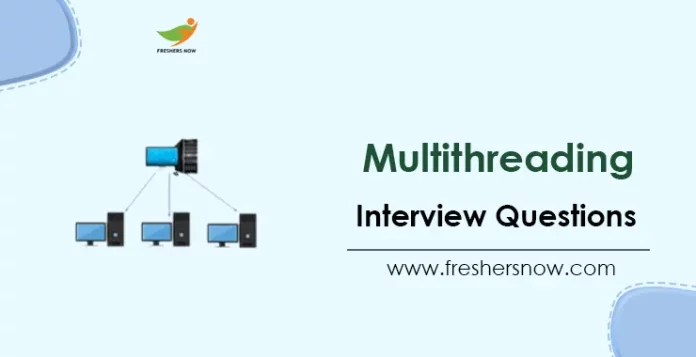
Multithreading Interview Questions and Answers: Our collection of Top 100 Multithreading Interview Questions and Answers can help you prepare for a wide range of topics and scenarios on Multi threading.
★★ Latest Technical Interview Questions ★★
Multithreading Technical Interview Questions
If you’re aiming to improve your understanding of the Latest Multithreading and want to be well-prepared for Multithreading Technical Interview, our collection of the Top 100 Multithreading Interview Questions and Answers can be a valuable resource. Multithreading Interview Questions cover a diverse range of topics and scenarios, helping you to broaden your knowledge and build confidence in your abilities.
Top 100 Multithreading Interview Questions and Answers
1. What is multithreading, and how does it differ from single-threaded programming?
Multithreading is the concurrent execution of two or more threads within a single program. It differs from single-threaded programming in that it allows for multiple threads to run simultaneously and share resources.
2. What are the advantages of multithreading?
The advantages of multithreading include improved program performance, better resource utilization, increased responsiveness, and enhanced scalability.
3. What’s the difference between notify() and notifyAll()?
notify(): It sends a notification and wakes up only a single thread instead of multiple threads that are waiting on the object’s monitor.
notifyAll(): It sends notifications and wakes up all threads and allows them to compete for the object’s monitor instead of a single thread.
4. What are the potential drawbacks of multithreading?
The potential drawbacks of multithreading include increased complexity, the potential for race conditions and deadlocks, and difficulties with debugging and testing.
5. What are the common use cases for multithreading?
Common use cases for multithreading include network programming, GUI development, scientific computing, and parallel processing.
6. Explain the meaning of the deadlock and when it can occur?
Deadlock is a scenario in which multiple threads are unable to proceed and are indefinitely blocked. This can occur when multiple threads acquire locks on separate resources and are waiting for other resources to become available before continuing their execution.
The diagram above illustrates a deadlock scenario where two threads are unable to proceed and are indefinitely blocked. Thread 1 has acquired a lock on Object 1 but requires access to Object 2 to complete its task, while Thread 2 holds a lock on Object 2 but needs access to Object 1 before it can proceed. As a result, both threads are stuck waiting for each other to release the required resource, and neither thread can make progress toward completing its task. This leads to a situation where both threads hold their respective locks indefinitely and are unable to finish their processing.
7. How does multithreading affect program performance?
Multithreading can significantly improve program performance by allowing multiple tasks to run simultaneously and take advantage of available system resources.
8. What is thread starvation?
Thread starvation occurs when a thread is unable to access shared resources on a regular basis, causing it to become stalled and unable to make progress. This can happen when other threads with higher priority occupy the shared resources for extended periods, leaving lower-priority threads without access to the resources they need to complete their tasks. Typically, low-priority threads are more susceptible to thread starvation, as they may not be allocated enough CPU time to execute their tasks and may have to wait for extended periods to access shared resources.
9. How do you create a new thread in Java?
In Java, you can create a new thread by extending the Thread class or implementing the Runnable interface and then calling the start() method.
10. What is the syntax for creating a new thread in Java?
To create a new thread in Java, you can either extend the Thread class or implement the Runnable interface and then call the start() method on the thread object. The syntax for extending the Thread class is:
public class MyThread extends Thread { public void run() { // thread code here } } MyThread thread = new MyThread(); thread.start();
The syntax for implementing the Runnable interface is:
public class MyRunnable implements Runnable { public void run() { // thread code here } } Thread thread = new Thread(new MyRunnable()); thread.start();
11. What is thread synchronization, and why is it necessary?
Thread synchronization is the process of coordinating the execution of multiple threads to ensure that they do not interfere with each other when accessing shared resources. It is necessary to prevent race conditions and ensure program correctness.
12. What is BlockingQueue?
A BlockingQueue is a thread-safe queue that allows for the storage and retrieval of elements. The producer thread can add elements to the queue using the put() method, which will block if the queue is full, while the consumer thread can retrieve elements from the queue using the take() method, which will block if the queue is empty. If a thread tries to remove an element from an empty queue, it will be blocked until another thread inserts an element into the queue.
13. What are the different types of thread synchronization?
The different types of thread synchronization include locking, signaling and wait/notify mechanisms.
14. How do you implement thread synchronization in Java?
Thread synchronization in Java can be implemented using the synchronized keyword, locks, semaphores, and other mechanisms.
15. Explain thread priority.
Thread priority refers to the relative importance of a thread and determines the order in which threads are scheduled for execution by the operating system. Threads with higher priority are given precedence over lower-priority threads for processor time. While it is possible to specify the priority of a thread, it is not guaranteed that a high-priority thread will always execute before a lower-priority thread. Ultimately, the thread scheduler of the operating system is responsible for assigning processor time to threads based on their priority.
16. Difference between multithreading and multiprocessing
Multithreading | Multiprocessing |
---|---|
In multithreading, multiple threads are created within a single process. | In multiprocessing, multiple processes are created. |
Threads share the same memory space and can access the same data within the process. | Processes have separate memory spaces and do not share data directly. |
Multithreading is useful for applications that perform multiple tasks concurrently but don’t require high levels of CPU usage. | Multiprocessing is useful for applications that require high levels of CPU usage, such as scientific computing or rendering. |
Multithreading is less expensive in terms of system resources because threads share the same memory space. | Multiprocessing is more expensive in terms of system resources because each process has its own memory space and requires more CPU usage. |
17. What is the difference between a parallel stream and a sequential stream?
- A sequential stream processes elements one by one in a single thread.
- A parallel stream splits the stream into multiple substreams that can be processed in parallel by multiple threads.
18. What is the ThreadPoolExecutor class, and how can you use it to implement a custom thread pool?
- The ThreadPoolExecutor class provides a thread pool implementation for executing tasks.
- It manages a pool of worker threads, allowing multiple tasks to be executed concurrently.
- To implement a custom thread pool using the ThreadPoolExecutor class, you can create an instance of the class and configure it with the desired parameters, such as the core pool size, maximum pool size, and work queue.
19. What is the syntax for acquiring a lock in Java?
To acquire a lock in Java, you can use the synchronized keyword followed by the object you want to lock on.
The syntax is:
synchronized (lockObject) { // critical section code here }
20. What is the ScheduledExecutorService class, and how can you use it to schedule tasks?
- The ScheduledExecutorService class extends ExecutorService and provides methods for scheduling tasks to be executed at a specific time or after a certain delay.
- To schedule a task using the ScheduledExecutorService class, you can create an instance of the class, submit the task to the executor using the schedule() method, and specify the desired time or delay.
21. What is the ConcurrentLinkedQueue class, and how can you use it to implement a thread-safe queue?
- The ConcurrentLinkedQueue class is a thread-safe implementation of the Queue interface that allows multiple threads to access the queue concurrently.
- To implement a thread-safe queue using the ConcurrentLinkedQueue class, you can create an instance of the class and use its methods to add and remove elements from the queue.
22. Difference between multithreading and asynchronous programming
Multithreading | Asynchronous Programming |
---|---|
Multithreading is a technique that allows multiple threads to execute concurrently within a single process. | Asynchronous programming is a technique that allows a single thread to execute multiple tasks concurrently without blocking. |
Multithreading is useful for applications that require parallelism and use multiple CPU cores. | Asynchronous programming is useful for applications that perform I/O operations and don’t require high levels of CPU usage. |
Multithreading can be more complicated to implement and debug because of potential race conditions and deadlocks. | Asynchronous programming can be simpler to implement because it doesn’t require multiple threads and can be done with just one thread. |
Multithreading can cause performance issues if there are too many threads competing for CPU resources. | Asynchronous programming can improve performance by allowing tasks to be executed without blocking the main thread. |
23. What is the BlockingQueue interface, and how can you use it to implement a producer-consumer pattern?
- The BlockingQueue interface extends the Queue interface and provides additional methods for blocking when the queue is full or empty.
- To implement a producer-consumer pattern using the BlockingQueue interface, you can create an instance of the interface and use its methods to add elements to the queue (producer) and remove elements from the queue (consumer).
24. What is the syntax for releasing a lock in Java?
To release a lock in Java, you can simply exit the synchronized block. The syntax is:
synchronized (lockObject) { // critical section code here } // lock is automatically released here
25. What is the ConcurrentHashMap class, and how can you use it to implement thread-safe map operations?
- The ConcurrentHashMap class is a thread-safe implementation of the Map interface that allows multiple threads to access the map concurrently.
- To implement thread-safe map operations using the ConcurrentHashMap class, you can create an instance of the class and use its methods to add, remove, and update key-value pairs in the map.
26. What is the Atomic Boolean class, and how can you use it to implement thread-safe boolean operations?
- The Atomic Boolean class is a class that provides atomic operations for a boolean value.
- To implement thread-safe boolean operations using the AtomicBoolean class, you can create an instance of the class and use its methods to perform operations on the boolean value.
27. What is the AtomicInteger class, and how can you use it to implement thread-safe integer operations?
- The AtomicInteger class is a class that provides atomic operations for an integer value.
- To implement thread-safe integer operations using the AtomicInteger class, you can create an instance of the class and use its methods to perform operations on the integer value.
28. Difference between a thread and a process in a multithreaded environment
Thread | Process |
---|---|
A thread is a unit of execution within a process that shares the same memory space as other threads within the same process. | A process is an instance of an executing program that has its own memory space, resources, and state. |
Threads can communicate with each other directly by accessing shared memory. | Processes communicate with each other indirectly through inter-process communication (IPC) mechanisms such as pipes, sockets, or message queues. |
Threads are lightweight and require fewer system resources than processes. | Processes are heavier and require more system resources than threads. |
Threads are suitable for applications that require parallelism and share data frequently. | Processes are suitable for applications that need to be isolated from each other and require more CPU usage. |
29. What is the syntax for waiting for a thread to finish in Java?
To wait for a thread to finish in Java, you can call the join() method on the thread object. The syntax is:
thread.join();
30. What is the AtomicLong class, and how can you use it to implement thread-safe long operations?
- The AtomicLong class is a class that provides atomic operations for a long value.
- To implement thread-safe long operations using the AtomicLong class, you can create an instance of the class and use its methods to perform operations on the long value.
31. What is a race condition, and how can you prevent it in multithreaded code?
A race condition occurs when two or more threads access a shared resource in an unexpected order, leading to unexpected behavior. To prevent race conditions in multithreaded code, you can use synchronization mechanisms like locks and semaphores or use thread-safe data structures.
32. What is a deadlock, and how can you prevent it in multithreaded code?
A deadlock is a situation in multithreaded programming where two or more threads are blocked and waiting for each other to release resources. Deadlocks can occur when threads are competing for resources such as memory, files, or network connections. To prevent deadlocks, you can use techniques such as avoiding circular waits, using a timeout mechanism to release resources, and using a resource allocation hierarchy.
33. What is a livelock, and how can you prevent it in multithreaded code?
Livelock is a situation in multithreaded programming where two or more threads are stuck in a loop, constantly changing their state but never making any progress. Livelocks can occur when threads are trying to resolve a deadlock, but the solution they choose only leads to more deadlocks. To prevent livelocks, you can use techniques such as randomizing the order in which threads acquire locks or using a timeout mechanism to force threads to give up their locks.
34. What is the difference between the synchronized and volatile keywords?
- Both keywords are used in multi-threaded programming to ensure thread safety and prevent race conditions.
- The main difference is that ‘synchronized’ ensures mutual exclusion by allowing only one thread to execute the synchronized code block or method at a time, while ‘volatile’ ensure visibility by guaranteeing that the value of a variable will be immediately visible to all threads.
- In other words, ‘synchronized’ controls access to shared resources, while ‘volatile’ guarantees visibility of changes to shared variables.
35. Difference between multithreading and parallel computing
Multithreading | Parallel Computing |
---|---|
Multithreading is a technique that allows multiple threads to execute concurrently within a single process. | Parallel computing is a technique that allows multiple processes or threads to execute concurrently across multiple CPUs or computers. |
Multithreading is limited by the number of available CPU cores on a single machine. | Parallel computing can scale to multiple CPUs or computers to increase processing power. |
Multithreading is suitable for applications that require parallelism and use multiple CPU cores. | Parallel computing is suitable for applications that require high levels of processing power, such as scientific computing or rendering. |
36. What is the synchronized block, and how does it work?
- The synchronized block is a Java language feature used to control access to shared resources by allowing only one thread to execute the block at a time.
- It is used to prevent race conditions and ensure thread safety.
- The synchronized block is defined by the ‘synchronized’ keyword followed by a lock object, which can be any object.
- Only one thread at a time can hold the lock object and execute the synchronized block, while all other threads that try to enter the block are blocked until the lock is released.
37. What is the synchronized method, and how does it work?
- The synchronized method is a Java language feature used to control access to shared resources by allowing only one thread to execute the method at a time.
- It is used to prevent race conditions and ensure thread safety.
- The synchronized method is defined by the ‘synchronized’ keyword before the method declaration.
- Only one thread at a time can execute the synchronized method, while all other threads that try to call the method are blocked until the execution of the method is completed.
38. What is the syntax for creating a thread pool in Java?
To create a thread pool in Java, you can use the Executor framework. The syntax for creating a fixed-size thread pool is:
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
39. What is the wait() method, and how does it work with threads?
- The wait() method is a Java language feature used to pause the execution of a thread and release the lock on the object until another thread notifies it.
- The wait() method can only be called from within a synchronized block or method.
- When a thread calls wait(), it releases the lock on the object and goes into a waiting state until another thread calls notify() or notifyAll() on the same object.
- Once notified, the waiting thread is reactivated and attempts to reacquire the lock on the object before continuing execution.
40. What is the notify() method, and how does it work with threads?
- The notify() method is a Java language feature used to wake up a single thread that is waiting on a particular object.
- The notify() method can only be called from within a synchronized block or method.
- When a thread calls notify() on an object, it wakes up a single thread that is waiting on that object and allows it to attempt to reacquire the lock on the object before continuing execution.
41. What is the notifyAll() method, and how does it work with threads?
- The notifyAll() method is a Java language feature used to wake up all threads that are waiting on a particular object.
- The notifyAll() method can only be called from within a synchronized block or method.
- When a thread calls notifyAll() on an object, it wakes up all threads that are waiting on that object and allows them to attempt to reacquire the lock on the object before continuing execution.
42. Difference between multithreading and multitasking
Multithreading | Multitasking |
---|---|
Multithreading is a technique that allows multiple threads to execute concurrently within a single process. | Multitasking is a technique that allows multiple processes or applications to run concurrently on a single machine. |
Multithreading is limited by the number of available CPU cores on a single machine. | Multitasking is limited by the number of available system resources such as memory and CPU usage. |
Multithreading is suitable for applications that require parallelism and use multiple CPU cores. | Multitasking is suitable for applications that need to run simultaneously on a single machine, such as running multiple applications at once. |
Multithreading can share memory and data between threads within the same process. | Multitasking cannot share memory and data directly between processes or applications. |
43. What is the yield() method, and how does it affect thread scheduling?
- The yield() method is a Java language feature used to give a hint to the scheduler that the current thread is willing to yield its current use of the CPU.
- When a thread calls yield(), it gives up its current time slice and allows another thread of equal or higher priority to run.
- However, there is no guarantee that the scheduler will honor the yield request
44. What is thread safety, and how can you achieve it?
Thread safety is the property of a program or system that ensures correct behavior in the presence of concurrent access by multiple threads. Achieving thread safety involves ensuring that shared data structures and resources are accessed in a mutually exclusive manner. This can be achieved through techniques such as using synchronization primitives like locks and semaphores, or by using immutable data structures.
45. What is the syntax for submitting a task to a thread pool in Java?
To submit a task to a thread pool in Java, you can use the submit() method of the ExecutorService interface. The syntax is:
executor.submit(new MyTask());
46. What are the different thread states in Java?
In Java, threads can be in one of several states:
- New: when a thread is first created, it is in a new state.
- Runnable: when a thread is ready to run, it is in the runnable state.
- Blocked: when a thread is waiting for a monitor lock, it is in the blocked state.
- Waiting: when a thread is waiting for another thread to perform a specific action, it is in the waiting state.
- Timed waiting: when a thread is waiting for a specified amount of time, it is in the timed waiting state.
- Terminated: when a thread has finished executing, it is in the terminated state.
46. What is a thread pool, and how does it work?
A thread pool is a collection of threads that can be used to execute tasks concurrently. The threads in a thread pool are created in advance and can be reused multiple times to perform tasks. This can improve performance by avoiding the overhead of creating and destroying threads for each task. Thread pools can be implemented using libraries such as Java’s Executor framework.
47. How do you implement a thread pool in Java?
In Java, you can implement a thread pool using the Executor framework, which provides a high-level API for managing a pool of threads. To create a thread pool, you can use the Executors.newFixedThreadPool() method, which creates a thread pool with a fixed number of threads. You can then submit tasks to the thread pool using the submit() method.
48. What is a semaphore, and how does it help with thread synchronization?
A semaphore is a synchronization primitive that can be used to control access to a shared resource. A semaphore maintains a count of the number of threads that are allowed to access the resource simultaneously. Threads can acquire or release the semaphore, and if the count reaches zero, subsequent requests for the semaphore will block until a thread releases it.
49. Difference between a mutex and a semaphore in multithreading
Mutex | Semaphore |
---|---|
A mutex is a synchronization mechanism that allows only one thread to access a shared resource at a time. | A semaphore is a synchronization mechanism that allows multiple threads to access a shared resource at a time. |
A mutex can be used to protect a critical section of code from concurrent access. | A semaphore can be used to limit the number of threads that can access a shared resource at a time. |
A mutex can be either locked or unlocked by a single thread. | A semaphore can be either binary or counting, with binary semaphores allowing only one thread to access a shared resource at a time and counting semaphores allowing a specified number of threads to access a shared resource at a time. |
A mutex is typically used to protect shared data structures such as linked lists or queues. | A semaphore is typically used to control access to a shared resource such as a database or network connection. |
50. What is a monitor, and how does it help with thread synchronization?
A monitor is a synchronization construct that provides mutual exclusion and condition synchronization. Monitors can be used to protect shared resources by ensuring that only one thread can access the resource at a time. Monitors also provide condition variables, which can be used to signal and wait for specific conditions to be met.
51. What is the syntax for scheduling a task to run after a delay in Java?
To schedule a task to run after a delay in Java, you can use the ScheduledExecutorService interface. The syntax for scheduling a task to run after a delay of 10 seconds is:
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1); scheduler.schedule(new MyTask(), 10, TimeUnit.SECONDS);
52. What is a condition variable, and how does it help with thread synchronization?
A condition variable is a synchronization primitive that can be used to coordinate threads and signal when a certain condition has been met. Condition variables are typically used in conjunction with a lock or monitor to ensure that threads wait for the condition to be true before proceeding. Threads can wait on a condition variable by calling its wait() method, and another thread can signal the condition variable by calling its signal() or signalAll() method.
53. What is a mutex, and how does it help with thread synchronization?
A mutex is a synchronization primitive that can be used to ensure mutual exclusion when accessing shared resources. A mutex provides a way for threads to acquire and release a lock on a resource to prevent other threads from accessing it simultaneously. This helps to prevent data races and other synchronization problems.
54. What is the difference between a thread and a process?
A process is an instance of a program that is running on a computer, while a thread is a lightweight unit of execution within a process. A process can have multiple threads, each of which can execute concurrently. Threads share memory and resources within a process, while processes are isolated from each other and have their own memory and resources.
55. What is the difference between a user thread and a daemon thread?
In Java, a user thread is a thread that is created by the application code, while a daemon thread is a thread that runs in the background and is created by the Java Virtual Machine (JVM). User threads are typically used for tasks that are initiated by the application, while daemon threads are used for tasks that need to run in the background and can be terminated when the main application terminates.
56. Difference between multithreading and a coroutine
Multithreading | Coroutine |
---|---|
Multithreading is a technique that allows multiple threads to execute concurrently within a single process. | A coroutine is a lightweight thread that can be scheduled by a single thread to perform multiple tasks concurrently. |
Multithreading can use multiple CPUs or computers to increase processing power. | Coroutines can only be scheduled by a single thread and cannot use multiple CPUs or computers. |
Multithreading is more complex to implement and debug because of potential race conditions and synchronization issues. | Coroutines are simpler to implement and debug because they do not require multiple threads and can be done with just one thread. |
Multithreading can cause performance issues if there are too many threads competing for CPU resources. | Coroutines can improve performance by allowing tasks to be executed without blocking the main |
57. What is the syntax for creating a thread-safe singleton in Java?
To create a thread-safe singleton in Java, you can use the double-checked locking pattern. The syntax is:
public class Singleton { private static volatile Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } }
58. What is the difference between a lightweight thread and a heavyweight thread?
A lightweight thread, also known as a green thread, is a thread that is managed by a user-level thread library rather than by the operating system. Lightweight threads are faster and have less overhead than heavyweight threads, which are managed by the operating system. Lightweight threads are used in languages like Java and Python, while heavyweight threads are used in lower-level languages like C and C++.
59. What is the ThreadLocal class, and how can you use it?
The ThreadLocal class in Java provides a way to create variables that are local to each thread. This can be useful in multithreaded applications where each thread requires its own copy of a variable.
60. What is the CopyOnWriteArrayList class, and how can you use it to implement a thread-safe list?
The CopyOnWriteArrayList class is a thread-safe implementation of the List interface in Java. It is designed for scenarios where reads are much more frequent than writes. It achieves thread safety by creating a new copy of the underlying list every time a modification is made, ensuring that reads are always performed on a consistent view of the list.
To use a CopyOnWriteArrayList, you can create an instance of the class and use its methods to add, remove, and retrieve elements from the list. Because the list is thread-safe, multiple threads can safely access the list at the same time without the need for external synchronization.
61. What is the syntax for interrupting a thread in Java?
To interrupt a thread in Java, you can call the interrupt() method on the thread object. The syntax is:
thread.interrupt();
62. What is the CopyOnWriteArraySet class, and how can you use it to implement a thread-safe set?
The CopyOnWriteArraySet class is a thread-safe implementation of the Set interface in Java. It is similar to the CopyOnWriteArrayList class but provides a set instead of a list.
To use a CopyOnWriteArraySet, you can create an instance of the class and use its methods to add, remove, and retrieve elements from the set. Because the set is thread-safe, multiple threads can safely access the set at the same time without the need for external synchronization.
63. What is the ThreadMXBean interface, and how can you use it to monitor and manage threads?
The ThreadMXBean interface is a Java Management Extension (JMX) interface that provides methods for monitoring and managing threads in a Java virtual machine (JVM). It allows you to retrieve information about threads, such as their CPU usage and stack traces, and to manipulate threads, such as suspending and resuming them.
To use the ThreadMXBean interface, you can obtain an instance of the interface using the ManagementFactory.getThreadMXBean() method. You can then call its methods to retrieve information about threads or manipulate threads as needed.
64. What is the ThreadInfo class, and how can you use it to retrieve information about a thread?
The ThreadInfo class is a class in Java that provides information about a thread, such as its state, CPU usage, and stack trace. It is used in conjunction with the ThreadMXBean interface to retrieve information about threads in a JVM.
To use the ThreadInfo class, you can call the ThreadMXBean.getThreadInfo(long[] threads) method, passing in an array of thread IDs for the threads you want to retrieve information about. This method will return an array of ThreadInfo objects containing the information for each thread.
65. What is the Garbage Collector MXBean, and how can you use it to monitor and manage the garbage collector?
The Garbage Collector MXBean is a JMX interface that provides methods for monitoring and managing the garbage collector in a JVM. It allows you to retrieve information about the garbage collector, such as its collection times and memory usage, and to manipulate the garbage collector, such as forcing a garbage collection.
66. What is the syntax for creating a thread-safe queue in Java?
To create a thread-safe queue in Java, you can use the ArrayBlockingQueue class. The syntax for creating a queue with a capacity of 100 is:
BlockingQueue<String> queue = new ArrayBlockingQueue<String>(100);
67. What is the Executor framework, and how does it simplify multithreaded programming?
The Executor framework is a built-in Java library that provides a simple way to manage and execute threads. It simplifies multithreaded programming by providing a standard way to manage thread pools and execute tasks asynchronously.
68. What is a Callable, and how does it differ from a Runnable?
A Callable is a functional interface in Java that represents a task that can return a value. It differs from a Runnable in that a Callable can return a value and can throw an exception.
69. What is a Future, and how can you use it to retrieve the result of a Callable?
A Future is an interface in Java that represents the result of an asynchronous computation. It can be used to retrieve the result of a Callable by calling the get() method on the Future object.
70. What is a CountDownLatch, and how does it help with thread synchronization?
A CountDownLatch is a synchronization mechanism in Java that allows one or more threads to wait until a set of operations has been completed. It helps with thread synchronization by ensuring that all threads have completed a set of tasks before continuing.
71. What is a CyclicBarrier, and how does it help with thread synchronization?
A CyclicBarrier is a synchronization mechanism in Java that allows a set of threads to wait for each other to reach a common point before continuing. It helps with thread synchronization by ensuring that all threads have completed a task before continuing.
72. What is a Phaser, and how does it help with thread synchronization?
A Phaser is a synchronization mechanism in Java that allows multiple threads to coordinate and synchronize their execution. It helps with thread synchronization by allowing threads to wait for each other to complete a set of tasks before continuing.
73. What is the CMS garbage collector, and how does it differ from other garbage collectors?
The CMS (Concurrent Mark Sweep) garbage collector is a low-pause collector that aims to minimize the interruption time of the application. It differs from other garbage collectors by running concurrently with the application threads and performing garbage collection in the background, without completely stopping the application threads. This approach reduces overall pause times and is ideal for applications that require high responsiveness.
74. What is the Parallel garbage collector, and how does it differ from other garbage collectors?
The Parallel garbage collector is a throughput collector that maximizes the application’s processing throughput. It works by using multiple threads to perform garbage collection in parallel with the application threads. This collector is best suited for applications that require maximum throughput and can tolerate brief pauses for garbage collection.
75. What is the Z garbage collector, and how does it differ from other garbage collectors?
The Z garbage collector is a low-latency garbage collector that aims to minimize overall pause times while maintaining high throughput. It works by performing garbage collection in short, concurrent phases that run concurrently with the application threads. The Z garbage collector is scalable and can handle heaps ranging from small to very large.
76. What is the difference between the Thread and Runnable interfaces?
The Thread interface represents a single thread of execution, while the Runnable interface represents a task that can be executed by a thread. The Thread interface provides a way to start and stop threads, while the Runnable interface provides a way to define the code that will be executed by a thread.
77. What is the Thread.sleep() method, and how does it affect thread scheduling?
The Thread.sleep() method suspends the execution of the current thread for a specified amount of time, introducing a delay in the current thread’s execution. This delay allows other threads to execute and can be useful for coordinating the execution of multiple threads.
78. What is the Thread.join() method, and how does it work with threads?
The Thread.join() method waits for the specified thread to complete its execution before continuing the execution of the current thread. It works by suspending the current thread until the specified thread has terminated.
79. What is the Thread. interrupt() method, and how does it work with threads?
The Thread. interrupt() method interrupts the specified thread if it is currently blocked by setting a flag on the thread. The thread can periodically check this flag to see if it has been interrupted.
80. What is the Thread.currentThread() method, and how can you use it to retrieve information about the current thread?
The Thread.currentThread() method returns a reference to the currently executing thread. It can be used to retrieve information about the current thread, such as its name, priority, and state.
81. What is the Thread.setDefaultUncaughtExceptionHandler() method, and how can you use it to handle uncaught exceptions in threads?
The Thread.setDefaultUncaughtExceptionHandler() method sets the default uncaught exception handler for all threads in the application. It can be used to handle uncaught exceptions that occur in threads that do not have their own exception handlers. By default, uncaught exceptions in threads are handled by printing the stack trace to the console.
82. What is the ForkJoin framework, and how does it work?
The ForkJoin framework is a built-in Java library that provides a way to perform parallel processing on large datasets. It works by dividing the data into smaller sub-tasks and processing them in parallel using a pool of worker threads.
83. How do you create a parallel stream in Java 8?
To create a parallel stream in Java 8, you can simply call the parallel stream () method on a collection or stream object. This will automatically create a parallel stream that can be processed in parallel by multiple threads.
84. What is the sleep() method and how does it affect thread scheduling?
- The sleep() method is a Java language feature used to pause the execution of a thread for a specified amount of time.
- When a thread calls sleep(), it gives up its time slice and goes into a dormant state for the specified duration.
- While sleeping, the thread is blocked and does not consume CPU resources, allowing other threads to run.
- After the specified time has elapsed, the thread is awakened and placed in the runnable state, where it waits for a CPU time slice to resume execution.
85. What is the join() method, and how does it work with threads?
- The join() method is a Java language feature used to wait for a thread to complete its execution before continuing with the execution of the current thread.
- When a thread calls join() on another thread, it blocks until the other thread completes its execution.
- If the other thread is already finished, the join() method returns immediately.
- The join() method can be used to coordinate the execution of multiple threads and ensure that they complete their tasks in a specific order.
86. What is the interrupt() method, and how does it work with threads?
- The interrupt() method is a Java language feature used to interrupt the execution of a thread that is currently blocked or sleeping.
- When a thread is interrupted, its interrupt status is set to true, which can be checked using the interrupted () method.
- If the interrupted thread is sleeping or waiting, it is awakened and throws an InterruptedException.
- If the interrupted thread is executing, the interrupt status is checked at periodic intervals, and the thread can choose to exit or continue running.
87. What is the interrupted () method, and how does it work with threads?
- The interrupted () method is a Java language feature used to check if a thread has been interrupted.
- When a thread is interrupted using the interrupt() method, its interrupt status is set to true, and the interrupted () method returns true.
- The interrupted () method can be used to check the interrupt status of a thread and take appropriate action based on the result.
88. What is the Thread.yield() method, and how does it affect thread scheduling?
- The Thread.yield() method is a static method of the Thread class that is used to give a hint to the scheduler that the current thread is willing to yield its current use of the CPU.
- When a thread calls Thread.yield(), it gives up its current time slice and allows another thread of equal or higher priority to run.
- However, there is no guarantee that the scheduler will honor the yield request.
- The behavior of Thread.yield() can vary depending on the operating system and the scheduler implementation.
89. What is the ThreadLocalRandom class, and how can you use it to generate random numbers in a multithreaded environment?
The ThreadLocalRandom class is a class in Java that provides a fast, efficient, and thread-safe way to generate random numbers in a multithreaded environment. It is similar to the java. util.Random class but provides better performance in a concurrent environment.
To use ThreadLocalRandom, you can call its static methods to generate random numbers.
For example, to generate a random integer between 0 and 100, you can use:
int randomNum = ThreadLocalRandom.current().nextInt(0, 101);
90. What is the Phaser class, and how can you use it to coordinate multiple threads?
The Phaser class is a synchronization aid that allows threads to wait for each other to reach a certain point in their execution before proceeding. It is useful for coordinating multiple threads that need to work together to complete a task.
To use a Phaser, you first create an instance of the class and specify the number of threads that will be using it. Then, each thread can call the arriveAndAwaitAdvance() method to indicate that it has reached a certain point in its execution and wait for the other threads to do the same. Once all threads have arrived, the Phaser will advance to the next phase, and the threads can continue with their execution.
91. What is the LockSupport class, and how can you use it to pause and resume threads?
The LockSupport class is a utility class that provides methods for suspending and resuming threads. It is useful for implementing low-level synchronization constructs. To pause a thread using LockSupport, you can call the park() method. This will cause the thread to wait until it is unparked. To resume a thread, you can call the unpark(Thread) method, passing in the thread you want to unpark.
92. What is the ExecutorCompletionService class, and how can you use it to retrieve results from multiple threads?
The ExecutorCompletionService class is a utility class that simplifies the process of retrieving results from multiple threads. It allows you to submit tasks to an executor service and retrieve the results of those tasks as they become available, in the order that they were completed.
To use an ExecutorCompletionService, you first create an instance of the class and provide it with an ExecutorService. Then, you submit tasks to the executor service using its submit() method and pass the resulting Future objects to the complete service submit() method. You can then use the completion service’s take() method to retrieve the completed tasks, which will be returned as Future objects.
93. What is the SynchronousQueue class, and how can you use it to implement a thread-safe blocking queue?
The SynchronousQueue class is a thread-safe blocking queue in Java. It is a queue that can only hold a single element and requires that a consumer be ready to take an element from the queue before a producer can add an element.
To use a SynchronousQueue, you can call its put() method to add an element to the queue, and its take() method to remove an element from the queue. If there is no element in the queue when take() is called, the thread will block until an element becomes available.
94. What is the ForkJoinPool class, and how can you use it to implement a recursive divide-and-conquer algorithm?
The ForkJoinPool class is a thread pool designed for executing recursive, divide-and-conquer algorithms in Java. It is particularly useful for algorithms that can be parallelized into smaller sub-tasks that can be executed independently.
95. Is it possible to call the run() method directly to start a new thread?
Yes, it is possible to call the run()
method directly to start a new thread, but this will not create a new thread of execution. Instead, it will simply execute the run()
method in the same thread that made the method call.
96. Is it possible that each thread can have its stack in multithreaded programming?
Yes, each thread can have its own stack in multithreaded programming. This is because each thread requires its own stack to maintain the state of its local variables and method calls.
97. What is the lock interface? Why is it better to use a lock interface rather than a synchronized block.?
The Lock
interface provides a more flexible and powerful way to synchronize access to shared resources than using synchronized blocks. An Lock
object can be used to acquire and release locks, and it allows for more advanced features such as fairness, incorruptibility, and timeout.
98. What is a semaphore?
A semaphore is a synchronization construct that allows a fixed number of threads to access a shared resource at the same time. A semaphore maintains a count of the number of available resources and provides methods for acquiring and releasing resources.
99. What is busy spinning?
Busy spinning is a technique in which a thread repeatedly checks a shared variable in a loop without yielding the processor or blocking. This technique is typically used in situations where the expected wait time for a shared resource is very short, and the cost of switching between threads is high.
100. What is a shutdown hook?
A shutdown hook is a thread in Java that is registered with the JVM to be executed when the JVM is about to shut down. A shutdown hook can be used to perform cleanup tasks, such as releasing resources and closing connections, before the program exits.
The article titled Top 100 Multithreading Interview Questions and Answers provides a comprehensive guide to Multithreading Technical Interview Questions, covering everything from installation basics to advanced concepts. It is a valuable resource for job seekers who want to prepare for Multithreading technical interviews. To gain access to more valuable insights and information, you can follow us at freshersnow.com.