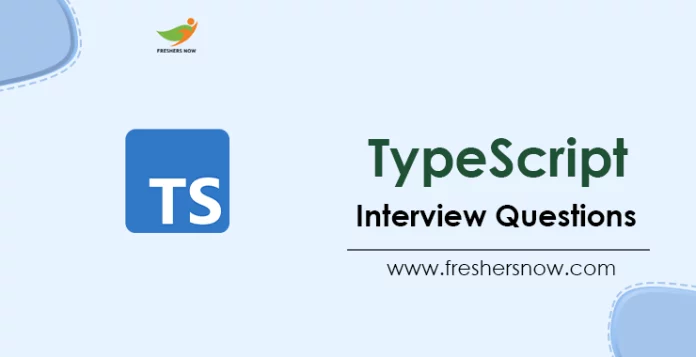
TypeScript Technical Interview Questions: TypeScript is a programming language that is open-source and was developed by Microsoft’s Anders Hejlsberg. It is a statically-typed language that extends the functionality of JavaScript and compiles it to plain JavaScript. It offers advanced features like IntelliSense, code completion, safe refactorings, and other developer-friendly tools.
To ace a TypeScript interview, it’s crucial to understand the Latest TypeScript Interview Questions. we have compiled a comprehensive list of the Top 50 TypeScript Interview Questions and Answers.
Latest TypeScript Interview Questions
Our list of Top 50 TypeScript Interview Questions and Answers is designed for both novice and experienced professionals and covers the TypeScript Interview Questions for Freshers that are currently relevant in the job market. It is an ideal resource for anyone looking to prepare for a TypeScript Technical Interview Questions, from beginners to experts.
Top 50 TypeScript Interview Questions and Answers
1. What is TypeScript and how is it different from JavaScript?
TypeScript is a superset of JavaScript that provides additional features to the language, including static typing and object-oriented programming concepts. TypeScript is compiled into JavaScript, which means that it can be used in any environment that supports JavaScript.
2. What are the primitive types in TypeScript?
- string: represents text values such as “javascript”, “typescript”, etc.
- number: represents numeric values like 1, 2, 32, 43, etc.
- boolean: represents a variable that can have either a ‘true’ or ‘false’ value.
JavaScript is a dynamic and loosely typed language, meaning that developers don’t have to specify the data type of a variable when they declare it. In contrast, TypeScript allows developers to specify the data type of a variable or function parameter using static typing.
3. Explain the concept of static typing in TypeScript.
Static typing is a concept in programming that requires variables, functions, and other elements of a program to be defined with a specific data type before they can be used. This helps to prevent errors and improve the maintainability of the code. In TypeScript, developers can specify the data type of a variable or function parameter using a colon followed by the type, for example:
let myNumber: number = 5; function addNumbers(a: number, b: number): number { return a + b; }
4. What are some of the features of TypeScript that make it popular among developers?
Some of the features of TypeScript that make it popular among developers include:
Optional static typing, which allows developers to gradually add types to their code as they see fit
Support for modern JavaScript features like classes, modules, and arrow functions
Better tooling and error checking, thanks to the TypeScript compiler
Improved readability and maintainability of code due to the use of types and interfaces
5. What is the difference between TypeScript and JavaScript, and why would you choose one over the other?
TypeScript | JavaScript |
---|---|
TypeScript is a superset of JavaScript that adds static typing and other features to the language. | JavaScript is a dynamic, interpreted language that is widely used for client-side scripting on the web. |
TypeScript allows for easier code maintenance, better tooling support, and improved error checking. | JavaScript is more flexible and easier to learn, making it a good choice for smaller projects or rapid prototyping. |
TypeScript code needs to be compiled into JavaScript before it can run in a browser or on a server. | JavaScript can be executed directly in a browser or on a server using Node.js. |
6. What are the different components of TypeScript?
TypeScript has mainly three components. These are-
Language
TypeScript enables developers to write code using the new syntax, keywords, and type annotations and provides better tooling and error-checking capabilities for more scalable and maintainable code.
Compiler
The TypeScript compiler, written in TypeScript, is open source and cross-platform. It transforms TypeScript code into equivalent JavaScript code, performing parsing and type checking. It can also concatenate multiple files into a single output file and generate source maps for better debugging.
Language Service
The language service in TypeScript offers information to editors and other tools, allowing for better assistance features, including automated refactoring and IntelliSense. It provides useful information, such as code completion suggestions, syntax highlighting, and error detection, to improve the development experience and facilitate clean and efficient code writing.
7. How do you define a variable in TypeScript?
In TypeScript, variables can be defined using the let or const keywords, like in JavaScript. For example:
let myString: string = "Hello, world!"; const number: number = 42; let x = 1; if (true) { let x = 2; // this is a different variable than the one defined above } console.log(x); // logs 1, not 2 }
8. How do you define a function in TypeScript?
Functions can be defined in TypeScript using the same syntax as in JavaScript, with the addition of static typing for the function parameters and return type, if desired. For example:
function and numbers(a: number, b: number): number { return a + b; } Here's an example: interface Person { firstName: string; lastName: string; age: number; email?: string; // optional property } let myPerson: Person = { firstName: "John", lastName: "Doe", age: 30, };
9. What is the difference between the “let” and “var” keywords in TypeScript
The “let” keyword declares a block-scoped variable, while the “var” keyword declares a variable with function-level scope.
10. List some features of Typescript?
11. How does TypeScript’s static typing differ from dynamic typing in JavaScript, and what are the advantages and disadvantages of each approach?
Static Typing | Dynamic Typing |
---|---|
Variables have types that are checked at compile-time. | Variables have types that are checked at runtime. |
Type errors can be caught early in the development process before the code is run. | Type errors may not be caught until the code is executed, which can lead to unexpected behavior. |
Code is more self-documenting, as types provide a clear indication of what a variable should be used for. | Code can be more flexible and adaptable to changing requirements, as types do not need to be specified in advance. |
12. How do you define a type alias in TypeScript?
To define a type alias in TypeScript, use the “type” keyword followed by the alias name and the type definition. For example: type MyType = string | number;
13. What are the object-oriented terms supported by TypeScript?
TypeScript supports the following object-oriented terms:
- Modules
- Classes
- Interfaces
- Inheritance
- Data Types
- Member functions
14. What is the purpose of the “?” operator in TypeScript?
The “?” operator denotes that a property or method is optional in an interface or a class. For example: interface MyInterface { optionalProp?: string; }
15. What are all the JSX modes TypeScript supports?
In TypeScript, the preserve mode retains the JSX as part of the output to be used by another transform step, resulting in a .jsx file extension. The react mode, on the other hand, outputs React.createElement directly and does not require a JSX transformation beforehand. This mode results in a .js file extension. The react-native mode is similar to the preserve mode, retaining all JSX, but generating a .js file extension instead.
16. How do you declare a function type in TypeScript?
To declare a function type in TypeScript, use the arrow function syntax followed by the return type. For example: (a: number, b: number) => number;
17. What is a namespace in TypeScript, and how can it be used to organize and encapsulate code?
Usage | Benefits |
---|---|
A way to group related functionality under a single, globally unique identifier. | Prevents naming conflicts between different parts of the application. |
Can be used to split code into modules or to encapsulate functionality within a class or object. | Makes it easier to reason about the code by grouping related functionality together. |
Can be used to declare global variables and functions, or to import and export functionality between modules. | Makes it easier to maintain and modify the codebase by providing clear boundaries between different parts of the application |
18. What is a union type in TypeScript?
A union type allows a variable or parameter to accept multiple types of values. For example: let myVar: string | number;
19. How do you define an interface with optional properties in TypeScript?
To define an interface with optional properties, use the “?” operator after the property name. For example: interface MyInterface { optionalProp?: string; }
20. What are generics in TypeScript, and how are they used to provide type safety and code reusability?
Usage | Benefits |
---|---|
A way to define type parameters that can be reused across multiple functions and classes. | Makes code more flexible and adaptable by allowing for the creation of generic functions and classes that can work with different types of data. |
Can be used to provide type safety in function parameters and return values. | Reduces the need to write redundant code for similar types of data. |
Can be used to define constraints on type parameters, such as requiring that they implement a certain interface or extend a certain class. | Improves code organization and maintainability by allowing for the creation of reusable code libraries that can be shared across multiple projects. |
21. What is the difference between an interface and a type in TypeScript?
An interface is a contract that defines a set of properties and methods that a class or an object must implement, while a type is a synonym for a specific type definition.
22. What is the difference between let
and const
in TypeScript, and when should you use each one?
let |
const |
---|---|
Used to declare a variable whose value can be reassigned. | Used to declare a variable whose value cannot be reassigned. |
The variable can be initialized later in the code. | The variable must be initialized when it is declared. |
Can be used for variables that are expected to change over time. | Should be used for variables that are expected to remain constant. |
23. How do you declare an array type in TypeScript?
To declare an array type in TypeScript, use the square brackets syntax followed by the array type definition. For example: let myArr: string[];
24. What is a tuple in TypeScript?
A tuple is a fixed-length array with predefined types for each element. For example: let myTuple: [string, number];
25. How does the this
keyword work in TypeScript, and how can it be used to refer to the current object instance?
Usage | Description |
---|---|
Used to refer to the current object instance within a class method. | Can be used to access properties and methods of the current object, or to pass the object to other methods or functions. |
Can be used in arrow functions to preserve the context of the current object. | Must be used within a class method or object context, otherwise, it will be undefined. |
26. How do you define an enum in TypeScript?
To define an enum in TypeScript, use the “enum” keyword followed by the enum name and its members. For example: enum MyEnum { First = 1, Second = 2, Third = 3 }
27. What are generics in TypeScript and how are they used?
Generics in TypeScript allow developers to create reusable components that can work with different data types. They provide a way to define a type parameter that can be used throughout a class or function, allowing it to work with any data type. Here’s an example of how generics can be used:
function reverseArray<T>(array: T[]): T[] { return array.reverse(); } let myArray: number[] = [1, 2, 3]; let reversedArray: number[] = reverseArray(myArray);
In this example, the reverse array function uses the type parameter T to define the data type of the input array and the return value. The function can be called with any type of array, as long as the data type of the elements in the array matches.
28. What are some of the key features of TypeScript that make it popular among developers, and how do these features enhance development productivity?
Key Features | Benefits |
---|---|
Static typing | Helps catch errors early in the development process |
Object-oriented programming constructs (classes, interfaces, inheritance, polymorphism) | Encourages modularity and code reuse |
Type inference | Reduces the need to explicitly specify types |
Optional parameters and default values | Simplify function signatures and reduce boilerplate code |
29. How do you define a class in TypeScript?
Classes in TypeScript are defined using the class keyword, along with properties, methods, and constructors. Here’s an example:
class Person { firstName: string; lastName: string; constructor(firstName: string, lastName: string) { this.firstName = firstName; this.lastName = lastName; } getFullName(): string { return `${this.firstName} ${this.lastName}`; } } let myPerson = new Person("John", "Doe"); console.log(myPerson.getFullName()); // logs "John Doe"
30. How does TypeScript support inheritance and polymorphism?
TypeScript supports inheritance and polymorphism through the use of classes and interfaces. Inheritance allows a subclass to inherit properties and methods from a parent class, while polymorphism allows objects to be treated as instances of their parent class or any of their parent’s interfaces. Here’s an example:
class Animal { name: string; constructor(name: string) { this.name = name; } makeSound() { console.log("Animal sound"); } } class Cat extends Animal { constructor(name: string) { super(name); } makeSound() { console.log("Meow"); } } let myAnimal: Animal = new Cat("Fluffy"); myAnimal.makeSound(); // logs "Meow"
In this example, the Cat class inherits from the Animal class and overrides the makeSound method. The minimal variable is declared as type Animal, but it can still be assigned a new instance of the Cat class and called an instance of the parent class.
31. How does TypeScript handle inheritance and polymorphism, and what are some of the benefits of using these object-oriented programming concepts?
Inheritance | Polymorphism |
---|---|
Allows a class to inherit properties and methods from a parent class. | Allows objects of different types to be treated as instances of a common type. |
Encourages code reuse and modularity by allowing classes to share common functionality. | Makes code more flexible and adaptable to changing requirements by allowing for the creation of extensible code frameworks. |
Can help improve code organization and maintainability by grouping related functionality into hierarchical class structures. | Encourages more modular and testable code by enabling the use of interfaces and abstract classes. |
32. What is the use of access modifiers in TypeScript?
Access modifiers in TypeScript allow developers to control the visibility and accessibility of class members, such as properties and methods. TypeScript supports three access modifiers: public, private, and protected.
33. How do you implement a private member in a TypeScript class?
A private member in a TypeScript class can be defined using the private keyword before the member name. Here’s an example:
class Person { private age: number; constructor(age: number) { this.age = age; } }
In this example, the age property is private and can only be accessed within the Person class.
34. How do you implement a public member in a TypeScript class?
A public member in a TypeScript class is the default and doesn’t require any keyword before the member name. Here’s an example:
class Person { firstName: string; lastName: string; constructor(firstName: string, lastName: string) { this.firstName = firstName; this.lastName = lastName; } }
35. How can you integrate TypeScript with a popular frontend framework like Angular?
To integrate TypeScript with Angular, you first need to install the TypeScript compiler using npm. Once TypeScript is installed, you can start writing Angular components in TypeScript by creating .ts files instead of .js files. TypeScript can also be used to create Angular services, directives, and pipes. Additionally, Angular provides built-in support for TypeScript through its command-line interface (CLI), which can generate TypeScript code for components, services, and other Angular constructs.
36. What are access modifiers in TypeScript, and how are they used to control access to class members?
Access Modifiers | Description |
---|---|
public |
Members are accessible from anywhere. This is the default for all class members. |
private |
Members are only accessible from within the class that defines them. |
protected |
Members are accessible from within the class that defines them, as well as any subclasses that extend the class. |
37. How do you use a namespace in TypeScript?
Namespaces in TypeScript allow for organizing code into logical containers. To use a namespace, you declare it using the namespace keyword, and then export its contents using the export keyword.
38. What is a module in TypeScript?
In TypeScript, a module is a way to organize code into separate files and namespaces, allowing for better code reuse and maintainability.
39. How do you use a module in TypeScript?
To use a module in TypeScript, you export its contents using the export keyword and then import it into another module using the import keyword.
40. How do you import and export modules in TypeScript?
To export a module, you use the export keyword before the module’s declaration. To import a module, you use the import keyword followed by the module’s path.
41. What are the primitive types in TypeScript?
The primitive types in TypeScript are boolean, number, string, null, undefined, and symbol.
42. What is a Promise in TypeScript?
A Promise in TypeScript is an object representing the eventual completion or failure of an asynchronous operation, typically a function that returns a value asynchronously.
43. How do you use a Promise in TypeScript?
To use a Promise in TypeScript, you can create a new instance of the Promise object, passing in a function that performs the asynchronous operation. You can then use the then-and-catch methods to handle the eventual completion or failure of the operation.
44. What is an enum in TypeScript?
An enum in TypeScript is a way to define a set of named constants. Each constant is assigned an underlying numeric value, which can be used to compare and order the constants.
45. How do you use an enum in TypeScript?
To use an enum in TypeScript, you declare it using the enum keyword, and then use its constants as needed in your code.
46. What is the role of a type alias in TypeScript?
A type alias in TypeScript is a way to create a new name for an existing type. It can be used to simplify complex types, provide more descriptive type names, or create reusable types.
47. What is the role of a type alias in TypeScript?
A type alias is used to create a new name for an existing type or to define a complex type. It helps to simplify complex types and make them easier to read and use.
48. How do you define a type alias in TypeScript?
To define a type alias, use the “type” keyword followed by the new name you want to create and the existing type you want to alias. For example, to create a type alias for a complex object type, you can do:
type MyTypeAlias = { name: string; age: number; address: string; }
49. What is the difference between an interface and a type alias in TypeScript?
The main difference between an interface and a type alias is that an interface is a declaration that defines a set of related properties and methods, while a type alias is just an alias for an existing type. Interfaces can also be implemented by classes, while type aliases cannot. Additionally, interfaces are generally preferred for defining object shapes, while type aliases are preferred for defining complex types and unions.
50. How does TypeScript support type inference?
TypeScript supports type inference by analyzing the values and expressions in the code to automatically determine their types. This allows developers to write code that is more concise and easier to read, while still benefiting from the safety and correctness guarantees of static typing.
This article covers the Top 50 TypeScript Interview Questions and Answers, which can be valuable for candidates preparing for TypeScript technical interviews. Following daily updates on freshersnow.com is also recommended to stay informed.