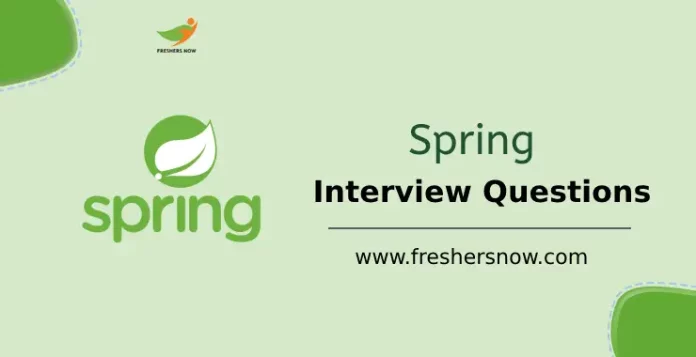
Spring Interview Questions and Answers: The Spring Framework, created by Rod Johnson in 2003, aims to simplify and expedite Java application development while ensuring safety. It is an open-source, lightweight framework comprising several sub-frameworks such as Dependency Injection, Spring MVC, and Spring JDBC, among others. This article presents Top 75 Spring Interview Questions and Answers. Spring Interview Questions for Freshers is designed to help prepare for Spring interviews and increase the chances of success.
★★ Latest Technical Interview Questions ★★
Spring Technical Interview Questions
Ace your Latest Spring Interview Questions with a curated collection of the Top 75 Interview Questions and Answers. Enhance your knowledge, stay updated, and boost your chances of success in Spring Interview Questions and Answers.
Top 75 Spring Interview Questions and Answers
1. What is the Spring Framework, and what are its key features?
The Spring Framework is a popular Java framework that provides a comprehensive programming and configuration model for Java applications. Its key features include dependency injection, aspect-oriented programming, transaction management, and MVC architecture support.
2. Explain the concept of inversion of control (IoC) in the Spring Framework.
Inversion of Control is a design principle where the control flow of a program is inverted. In the context of the Spring Framework, IoC refers to the framework managing the creation and injection of dependencies into objects, rather than the objects creating their dependencies.
3. What is dependency injection (DI) in Spring? How is it achieved?
Dependency Injection is a pattern used in software development to achieve loose coupling between components. In Spring, DI is achieved by declaring dependencies in the configuration file or using annotations such as @Autowired. The framework then injects the dependencies at runtime.
4. Differentiate between constructor injection and setter injection in Spring.
Constructor injection involves passing dependencies through a constructor, while setter injection involves using setter methods to inject dependencies. Constructor injection ensures that all required dependencies are provided during object creation, while setter injection allows for optional dependencies and easier modification.
5. What are the different modules in the Spring Framework?
There are around 20 modules which are generalized into the following types:
- Core Container
- Data Access/Integration
- Web
- AOP (Aspect Oriented Programming)
- Instrumentation
- Messaging
Test Each module provides specific functionality and can be used individually or in combination to build robust Java applications.
6. What is Spring Data JPA, and how do you use it in a Spring Boot application?
- Spring Data JPA is a subproject of the Spring Framework that simplifies working with JPA (Java Persistence API) for database interactions in Spring applications.
- To use Spring Data JPA in a Spring Boot application, you can define interfaces that extend the JpaRepository interface and leverage its built-in methods for common CRUD operations.
7. What do you mean by IoC (Inversion of Control) Container?
The Spring container serves as the central component in the Spring Framework, responsible for managing application components through Dependency Injection (DI). It creates objects, establishes their interconnections, and handles their life cycles. Configuration instructions for the Spring container can be provided using XML configuration, Java annotations, or Java code. These instructions dictate how the container should carry out its tasks.
8. Explain the Spring Boot Actuator.
- Spring Boot Actuator is a sub-project of Spring Boot that provides production-ready features for monitoring and managing Spring applications.
- It offers endpoints that expose useful information about the application, such as health, metrics, environment details, logging, and more.
9. What are the different types of bean scopes in Spring?
- The different bean scopes in Spring are Singleton, Prototype, Request, Session, and Global Session.
- Singleton creates a single instance per Spring container, Prototype creates a new instance every time it is requested, Request creates a new instance per HTTP request, Session creates a new instance per user session, and Global Session is similar to Session but for portlet-based applications.
10. What is DispatcherServlet in Spring MVC? In other words, can you explain the Spring MVC architecture?
Spring MVC framework is built around a central servlet called DispatcherServlet that handles all the HTTP requests and responses. The DispatcherServlet does a lot more than that:
- The Spring container seamlessly integrates with the IoC (Inversion of Control) container, simplifying the utilization of all Spring features.
- The DispatcherServlet is responsible for receiving requests and contacting the HandlerMapping component to determine the appropriate Controller for request processing.
- The Controller then invokes the necessary service methods to manipulate or process the Model data.
- The service layer performs the required operations on the data and returns the name of the view to the DispatcherServlet.
- The DispatcherServlet employs the ViewResolver to locate the designated view for the request.
- Once the view is determined, the DispatcherServlet passes the Model data to the View, which ultimately renders the content on the user’s browser.
11. How do you create a custom bean scope in Spring?
- To create a custom bean scope, you need to implement the Scope interface and define your custom scope’s behavior.
- Implement the methods of the Scope interface, such as
get()
,remove()
,registerDestructionCallback()
, andresolveContextualObject()
, to handle the lifecycle and retrieval of your custom-scoped beans.
12. Explain the concept of profiles in Spring.
- Profiles in Spring allow you to define and activate specific configurations based on different runtime environments or application scenarios.
- By using profiles, you can configure different sets of beans, properties, or other components for development, testing, production, or any other custom profile.
13. What does @SpringBootApplication annotation do internally?
According to the Spring Boot documentation, the @SpringBootApplication annotation serves as a consolidated replacement for using @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations with their default attributes. Instead of applying these annotations individually, @SpringBootApplication can be used as a single point of configuration.
This enables the developer to use a single annotation instead of using multiple annotations thus lessening the lines of code. However, Spring provides loosely coupled features which is why we can use these annotations as per our project needs.
14. What is the purpose of the @Value annotation in Spring?
- The @Value annotation in Spring is used to inject values into beans from various sources, such as property files, environment variables, system properties, or inline values.
- It can be used to inject primitive values, expressions, or references to other beans into fields, constructor parameters, or method parameters.
15. How do you implement caching in Spring?
- Spring provides caching support through the use of annotations such as @Cacheable, @CacheEvict, and @CachePut.
- By adding these annotations to methods, Spring can automatically cache the results of method calls and retrieve them from the cache on subsequent invocations, improving performance.
16. Explain the concept of Spring Security.
- Spring Security is a powerful framework that provides authentication, authorization, and other security features for Java applications.
- It enables developers to secure their applications at various levels, including URL-based security, method-level security, and more, with support for various authentication mechanisms like form-based, basic, OAuth, and others.
17. Difference between Docker and virtualization
Docker | Virtualization |
---|---|
Uses containerization technology | Uses hypervisor technology |
Containers share the host OS kernel | Each virtual machine has its own OS and kernel |
Lightweight and efficient | Resource-intensive and less efficient |
Faster startup and deployment | Slower startup and deployment |
More suitable for microservices architecture | More suitable for monolithic applications |
18. How do you configure authentication and authorization in Spring Security?
- Authentication and authorization in Spring Security are configured through the use of security configurations and appropriate security-related annotations.
- Authentication can be configured using authentication providers, user details services or custom authentication logic. Authorization can be defined using method-level security annotations, URL-based security configurations, or expression-based access control.
19. What is the purpose of the @Secured annotation in Spring Security?
- The @Secured annotation in Spring Security is used to apply method-level security to control access to specific methods or functionalities in an application.
- By specifying roles or privileges in the @Secured annotation, only users with the required roles or privileges can access the annotated method.
20. How does Spring support declarative transaction management?
Spring provides declarative transaction management through AOP proxies. By applying transactional annotations such as @Transactional, developers can define the scope and behavior of transactions declaratively without writing low-level transaction management code.
21. Difference between Docker containers and traditional virtual machines
Docker Containers | Traditional Virtual Machines |
---|---|
Lightweight and share host OS kernel | Each VM has its own OS and runs a full OS instance |
Faster startup time | Slower startup time |
Consumes fewer system resources | Consumes more system resources |
Better scalability and density | Lower scalability and density |
Isolation at the process level | Isolation at the hardware level |
22. What is the purpose of the @Autowired annotation in Spring?
The @Autowired annotation is used for automatic dependency injection in Spring. It allows Spring to resolve and inject dependencies into a class, eliminating the need for explicit wiring.
23. Explain the Spring Bean lifecycle.
The Spring Bean lifecycle consists of several phases, including bean instantiation, dependency injection, initialization, and destruction. During these phases, various callbacks and methods can be used to customize and manipulate the bean’s behavior.
24. What is the difference between @Component, @Service, @Repository, and @Controller annotations in Spring?
@Component is a generic stereotype annotation for any Spring-managed component. @Service is used to indicate a service component, @Repository is used for DAO (Data Access Object) components, and @Controller is used for web controllers in the MVC architecture.
25. Difference between Docker and container orchestration platforms like Kubernetes
Docker | Container Orchestration Platforms (e.g., Kubernetes) |
---|---|
Focuses on the packaging and running containers | Provides container orchestration and management capabilities |
Manages individual Docker containers | Manages clusters of containers and automates deployment |
Does not have built-in scaling capabilities | Offers automatic scaling and self-healing capabilities |
Lacks advanced networking features | Supports advanced networking and service discovery |
Does not provide built-in load balancing | Provides load balancing and traffic routing functionality |
26. How does Spring support aspect-oriented programming (AOP)?
Spring integrates AOP concepts through its AOP module. It allows developers to define aspects and apply them to specific join points in the application, enabling cross-cutting concerns such as logging, security, and caching to be implemented separately from the business logic.
27. What is the purpose of the @Autowired
annotation in Spring? Can it be used for constructor injection?
The @Autowired
annotation in Spring is used for automatic dependency injection. It allows Spring to automatically wire the beans together without explicitly using setters or constructors. Yes, it can be used for constructor injection by annotating the constructor with @Autowired
or by omitting the @Autowired
annotation if there is only one constructor.
29. Explain the difference between @RequestMapping
and @GetMapping
annotations in Spring MVC.
The @RequestMapping
annotation in Spring MVC is used to map a URL pattern to a controller method, while the @GetMapping
annotation is a shortcut for specifying a mapping for HTTP GET requests. The @GetMapping
annotation is more specific and can only be used for GET requests, whereas @RequestMapping
can handle different types of requests (GET, POST, PUT, DELETE, etc.) by specifying the appropriate method attribute.
30. Difference between Docker and traditional software packaging and deployment methods
Docker | Traditional Software Packaging and Deployment |
---|---|
Uses containerization for application packaging | Relies on manual installation and configuration |
Provides consistent and reproducible environments | The environment can vary across different systems |
Simplifies application deployment and portability | Deployment can be complex and platform-dependent |
Offers versioning and image management | Versioning and updates need to be managed separately |
Supports isolation and resource control | Limited control over resource allocation and isolation |
31. How do you implement message-driven communication using Spring Integration?
- Message-driven communication in Spring Integration is implemented using message channels, endpoints, and message-driven adapters.
- You can define channels to send and receive messages, configure message-driven endpoints, and use adapters to integrate with various messaging systems like JMS or AMQP.
32. Explain the concept of Spring Security OAuth.
- Spring Security OAuth is a module that provides support for implementing OAuth 2.0-based authentication and authorization in Spring applications.
- It allows for securing APIs and web applications by integrating with an OAuth 2.0 provider, handling token-based authentication and authorization flows.
33. How do you implement distributed transactions in Spring?
- Distributed transactions in Spring can be achieved using the Java Transaction API (JTA) and appropriate transaction managers.
- By configuring JTA transaction managers, you can coordinate transactions across multiple resources and ensure consistency in distributed environments.
34. Difference between Docker images and Docker containers
Docker Images | Docker Containers |
---|---|
Immutable and used for creating containers | Runnable instances of images |
Contains the application and its dependencies | Runs the application in an isolated environment |
Can be shared, distributed, and versioned | Created from images and can be started, stopped, and deleted |
Consumes disk space | Consumes system resources while running |
Provides the foundation for containerization | Represents a specific runtime instance of an image |
35. What is the purpose of Spring Web Services?
- Spring Web Services is a framework that simplifies the development of SOAP-based web services.
- It provides abstractions for creating web service endpoints, handling SOAP message marshaling and unmarshaling, and integrating with different web service stacks.
36. How do you consume RESTful APIs using Spring RestTemplate?
- RestTemplate is a class provided by Spring that allows for consuming RESTful APIs.
- By creating RestTemplate instances and using its methods, you can send HTTP requests, handle responses, and deserialize JSON/XML representations into Java objects.
37. What is the purpose of the @Qualifier
annotation in Spring? How does it work?
The @Qualifier
annotation is used in conjunction @Autowired
to specify which bean should be injected when there are multiple beans of the same type. By using the @Qualifier
annotation, you can provide a unique identifier for the desired bean, allowing Spring to resolve the ambiguity and inject the correct one.
38. Difference between Docker Swarm and Docker Compose
Docker Swarm | Docker Compose |
---|---|
Native Docker clustering and orchestration | Defines and runs multi-container applications on a single host |
Manages and scales services across multiple nodes | Runs and manages multiple containers on a single host |
Offers automated service discovery and load balancing | Does not provide built-in service discovery or load balancing |
Supports high availability and fault tolerance | Designed for development and testing environments |
Suitable for production environments | Suitable for local development and single-host deployments |
39. Explain the usage of the @Transactional
annotation in Spring.
The @Transactional
annotation is used to define the transactional boundaries for methods in Spring. When a method is annotated with @Transactional
, Spring ensures that the method is executed within a transaction context. If an exception occurs, the transaction is rolled back, and if the method completes successfully, the transaction is committed.
40. What is the purpose of the @ComponentScan
annotation in Spring? How is it used?
The @ComponentScan
annotation is used to enable component scanning in Spring. It tells Spring where to look for Spring-managed components such as controllers, services, and repositories. By specifying the base package(s) to scan, Spring will automatically detect and register the annotated classes as beans in the application context.
41. Explain the usage of the @PathVariable
annotation in Spring MVC.
The @PathVariable
annotation is used to bind a method parameter to a URI template variable in Spring MVC. It allows you to extract a specific portion of the request URL and use it as a method argument. By annotating a method parameter with @PathVariable
, Spring will automatically populate its value based on the corresponding variable in the URL.
42. Difference between Docker volumes and bind mounts
Docker Volumes | Bind Mounts |
---|---|
Managed by Docker, independent of containers | Linked to a specific path on the host filesystem |
Can be shared among multiple containers | Tied to a specific container or host |
Supports data persistence and can be backed up | No inherent data persistence or backup capability |
Offers more control over data management | Simpler and easier to set up |
Provides better performance for data-intensive operations | Performs similarly to host filesystem operations |
43. What is the purpose of the @Value
annotation in Spring? How is it used?
The @Value
annotation in Spring is used to inject values from properties files or other sources into bean properties. It can be used to inject simple values, such as strings or numbers, as well as complex objects. By specifying the key or expression within @Value
, Spring will resolve and inject the corresponding value into the annotated field or method parameter.
44. Explain the usage of the @ExceptionHandler
annotation in Spring MVC.
The @ExceptionHandler
annotation is used to handle exceptions in Spring MVC. By annotating a method with @ExceptionHandler
and specifying the exception type(s) it handles, Spring will invoke that method whenever an exception of the specified type occurs within the controller. This allows you to centralize the handling of exceptions and return appropriate responses to the client.
45. Difference between Docker and serverless computing
Docker | Serverless Computing |
---|---|
Provides containerization for applications | Allows running code in a serverless execution environment |
Requires managing and maintaining the underlying infrastructure | Abstracts away infrastructure management |
Offers more control and flexibility over the application environment | Provides a managed runtime environment |
Suitable for long-running and persistent applications | Ideal for event-driven and short-lived functions |
Requires manual scaling and resource management | Autoscales and dynamically allocates resources |
46. What is the purpose of the @Bean
annotation in Spring configuration classes?
The @Bean
annotation is used to declare a bean in a Spring configuration class. By annotating a method, you instruct Spring to create a bean of the specified type and register it in the application context. The method can also contain the logic to configure and initialize the bean before returning it.
47. Explain the usage of the @Async
annotation in Spring.
The @Async
annotation is used to enable asynchronous method execution in Spring. By annotating a method with @Async
, Spring will execute that method in a separate thread, allowing it to run concurrently with other methods. This is particularly useful for time-consuming tasks that don’t require immediate results, improving the overall responsiveness and scalability of the application
48. Difference between Docker and containerization technologies like LXC or LXD
Docker | LXC/LXD |
---|---|
Focuses on application-centric containerization | Offers system-level containerization |
Uses layered images for efficient storage and sharing | Uses full machine containers for isolation and security |
Provides extensive tooling and ecosystem | Offers a more lightweight and minimalistic approach |
Emphasizes portability across different environments | Designed for running Linux containers on Linux hosts |
Optimized for running distributed microservices | Suited for running system-level services and workloads |
49. How do you handle exceptions in Spring MVC?
In Spring MVC, exceptions can be handled using the @ExceptionHandler annotation to define methods that handle specific exceptions or by implementing the HandlerExceptionResolver interface to provide custom exception-handling logic.
50. What is the purpose of the @RequestMapping annotation in Spring MVC?
The @RequestMapping annotation is used to map HTTP requests to specific methods in a controller class. It allows developers to define the URL pattern, HTTP methods, and other request parameters to handle incoming requests.
51. Explain the Model-View-Controller (MVC) architecture in Spring MVC.
In Spring MVC, the MVC architecture separates the application into three components: the Model represents the data, the View renders the UI, and the Controller handles the incoming requests, processes them, and interacts with the Model and View to generate the response.
52. Difference between Docker and containerization platforms like Docker and Podman
Docker | Podman |
---|---|
Has a client-server architecture with a daemon | Works as a daemon less container engine |
Relies on a centralized image registry | Uses local image management and distribution |
Requires root or sudo access for running containers | Can run containers as a non-root user |
Provides a full ecosystem with extensive tooling and support | Offers a lightweight and standalone container engine |
Primarily used in development and production environments | Gaining popularity for local development and testing |
53. What is the purpose of the DispatcherServlet in Spring MVC?
The DispatcherServlet acts as the front controller in Spring MVC. It receives incoming requests, dispatches them to the appropriate controller, and manages the overall request processing flow.
54. What is the difference between @PathVariable and @RequestParam annotations in Spring MVC?
@PathVariable is used to extract values from the URI path of the request, while @RequestParam is used to retrieve values from query parameters or form data. @PathVariable is typically used for RESTful APIs, while @RequestParam is more suitable for traditional form-based requests.
55. How do you implement validation in Spring MVC?
Spring MVC provides validation support through the use of java. validation API and annotations such as @Valid. By applying validation annotations to model attributes or request parameters, Spring can automatically perform validation and handle validation errors.
56. Explain the concept of RESTful web services in Spring MVC.
RESTful web services in Spring MVC follow the principles of the Representational State Transfer (REST) architectural style. They use HTTP methods, such as GET, POST, PUT, and DELETE, to perform CRUD operations on resources and return responses in a stateless manner.
57. How do you handle file uploads in Spring MVC?
File uploads in Spring MVC can be handled using the MultipartFile interface. By using the @RequestParam annotation with the “multipart/form-data” encoding type, files can be received and processed in the controller method.
58. What is Spring Boot, and what are its advantages?
Spring Boot is a framework that simplifies the development and deployment of Spring-based applications. It provides auto-configuration, embedded servers, production-ready features, and streamlined dependency management, allowing developers to focus on writing business logic rather than boilerplate configuration.
59. How does Spring Boot simplify the configuration of Spring applications?
Spring Boot eliminates the need for manual configuration by leveraging convention-over-configuration principles and providing sensible defaults. It automatically configures beans, data sources, web servers, and other components based on the project’s classpath and dependencies
60. Explain the concept of internationalization (i18n) and localization (l10n) in Spring.
- Internationalization (i18n) is the process of designing an application to support multiple languages and cultures.
- Localization (l10n) is the process of adapting the application to a specific locale, including language translation, date and number formatting, and other locale-specific adjustments. Spring provides support for i18n and l10n through the use of message bundles, locale resolvers, and localization annotations.
61. How do you handle asynchronous processing in Spring?
- Spring supports asynchronous processing through the use of the @Async annotation and the TaskExecutor interface.
- By annotating methods with @Async, Spring creates a proxy for the method and executes it asynchronously, allowing for non-blocking execution and improved application performance.
62. Explain the concept of Spring Integration.
- Spring Integration is a framework that enables the integration of different systems and components within an application using messaging-based communication.
- It provides a set of building blocks, such as channels, message handlers, transformers, and routers, to create scalable and modular integration solutions.
63. How do you schedule tasks using Spring’s scheduling support?
- Spring’s scheduling support allows for the scheduling and execution of tasks at specific times or intervals.
- You can use annotations such as @Scheduled or configure task scheduling programmatically using the TaskScheduler interface.
64. What is Spring Batch, and how do you use it?
- Spring Batch is a lightweight, scalable framework for batch processing in Java applications.
- It provides support for reading, processing, and writing large volumes of data in a batch-oriented manner. You can define batch jobs using job configurations, steps, and item readers/writers.
65. Explain the purpose of Spring WebFlux.
- Spring WebFlux is a non-blocking, reactive web framework introduced in Spring 5.
- It allows for building web applications with support for high-performance, asynchronous request processing using reactive programming principles.
66. How do you handle WebSocket communication in Spring?
- Spring provides WebSocket support through the use of the WebSocket API and the implementation of WebSocketHandler.
- You can configure WebSocket endpoints, handle incoming WebSocket messages, and broadcast messages to connected clients.
67. What is the purpose of the @Async annotation in Spring?
- The @Async annotation in Spring is used to indicate that a method should be executed asynchronously.
- It allows for the concurrent execution of methods, offloading the processing to separate threads or thread pools.
68. How do you configure logging in Spring applications?
- Spring applications can be configured to use various logging frameworks such as Logback or Log4j.
- By adding the necessary dependencies and configuration files, you can control logging levels, log formatting, and log output destinations.
69. Explain the concept of Spring Cloud and its components.
- Spring Cloud is a collection of tools and frameworks that provide support for building distributed systems and cloud-native applications.
- Its components include service discovery (Eureka), API gateway (Zuul or Spring Cloud Gateway), circuit breaker (Hystrix or Resilience4j), configuration management (Spring Cloud Config), and more.
70. How do you implement service discovery using Spring Cloud?
- Service discovery in Spring Cloud is typically achieved using the Eureka server, which acts as a registry for services.
- Services register themselves with the Eureka server, and clients can discover and communicate with the registered services through the Eureka client.
71. What is the purpose of the @EnableCircuitBreaker annotation in Spring Cloud?
- The @EnableCircuitBreaker annotation enables the circuit breaker pattern in a Spring Cloud application.
- It allows for fault tolerance and resilience by wrapping remote service invocations with circuit breaker logic to handle failures and fallback mechanisms.
72. How do you implement distributed tracing in Spring Cloud?
- Distributed tracing in Spring Cloud is commonly implemented using tools like Zipkin or Spring Cloud Sleuth.
- By integrating these tools, you can trace requests across multiple services and gather timing and correlation information for better visibility and troubleshooting.
73. Explain the concept of Spring Cloud Config Server.
- Spring Cloud Config Server is a centralized configuration management tool that provides externalized configuration for Spring applications.
- It allows for storing configuration files in a central repository and provides a RESTful API for retrieving the configuration properties at runtime.
74. How do you implement load balancing in Spring Cloud?
- Spring Cloud provides load-balancing capabilities through integrations with load-balancing libraries such as Netflix Ribbon or Spring Cloud LoadBalancer.
- By configuring client-side load balancing, requests to a service are automatically distributed across multiple instances to achieve better performance and high availability.
75. What is the purpose of the Spring Cloud Gateway?
- Spring Cloud Gateway is a lightweight, API-centric gateway that provides routing, filtering, and load-balancing functionalities for microservices.
- It acts as an entry point for client requests and can handle common cross-cutting concerns such as authentication, rate limiting, and request transformation.
Boost your Spring interview preparation with our curated collection of the Top 75 Spring Interview Questions and Answers. Stay up-to-date and broaden your knowledge by visiting us at freshersnow.com. Approach your Spring job interview with confidence and excel in showcasing your skills and expertise