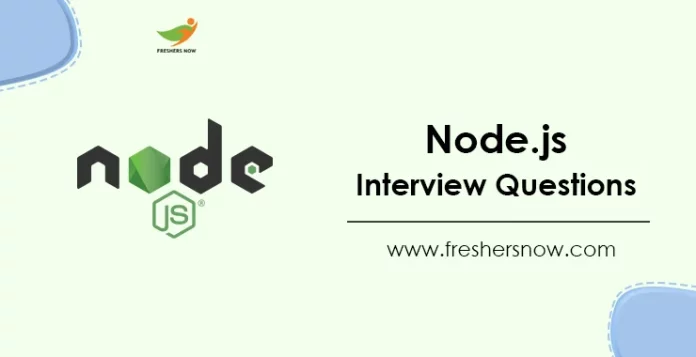
Node.js Interview Questions and Answers: Are you preparing for a Node.js technical interview? Whether you’re a fresh graduate or an experienced developer, having a good grasp of Node.js Interview Questions and Answers is essential to ace the interview process. This article will provide you with the top 100 Node.js interview questions and answers, including the latest Node.js interview questions.
★★ Latest Technical Interview Questions ★★
Latest Node.js Interview Questions
The Node.js Interview Questions for Freshers covered in this article range from fundamental concepts to advanced topics in Node.js, making it a comprehensive resource for Node.js technical interview questions. So, whether you’re a fresher or an experienced developer, read on to prepare yourself for the Node.js technical interview.
Top 100 Node.js Interview Questions and Answers
1. What is Node.js and what are its advantages?
Node.js is an open-source, cross-platform JavaScript runtime environment used for developing server-side applications. Its advantages include:
- Fast and scalable performance due to its non-blocking, event-driven I/O model.
- Large and active developer community, making it easy to find support and libraries.
- Ability to easily create real-time applications, such as chat apps, using WebSockets.
- Ability to share code between the server and client sides of an application, increasing code reuse and reducing duplication.
2. How does Node.js differ from other server-side languages?
Node.js differs from other server-side languages in the following ways:
- It is based on JavaScript, a language that is typically used for client-side scripting, which means that developers can write both the server and client-side code in the same language.
- It uses an event-driven, non-blocking I/O model, which makes it highly scalable and efficient in handling large numbers of concurrent connections.
- It is lightweight and fast, making it ideal for building real-time applications.
3. What is the syntax for declaring a variable in Node.js using the var keyword?
The syntax for declaring a variable using the var keyword is:
var variableName = value; |
4. What is the difference between the require() function and import statement in Node.js?
require() | import statement |
---|---|
Used to load modules in CommonJS format | Used to load modules in ES6 format |
Synchronous, blocking call | Asynchronous, non-blocking call |
Can be called anywhere in the code | Must be called at the top level of the module |
Returns an object with module.exports as a property | Returns a binding to the exported module |
Can load a module dynamically using a variable | Cannot load a module dynamically using a variable |
Can load a module with a relative or absolute path | Can only load a module with a relative path |
Can be used in both front-end and back-end JavaScript | Currently not supported in all browsers, primarily used in back-end JavaScript |
5. What is NPM and why is it used with Node.js?
NPM (Node Package Manager) is a package manager for Node.js that allows developers to easily manage and share packages (libraries, tools, and other code) with other developers. It is used with Node.js because:
- It simplifies the process of installing and managing third-party packages and dependencies.
- It helps prevent version conflicts and ensures that all required dependencies are installed.
- It makes it easy to publish and share your own packages with other developers.
6. What is an event-loop in Node JS?
The event-loop utilizes a queue and listener to handle anything that is asynchronous. A diagram can aid in understanding the concept.
In situations where an asynchronous function must be executed or input/output is required, the main thread dispatches it to a separate thread, enabling V8 to continue executing the primary code. The event loop encompasses various stages with specific responsibilities, including timers, pending callbacks, idle or prepare, poll, check, and close callbacks, each with its own first-in-first-out queue. Additionally, during each iteration, it examines asynchronous input/output or timers and gracefully terminates if none exist.
7. What are the different types of modules in Node.js?
There are three types of modules in Node.js:
- Core Modules: built-in modules that provide basic functionality such as file system I/O, networking, and cryptography.
- Local Modules: modules created by the developer that can be reused within the application.
- Third-Party Modules: modules created by other developers and made available through NPM.
8. How do you declare a constant in Node.js using the const keyword?
The syntax for declaring a constant using the const keyword is:
const constantName = value; |
9. How can you include external libraries in a Node.js application?
External libraries can be included in a Node.js application using NPM. Developers can simply add the package name to the package.json file or use the command “npm install [package-name]” to download and install the package.
10. How does Node.js handle asynchronous operations?
Node.js uses an event-driven, non-blocking I/O model to handle asynchronous operations. It uses callbacks to notify the calling code when an operation is complete, allowing it to continue processing other requests while waiting for the operation to complete.
11. Can you explain the concept of event-driven programming in Node.js?
Event-driven programming is a programming paradigm where the flow of a program is determined by events or signals that occur within the system. In Node.js, this means that code is executed in response to events such as user input, file system events, or network requests. Node.js uses the EventEmitter module to facilitate event-driven programming.
12. What is the syntax for a function declaration in Node.js?
The syntax for a function declaration is:
function functionName(parameters) { // function body } |
13. What is a callback function in Node.js?
A callback function in Node.js is a function that is passed as an argument to another function and is executed when the first function completes. It is commonly used to handle asynchronous operations in Node.js.
14. Enhancing Node.js performance through clustering.
By default, Node.js applications operate on a single processor, which implies that they cannot utilize a multi-core system. However, the cluster mode allows for the launch of several node.js processes, creating multiple instances of the event loop. When employing cluster in a Node.js application, multiple node.js processes are created in the background, accompanied by a parent process referred to as the cluster manager, which is responsible for overseeing the health of each instance of the application.
15. How can you debug a Node.js application?
Debugging a Node.js application can be done using various tools and techniques. Here are a few options:
- Console.log(): One of the easiest ways to debug a Node.js application is to use console.log statements. You can add console.log statements throughout your code to output relevant information to the console. This will help you understand the flow of your code and the values of your variables at different points in the execution.
- Node.js Debugger: Node.js comes with a built-in debugger that can be used to debug your application. You can start the debugger by running your application with the –inspect flag. This will start the debugger and allow you to set breakpoints and step through your code.
- Chrome DevTools: Chrome DevTools can be used to debug Node.js applications. You can start your application with the –inspect flag and then open Chrome DevTools. In the Sources tab, you can select your Node.js application and set breakpoints and step through your code.
- Visual Studio Code: Visual Studio Code has built-in support for debugging Node.js applications. You can use the built-in debugger in Visual Studio Code to set breakpoints and step through your code.
- Node.js Profiler: The Node.js profiler can be used to identify performance issues in your application. You can run your application with the –prof flag to generate profiling data. The profiling data can then be analyzed using various tools to identify performance bottlenecks in your code.
16. What is the difference between module.exports and exports in Node.js?
module.exports |
exports |
---|---|
A reference to the object that is actually returned as the result of a require() call |
An alias for module.exports |
Can be assigned to any value, including a function or an object | Can only be assigned to an object |
Overrides the default behavior of exports |
Cannot override the default behavior of module.exports |
Can be used to expose a single function or object from a module | Used to expose multiple properties or methods from a module |
Allows for more flexibility in defining the exports of a module | Provides a simpler interface for defining exports |
17. What are streams in Node.js?
- Streams are a way to handle reading and writing data in Node.js.
- They allow for handling large amounts of data without requiring a lot of memory.
- Streams are classified into readable, writable, and duplex streams.
- Readable streams allow reading data from a source.
- Writable streams allow writing data to a destination.
- Duplex streams allow both reading and writing data.
18. How do you define a function using the arrow function syntax in Node.js?
The syntax for defining a function using the arrow function syntax is:
const functionName = (parameters) => { // function body }; |
19. What is a thread pool and which library handles it in Node.js
Management of the thread pool is facilitated by the libuv library, a cross-platform C library that offers assistance for concurrency, networking, and file system operations that rely on asynchronous I/O.
20. How can you implement file handling in Node.js?
- Node.js provides built-in modules for file handling, such as fs and path.
- To read a file, use fs.readFile() or fs.readFileSync() methods.
- To write to a file, use fs.writeFile() or fs.appendFile() methods.
- To delete a file, use fs.unlink() method.
- To create a directory, use fs.mkdir() method.
21. Can you explain the difference between process.nextTick() and setImmediate()?
- process.nextTick() executes a callback function immediately after the current operation is completed and before the event loop continues.
- setImmediate() executes a callback function in the next iteration of the event loop after I/O events callbacks and before timers.
22. What is middleware in Node.js?
- Middleware is a function that is executed in the request-response cycle of an application.
- It can modify the request or response objects, end the request-response cycle, or call the next middleware function in the stack.
- Middleware can be used for authentication, logging, error handling, etc.
23. How can you handle errors in Node.js?
- Node.js provides try-catch blocks for handling errors synchronously.
- For asynchronous code, use callback functions with an error object as the first parameter.
- Use the built-in event ‘error’ to handle errors in streams.
- Use the third-party module like ‘express-validator’ for validating user input.
24. What is the difference between the readFileSync() and readFile() functions in Node.js?
readFileSync() | readFile() |
---|---|
Synchronous function | Asynchronous function |
Blocks the execution of the program until the file is read | Does not block the execution of the program |
Returns the contents of the file as a string or buffer | Uses a callback function to return the contents of the file |
Throws an error if there is a problem reading the file | Calls the callback function with an error if there is a problem reading the file |
Can only read files on the local filesystem | Can read files on the local filesystem as well as remote filesystems and HTTP servers |
Can be easier to use for small files or simple scripts | Should be used for large files or in situations where the program needs to continue executing while the file is being read |
25. What is the syntax for a conditional statement (if statement) in Node.js?
The syntax for a conditional statement in Node.js is:
if (condition) { // code to execute if condition is true } |
26. How can you handle authentication in Node.js?
- Passport is a popular authentication middleware for Node.js.
- It supports various authentication strategies such as local, OAuth, and OpenID.
- The user’s credentials are verified, and if valid, a session is created for the user.
- The session is maintained using cookies or tokens.
27. Can you explain the concept of clustering in Node.js?
- Clustering is a way to take advantage of multi-core CPUs in Node.js applications.
- It creates child processes, each running on a separate core, to handle incoming requests.
- The parent process manages the child processes and distributes the incoming requests among them.
28. How can you scale a Node.js application?
- Scaling a Node.js application can be achieved by increasing the number of processes or threads.
- Use load balancers to distribute incoming requests among different processes.
- Use caching to reduce the load on the server.
29. Can you explain the concept of worker threads in Node.js?
- Worker threads are a way to handle CPU-intensive tasks in Node.js applications.
- They create separate threads to perform the task, without blocking the main thread.
- Worker threads communicate with the main thread using messages.
30. How do you declare and initialize an array in Node.js?
The syntax for declaring and initializing an array in Node.js is:
const arrayName = [element1, element2, …]; |
31. What is the difference between process.argv and process.env in Node.js?
process.argv | process.env | |
---|---|---|
Definition | An array containing the command-line arguments passed to Node | An object containing the environment variables available to the Node process |
Usage | To access command-line arguments passed to the Node script | To access environment variables set on the operating system or in the Node script using process.env |
Format | An array of strings | An object with key-value pairs, where the key is the environment variable name and the value is the value |
Example | node script.js arg1 arg2 |
process.env.NODE_ENV or process.env.DB_HOST |
Accessing an element | process.argv[0] for the first argument, process.argv[1] for the second argument, and so on |
process.env.VARIABLE_NAME to access the value of the environment variable with name VARIABLE_NAME |
Modifying the value | Not applicable | You can set or modify the value of an environment variable using process.env.VARIABLE_NAME = value |
32. How would you optimize the performance of a Node.js application?
- Use caching to reduce the load on the server.
- Use a load balancer to distribute the incoming requests among different processes.
- Use compression to reduce the size of the response data.
- Use asynchronous code to avoid blocking the event loop.
- Use a profiling tool to identify and optimize the bottlenecks.
33. What are the different types of testing frameworks available for Node.js?
Node.js has several testing frameworks available, including:
- Mocha
- Jasmine
- Tape
- AVA
- Jest
- NodeTap
- Intern
34. Can you explain the difference between TDD and BDD in Node.js?
- TDD (Test Driven Development) and BDD (Behavior Driven Development) are two approaches to software testing.
- TDD focuses on testing individual units of code, whereas BDD is more focused on testing the behavior of the system as a whole.
- In TDD, tests are written before the code is implemented, while in BDD, tests are written to describe the behavior of the system in a more natural language format.
- Both approaches can be used in Node.js testing, depending on the specific needs of the application.
35. What is Mocha and how can you use it for testing Node.js applications?
- Mocha is a popular testing framework for Node.js applications.
- It allows developers to write tests in a variety of styles, including BDD and TDD.
- Mocha provides a simple and flexible interface for writing and executing tests, and includes features like before/after hooks, timeouts, and test reporters.
- To use Mocha for testing a Node.js application, you would install the package using npm, create test files with the appropriate naming conventions, and run the tests using the Mocha CLI.
36. What is Chai and how can you use it for testing Node.js applications?
- Chai is an assertion library for Node.js applications that works alongside testing frameworks like Mocha.
- It provides a variety of assertion styles, including BDD and TDD, and allows developers to write readable and expressive tests.
- Chai also supports plugins for adding additional assertion styles and integrating with other libraries.
- To use Chai for testing a Node.js application, you would install the package using npm, require the library in your test files, and use the appropriate assertion methods to test your code.
37. What is the syntax for a for loop in Node.js?
The syntax for a for loop in Node.js is:
for (let i = 0; i < array.length; i++) { // code to execute for each iteration } |
38. What is the difference between an event emitter and a callback function in Node.js?
Event Emitter | Callback Function | |
---|---|---|
Definition | An object that emits events that can be listened to | A function that is passed as an argument to another function and is called when complete |
Usage | To handle and trigger events in Node.js applications | To handle the result of an asynchronous operation in Node.js |
Format | An object with methods for adding and removing listeners | A function that takes arguments and returns a value |
Example | const emitter = new EventEmitter(); emitter.on('event', callback); |
fs.readFile('file.txt', (err, data) => { /* handle result */ }); |
Multiple Invocations | Can emit events multiple times | Can be called multiple times |
Execution Order | The order in which listeners are executed is not guaranteed | The order in which callbacks are executed is guaranteed to be sequential |
Handling Errors | Must handle errors with event listeners | Must handle errors using try-catch blocks or by passing them as the first argument |
39. Can you explain the concept of stubbing in Node.js testing?
- Stubbing is a technique used in testing to replace a function or module with a mock implementation.
- This allows developers to test code in isolation, without relying on external dependencies or complex setup.
- In Node.js testing, stubbing is often used to simulate the behavior of external APIs or services.
- Stubs can be created using libraries like Sinon, which provides tools for creating and manipulating stubs, spies, and mocks.
40. How would you handle security issues in a Node.js application?
There are several best practices for handling security issues in Node.js applications, including:
- Keeping dependencies up to date to ensure that security vulnerabilities are addressed.
- Using secure coding practices to prevent common vulnerabilities like cross-site scripting (XSS) and SQL injection.
- Implementing authentication and authorization to control access to sensitive resources.
- Using encryption to protect sensitive data in transit and at rest.
- Regularly auditing and monitoring the application for security vulnerabilities.
41. How would you handle cross-site scripting (XSS) attacks in a Node.js application?
Cross-site scripting (XSS) attacks can be prevented in Node.js applications by:
- Validating and sanitizing user input to prevent malicious code from being executed.
- Using content security policies (CSP) to limit the sources of executable code in a web page.
- Escaping user input before rendering it in a web page.
- Using frameworks and libraries that provide built-in XSS protection, like Express.js.
42. How do you create and use a module in Node.js?
To create a module, you declare functions or variables in a JavaScript file and export them using the module.exports object. To use a module, you import it using the require() function.
43. How would you handle SQL injection attacks in a Node.js application?
SQL injection attacks can be prevented in Node.js applications by:
- Using parameterized queries to ensure that user input is properly escaped and validated before being used in a SQL query.
- Avoiding the use of dynamic SQL queries that concatenate user input
- Using an ORM (Object Relational Mapping) library like Sequelize, which automatically escapes and validates user input.
- Limiting the privileges of database users to only the necessary operations.
44. What is the difference between the find() and filter() functions in Node.js?
find() | filter() | |
---|---|---|
Definition | Returns the first element that satisfies the condition | Returns an array of all elements that satisfy the condition |
Usage | To find a specific element in an array based on a condition | To filter elements from an array based on a condition |
Return Value | Returns the first matching element or undefined if no element is found | Returns an array of all matching elements or an empty array if no element is found |
Callback Function | The callback function should return a Boolean value | The callback function should return a Boolean value |
Execution | Stops after the first matching element is found | Continues to search through the entire array even after matching elements are found |
Example | const arr = [1, 2, 3, 4]; const result = arr.find(item => item > 2); |
const arr = [1, 2, 3, 4]; const result = arr.filter(item => item > 2); |
Use Case | When you only need to find the first matching element | When you need to filter an array based on a specific condition |
45. Can you explain the concept of JSON Web Tokens (JWT) in Node.js?
- JSON Web Tokens (JWTs) are a way of securely transmitting information between parties in JSON format.
- JWTs consist of a header, payload, and signature, and are often used for authentication and authorization in web applications.
- In a Node.js application, JWTs can be generated and verified using libraries like jsonwebtoken or passport-jwt.
- JWTs are often used as an alternative to session-based authentication, as they can be easily transmitted and stored in a client-side cookie or local storage.
46. How can you implement caching in a Node.js application?
- Caching can be implemented in a Node.js application to improve performance and reduce server load.
- One approach is to use an in-memory cache like Redis, which can store key-value pairs and expire them after a certain time interval.
- Another approach is to use a CDN (Content Delivery Network) to cache frequently accessed static assets like images and CSS files.
- Middleware like express-cache-controller can also be used to set cache headers on responses, instructing clients to cache the response for a certain period of time.
47. Can you explain the difference between Redis and Memcached in Node.js?
- Both Redis and Memcached are in-memory data stores, but Redis is more than a cache and has a richer set of data structures.
- Redis can also persist data to disk while Memcached only stores data in memory.
- Redis can perform atomic operations, while Memcached cannot.
- Redis supports replication and high availability, while Memcached does not.
48. What is the syntax for a try-catch block in Node.js?
The syntax for a try-catch block in Node.js is:
try { // code that might throw an error } catch (error) { // code to handle the error } |
49. How would you handle memory leaks in a Node.js application?
- Monitor the application for memory leaks using tools like heapdump, node-memwatch, and Chrome DevTools.
- Analyze heap snapshots to identify potential memory leaks.
- Remove circular references and unused objects.
- Use a garbage collector like the one in Node.js. •
- Implement memory leak detection and prevention in the code.
50. How would you handle a large number of concurrent connections in a Node.js application?
- Use a load balancer to distribute traffic among multiple Node.js servers.
- Implement clustering to take advantage of multiple CPU cores. •
- Optimize the code for better performance, such as reducing the number of database queries.
- Use a caching mechanism like Redis or Memcached to reduce the load on the database.
- Use streams to handle large amounts of data efficiently.
51. What is the difference between a buffer and a stream in Node.js?
Feature | Buffer | Stream |
---|---|---|
Data transfer | Transfers data as a whole | Transfers data in chunks |
Memory allocation | Pre-allocated memory space | Dynamic memory allocation |
Speed | Slower for large amounts of data | Faster for large amounts of data |
Flexibility | Not very flexible | Highly flexible |
Compatibility | Compatible with TCP and file systems | Compatible with all types of input/output (TCP, file systems, HTTP, etc.) |
52. Can you explain the concept of serverless architecture in Node.js?
- Serverless architecture is an approach to building applications without the need for servers or infrastructure management.
- Applications are broken down into functions that are triggered by events, such as HTTP requests or database changes.
- The functions are executed on demand by a cloud provider, like AWS or Google Cloud, and users only pay for the actual usage.
- Node.js is a popular choice for serverless applications due to its event-driven and non-blocking I/O nature.
53. How can you deploy a Node.js application to AWS?
- Use AWS Elastic Beanstalk, which automates the deployment and scaling of Node.js applications.
- Use AWS Lambda, which allows you to run Node.js functions in a serverless environment.
- Use AWS EC2 to create a virtual machine and manually deploy the application.
- Use AWS App Runner, which builds and runs containerized Node.js applications.
54. How do you declare a class in Node.js using the ES6 class syntax?
The syntax for declaring a class using the ES6 class syntax in Node.js is:
class ClassName { constructor(parameters) { // constructor code } methodName(parameters) { // method body } } |
55. Can you explain the concept of microservices in Node.js?
- Microservices is an architectural approach that involves breaking down an application into small, independent services.
- Each service can be developed, tested, and deployed separately.
- Communication between services can be done via APIs or message queues.
- Node.js is a popular choice for developing microservices due to its lightweight and scalable nature.
56. How can you implement a RESTful API in Node.js?
- Use a web framework like Express.js to define routes for HTTP methods like GET, POST, PUT, and DELETE.
- Use middleware functions to handle common tasks like authentication and input validation.
- Return data in JSON format.
- Use HTTP status codes to indicate the outcome of an operation.
- Implement a versioning scheme to support backward compatibility.
57. Can you explain the concept of GraphQL and how it can be used with Node.js?
- GraphQL is a query language for APIs that allows clients to request only the data they need.
- Clients can specify the shape of the data they want using a GraphQL schema.
- Node.js can be used as a GraphQL server, where it receives queries from clients and returns the requested data.
- Popular Node.js libraries for building GraphQL servers include Apollo Server and Express-GraphQL.
58. How would you handle long-running tasks in a Node.js application?
- Use worker threads to offload long-running tasks to separate threads.
- Use child processes to spawn new Node.js processes to handle the task.
- Use a task queue like Bull or RabbitMQ to manage and distribute tasks.
- Use async/await and Prom ises to handle long-running operations without blocking the event loop.
- Use timeouts or other mechanisms to cancel long-running tasks if necessary.
59. Can you explain the difference between Node.js and Express.js?
- Node.js is a runtime environment for executing JavaScript on the server-side, while Express.js is a web application framework built on top of Node.js.
- Node.js provides the core functionality for building server-side applications, such as I/O operations and networking, while Express.js provides a set of tools for building web applications, such as routing and middleware.
- Node.js is low-level and requires more code to build a complete application, while Express.js is higher-level and provides a more structured approach to building web applications.
- Node.js is designed to be flexible and can be used with different web frameworks, while Express.js is tightly coupled with Node.js and is specifically designed for building web applications.
60. What is the difference between setInterval() and setTimeout() in Node.js?
setInterval() | setTimeout() | |
---|---|---|
Functionality | Repeatedly calls a function at a set interval until cleared | Calls a function after a set amount of time |
Usage | setInterval(callback, delay) | setTimeout(callback, delay) |
Return Value | Interval ID, which can be used to stop the interval with clearInterval() | Timeout ID, which can be used to clear the timeout with clearTimeout() |
Behavior with zero delay | Calls the callback function immediately and repeats at the specified interval | Waits for the specified delay before calling the callback function |
Pausing/Stopping | Can be paused or stopped with clearInterval() | Can be cleared with clearTimeout() |
Impact on Event Loop | Keeps the Node.js event loop busy for the duration of the interval | Only keeps the Node.js event loop busy for the duration of the timeout |
Examples | setInterval(() => console.log(‘hello’), 1000); | setTimeout(() => console.log(‘hello’), 1000); |
61. How can you implement routing in a Node.js application using Express.js?
- Express.js is a popular Node.js web application framework that provides a simple and flexible way to implement routing.
- To create a route, you can use the
app.METHOD(PATH, HANDLER)
method, where METHOD is the HTTP request method (e.g. GET, POST), PATH is the URL path, and HANDLER is the function that will be executed when the route is accessed. - You can also use route parameters to create dynamic routes that can handle different input values.
62. What is middleware in Express.js and how can it be used?
- Middleware is a function that can be used in Express.js to modify the request and response objects.
- Middleware functions can be used to perform tasks such as logging, authentication, and error handling.
- To use middleware in Express.js, you can use the
app.use()
method to register the middleware function. - Middleware functions can also be chained together to create a sequence of tasks that need to be performed for a particular route.
63. How can you implement authentication in an Express.js application?
- There are several ways to implement authentication in an Express.js application, including using third-party authentication providers such as Google or Facebook, or implementing your own authentication system.
- To implement your own authentication system, you can use packages such as Passport.js or bcrypt.
- Passport.js is a popular authentication middleware for Node.js that supports a variety of authentication methods, including local username and password authentication, OAuth, and OpenID.
- bcrypt is a password hashing library that can be used to securely store user passwords.
64. Can you explain the difference between passport.js and express-session for authentication in Express.js?
- Passport.js and express-session are both middleware packages that can be used for authentication in Express.js, but they serve different purposes.
- Passport.js is an authentication middleware that provides a flexible and modular authentication framework, while express-session is a session middleware that provides a way to manage user sessions in an Express.js application.
- Passport.js can be used to authenticate users using a variety of methods, including local username and password, OAuth, and OpenID, while express-session is used to manage user sessions by storing session data in a server-side session store.
65. Can you explain the concept of template engines in Express.js?
- Template engines in Express.js provide a way to generate HTML pages dynamically.
- Popular template engines for Express.js include Pug (formerly known as Jade), EJS, and Handlebars.
- Template engines allow you to create reusable components, such as headers and footers, and dynamically generate content based on data retrieved from a database or other sources.
- To use a template engine in Express.js, you can set the
view engine
property to the name of the template engine, and then use theres.render()
method to render a template.
66. How would you handle file uploads in an Express.js application?
- To handle file uploads in an Express.js application, you can use the
multer
middleware package. - Multer provides a way to handle
multipart/form-data
, which is typically used for file uploads. - You can configure Multer to store uploaded files in a specified location on the server, and then access the uploaded files using the
req.file
object in the route handler.
67. What is the difference between a promise and a callback function in Node.js?
Callback Functions | Promises | |
---|---|---|
Definition | A function that is passed as an argument to another function, and is called when the parent function completes. | An object representing the eventual completion or failure of an asynchronous operation. |
Error Handling | Callback functions use the first argument to indicate an error, which can be passed to subsequent functions. Error handling is often handled through conditional statements in the code. | Promises have built-in error handling using .catch() method. If an error occurs, it will propagate down the promise chain until it is caught by a .catch() statement. |
Chaining | Callback functions require nesting, which can lead to callback hell. | Promises are chainable using the .then() method, allowing for cleaner and more readable code. |
Execution Control | Callback functions have less control over execution flow. They execute in the order they are called, and can be prone to errors if they depend on each other. | Promises have better execution control through features such as .all() and .race() , which can execute multiple promises simultaneously and handle their results as a group. |
Usage | Callback functions are commonly used in older Node.js APIs and libraries. | Promises are becoming more commonly used in modern Node.js development, and are especially popular with async/await syntax. |
68. How can you handle cookies and sessions in an Express.js application?
- Cookies and sessions can be used in Express.js to maintain state between client requests.
- To handle cookies, you can use the
cookie-parser
middleware package, which parses cookies from the request headers and makes them available in thereq.cookies
object. - To handle sessions, you can use the
express-session
middleware package, which stores session data on the server and generates a session ID that is stored in a cookie on the client side. - The
express-session
package provides a way to store session data in a variety of session stores, including in-memory, Redis, and MongoDB. - You can configure the
express-session
package with options such as the session secret, session expiration time, and session store.
69. Can you explain the concept of socket.io in Node.js?
- Socket.io is a library for Node.js that provides real-time, bidirectional communication between the server and the client.
- Socket.io works by using WebSockets, a protocol that provides a persistent, low-latency connection between the server and the client.
- Socket.io also provides fallback mechanisms for older browsers that do not support WebSockets, such as long polling and JSONP.
70. How can you implement real-time communication in a Node.js application using socket.io?
- To implement real-time communication in a Node.js application using socket.io, you can use the
socket.io
library to create a WebSocket server. - You can then listen for connection events on the server side and emit events to the client using the
socket.emit()
method. - On the client side, you can connect to the WebSocket server using the
io.connect()
method and listen for events using thesocket.on()
method. - You can also broadcast events to all connected clients using the
socket.broadcast.emit()
method.
71. Can you explain the concept of WebSockets in Node.js?
- WebSockets are a protocol for providing a persistent, low-latency connection between the server and the client.
- WebSockets work by using a handshake process to establish a connection between the client and the server, and then providing a persistent connection over a single TCP socket.
- Unlike traditional HTTP requests, which require a new connection for each request, WebSockets provide a persistent connection that allows for real-time, bidirectional communication between the server and the client.
- WebSockets are supported in most modern browsers, and can be used with Node.js using libraries such as socket.io or ws.
72. How can you use WebSockets for real-time communication in a Node.js application?
- WebSockets are a protocol for bi-directional, real-time communication between clients and servers.
- The ws module in Node.js can be used to implement WebSockets.
- The server listens to incoming WebSocket connections using the ‘ws’ module and establishes a connection.
- The client establishes a WebSocket connection with the server using the WebSocket API.
73. Can you explain the concept of PM2 in Node.js?
- PM2 is a process manager for Node.js applications.
- It provides features like process monitoring, auto-restart, and zero-downtime deployment.
- It can be used to manage multiple Node.js processes on a single machine.
74. How can you use PM2 for process monitoring and management in a Node.js application?
- PM2 can be used to monitor the health and performance of Node.js processes.
- It can restart a process if it crashes or exceeds memory usage limits.
- It can also be used to manage process logs, enable clustering, and deploy a Node.js application with zero downtime.
75. Can you explain the concept of Docker and how it can be used with Node.js?
- Docker is a containerization platform that allows developers to create, deploy, and run applications in a container.
- Containers are lightweight, portable, and can run on any platform that supports Docker.
- Docker can be used to package a Node.js application along with its dependencies and configuration in a container.
76. How can you deploy a Node.js application using Docker?
- Create a Dockerfile that specifies the application’s dependencies and configuration.
- Build a Docker image from the Dockerfile.
- Push the Docker image to a Docker registry like Docker Hub.
- Deploy the Docker image to a server or cloud platform that supports Docker.
77. Can you explain the concept of Kubernetes and how it can be used with Node.js?
- Kubernetes is an open-source container orchestration platform.
- It automates the deployment, scaling, and management of containerized applications.
- Kubernetes can be used to manage a cluster of Docker containers running a Node.js application.
78. How can you implement load balancing in a Node.js application using Kubernetes?
- Kubernetes provides a built-in load balancer called the Kubernetes Service.
- The Service can distribute incoming traffic to multiple replicas of a Node.js application running in different containers.
- Load balancing can improve the performance and availability of the Node.js application.
79. Can you explain the concept of DevOps and how it relates to Node.js development?
- DevOps is a set of practices that combines software development and IT operations.
- DevOps aims to shorten the development lifecycle and improve the quality of software.
- Node.js development can benefit from DevOps practices like automation, continuous integration, and continuous delivery.
80. How would you automate deployment and testing in a Node.js application using DevOps tools?
- Use a tool like Jenkins or Travis CI to automate the deployment and testing process.
- Create a pipeline that builds the Node.js application, runs automated tests, and deploys the application to a server or cloud platform.
- Use a containerization platform like Docker to package the Node.js application and its dependencies.
81. Can you explain the concept of continuous integration and delivery (CI/CD) in Node.js development?
- CI/CD is a set of practices that automate the building, testing, and deployment of software.
- CI/CD aims to reduce the time between writing code and deploying it to production.
- Node.js development can benefit from CI/CD practices like automated testing, version control, and containerization.
82. How would you implement CI/CD in a Node.js application using tools like Jenkins or CircleCI?
- CI/CD (Continuous Integration/Continuous Deployment) is a development practice that involves automating the building, testing, and deployment of software.
- In a Node.js application, CI/CD can be implemented using tools like Jenkins or CircleCI, which can be configured to automatically build and deploy the application when changes are pushed to a repository.
- The process typically involves setting up a pipeline that consists of several stages, including building the application, running tests, and deploying the application to a production environment.
83. Can you explain the concept of serverless computing and how it can be used with Node.js?
- Serverless computing is a cloud computing model where the cloud provider manages the infrastructure required to run an application, and the application developer only needs to worry about writing code.
- In a serverless architecture, the application is broken down into small functions that are executed in response to events triggered by user actions or other services.
- Node.js is a popular choice for serverless computing due to its lightweight and event-driven nature, which makes it well-suited for writing small, focused functions.
84. How can you use AWS Lambda for serverless computing with Node.js?
- AWS Lambda is a serverless computing service provided by Amazon Web Services (AWS) that allows developers to run code in response to events without needing to manage any servers.
- To use AWS Lambda with Node.js, developers can write their functions in JavaScript using the Node.js runtime, and then upload the code to AWS Lambda using the AWS CLI or web console.
- AWS Lambda supports a wide range of event sources, including API Gateway, S3, DynamoDB, and Kinesis, making it a flexible option for building serverless applications.
85. Can you explain the concept of AWS Elastic Beanstalk and how it can be used with Node.js?
- AWS Elastic Beanstalk is a platform as a service (PaaS) offered by AWS that simplifies the process of deploying and scaling web applications.
- Elastic Beanstalk allows developers to upload their code in a variety of languages, including Node.js, and then takes care of provisioning the necessary infrastructure, including EC2 instances, load balancers, and databases.
- Elastic Beanstalk also supports a variety of deployment options, including rolling deployments, blue-green deployments, and canary deployments, allowing developers to update their applications with zero downtime.
86. How would you use Elastic Beanstalk to deploy a Node.js application to AWS?
- To deploy a Node.js application to AWS using Elastic Beanstalk, developers can create an application and environment using the Elastic Beanstalk console or CLI, and then upload their code to the environment.
- Elastic Beanstalk automatically detects the Node.js runtime and installs the necessary dependencies specified in the package.json file.
- Developers can configure environment variables, set up a database, and scale the environment based on traffic using the Elastic Beanstalk console or CLI.
87. Can you explain the concept of AWS API Gateway and how it can be used with Node.js?
- AWS API Gateway is a fully managed service provided by AWS that allows developers to create, publish, and manage APIs for their applications.
- API Gateway can be used with Node.js to create RESTful APIs that can be integrated with other AWS services, such as Lambda or DynamoDB.
- API Gateway provides features such as rate limiting, authentication and authorization, and caching, making it a powerful tool for building scalable and secure APIs.
88. How would you use API Gateway to create and manage APIs for a Node.js application on AWS?
- To create and manage APIs for a Node.js application on AWS using API Gateway, developers can use the AWS console or CLI to create a new API Gateway instance and define the API’s resources and methods.
- The resources and methods can be configured to trigger Lambda functions or other backend services.
- API Gateway provides several features that can be used to secure the API, including OAuth 2.0 authentication, JSON Web Tokens (JWTs), and API keys.
- API Gateway also provides monitoring and logging capabilities, allowing developers to track API usage and troubleshoot issues.
89. Can you explain the concept of server-side rendering in Node.js?
- Server-side rendering is a technique where web pages are generated on the server and then sent to the client as HTML, rather than generating the HTML on the client-side using JavaScript.
- Server-side rendering is commonly used in Node.js applications to improve performance and search engine optimization (SEO).
- Server-side rendering can be achieved using frameworks like Next.js, which provide built-in support for server-side rendering.
90. How can you use server-side rendering with Node.js to improve application performance?
- Server-side rendering can improve application performance by reducing the amount of JavaScript that needs to be downloaded and executed by the client.
- By rendering the initial HTML on the server, the client can start rendering the page immediately, without waiting for the JavaScript to load and execute.
- This can result in faster page load times and a better user experience.
91. Can you explain the concept of client-side rendering in Node.js?
- Client-side rendering is a technique where web pages are generated on the client-side using JavaScript, rather than on the server.
- Client-side rendering is commonly used in single-page applications (SPAs) built with frameworks like React, Vue, or Angular.
- Client-side rendering can provide a more dynamic and interactive user experience, but can also result in slower page load times and poor SEO.
92. How can you use client-side rendering with Node.js to improve application performance?
- Client-side rendering involves rendering the UI on the client-side (browser) instead of the server-side (Node.js).
- This approach can improve application performance by reducing the amount of data that needs to be sent over the network and decreasing server-side load.
- With Node.js, you can use frameworks like React or Angular to build client-side rendered applications.
93. Can you explain the concept of isomorphic rendering in Node.js?
- Isomorphic rendering is the approach of rendering the same code on both the client-side and server-side.
- This approach can improve application performance and user experience by reducing page load times and enabling search engines to index the page content.
- With Node.js, you can use frameworks like Next.js or Nuxt.js to build isomorphic applications.
94. How can you use isomorphic rendering with Node.js to improve application performance?
- Isomorphic rendering with Node.js can improve application performance by reducing the number of requests sent to the server and decreasing the load on the server.
- It can also improve user experience by rendering content on the server-side and enabling search engines to index the page content.
95. Can you explain the concept of load testing and how it can be used to test a Node.js application?
- Load testing is the process of simulating high levels of traffic to test the performance and stability of an application.
- It involves measuring response times, error rates, and resource usage under different load conditions to identify performance bottlenecks and areas for optimization.
- Load testing can be used to ensure that a Node.js application can handle high levels of traffic and maintain performance under heavy loads.
96. How would you use tools like Apache JMeter or LoadRunner to perform load testing on a Node.js application?
- To perform load testing with tools like Apache JMeter or LoadRunner, you would first need to set up a test environment with a representative workload and traffic pattern.
- You would then configure the load testing tool to simulate the desired load and measure the performance of the Node.js application under different conditions.
- You can use the data collected from load testing to identify performance bottlenecks and optimize the application for high traffic conditions.
97. Can you explain the concept of code profiling and how it can be used to optimize a Node.js application?
- Code profiling is the process of analyzing the performance and behavior of an application to identify performance bottlenecks and areas for optimization.
- In a Node.js application, code profiling can involve measuring the execution time, memory usage, and I/O performance of different parts of the application.
- By identifying performance bottlenecks and areas for optimization, code profiling can help improve the overall performance and efficiency of a Node.js application.
98. How would you use tools like Node.js Profiler or Chrome DevTools to perform code profiling on a Node.js application?
- To perform code profiling with tools like Node.js Profiler or Chrome DevTools, you would need to first set up a test environment and configure the profiling tool to collect data on the desired metrics.
- You would then run the Node.js application and analyze the data collected by the profiling tool to identify performance bottlenecks and areas for optimization.
- You can use the insights gained from code profiling to optimize the application code and improve its performance and efficiency.
99. Can you explain the concept of code coverage and how it can be used to ensure high code quality in a Node.js application?
- Code coverage is a measure of how much of your codebase is executed by your automated tests.
- It can be used to ensure that your tests are comprehensive and that all parts of your code are being tested.
- Code coverage is usually expressed as a percentage of lines, functions, or branches covered by tests.
- A high code coverage percentage does not guarantee that your tests are effective, but a low coverage percentage indicates that some parts of your code are not being tested at all.
- Code coverage can be used to identify areas of your code that need additional tests, or to identify parts of your code that are no longer being used and can be removed.
100. How would you use tools like Istanbul or Coveralls to measure code coverage in a Node.js application?
- Install the code coverage tool as a development dependency using npm or Yarn.
- Add a script to your package.json file that runs the code coverage tool and generates a report.
- Run your test suite with the coverage tool enabled to generate the coverage report.
- Review the report to identify areas of your code that need additional tests or that can be removed.
- Use a service like Coveralls to track code coverage over time and to compare coverage between different branches or pull requests.
Mastering the Top 100 Node.js Interview Questions and Answers is crucial for acing technical interviews. Prepare yourself with this comprehensive resource to boost your chances of success. To gain more knowledge, follow us at freshersnow.com.