the
hasOne()
orbelongsTo()
method, depending on which model has the foreign key. - For example, if you have a
User
model and aProfile
model, where each user has one profile, you can define the one-to-one relationship between them by adding the following code to theUser
model:public function profile()
{
return $this->hasOne(Profile::class);
}
61. How do you use the belongs-to relationship in Laravel?
- The belongs-to relationship in Laravel is used to define a relationship where one model belongs to another model.
- This is usually done by adding a foreign key to the model’s table that references the primary key of the related model’s table.
- In the model file of the related model, you can define the belongs-to relationship by using the
belongsTo()
method. - For example, if you have a
Comment
model that belongs to aPost
model, you can define the belongs-to relationship by adding the following code to theComment
model:public function post()
{
return $this->belongsTo(Post::class);
}
62. How does Laravel’s “Query Builder” differ from using raw SQL queries?
Query Builder | Raw SQL Queries | |
---|---|---|
1 | Object-oriented syntax that allows for more readable and maintainable code. | Requires a good knowledge of SQL syntax and can lead to complex and unreadable code. |
2 | Provides a high-level abstraction of SQL queries, making it easier to work with multiple database systems. | SQL queries need to be modified for different database systems. |
3 | Provides protection against SQL injection attacks through parameter binding. | Requires manual sanitization of user input to prevent SQL injection attacks. |
4 | Allows for easier manipulation of query results through collection methods. | Requires additional code to manipulate query results. |
5 | Supports building complex queries with query chaining and query scopes. | Requires writing complex SQL queries for complex scenarios. |
6 | Supports pagination, ordering, and filtering of query results. | Requires additional code to implement pagination, ordering, and filtering. |
7 | Provides easier integration with Laravel’s Eloquent ORM. | Requires additional code to integrate with an ORM or database abstraction layer. |
8 | Syntax: $users = DB::table('users')->where('name', 'John')->get(); | Syntax: $users = DB::select('SELECT * FROM users WHERE name = ?', ['John']); |
63. How do you use the has-many-through relationship in Laravel?
- The has-many-through relationship in Laravel is used to define a relationship where a model has many other models through a third intermediate model.
- To define this relationship, you need to add foreign keys to both the intermediate model’s table and the related model’s table.
- In the model file of the original model, you can define the has-many-through relationship by using the
hasManyThrough()
method.
64. What is the difference between the where() and find() methods in Laravel?
- The where() method is used to retrieve a collection of records that match the given condition.
- The find() method is used to retrieve a single record by its primary key.
65. How do you use the groupBy() method in Laravel?
- The groupBy() method is used to group the results of a query by a specified column.
- To use it, we can chain the method onto a query builder instance and pass the name of the column we want to group by as an argument.
66. How do you use the selectRaw() method in Laravel?
- The selectRaw() method is used to select raw SQL expressions in a query.
- To use it, we can chain the method onto a query builder instance and pass the raw SQL expression as an argument.
67. How do you use the orderBy() method in Laravel?
- The orderBy() method is used to sort the results of a query by one or more columns.
- To use it, we can chain the method onto a query builder instance and pass the name of the column we want to sort by as an argument.
68. What is the difference between the first() and get() methods in Laravel?
- The first() method is used to retrieve the first record that matches a query.
- The get() method is used to retrieve a collection of records that match a query.
- The first() method returns a single model instance, while the get() method returns a collection of model instances.
69. How do you use the pluck() method in Laravel?
The pluck() method is used to retrieve a single column’s value from the first result of a query or a collection.
70. How does Laravel’s Blade templating engine differ from other templating engines?
Feature | Laravel Blade | Other Templating Engines |
---|---|---|
Syntax | Uses double curly braces {{ }} | Varies |
Template Inheritance | Yes, supports it | Varies |
Conditional Statements | Yes, supports it | Varies |
Loops | Yes, supports it | Varies |
Extensibility through Directives | Yes, supports it | Varies |
Precompilation of Templates | Yes, with Laravel Mix | Varies |
Template Caching | Yes, supports it | Varies |
Blade Directives | Includes various built-in directives | Varies |
Laravel-specific syntax and functionality | Yes, includes Laravel-specific tags | Varies |
Debugging | Yes, supports it | Varies |
71. How do you use the chunk() method in Laravel?
The chunk() method is used to process a large number of database records in smaller chunks to reduce memory usage.
72. How do you use the paginate() method in Laravel?
The paginate() method is used to retrieve a subset of results from a database query and display them using pagination.
73. How do you use the count() method in Laravel?
The count() method is used to retrieve the number of results returned by a database query.
74. How do you use the sum() method in Laravel?
The sum() method is used to retrieve the sum of values of a given column from a database query.
75. How do you use the max() and min() methods in Laravel?
- The max() method is used to retrieve the maximum value of a given column from a database query.
- The min() method is used to retrieve the minimum value of a given column from a database query.
76. How do you use the average() method in Laravel?
The average() method is used to retrieve the average value of a given column from a database query.
77. How do you use the join() method in Laravel?
The join() method is used to perform a SQL join between two or more database tables.
78. How do you use the whereIn() and whereNotIn() methods in Laravel?
- The whereIn() method is used to filter a database query by a set of values in a given column.
- The whereNotIn() method is used to filter a database query by values not in a given set of values.
79. How do you use the whereBetween() and whereNotBetween() methods in Laravel?
- The whereBetween() method is used to filter a database query by values within a given range.
- The whereNotBetween() method is used to filter a database query by values outside a given range.
80. How do you use the whereDate() and whereMonth() methods in Laravel?
- The whereDate() method is used to retrieve records where a specific column’s date matches a given date.
- The whereMonth() method is used to retrieve records where a specific column’s month matches a given month.
- To use either method, we can chain the method onto a query builder instance and pass the name of the column we want to compare, followed by the date or month we want to compare it to.
81. How do you use the whereYear() and whereTime() methods in Laravel?
- The whereYear() method is used to retrieve records where a specific column’s year matches a given year.
- The whereTime() method is used to retrieve records where a specific column’s time matches a given time.
- To use either method, we can chain the method onto a query builder instance and pass the name of the column we want to compare, followed by the year or time we want to compare it to.
82. What is the difference between Laravel’s “Eloquent” ORM and other popular ORM solutions?
Feature | Laravel Eloquent | Other ORM Solutions |
---|---|---|
Syntax | Uses a fluent, chainable syntax | Varies |
Database Support | Supports multiple databases | Varies |
Model Relationships | Yes, supports multiple types | Varies |
Query Building | Yes, supports query building | Varies |
Data Validation | Yes, supports data validation | Varies |
Soft Deleting | Yes, supports soft deleting | Varies |
Pagination Support | Yes, supports pagination | Varies |
Mass Assignment Protection | Yes, supports it | Varies |
Performance Optimization | Includes caching and eager loading | Varies |
Database Migrations | Includes built-in migration support | Varies |
Laravel-specific syntax and functionality | Yes, includes Laravel-specific tags | Varies |
83. How do you use the has() and doesntHave() methods in Laravel?
- The has() method is used to retrieve records that have a related record in another table.
- The doesntHave() method is used to retrieve records that do not have a related record in another table.
- To use either method, we can chain the method onto a query builder instance and pass the name of the relationship we want to check.
84. How do you use the with() and withCount() methods in Laravel?
- The with() method is used to eager load related records onto a query.
- The withCount() method is used to retrieve the count of related records onto a query.
- To use either method, we can chain the method onto a query builder instance and pass the name of the relationship we want to load or count.
85. How do you use the load() and loadMissing() methods in Laravel?
- The load() method is used to lazy load related records onto a model.
- The loadMissing() method is used to lazy load only the related records that have not been loaded yet.
- To use either method, we can call the method on a model instance and pass the name of the relationship we want to load or loadMissing.
86. How do you use the create() and firstOrCreate() methods in Laravel?
- The create() method is used to create a new model instance and persist it to the database.
- The firstOrCreate() method is used to retrieve the first record that matches a query or create a new record if no matches are found.
- To use either method, we can call the method on a model class and pass an array of attributes we want to assign to the new or retrieved record.
87. How do you use the update() and updateOrCreate() methods in Laravel?
- The update() method is used to update one or more existing records in the database.
- The updateOrCreate() method is used to update an existing record if it exists or create a new record if it does not.
- To use either method, we can call the method on a query builder instance and pass an array of attributes we want to update or create.
88. How do you use the increment() and decrement() methods in Laravel?
- The increment() method is used to increment the value of a column in the database.
- The decrement() method is used to decrement the value of a column in the database.
- To use either method, we can call the method on a model instance or query builder instance and pass the name of the column we want to increment or decrement.
89. How do you use the delete() and forceDelete() methods in Laravel?
- The delete() method is used to delete a record from the database.
- The forceDelete() method is used to permanently delete a record from the database without triggering any model events.
90. How do you use the toArray() and toJson() methods in Laravel?
- The toArray() method is used to convert a model instance or a collection of instances to an array.
- The toJson() method is used to convert a model instance or a collection of instances to a JSON string.
91. How do you use the whereJsonContains() method in Laravel?
- The
whereJsonContains()
method in Laravel is used to perform a search for a specific JSON value in a JSON column. - To use this method, you can call it on a query builder instance and pass in the name of the JSON column you want to search in, followed by the value you want to search for.
- You can also use the
orWhereJsonContains()
method to search for multiple values in the same JSON column.
92. How do you use the hasOneThrough() and hasManyThrough() methods in Laravel?
- The
hasOneThrough()
andhasManyThrough()
methods in Laravel are used to define a relationship that links two tables through a third table. - To use these methods, you must first define the relationships between each of the tables in the model classes, and then define the relationship method using the
hasOneThrough()
orhasManyThrough()
method in the parent model class.
93. How do you use the morphTo() and morphMany() methods in Laravel?
- The
morphTo()
andmorphMany()
methods in Laravel are used to define a polymorphic relationship between two tables. - To use these methods, you must first add a
morphs
column to the table that will contain the relationship, and then define the relationship method using themorphTo()
ormorphMany()
method in the model classes.
94. How do you use the belongsToMany() method in Laravel?
- The
belongsToMany()
method in Laravel is used to define a many-to-many relationship between two tables. - To use this method, you must first define the relationship between the two tables in the corresponding model classes, and then define a new method for the relationship in each model.
95. How do you use the morphToMany() and morphedByMany() methods in Laravel?
- The
morphToMany()
andmorphedByMany()
methods in Laravel are used to define a many-to-many polymorphic relationship between two tables. - To use these methods, you must first add a
morphs
column to the table that will contain the relationship, and then define the relationship method using themorphToMany()
ormorphedByMany()
method in the model classes.
96. How do you use the touch() method in Laravel?
- The
touch()
method in Laravel is used to update theupdated_at
timestamp on a related model when a new model is created or updated. - To use this method, you must first define the relationship between the two models in the corresponding model classes, and then call the
touch()
method on the related model in the create or update method of the parent model.
97. How do you use the withTimestamps() and as() methods in Laravel?
- The
withTimestamps()
method in Laravel is used to automatically set thecreated_at
andupdated_at
timestamps on a many-to-many relationship. - The
as()
method in Laravel is used to specify a custom name for the pivot table in a many-to-many relationship.
98. How do you use the morphMap() method in Laravel?
- The
morphMap()
method in Laravel is used to define a custom mapping between a polymorphic type and its corresponding model class. - To use this method, you must define the mapping in the
boot()
method of yourAppServiceProvider
.
99. How do you use the static method resolution in Laravel?
- The static method resolution in Laravel is used to resolve dependencies for a class without using constructor injection.
- To use this method, you can define a static method on the class that returns an instance of the dependency, and then use the
app()->call()
method to resolve the dependency when the method is called.
100. How do you use the deferred provider loading in Laravel?
- Deferred provider loading in Laravel is used to improve the performance of your application by only loading service providers when they are actually needed.
- To use this method, you can define the service provider as “deferred” in the
register()
method of the service provider class, and then add it to theproviders
array in theconfig/app.php
file with thedeferred
key set totrue
. - Then, when you need to use the service provided by the provider, you can call the
app()->loadDeferredProvider()
method to load the provider on demand.
The Top 100 Laravel Interview Questions and Answers provided comprehensive insights into Laravel framework, covering all aspects from installation to advanced concepts, helping job seekers prepare for their next technical interview. To expand your knowledge, be sure to follow us at freshersnow.com, where you can find more valuable insights and information.