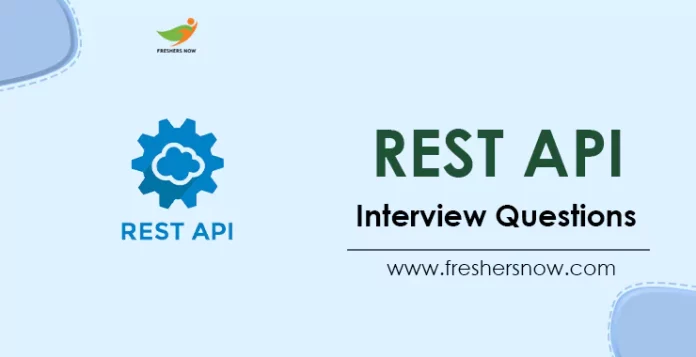
This article features the Top 100 REST API interview questions and answers which are very useful freshers and experienced candidates who are attending for a technical interview.
★★ Latest Technical Interview Questions ★★
Top 100 REST API Interview Questions and Answers
1. Can you explain the concept of resources in RESTful web services?
In RESTful web services, a resource is any piece of information that can be identified using a unique URI (Uniform Resource Identifier). Resources can be anything that can be represented in a digital format, such as text, images, audio, or video. The resource is typically the focal point of the RESTful service, and the RESTful API provides a way to interact with it using HTTP methods such as GET, POST, PUT, and DELETE.
2. What are the HTTP Methods?
- GET: Used to fetch details from the server in a read-only operation.
- POST: Used to create new resources on the server.
- PUT: Used to update or replace existing resources on the server.
- DELETE: Used to delete resources from the server.
- PATCH: Used to modify resources on the server.
- OPTIONS: Used to fetch the list of supported options of resources on the server.
3. What is HATEOAS and how is it used in RESTful web services?
HATEOAS stands for “Hypermedia As The Engine Of Application State”. It’s a principle of RESTful web services that suggests that the service should provide links to related resources along with the response data. This means that clients can discover the available actions they can take by following links in the response, rather than being hard-coded to a specific set of API endpoints. This makes the API more flexible and easier to evolve over time.
4. What is the difference between URI and URL?
URI (Uniform Resource Identifier) is a string of characters that identifies a name or a resource on the Internet, while URL (Uniform Resource Locator) is a type of URI that specifies the network location of a resource and how to access it. In other words, a URL is a type of URI that includes the protocol (HTTP, FTP, etc.), the domain name, and the path to the resource.
5. How do you handle errors in RESTful web services?
RESTful web services use HTTP status codes to communicate the outcome of a request to the client. The server can respond with an appropriate status code (such as 200 for success, 404 for resource not found, 500 for server error, etc.) and an error message if necessary. It’s important to provide clear and concise error messages to help clients understand the problem and take appropriate action.
6. Can you explain the concept of versioning in RESTful web services?
Versioning is the practice of maintaining different versions of an API to support evolving requirements and changes. In RESTful web services, versioning can be implemented using URI or header parameters. URI versioning involves adding a version number to the API endpoint, while header versioning involves adding a custom header to the HTTP request.
7. Can you tell what constitutes the core components of HTTP requests?
- Method/ Verb: Indicates the type of operation to be performed such as GET, PUT, POST, DELETE, etc.
- URI: Used to uniquely identify the resources on the server.
- HTTP Version: Indicates the version of the HTTP protocol being used, such as HTTP v1.1.
- Request Header: Contains metadata details of the request, including client type, supported content format, message format, cache settings, etc.
- Request Body: Contains the actual message content to be sent to the server.
8. What is the difference between versioning through URI and through headers?
URI versioning involves adding a version number to the API endpoint, while header versioning involves adding a custom header to the HTTP request. URI versioning is easier to implement, but it can clutter the API endpoint and make it less readable. Header versioning is more flexible and allows for more granular control, but it requires more complex server-side logic to handle.
9. What is content negotiation in RESTful web services?
Content negotiation is the process of selecting the appropriate representation of a resource based on the client’s request. Clients can specify their preferred representation format (such as JSON, XML, or HTML) using the Accept header in the HTTP request. The server can then return the resource in the requested format if it’s available.
10. What constitutes the core components of HTTP Response?
- Response Status Code: Indicates the server’s response status code for the requested resource, such as 400 for a client-side error or 200 for a successful response.
- HTTP Version: Indicates the version of the HTTP protocol being used in the server’s response.
- Response Header: Contains metadata details of the response message, including the content length, content type, response date, server type, etc.
- Response Body: Contains the actual resource/message returned from the server.
11. What are the different media types used in content negotiation?
Media types are identifiers used to indicate the format of a resource representation. Common media types used in content negotiation include JSON (application/JSON), XML (application/xml), and HTML (text/html), among others.
12. What is the concept of statelessness in REST?
In the REST architecture, the server is designed to be stateless, which means that it does not maintain any information about the client’s state. Instead, the client provides the necessary context to the server, which is used to process the client’s request. The session on the server is identified by the session identifier that is sent by the client.
13. How do you implement caching in RESTful web services?
Caching is the process of storing frequently accessed resources in memory or on disk to improve performance and reduce server load. RESTful web services can use HTTP caching headers (such as Cache-Control and ETag) to indicate whether a response can be cached and for how long.
14. Can you explain the concept of conditional requests in RESTful web services?
Conditional requests are a way for clients to reduce the amount of data transferred between the server and the client by sending a request only if a certain condition is met. The condition can be based on the resource’s state, modification time, or other metadata. The server can then respond with a 304 Not Modified status code if the resource hasn’t changed since the last request.
15. What is the difference between a GET request and a POST request in REST API?
GET Request | POST Request |
---|---|
Used for retrieving data from the server. | Used for submitting data to the server. |
The parameters are sent in the URL. | The parameters are sent in the request body. |
It is a safe and idempotent operation, meaning that the same request can be sent multiple times without changing the state of the server. | It is not idempotent, meaning that sending the same request multiple times can result in multiple operations being performed on the server. |
GET requests can be cached. | POST requests are not cached. |
16. How do you secure a RESTful API?
Securing a RESTful API involves implementing authentication and authorization mechanisms, using HTTPS encryption to protect data in transit, and validating input to prevent attacks such as injection and cross-site scripting.
17. What are the different types of authentication mechanisms used in RESTful web services?
The different types of authentication mechanisms used in RESTful web services include basic authentication, token-based authentication, and OAuth.
18. Can you explain the concept of token-based authentication?
Token-based authentication is a mechanism for authenticating users by exchanging a token for a set of permissions. The token can be issued by the server after the user logs in and is stored on the client side. The client includes the token in subsequent requests to access protected resources.
19. How do you handle CORS in RESTful web services?
- CORS (Cross-Origin Resource Sharing) is a security mechanism implemented in web browsers to restrict cross-domain requests.
- To enable CORS in RESTful web services, you need to set the Access-Control-Allow-Origin header in the response to allow access from specific domains.
- You can also set other headers, such as Access-Control-Allow-Methods and Access-Control-Allow-Headers, to restrict the allowed HTTP methods and headers for the cross-origin request.
20. How does a PUT request differ from a PATCH request in REST API?
PUT Request | PATCH Request |
---|---|
Used to update a resource on the server. | Used to update part of a resource on the server. |
Requires the entire resource to be sent in the request body. | Requires only the changes to be sent in the request body. |
If the resource already exists, it is replaced entirely. | If the resource already exists, only the specified fields are updated. |
PUT requests are idempotent, meaning that the same request can be sent multiple times without changing the state of the server. | PATCH requests are not idempotent, meaning that sending the same request multiple times can result in different states of the server. |
21. Can you explain the concept of rate limiting in RESTful web services?
- Rate limiting is a technique used to limit the number of requests a client can make to a RESTful API within a certain time frame.
- It helps to prevent abuse of the API by limiting the number of requests made by a single client.
- Rate limiting can be based on various factors, such as the IP address of the client, the API endpoint being accessed, or the user account making the request.
22. How do you implement rate limiting in RESTful web services?
Rate limiting can be implemented using various tools and techniques, such as:
- Using a middleware or library that provides rate-limiting functionality.
- Using a reverse proxy or API gateway that supports rate limiting.
- Manually implementing rate limiting logic in the application code.
23 What is API throttling and how is it used in RESTful web services?
- API throttling is similar to rate limiting, but it limits the rate at which an API can be accessed by a single client or user.
- It helps to prevent API abuse by limiting the number of requests made by a single client within a certain time frame.
- API throttling can be used in combination with rate limiting to provide additional protection against abuse.
24. Can you explain the concept of load balancing in RESTful web services?
- Load balancing is the process of distributing incoming network traffic across multiple servers or instances.
- It helps to improve the availability, scalability, and reliability of web applications and APIs.
- Load balancing can be performed using various techniques, such as round-robin, least connections, IP hash, and session persistence.
25. How do you implement load balancing in RESTful web services?
- Load balancing can be implemented using various tools and techniques, such as:
- Using a load balancer appliance or software.
- Using a cloud-based load balancer service.
- Manually implementing load balancing logic in the application code.
26. What is service discovery and how is it used in RESTful web services?
- Service discovery is the process of automatically discovering and registering network services in a distributed system.
- It helps to simplify the management and scaling of microservices and containerized applications.
- Service discovery can be performed using various tools and techniques, such as DNS-based discovery, client-side discovery, and server-side discovery.
27. Can you explain the concept of API gateway and how is it used in RESTful web services?
- An API gateway is a reverse proxy that acts as an entry point for a set of microservices or APIs.
- It helps to simplify the architecture, management, and security of a distributed system.
- An API gateway can perform various tasks, such as routing, load balancing, rate limiting, authentication, and monitoring.
29. What distinguishes a DELETE request from a HEAD request in REST API?
DELETE Request | HEAD Request |
---|---|
Used to delete a resource on the server. | Used to retrieve metadata about a resource on the server. |
The response body is optional. | The response body is not included. |
A successful DELETE request results in the resource being removed from the server. | A successful HEAD request results in the server returning metadata about the requested resource. |
DELETE requests are not idempotent, meaning that sending the same request multiple times can result in different states of the server. | HEAD requests are idempotent, meaning that the same request can be sent multiple times without changing the state of the server. |
30. What is the difference between API gateway and reverse proxy?
- A reverse proxy is a server that sits between a client and a set of servers, forwarding client requests to the appropriate server.
- An API gateway is a reverse proxy that specifically handles API traffic and provides additional functionality, such as routing, load balancing, and security.
31. How do you implement token-based authentication in RESTful web services?
To implement token-based authentication in RESTful web services, the server must generate and issue tokens, validate tokens on subsequent requests, and enforce authorization based on the token’s permissions.
32. What is JWT and how is it used in RESTful web services?
JWT (JSON Web Token) is a format for encoding tokens as JSON objects that can be used for authentication and authorization in RESTful web services. JWT tokens include a set of claims (such as user ID and expiration time) that are signed with a secret key to prevent tampering.
33. Can you explain the concept of OAuth and how is it used in RESTful web services?
OAuth is an authorization framework that allows third-party applications to access protected resources on behalf of a user. In RESTful web services, OAuth is typically used to authenticate users and issue access tokens that can be used to access protected resources.
34. What is CORS and how does it work?
CORS (Cross-Origin Resource Sharing) is a security mechanism that allows web browsers to make cross-origin HTTP requests. It works by adding HTTP headers to the response that indicate which origins are allowed to access the resource.
35. What is the difference between a PUT and a PATCH request in REST API?
PUT is used to update an entire resource, while PATCH is used to update specific fields of a resource.
36. What is the difference between a query parameter and a path parameter in REST API?
Query Parameter | Path Parameter |
---|---|
Used to filter and sort data. | Used to identify a specific resource. |
Appears after the “?” in the URL. | Appears as a part of the URL path. |
Not required for the URL to function. | Required for the URL to function. |
Can be optional. | Must be included in the URL. |
36. How do you handle authentication and authorization in a REST API?
Authentication is typically handled using tokens or API keys, while authorization is managed using role-based access control or other similar mechanisms.
37. What is the difference between a GET and a POST request in REST API?
GET is used to retrieve data from a resource, while POST is used to create new resources.
38. How do you handle errors in a REST API?
Errors should be returned with appropriate HTTP status codes (e.g. 404 for not found, 500 for server errors), and accompanied by a message explaining the error.
39. How do you version a REST API?
Versioning can be achieved through URL versioning, header versioning, or query parameter versioning.
40. What are the benefits of using RESTful web services?
RESTful web services are flexible, scalable, and platform-independent, and they can be easily integrated with other systems.
41. How do you ensure the security of a REST API?
Security can be ensured through authentication, encryption, and other security measures such as firewalls and intrusion detection systems.
42. What is the difference between SOAP and REST?
SOAP is a protocol that uses XML to send messages, while REST uses HTTP to transfer data in a lightweight and flexible manner.
43. How does the use of HTTP status codes differ between a successful and failed request in REST API?
Successful Request | Failed Request |
---|---|
HTTP status code between 200 and 299. | HTTP status code outside the range of 200 to 299. |
The response body contains the requested resource or a message indicating success. | The response body contains an error message indicating the reason for the failure. |
The client can use the response for further processing. | The client cannot use the response for further processing. |
The request was successful and has been completed. | The request was not successful and has not been completed |
44. How do you handle pagination in a REST API?
Pagination can be achieved using query parameters such as offset and limit, or by using links to the next and previous pages.
45. What is HATEOAS and why is it important in REST API?
HATEOAS (Hypermedia as the Engine of Application State) is a constraint of REST that requires that a client can discover all available actions and resources through hypermedia links. This helps to decouple the client from the server and allows for more flexible and scalable systems.
46. Can you explain the difference between CORS and CSRF?
CORS is a mechanism that allows web browsers to make cross-origin HTTP requests, while CSRF (Cross-Site Request Forgery) is an attack that tricks a user into executing unwanted actions on a web application on behalf of the attacker. CORS is a security feature, while CSRF is a security vulnerability.
47. What is REST API and how does it work?
REST (Representational State Transfer) API is an architectural style for building web services that are scalable, flexible, and loosely coupled. It uses HTTP protocols for communication between client and server and provides resources that can be accessed using standard HTTP methods like GET, POST, PUT, PATCH, and DELETE.
48. Can you explain the difference between REST and SOAP?
REST and SOAP are two different architectural styles used for building web services. REST is lightweight and uses simple HTTP protocols, while SOAP is heavier and uses XML messaging format and other protocols like SOAP, WSDL, and UDDI.
49. What are the key features of RESTful web services?
The key features of RESTful web services include being stateless, using standard HTTP methods, providing resources that can be accessed through unique URIs, supporting multiple data formats like JSON and XML, and being scalable and flexible.
50. How do you design a RESTful API?
To design a RESTful API, you need to identify the resources that you want to expose, define the URIs for each resource, choose the appropriate HTTP methods for each resource, determine the data format, and consider the security and scalability of the API.
51. Can you explain the HTTP methods used in RESTful web services?
HTTP methods used in RESTful web services are:
- GET: used to retrieve a resource
- POST: used to create a new resource
- PUT: used to update an existing resource
- PATCH: used to update a part of an existing resource
- DELETE: used to delete a resource
52. What is the difference between the GET and POST methods?
The GET method is used to retrieve data from the server, while the POST method is used to submit data to the server to create a new resource.
53. What is the difference between the PUT and PATCH methods?
The PUT method is used to replace an entire resource with a new one, while the PATCH method is used to update a part of an existing resource.
54. What is the difference between DELETE and TRUNCATE methods?
The DELETE method is used to delete a specific resource, while the TRUNCATE method is used to delete all the records in a database table.
55. What are the benefits of using RESTful web services?
The benefits of using RESTful web services are:
- Simplicity and ease of use
- Scalability and flexibility
- Platform and language independence
- Reduced bandwidth and improved performance
- Better caching support
- Support for multiple data formats
- Reduced coupling between client and server
- Improved security and reliability
56. How do you implement logging in to RESTful web services?
- Logging can be implemented using various logging frameworks and libraries, such as 4j, Logback, and SLF4J.
- Logging can be used to capture important events and errors in the application, as well as performance metrics and request/response data.
- Logging can be configured to write log messages to various destinations, such as a file, console, or remote server.
- Logging can also be configured to capture different levels of severity, such as DEBUG, INFO, WARN, and ERROR.
57. How does a stateless API differ from a stateful API in REST API?
Stateless API | Stateful API |
---|---|
The server does not maintain any session information between requests. | The server maintains session information between requests. |
Each request is independent and self-contained. | Each request depends on the state of previous requests. |
The client must include all necessary information in each request. | The client may not need to include all necessary information in each request. |
Scaling is easier as no session information needs to be maintained. | Scaling can be more difficult as session information needs to be maintained. |
58. Can you explain the concept of tracing in RESTful web services?
- Tracing is the process of tracking a request as it passes through a distributed system.
- It helps to diagnose performance and functional issues in a distributed system.
- Tracing can be performed using various tracing frameworks and libraries, such as OpenTelemetry, Zipkin, and Jaeger.
- Tracing can capture important information, such as request IDs, service names, timestamps, and request/response data.
59. How do you implement tracing in RESTful web services?
Tracing in RESTful web services involves adding a unique identifier to each request as it travels through the various components of the system. This identifier, also known as a trace ID, allows developers to track a request’s path through the system and identify any issues or bottlenecks that may occur. To implement tracing, developers can use tools like OpenTracing or Zipkin, which provide APIs for generating and propagating trace IDs.
60. Can you explain the concept of observability in RESTful web services?
Observability is the ability to understand and monitor the behavior of a system from the outside. In the context of RESTful web services, observability involves collecting and analyzing data about requests, responses, and other metrics to gain insight into how the system is performing. This can include data such as response times, error rates, and resource utilization. Observability is crucial for ensuring the reliability and availability of RESTful web services.
61. How do you implement observability in RESTful web services?
To implement observability in RESTful web services, developers can use tools such as monitoring and logging frameworks, which provide visibility into system performance and behavior. These tools can capture data about requests, responses, and other metrics, which can then be analyzed and visualized using tools such as Grafana or Kibana. Additionally, developers can use distributed tracing frameworks such as Jaeger or Zipkin to gain insight into how requests are being handled across multiple services.
62. What is the difference between synchronous and asynchronous communication in RESTful web services?
Synchronous communication in RESTful web services involves a client sending a request to a server and waiting for a response before proceeding. Asynchronous communication, on the other hand, involves a client sending a request to a server and continuing to perform other tasks while waiting for a response. In RESTful web services, asynchronous communication is often used for long-running or resource-intensive operations, where synchronous communication would result in long wait times for the client.
63. What is the difference between versioning an API via URI and via media type in REST API?
Versioning via URI | Versioning via Media Type |
---|---|
The version number is included in the URI. | The version number is included in the media type. |
Example: /api/v1/users | Example: application/vnd.company.v1+json |
The URI changes with each version, which can cause issues with caching and bookmarks. | The URI remains the same, and the media type indicates the version. |
The URI can become cluttered with version numbers, making it harder to read. | The media type can become lengthy, making it harder to read. |
64. How do you implement asynchronous communication in RESTful web services?
To implement asynchronous communication in RESTful web services, developers can use techniques such as message queues, which allow clients to send requests to a server and receive a response at a later time. Additionally, developers can use frameworks such as ReactiveX or Spring WebFlux, which provide support for asynchronous programming models.
65. What are the different message formats used in RESTful web services?
The most common message format used in RESTful web services is JSON (JavaScript Object Notation), which is a lightweight, text-based format that is easy to read and parse. Other formats such as XML and YAML are also used, although less frequently.
66. Can you explain the concept of message brokers and how they are used in RESTful web services?
Message brokers are middleware components that allow messages to be sent and received between different systems or services. In RESTful web services, message brokers are often used to implement asynchronous communication, where a client sends a request to a broker, which then routes the request to the appropriate service. Popular message brokers used in RESTful web services include Apache Kafka and RabbitMQ.
67. What is the difference between RESTful web services and microservices?
RESTful web services and microservices are both architectural styles for building distributed systems, but they differ in their approach to service design. RESTful web services focus on exposing resources over HTTP using a standard set of operations (GET, POST, PUT, DELETE), while microservices emphasize the development of small, loosely coupled services that can be developed and deployed independently.
68. Can you explain the concept of containerization and how it is used in RESTful web services?
Containerization is the process of packaging an application and its dependencies in a container, which can be deployed on any infrastructure that supports containerization. Containers provide a lightweight and portable runtime environment, isolating the application from the underlying infrastructure. RESTful web services can be packaged in containers and deployed on container platforms such as Docker and Kubernetes. Containerization allows for easier deployment, scaling, and management of RESTful web services.
69. What is the difference between RESTful web services and GraphQL?
RESTful web services and GraphQL are both used to build APIs for web applications. The main difference between the two is that RESTful APIs use a fixed set of endpoints and data structures, while GraphQL allows clients to request specific data structures and only receive the data they need. RESTful APIs are better suited for simple use cases where a fixed data structure is sufficient, while GraphQL is better suited for complex use cases where clients need more control over the data they receive.
70. Can you explain the concept of webhooks and how they are used in RESTful web services?
Webhooks are a way for RESTful web services to notify clients of events that occur on the server. When an event occurs, the server sends an HTTP request to a URL specified by the client. This allows the client to receive real-time updates without having to constantly poll the server for changes.
71. How does the use of caching differ between a client-side cache and a server-side cache in REST API?
Client-side Cache | Server-side Cache |
---|---|
Caching is done on the client side, typically in the user’s web browser. | Caching is done on the server side, typically in a cache layer between the API and the database. |
Reduces the number of requests made to the server, improving performance. | Reduces the load on the server, improving performance. |
This can cause stale data to be displayed if the cache is not updated frequently enough. | This can cause stale data to be returned if the cache is not updated frequently enough. |
Can be cleared or updated by the user, such as by clearing their browser cache. | Can be cleared or updated by the server, such as by invalidating the cache when data is updated. |
72. How do you implement webhooks in RESTful web services?
To implement webhooks in RESTful web services, the server needs to provide a way for clients to register a URL to receive webhook notifications. When an event occurs, the server sends an HTTP POST request to the registered URL, including any relevant data in the request body. The client can then process the data and take appropriate action.
73. What are the best practices for designing a RESTful API?
Some best practices for designing a RESTful API include using HTTP methods correctly, using resource URIs that are easy to understand and follow a consistent pattern, providing clear and consistent error responses, using pagination for large data sets, and versioning the API to avoid breaking changes.
74. How do you handle versioning changes in a RESTful API?
Versioning in RESTful APIs can be done using URL versioning, header versioning, or media type versioning. In URL versioning, the version number is included in the URI path. In header versioning, the version number is included in a custom header. In media-type versioning, the version number is included in the media type of the response. When making changes to the API, it is important to communicate these changes to users and provide a clear upgrade path.
75. What is the difference between JSON and XML in RESTful web services?
JSON and XML are both data interchange formats used in RESTful web services. JSON is lightweight and easier to read and parse than XML, making it a popular choice for web applications. XML is more verbose and has more complex data types, making it better suited for more complex use cases.
76. Can you explain the concept of hypermedia in RESTful web services?
Hypermedia is the concept of adding links to resources in response to a RESTful API. These links provide clients with a way to navigate the API and discover new resources without prior knowledge of the API structure. Hypermedia allows for more flexible and dynamic APIs, as the structure of the API can change over time without breaking client applications.
77. What are the different tools used for testing RESTful web services?
There are many tools available for testing RESTful web services, including:
- Postman: A popular API testing tool that allows you to send HTTP requests and inspect responses.
- curl: A command-line tool for sending HTTP requests and receiving responses.
- SoapUI: A testing tool specifically designed for web services, including RESTful APIs.
- JMeter: A load testing tool that can be used to test the performance and scalability of RESTful APIs.
- Swagger: A tool for designing, building, and documenting RESTful APIs.
78. How do you perform load testing for a RESTful API?
Load testing is the process of testing an application or system under simulated high-load conditions to evaluate its performance and identify potential issues. To perform load testing for a RESTful API, you can use tools such as JMeter or Gatling to simulate a large number of concurrent users or requests. You can also use these tools to measure the response time and throughput of the API under different load conditions. It is important to perform load testing regularly to ensure that the API can handle the expected traffic and load.
79. What distinguishes a RESTful API from a SOAP API in REST API?
RESTful API | SOAP API |
---|---|
Uses HTTP methods to perform CRUD (Create, Read, Update, Delete) operations. | Uses a fixed set of operations, such as “get”, “put”, and “delete”. |
Communicates via JSON or XML. | Communicates via XML. |
Is typically more lightweight and easier to implement. | Is more complex and can be harder to implement. |
Is more flexible in terms of the data formats that can be used. | Is less flexible and typically only supports XML. |
80. Can you explain the concept of contract testing in RESTful web services?
Contract testing is a technique used to verify that the consumer and provider of an API agree on the format and content of the messages exchanged between them. In the context of RESTful web services, contract testing involves creating and verifying a contract between the client and server to ensure that the API works as expected.
81. How do you implement contract testing in RESTful web services?
To implement contract testing in RESTful web services, you can use a testing framework like Pact, which allows you to define and verify contracts between the client and server. The process involves the client and server agreeing on a contract that defines the requests and responses of the API. Once the contract is defined, the client can use the contract to generate tests that ensure the API behaves as expected.
82. What are the different tools used for the documentation of RESTful web services?
There are several tools available for documenting RESTful web services, including Swagger, OpenAPI, RAML, and API Blueprint. These tools provide a way to document the API endpoints, parameters, and responses in a standardized format that can be easily understood by developers and other stakeholders.
83. How do you handle versioning changes in API documentation?
When making versioning changes in API documentation, it’s important to clearly indicate which version of the API the documentation refers to. One approach is to use a version number in the API endpoint URL, such as /API/v1/my endpoint. Another approach is to use HTTP headers to indicate the API version.
84. What is the difference between Swagger and OpenAPI?
Swagger is a set of open-source tools that help developers design, build, and document RESTful web services. OpenAPI, on the other hand, is a specification for building APIs that was formerly known as Swagger. The OpenAPI specification defines a standard format for describing RESTful APIs that can be used by tools like Swagger.
85. How do you implement validation for input parameters in a RESTful API?
To implement validation for input parameters in a RESTful API, you can use a validation library like Joi or Validator.js. These libraries allow you to define validation rules for each input parameter and ensure that the data provided by the client meet those rules.
86. Can you explain the concept of pagination in RESTful web services?
Pagination is the process of breaking up a large set of data into smaller, more manageable chunks or pages. In the context of RESTful web services, pagination allows clients to request data in smaller, more manageable chunks rather than getting all the data at once. This helps to reduce the amount of data transferred over the network and improves performance.
87. How do you implement pagination in RESTful web services?
To implement pagination in RESTful web services, you can use query parameters to specify the page size and the page number. For example, you could use the parameters ?page=2&limit=10 to request the second page of data with a limit of 10 items per page.
88. What are the different techniques used for searching in RESTful web services?
There are several techniques used for searching in RESTful web services, including:
- Query parameters: Use query parameters to filter and sort the data returned by the API.
- Full-text search: Use a full-text search engine like Elasticsearch to allow users to search for specific text within the data.
- Custom search endpoints: Create custom endpoints that allow users to search for specific data using custom search criteria.
- Faceted search: Use facets to allow users to refine their search results based on specific attributes of the data.
89. How do you implement search functionality in RESTful web services?
To implement search functionality in RESTful web services, you can use query parameters to pass search criteria to the server. For example, you can use the “q” parameter to pass a search string and use other parameters to specify filters, sorting options, and pagination. The server can then use this information to query the database and return the search results in the response body.
90. Can you explain the concept of web caching in RESTful web services?
Web caching is a technique used to improve the performance of web applications by storing frequently accessed resources in memory or on disk so that they can be served quickly to clients without needing to be generated anew each time. In RESTful web services, caching can be used to cache the response to GET requests for resources that are unlikely to change frequently, such as static content, and can be invalidated or refreshed when the resource is updated.
91. How do you implement web caching in RESTful web services?
To implement web caching in RESTful web services, you can use caching headers in the response to instruct clients and intermediaries on how long they should cache the response, and when they should request a fresh copy of the resource. The most commonly used caching headers are Cache-Control and Expires, which can be set to control the caching behavior of clients and intermediaries.
92. What is the difference between monolithic and microservice architectures?
Monolithic architecture is a software design pattern in which all the components of an application are tightly coupled together and deployed as a single unit, whereas microservice architecture is a design pattern in which an application is composed of loosely coupled and independently deployable services, each of which provides specific functionality.
93. How do you migrate a monolithic application to a microservices architecture?
To migrate a monolithic application to a microservices architecture, you can identify the distinct and independent functionality of the application and break them into smaller, independent services. This requires refactoring the application code and designing a new architecture that supports distributed systems and service communication. You can also gradually migrate parts of the application to microservices over time to minimize disruption.
94. What are the different testing strategies for a RESTful API?
Some of the different testing strategies for a RESTful API include unit testing, integration testing, functional testing, performance testing, security testing, and end-to-end testing. Unit testing involves testing individual functions or components of the API, while integration testing involves testing the interaction between components and services. Functional testing involves testing the API against expected behavior and outcomes, while performance testing involves testing the API’s response time and throughput. Security testing involves testing the API for vulnerabilities and ensuring that it is secure. End-to-end testing involves testing the API in a complete system or application environment.
95. How do you handle backward compatibility in RESTful web services?
To handle backward compatibility in RESTful web services, you can version your API by using the URL or media type to indicate the version number. This allows you to make changes to the API without breaking existing clients that depend on the previous version. You can also use techniques such as feature flags, deprecation warnings, and API gateways to manage the transition to newer versions of the API.
96. What is the role of API documentation in a RESTful web service?
The role of API documentation in a RESTful web service is to provide developers with a clear and complete understanding of the API’s functionality, resources, parameters, and responses. Good documentation helps developers to use the API effectively and efficiently, reduces the time required to integrate the API, and improves the overall quality of the client code.
97. What are the best practices for securing a RESTful API?
Best practices for securing a RESTful API:
- Use HTTPS to encrypt all data transferred between clients and servers.
- Use strong and unique passwords for user authentication, and encourage users to use 2FA (two-factor authentication) to further secure their accounts.
- Implement proper access controls to limit who can access certain resources and operations.
- Use input validation to ensure that data sent to the API is of the expected type and format, and free of malicious code.
- Sanitize any input received from users or third-party sources to prevent SQL injection attacks and other security vulnerabilities.
- Log all API calls and monitor them for suspicious activity or anomalies.
98. How do you implement validation for output parameters in a RESTful API?
- Define the expected format for each output parameter in your API documentation.
- Use JSON Schema or other validation libraries to validate the response data against the expected format.
- Validate that any data returned from your API is not empty or null.
- Use HTTP response codes to indicate any errors or issues with the API response.
- Provide clear error messages in the response body to help clients understand any issues with their API requests.
99. Can you explain the concept of content negotiation in RESTful web services?
Content negotiation is the process of determining the most appropriate content type to return in an API response based on the client’s preferences and capabilities. In a RESTful API, content negotiation is typically done using the Accept and Content-Type headers in the HTTP request and response, respectively.
100. Is it possible to send the payload in the GET and DELETE methods?
No, the payload is not the same as the request parameters. Hence, it is not possible to send payload data through these methods
This article on the Top 100 REST API Interview Questions and Answers offers a thorough introduction to the fundamental concepts and real-world applications of REST API, and REST API Technical Interview Questions for better prepare for their interviews. For more valuable insights, be sure to check out freshersnow.com and stay updated on the latest industry developments.